Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Imaging / ColorConvertedBitmap.cs / 1 / ColorConvertedBitmap.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: ColorConvertedBitmap.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region ColorConvertedBitmap ////// ColorConvertedBitmap provides caching functionality for a BitmapSource. /// public sealed partial class ColorConvertedBitmap : Imaging.BitmapSource, ISupportInitialize { ////// Constructor /// public ColorConvertedBitmap() : base(true) { } ////// Construct a ColorConvertedBitmap /// /// Input BitmapSource to color convert /// Source Color Context /// Destination Color Context /// Destination Pixel format public ColorConvertedBitmap(BitmapSource source, ColorContext sourceColorContext, ColorContext destinationColorContext, PixelFormat format) : base(true) // Use base class virtuals { if (source == null) { throw new ArgumentNullException("source"); } if (sourceColorContext == null) { throw new ArgumentNullException("sourceColorContext"); } if (destinationColorContext == null) { throw new ArgumentNullException("destinationColorContext"); } _bitmapInit.BeginInit(); Source = source; SourceColorContext = sourceColorContext; DestinationColorContext = destinationColorContext; DestinationFormat = format; _bitmapInit.EndInit(); FinalizeCreation(); } // ISupportInitialize ////// Prepare the bitmap to accept initialize paramters. /// public void BeginInit() { WritePreamble(); _bitmapInit.BeginInit(); } ////// Prepare the bitmap to accept initialize paramters. /// ////// Critical - access critical resources /// PublicOK - All inputs verified /// [SecurityCritical ] public void EndInit() { WritePreamble(); _bitmapInit.EndInit(); if (Source == null) { throw new InvalidOperationException(SR.Get(SRID.Image_NoArgument, "Source")); } if ( SourceColorContext == null) { throw new InvalidOperationException(SR.Get(SRID.Color_NullColorContext)); } if (DestinationColorContext == null) { throw new InvalidOperationException(SR.Get(SRID.Image_NoArgument, "DestinationColorContext")); } FinalizeCreation(); } private void ClonePrequel(ColorConvertedBitmap otherColorConvertedBitmap) { BeginInit(); } private void ClonePostscript(ColorConvertedBitmap otherColorConvertedBitmap) { EndInit(); } /// /// Create the unmanaged resources /// ////// Critical - access critical resource /// [SecurityCritical] internal override void FinalizeCreation() { _bitmapInit.EnsureInitializedComplete(); BitmapSourceSafeMILHandle wicConverter = null; HRESULT.Check(UnsafeNativeMethods.WICCodec.CreateColorTransform( out wicConverter)); lock (_syncObject) { Guid fmtDestFmt = DestinationFormat.Guid; HRESULT.Check(UnsafeNativeMethods.WICColorTransform.Initialize( wicConverter, Source.WicSourceHandle, SourceColorContext.ColorContextHandle, DestinationColorContext.ColorContextHandle, ref fmtDestFmt)); } WicSourceHandle = wicConverter; _isSourceCached = Source.IsSourceCached; CreationCompleted = true; UpdateCachedSettings(); } ////// Notification on source changing. /// private void SourcePropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { BitmapSource newSource = e.NewValue as BitmapSource; _source = newSource; _syncObject = (newSource != null) ? newSource.SyncObject : _bitmapInit; } } ////// Notification on source colorcontext changing. /// private void SourceColorContextPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _sourceColorContext = e.NewValue as ColorContext; } } ////// Notification on destination colorcontext changing. /// private void DestinationColorContextPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _destinationColorContext = e.NewValue as ColorContext; } } ////// Notification on destination format changing. /// private void DestinationFormatPropertyChangedHook(DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { _destinationFormat = (PixelFormat)e.NewValue; } } ////// Coerce Source /// private static object CoerceSource(DependencyObject d, object value) { ColorConvertedBitmap bitmap = (ColorConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._source; } else { return value; } } ////// Coerce SourceColorContext /// private static object CoerceSourceColorContext(DependencyObject d, object value) { ColorConvertedBitmap bitmap = (ColorConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._sourceColorContext; } else { return value; } } ////// Coerce DestinationColorContext /// private static object CoerceDestinationColorContext(DependencyObject d, object value) { ColorConvertedBitmap bitmap = (ColorConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._destinationColorContext; } else { return value; } } ////// Coerce DestinationFormat /// private static object CoerceDestinationFormat(DependencyObject d, object value) { ColorConvertedBitmap bitmap = (ColorConvertedBitmap)d; if (!bitmap._bitmapInit.IsInInit) { return bitmap._destinationFormat; } else { return value; } } #region Data members private BitmapSource _source; private ColorContext _sourceColorContext; private ColorContext _destinationColorContext; private PixelFormat _destinationFormat; #endregion } #endregion // ColorConvertedBitmap } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
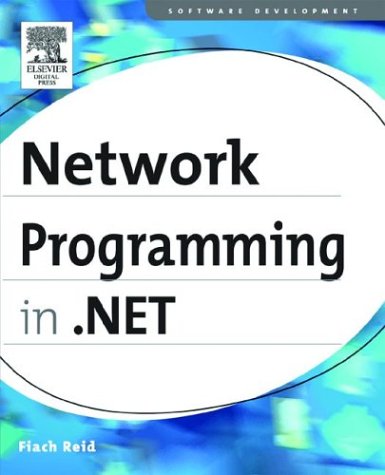
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BuilderPropertyEntry.cs
- ApplicationCommands.cs
- DataGridViewRowHeaderCell.cs
- CapacityStreamGeometryContext.cs
- ProfileParameter.cs
- GenericXmlSecurityTokenAuthenticator.cs
- SkinIDTypeConverter.cs
- ManagementOperationWatcher.cs
- EnumerableRowCollectionExtensions.cs
- TreeNode.cs
- ParentUndoUnit.cs
- CounterCreationDataCollection.cs
- XmlAttributeCollection.cs
- InstanceDescriptor.cs
- Underline.cs
- DetailsViewRow.cs
- OneOfTypeConst.cs
- XmlBinaryReader.cs
- FunctionMappingTranslator.cs
- DeferredElementTreeState.cs
- WsdlBuildProvider.cs
- UIHelper.cs
- XmlBufferReader.cs
- RefreshEventArgs.cs
- ToolStripContainer.cs
- MenuItemBinding.cs
- XmlSchemaValidationException.cs
- FtpWebRequest.cs
- Pair.cs
- Compiler.cs
- IntegerCollectionEditor.cs
- CallContext.cs
- ComboBoxAutomationPeer.cs
- SerializationInfoEnumerator.cs
- EventPrivateKey.cs
- ComplexObject.cs
- DataTableReaderListener.cs
- PagerSettings.cs
- sapiproxy.cs
- StringAttributeCollection.cs
- Grant.cs
- WindowsListViewItemCheckBox.cs
- DynamicScriptObject.cs
- NamedPipeConnectionPool.cs
- CodeTypeReferenceExpression.cs
- SymbolPair.cs
- Rfc4050KeyFormatter.cs
- diagnosticsswitches.cs
- HttpCacheVaryByContentEncodings.cs
- ChannelSinkStacks.cs
- XmlDomTextWriter.cs
- HotSpot.cs
- DoubleKeyFrameCollection.cs
- CustomTypeDescriptor.cs
- WindowsComboBox.cs
- ECDsaCng.cs
- AppLevelCompilationSectionCache.cs
- ReceiveSecurityHeaderEntry.cs
- StylusPointPropertyId.cs
- CFGGrammar.cs
- Rotation3DAnimation.cs
- Transform.cs
- TraceUtility.cs
- _ConnectStream.cs
- TreeViewAutomationPeer.cs
- ApplicationDirectory.cs
- RuleDefinitions.cs
- TemplatePropertyEntry.cs
- Helper.cs
- DefaultDialogButtons.cs
- ResourceReferenceKeyNotFoundException.cs
- CardSpaceSelector.cs
- Int32EqualityComparer.cs
- SessionConnectionReader.cs
- Console.cs
- SoapInteropTypes.cs
- BinaryFormatterWriter.cs
- RectangleHotSpot.cs
- CodeGenerator.cs
- String.cs
- SafeThreadHandle.cs
- ProfessionalColorTable.cs
- CaseStatement.cs
- PageAsyncTaskManager.cs
- XmlNodeList.cs
- CharStorage.cs
- TableStyle.cs
- DataGridViewCell.cs
- IImplicitResourceProvider.cs
- CngKeyCreationParameters.cs
- DataBoundLiteralControl.cs
- OpCodes.cs
- PowerStatus.cs
- MDIClient.cs
- oledbconnectionstring.cs
- PolyQuadraticBezierSegment.cs
- DependencyPropertyDescriptor.cs
- DrawingAttributes.cs
- MetadataArtifactLoaderComposite.cs
- RSAPKCS1SignatureFormatter.cs