Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / EventHandlerService.cs / 1 / EventHandlerService.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Diagnostics; using System; using System.Windows.Forms; ////// /// /// public sealed class EventHandlerService : IEventHandlerService { // We cache the last requested handler for speed. // private object lastHandler; private Type lastHandlerType; // The handler stack // private HandlerEntry handlerHead; // Our change event // private EventHandler changedEvent; private readonly Control focusWnd; ////// /// public EventHandlerService(Control focusWnd) { this.focusWnd = focusWnd; } ///[To be supplied.] ////// /// public event EventHandler EventHandlerChanged { add { changedEvent += value; } remove { changedEvent -= value; } } ///[To be supplied.] ////// /// public Control FocusWindow { get { return focusWnd; } } ///[To be supplied.] ////// /// public object GetHandler(Type handlerType) { if (handlerType == lastHandlerType) { return lastHandler; } for (HandlerEntry entry = handlerHead; entry != null; entry = entry.next) { if (entry.handler != null && handlerType.IsInstanceOfType(entry.handler)) { lastHandlerType = handlerType; lastHandler = entry.handler; return entry.handler; } } return null; } ////// Gets the currently active event handler of the specified type. ////// /// Fires an OnEventHandlerChanged event. /// private void OnEventHandlerChanged(EventArgs e) { if (changedEvent != null) { ((EventHandler)changedEvent)(this, e); } } ////// /// public void PopHandler(object handler) { for (HandlerEntry entry = handlerHead; entry != null; entry = entry.next) { if (entry.handler == handler) { handlerHead = entry.next; lastHandler = null; lastHandlerType = null; OnEventHandlerChanged(EventArgs.Empty); return; } } Debug.Assert(handler == null || handlerHead == null, "Failed to locate handler to remove from list."); } ////// Pops /// the given handler off of the stack. ////// /// public void PushHandler(object handler) { handlerHead = new HandlerEntry(handler, handlerHead); // Update the handlerType if the Handler pushed is the same type as the last one .... // This is true when SplitContainer is on the form and Edit Properties pushed another handler. lastHandlerType = handler.GetType(); lastHandler = handlerHead.handler; OnEventHandlerChanged(EventArgs.Empty); } ///Pushes a new event handler on the stack. ////// /// Contains a single node of our handler stack. We typically /// have very few handlers, and the handlers are long-living, so /// I just implemented this as a linked list. /// private sealed class HandlerEntry { public object handler; public HandlerEntry next; ////// /// Creates a new handler entry objet. /// public HandlerEntry(object handler, HandlerEntry next) { this.handler = handler; this.next = next; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
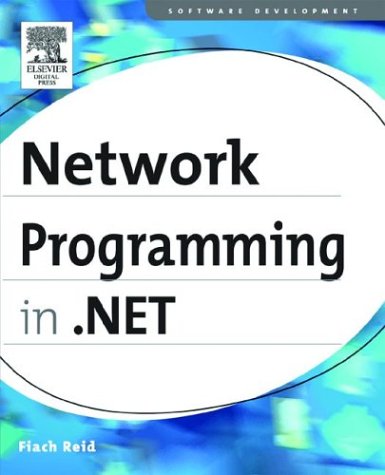
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsFormsLinkLabel.cs
- TextTreeUndo.cs
- SHA1.cs
- RegexStringValidator.cs
- RIPEMD160.cs
- WaitHandleCannotBeOpenedException.cs
- RequestCachePolicy.cs
- ObjectManager.cs
- PingOptions.cs
- BezierSegment.cs
- DelimitedListTraceListener.cs
- ToolBar.cs
- XmlSchemaGroupRef.cs
- StoreItemCollection.cs
- ListViewItem.cs
- EventDescriptor.cs
- OracleCommandSet.cs
- LogicalExpr.cs
- RuntimeEnvironment.cs
- ProjectionPruner.cs
- DSGeneratorProblem.cs
- UnmanagedMemoryStreamWrapper.cs
- WinFormsComponentEditor.cs
- NamedElement.cs
- TeredoHelper.cs
- RSAPKCS1KeyExchangeFormatter.cs
- COM2FontConverter.cs
- XmlSchemaIdentityConstraint.cs
- ImageIndexConverter.cs
- Double.cs
- RoleGroupCollection.cs
- HttpStaticObjectsCollectionBase.cs
- WebPartTransformerCollection.cs
- TextRange.cs
- AttachmentCollection.cs
- QEncodedStream.cs
- ScrollBarAutomationPeer.cs
- XmlSchemaFacet.cs
- COM2ExtendedBrowsingHandler.cs
- NetworkInformationException.cs
- EntityDataSourceWrapper.cs
- MenuEventArgs.cs
- WorkflowMarkupSerializationException.cs
- TextLine.cs
- FillRuleValidation.cs
- NativeMethods.cs
- HttpDebugHandler.cs
- MatrixAnimationBase.cs
- DigestComparer.cs
- SafeSecurityHandles.cs
- ListenerBinder.cs
- ObjectTypeMapping.cs
- FontFamilyConverter.cs
- ObjectTag.cs
- Polyline.cs
- ResourceProviderFactory.cs
- RoutedEventValueSerializer.cs
- VersionedStreamOwner.cs
- Translator.cs
- AnchoredBlock.cs
- DateBoldEvent.cs
- XmlEntity.cs
- EdmType.cs
- ByteKeyFrameCollection.cs
- PropVariant.cs
- DictionaryBase.cs
- FileRegion.cs
- PerformanceCounterLib.cs
- _Semaphore.cs
- Stackframe.cs
- InvalidAsynchronousStateException.cs
- DodSequenceMerge.cs
- CheckBoxStandardAdapter.cs
- FormatException.cs
- ConsumerConnectionPointCollection.cs
- Profiler.cs
- GetCardDetailsRequest.cs
- OracleTimeSpan.cs
- ColorConvertedBitmap.cs
- HtmlInputFile.cs
- ContextMenuStripGroupCollection.cs
- GenerateTemporaryAssemblyTask.cs
- FontStyle.cs
- DataGridItem.cs
- DirectionalLight.cs
- RootBrowserWindow.cs
- TextOnlyOutput.cs
- WebUtil.cs
- TaiwanCalendar.cs
- DeferredElementTreeState.cs
- WindowsProgressbar.cs
- _ConnectOverlappedAsyncResult.cs
- HttpCapabilitiesSectionHandler.cs
- AutomationTextAttribute.cs
- MultiPageTextView.cs
- safemediahandle.cs
- Attributes.cs
- PreloadHost.cs
- Point3DCollectionValueSerializer.cs
- MediaEntryAttribute.cs