Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / MetadataArtifactLoaderComposite.cs / 1305376 / MetadataArtifactLoaderComposite.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Text; using System.Xml; using System.Data.Mapping; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections.ObjectModel; namespace System.Data.Metadata.Edm { ////// This class represents a super-collection (a collection of collections) /// of artifact resources. Typically, this "meta-collection" would contain /// artifacts represented as individual files, directories (which are in /// turn collections of files), and embedded resources. /// ///This is the root class for access to all loader objects. internal class MetadataArtifactLoaderComposite : MetadataArtifactLoader, IEnumerable{ /// /// The list of loaders aggregated by the composite. /// private readonly ReadOnlyCollection_children; /// /// Constructor - loads all resources into the _children collection /// /// A list of collections to aggregate public MetadataArtifactLoaderComposite(Listchildren) { Debug.Assert(children != null); _children = new List (children).AsReadOnly(); } public override string Path { get { return string.Empty; } } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { foreach (MetadataArtifactLoader loader in _children) { loader.CollectFilePermissionPaths(paths, spaceToGet); } } public override bool IsComposite { get { return true; } } /// /// Get the list of paths to all artifacts in the original, unexpanded form /// ///A List of strings identifying paths to all resources public override ListGetOriginalPaths() { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths()); } return list; } /// /// Get paths to artifacts for a specific DataSpace, in the original, unexpanded /// form /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetOriginalPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths(spaceToGet)); } return list; } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetPaths(spaceToGet)); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetPaths()); } return list; } /// /// Aggregates all resource streams from the _children collection /// ///A List of XmlReader objects; cannot be null public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetReaders(sourceDictionary)); } return list; } /// /// Get XmlReaders for a specific DataSpace. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.CreateReaders(spaceToGet)); } return list; } #region IEnumerable Members public IEnumerator GetEnumerator() { return this._children.GetEnumerator(); } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return this._children.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Text; using System.Xml; using System.Data.Mapping; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections.ObjectModel; namespace System.Data.Metadata.Edm { ////// This class represents a super-collection (a collection of collections) /// of artifact resources. Typically, this "meta-collection" would contain /// artifacts represented as individual files, directories (which are in /// turn collections of files), and embedded resources. /// ///This is the root class for access to all loader objects. internal class MetadataArtifactLoaderComposite : MetadataArtifactLoader, IEnumerable{ /// /// The list of loaders aggregated by the composite. /// private readonly ReadOnlyCollection_children; /// /// Constructor - loads all resources into the _children collection /// /// A list of collections to aggregate public MetadataArtifactLoaderComposite(Listchildren) { Debug.Assert(children != null); _children = new List (children).AsReadOnly(); } public override string Path { get { return string.Empty; } } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { foreach (MetadataArtifactLoader loader in _children) { loader.CollectFilePermissionPaths(paths, spaceToGet); } } public override bool IsComposite { get { return true; } } /// /// Get the list of paths to all artifacts in the original, unexpanded form /// ///A List of strings identifying paths to all resources public override ListGetOriginalPaths() { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths()); } return list; } /// /// Get paths to artifacts for a specific DataSpace, in the original, unexpanded /// form /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetOriginalPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths(spaceToGet)); } return list; } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetPaths(spaceToGet)); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetPaths()); } return list; } /// /// Aggregates all resource streams from the _children collection /// ///A List of XmlReader objects; cannot be null public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetReaders(sourceDictionary)); } return list; } /// /// Get XmlReaders for a specific DataSpace. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.CreateReaders(spaceToGet)); } return list; } #region IEnumerable Members public IEnumerator GetEnumerator() { return this._children.GetEnumerator(); } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return this._children.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
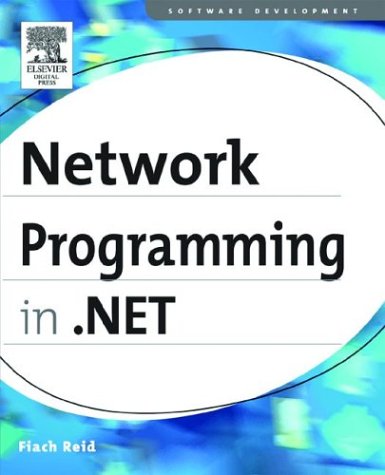
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrintPreviewDialog.cs
- TableAdapterManagerHelper.cs
- SapiRecoInterop.cs
- EmbeddedMailObjectsCollection.cs
- Subtree.cs
- DefaultHttpHandler.cs
- RawStylusInputCustomData.cs
- PrePostDescendentsWalker.cs
- ExtenderProviderService.cs
- PrimitiveCodeDomSerializer.cs
- ExeConfigurationFileMap.cs
- CmsInterop.cs
- PropertyTabChangedEvent.cs
- SessionStateContainer.cs
- recordstatescratchpad.cs
- BaseCollection.cs
- ContextQuery.cs
- MenuAutoFormat.cs
- QuotedPairReader.cs
- TypeNameParser.cs
- Vector3DKeyFrameCollection.cs
- SendSecurityHeader.cs
- FrameworkTemplate.cs
- ImageListStreamer.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- CfgSemanticTag.cs
- UpdateProgress.cs
- UnionCodeGroup.cs
- BitmapMetadataBlob.cs
- Accessible.cs
- NativeMethods.cs
- XmlDesignerDataSourceView.cs
- HtmlElement.cs
- LoginName.cs
- InfoCardXmlSerializer.cs
- DbConnectionStringBuilder.cs
- PointCollectionConverter.cs
- ImagingCache.cs
- DataServiceBehavior.cs
- PatternMatcher.cs
- ShaderEffect.cs
- OrCondition.cs
- TextRenderer.cs
- RotateTransform.cs
- KnownTypeAttribute.cs
- CompilationSection.cs
- StaticExtensionConverter.cs
- TrustManagerMoreInformation.cs
- GridViewSelectEventArgs.cs
- XmlComplianceUtil.cs
- ImageIndexConverter.cs
- BoundConstants.cs
- documentsequencetextview.cs
- GridEntryCollection.cs
- RequestStatusBarUpdateEventArgs.cs
- AttributeEmitter.cs
- Separator.cs
- ProviderUtil.cs
- ObjectParameter.cs
- MethodBody.cs
- RegionInfo.cs
- DbProviderFactory.cs
- XmlWriterTraceListener.cs
- datacache.cs
- WebServiceClientProxyGenerator.cs
- MobileListItem.cs
- SmtpReplyReader.cs
- ListQueryResults.cs
- XPathDocument.cs
- TextBoxLine.cs
- FocusChangedEventArgs.cs
- DrawingDrawingContext.cs
- MultipleViewPattern.cs
- SqlDataSourceCommandEventArgs.cs
- AssemblyUtil.cs
- SystemSounds.cs
- DataGridViewCellConverter.cs
- CallbackTimeoutsElement.cs
- DescriptionAttribute.cs
- XdrBuilder.cs
- LicenseProviderAttribute.cs
- ProtectedConfiguration.cs
- CodePropertyReferenceExpression.cs
- EncodingNLS.cs
- XmlILConstructAnalyzer.cs
- ModuleBuilder.cs
- MultipartContentParser.cs
- DomainUpDown.cs
- ButtonAutomationPeer.cs
- RenderDataDrawingContext.cs
- Int32RectValueSerializer.cs
- Point3DAnimation.cs
- configsystem.cs
- LicenseProviderAttribute.cs
- Stopwatch.cs
- ParseElement.cs
- UnitySerializationHolder.cs
- CharacterHit.cs
- MembershipPasswordException.cs
- Exception.cs