Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebParts / WebPartConnectionCollection.cs / 1305376 / WebPartConnectionCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Globalization; using System.Web.Util; // [ Editor("System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] public sealed class WebPartConnectionCollection : CollectionBase { private bool _readOnly; private string _readOnlyExceptionMessage; private WebPartManager _webPartManager; internal WebPartConnectionCollection(WebPartManager webPartManager) { _webPartManager = webPartManager; } public bool IsReadOnly { get { return _readOnly; } } ////// Returns the WebPartConnection at a given index. /// public WebPartConnection this[int index] { get { return (WebPartConnection)List[index]; } set { List[index] = value; } } ////// Returns the WebPartConnection with the specified id, performing a case-insensitive comparison. /// Returns null if there are no matches. /// public WebPartConnection this[string id] { // PERF: Use a hashtable for lookup, instead of a linear search get { foreach (WebPartConnection connection in List) { if (String.Equals(connection.ID, id, StringComparison.OrdinalIgnoreCase)) { return connection; } } return null; } } ////// Adds a Connection to the collection. /// public int Add(WebPartConnection value) { return List.Add(value); } private void CheckReadOnly() { if (_readOnly) { throw new InvalidOperationException(SR.GetString(_readOnlyExceptionMessage)); } } ////// True if the WebPartConnection is contained in the collection. /// public bool Contains(WebPartConnection value) { return List.Contains(value); } internal bool ContainsProvider(WebPart provider) { foreach (WebPartConnection connection in List) { if (connection.Provider == provider) { return true; } } return false; } public void CopyTo(WebPartConnection[] array, int index) { List.CopyTo(array, index); } public int IndexOf(WebPartConnection value) { return List.IndexOf(value); } ////// Inserts a WebPartConnection into the collection. /// public void Insert(int index, WebPartConnection value) { List.Insert(index, value); } ////// protected override void OnClear() { CheckReadOnly(); base.OnClear(); } protected override void OnInsert(int index, object value) { CheckReadOnly(); ((WebPartConnection)value).SetWebPartManager(_webPartManager); base.OnInsert(index, value); } protected override void OnRemove(int index, object value) { CheckReadOnly(); ((WebPartConnection)value).SetWebPartManager(null); base.OnRemove(index, value); } protected override void OnSet(int index, object oldValue, object newValue) { CheckReadOnly(); ((WebPartConnection)oldValue).SetWebPartManager(null); ((WebPartConnection)newValue).SetWebPartManager(_webPartManager); base.OnSet(index, oldValue, newValue); } ////// Validates that an object is a WebPartConnection. /// protected override void OnValidate(object value) { base.OnValidate(value); if (value == null) { throw new ArgumentNullException("value", SR.GetString(SR.Collection_CantAddNull)); } if (!(value is WebPartConnection)) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartConnection"), "value"); } } ////// Removes a WebPartConnection from the collection. /// public void Remove(WebPartConnection value) { List.Remove(value); } ////// Marks the collection readonly. This is useful, because we assume that the list /// of static connections is known at Init time, and new connections are added /// through the WebPartManager. /// internal void SetReadOnly(string exceptionMessage) { _readOnlyExceptionMessage = exceptionMessage; _readOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
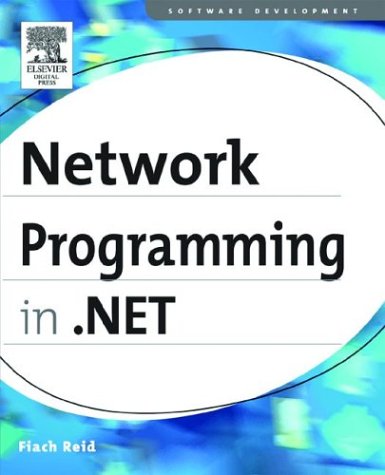
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceContext.cs
- Mutex.cs
- CommonDialog.cs
- ApplicationSecurityManager.cs
- PolyLineSegmentFigureLogic.cs
- OracleInfoMessageEventArgs.cs
- Calendar.cs
- MultiAsyncResult.cs
- BinaryVersion.cs
- MergeFailedEvent.cs
- RegexGroup.cs
- StylusEditingBehavior.cs
- UnmanagedMemoryStream.cs
- HotCommands.cs
- ObjectDataSourceMethodEventArgs.cs
- DecimalAnimationUsingKeyFrames.cs
- CorePropertiesFilter.cs
- RegexInterpreter.cs
- hresults.cs
- SqlTriggerContext.cs
- EventRouteFactory.cs
- NativeMethods.cs
- ServiceModelEnumValidatorAttribute.cs
- RowUpdatingEventArgs.cs
- PolyQuadraticBezierSegment.cs
- activationcontext.cs
- Rule.cs
- SyndicationFeedFormatter.cs
- NavigationPropertyEmitter.cs
- SynchronizedPool.cs
- ObjectStorage.cs
- ParameterToken.cs
- arabicshape.cs
- recordstatefactory.cs
- PreparingEnlistment.cs
- KeyedHashAlgorithm.cs
- DatePicker.cs
- DBBindings.cs
- unsafeIndexingFilterStream.cs
- SocketException.cs
- IntranetCredentialPolicy.cs
- IxmlLineInfo.cs
- RegexGroup.cs
- TraceHandlerErrorFormatter.cs
- QilLiteral.cs
- Double.cs
- XmlCharCheckingWriter.cs
- ReadOnlyObservableCollection.cs
- HttpException.cs
- ColorConvertedBitmap.cs
- CategoryList.cs
- ServiceNameCollection.cs
- CodeExporter.cs
- SoapExtensionTypeElement.cs
- FormParameter.cs
- ReadOnlyMetadataCollection.cs
- ConsoleEntryPoint.cs
- HandlerBase.cs
- AspNetHostingPermission.cs
- StatusBarDesigner.cs
- HttpProfileBase.cs
- ArgumentNullException.cs
- ColumnResizeAdorner.cs
- QilInvokeLateBound.cs
- XAMLParseException.cs
- ExpressionBinding.cs
- ExpressionHelper.cs
- MessageSmuggler.cs
- WebPartManager.cs
- AnnotationResourceCollection.cs
- Certificate.cs
- TextMetrics.cs
- ActivityDesigner.cs
- AnnotationDocumentPaginator.cs
- SignatureToken.cs
- PropertyChange.cs
- AccessibleObject.cs
- DataGridColumnHeader.cs
- SmiSettersStream.cs
- XmlTextReaderImplHelpers.cs
- BitmapEffectrendercontext.cs
- WorkflowRuntimeBehavior.cs
- ArgumentNullException.cs
- PageContentCollection.cs
- TagPrefixCollection.cs
- CursorEditor.cs
- HttpConfigurationSystem.cs
- WebServiceFaultDesigner.cs
- ToolStripItemCollection.cs
- HandleInitializationContext.cs
- ComPlusAuthorization.cs
- SqlDeflator.cs
- InheritablePropertyChangeInfo.cs
- ComplexType.cs
- mediaeventshelper.cs
- WebPartConnection.cs
- HashAlgorithm.cs
- XmlSchemaGroupRef.cs
- FormViewDeleteEventArgs.cs
- PageBreakRecord.cs