Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / MultipleViewPattern.cs / 1 / MultipleViewPattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for MultipleView Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ///wrapper class for MultipleView pattern #if (INTERNAL_COMPILE) internal class MultipleViewPattern: BasePattern #else public class MultipleViewPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private MultipleViewPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///MultipleView pattern public static readonly AutomationPattern Pattern = MultipleViewPatternIdentifiers.Pattern; ///Property ID: CurrentView - The view ID corresponding to the control's current state. This ID is control-specific. public static readonly AutomationProperty CurrentViewProperty = MultipleViewPatternIdentifiers.CurrentViewProperty; ///Property ID: SupportedViews - Returns an array of ints representing the full set of views available in this control. public static readonly AutomationProperty SupportedViewsProperty = MultipleViewPatternIdentifiers.SupportedViewsProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// The string view name string must be suitable for use by TTS, Braille, etc. /// /// /// The view ID corresponding to the control's current state. This ID is control-specific and can should /// be the same across instances. /// ///Return a localized, human readable string in the application's current UI language. /// ////// This API does not work inside the secure execution environment. /// public string GetViewName( int viewId ) { return UiaCoreApi.MultipleViewPattern_GetViewName(_hPattern, viewId); } ////// /// Change the current view using an ID returned from GetSupportedViews() /// /// ////// This API does not work inside the secure execution environment. /// public void SetCurrentView( int viewId ) { UiaCoreApi.MultipleViewPattern_SetCurrentView(_hPattern, viewId); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// /// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public MultipleViewPatternInformation Cached { get { Misc.ValidateCached(_cached); return new MultipleViewPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public MultipleViewPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new MultipleViewPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new MultipleViewPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; private bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct MultipleViewPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal MultipleViewPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ///The view ID corresponding to the control's current state. This ID is control-specific /// ////// This API does not work inside the secure execution environment. /// public int CurrentView { get { return (int)_el.GetPatternPropertyValue(CurrentViewProperty, _useCache); } } ////// Returns an array of ints representing the full set of views available in this control. /// ////// This API does not work inside the secure execution environment. /// public int [] GetSupportedViews() { return (int [])_el.GetPatternPropertyValue(SupportedViewsProperty, _useCache); } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // ///// // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for MultipleView Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ///wrapper class for MultipleView pattern #if (INTERNAL_COMPILE) internal class MultipleViewPattern: BasePattern #else public class MultipleViewPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private MultipleViewPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///MultipleView pattern public static readonly AutomationPattern Pattern = MultipleViewPatternIdentifiers.Pattern; ///Property ID: CurrentView - The view ID corresponding to the control's current state. This ID is control-specific. public static readonly AutomationProperty CurrentViewProperty = MultipleViewPatternIdentifiers.CurrentViewProperty; ///Property ID: SupportedViews - Returns an array of ints representing the full set of views available in this control. public static readonly AutomationProperty SupportedViewsProperty = MultipleViewPatternIdentifiers.SupportedViewsProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// The string view name string must be suitable for use by TTS, Braille, etc. /// /// /// The view ID corresponding to the control's current state. This ID is control-specific and can should /// be the same across instances. /// ///Return a localized, human readable string in the application's current UI language. /// ////// This API does not work inside the secure execution environment. /// public string GetViewName( int viewId ) { return UiaCoreApi.MultipleViewPattern_GetViewName(_hPattern, viewId); } ////// /// Change the current view using an ID returned from GetSupportedViews() /// /// ////// This API does not work inside the secure execution environment. /// public void SetCurrentView( int viewId ) { UiaCoreApi.MultipleViewPattern_SetCurrentView(_hPattern, viewId); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// /// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public MultipleViewPatternInformation Cached { get { Misc.ValidateCached(_cached); return new MultipleViewPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public MultipleViewPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new MultipleViewPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new MultipleViewPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; private bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct MultipleViewPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal MultipleViewPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ///The view ID corresponding to the control's current state. This ID is control-specific /// ////// This API does not work inside the secure execution environment. /// public int CurrentView { get { return (int)_el.GetPatternPropertyValue(CurrentViewProperty, _useCache); } } ////// Returns an array of ints representing the full set of views available in this control. /// ////// This API does not work inside the secure execution environment. /// public int [] GetSupportedViews() { return (int [])_el.GetPatternPropertyValue(SupportedViewsProperty, _useCache); } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
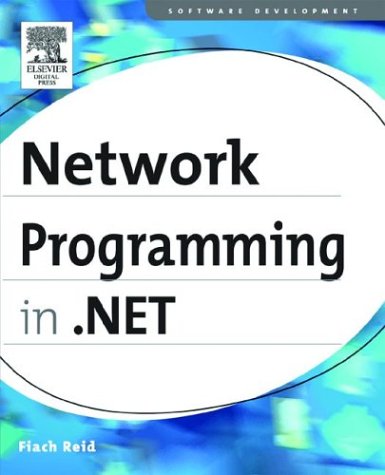
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnderstoodHeaders.cs
- ItemList.cs
- XmlWriterTraceListener.cs
- SlotInfo.cs
- WebEventCodes.cs
- BrushMappingModeValidation.cs
- Line.cs
- AtomicFile.cs
- StoryFragments.cs
- FixedHighlight.cs
- DetailsViewPagerRow.cs
- GridView.cs
- CatalogZone.cs
- DbConnectionClosed.cs
- ContextProperty.cs
- RootDesignerSerializerAttribute.cs
- ListViewPagedDataSource.cs
- GZipStream.cs
- BufferManager.cs
- AssociationTypeEmitter.cs
- DEREncoding.cs
- WpfWebRequestHelper.cs
- ToolStripDropTargetManager.cs
- CompositeFontInfo.cs
- DbXmlEnabledProviderManifest.cs
- InputLanguageCollection.cs
- TextPattern.cs
- DBSchemaTable.cs
- COM2ExtendedBrowsingHandler.cs
- TypeDependencyAttribute.cs
- HwndSourceKeyboardInputSite.cs
- WmlTextBoxAdapter.cs
- Light.cs
- JavascriptCallbackMessageInspector.cs
- GB18030Encoding.cs
- SqlBuilder.cs
- GridViewSelectEventArgs.cs
- ConnectionManager.cs
- SortedSet.cs
- WindowsRegion.cs
- ProviderBase.cs
- MapPathBasedVirtualPathProvider.cs
- MetabaseReader.cs
- ZipArchive.cs
- DataGridViewCheckBoxCell.cs
- BuildProviderCollection.cs
- SchemaImporterExtension.cs
- DataGridRow.cs
- BindableTemplateBuilder.cs
- WebPartConnectionCollection.cs
- EventItfInfo.cs
- Schedule.cs
- UriScheme.cs
- GeometryHitTestParameters.cs
- ListBindingHelper.cs
- DocumentGridContextMenu.cs
- PropertyChangedEventArgs.cs
- Themes.cs
- DateTimeFormatInfoScanner.cs
- PathFigureCollection.cs
- PersonalizationAdministration.cs
- Util.cs
- SqlNodeAnnotation.cs
- PcmConverter.cs
- ScriptControlManager.cs
- WindowPattern.cs
- XmlSchemaResource.cs
- JournalEntryStack.cs
- Table.cs
- ConfigurationException.cs
- InitializationEventAttribute.cs
- CaseStatement.cs
- mongolianshape.cs
- SamlAuthorizationDecisionClaimResource.cs
- DocumentCollection.cs
- RequestChannel.cs
- COM2ExtendedBrowsingHandler.cs
- SQLBytesStorage.cs
- TextSearch.cs
- Sorting.cs
- TimelineGroup.cs
- AmbientValueAttribute.cs
- ToolStripEditorManager.cs
- TreeNodeSelectionProcessor.cs
- _LazyAsyncResult.cs
- Cursor.cs
- RoutedEventConverter.cs
- DomainConstraint.cs
- SectionRecord.cs
- XmlChildEnumerator.cs
- LinkButton.cs
- SmtpCommands.cs
- SmtpReplyReaderFactory.cs
- ConfigurationManagerHelperFactory.cs
- PingReply.cs
- UnionExpr.cs
- ReflectionUtil.cs
- PrintDialog.cs
- PrefixQName.cs
- WebPartConnectionCollection.cs