Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / Drawing / System / Drawing / Design / CursorEditor.cs / 1 / CursorEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Design { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.IO; using System.Collections; using System.Reflection; using System.Runtime.Serialization.Formatters; using System.Windows.Forms; using System.Windows.Forms.ComponentModel; using System.Windows.Forms.Design; ////// /// /// Provides an editor that can perform default file searching for cursor (.cur) /// files. /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class CursorEditor : UITypeEditor { private CursorUI cursorUI; ////// /// Returns true, indicating that this drop-down control can be resized. /// public override bool IsDropDownResizable { get { return true; } } ////// /// Edits the given object value using the editor style provided by /// GetEditorStyle. A service provider is provided so that any /// required editing services can be obtained. /// public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { object returnValue = value; if (provider != null) { IWindowsFormsEditorService edSvc = (IWindowsFormsEditorService)provider.GetService(typeof(IWindowsFormsEditorService)); if (edSvc != null) { if (cursorUI == null) { cursorUI = new CursorUI(this); } cursorUI.Start(edSvc, value); edSvc.DropDownControl(cursorUI); value = cursorUI.Value; cursorUI.End(); } } return value; } ////// /// Retrieves the editing style of the Edit method. If the method /// is not supported, this will return None. /// public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.DropDown; } ////// /// The user interface for the cursor drop-down. This is just an owner-drawn /// list box. /// private class CursorUI : ListBox { private object value; private IWindowsFormsEditorService edSvc; private TypeConverter cursorConverter; private UITypeEditor editor; public CursorUI(UITypeEditor editor) { this.editor = editor; Height = 310; ItemHeight = (int) Math.Max(4 + Cursors.Default.Size.Height, Font.Height); DrawMode = DrawMode.OwnerDrawFixed; BorderStyle = BorderStyle.None; cursorConverter = TypeDescriptor.GetConverter(typeof(Cursor)); Debug.Assert(cursorConverter.GetStandardValuesSupported(), "Converter '" + cursorConverter.ToString() + "' does not support a list of standard values. We cannot provide a drop-down"); // Fill the list with cursors. // if (cursorConverter.GetStandardValuesSupported()) { foreach (object obj in cursorConverter.GetStandardValues()) { Items.Add(obj); } } } public object Value { get { return value; } } public void End() { edSvc = null; value = null; } protected override void OnClick(EventArgs e) { base.OnClick(e); value = SelectedItem; edSvc.CloseDropDown(); } protected override void OnDrawItem(DrawItemEventArgs die) { base.OnDrawItem(die); if (die.Index != -1) { Cursor cursor = (Cursor)Items[die.Index]; string text = cursorConverter.ConvertToString(cursor); Font font = die.Font; Brush brushText = new SolidBrush(die.ForeColor); die.DrawBackground(); die.Graphics.FillRectangle(SystemBrushes.Control, new Rectangle(die.Bounds.X + 2, die.Bounds.Y + 2, 32, die.Bounds.Height - 4)); die.Graphics.DrawRectangle(SystemPens.WindowText, new Rectangle(die.Bounds.X + 2, die.Bounds.Y + 2, 32 - 1, die.Bounds.Height - 4 - 1)); cursor.DrawStretched(die.Graphics, new Rectangle(die.Bounds.X + 2, die.Bounds.Y + 2, 32, die.Bounds.Height - 4)); die.Graphics.DrawString(text, font, brushText, die.Bounds.X + 36, die.Bounds.Y + (die.Bounds.Height - font.Height)/2); brushText.Dispose(); } } protected override bool ProcessDialogKey(Keys keyData) { if ((keyData & Keys.KeyCode) == Keys.Return && (keyData & (Keys.Alt | Keys.Control)) == 0) { OnClick( EventArgs.Empty ); return true; } return base.ProcessDialogKey(keyData); } public void Start(IWindowsFormsEditorService edSvc, object value) { this.edSvc = edSvc; this.value = value; // Select the current cursor // if (value != null) { for (int i = 0; i < Items.Count; i++) { if (Items[i] == value) { SelectedIndex = i; break; } } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
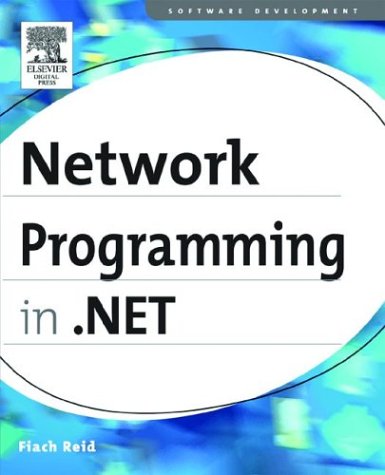
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSourceQueryBuilder.cs
- UserInitiatedNavigationPermission.cs
- PasswordTextNavigator.cs
- bindurihelper.cs
- CqlLexer.cs
- Adorner.cs
- ClipboardProcessor.cs
- MaterialGroup.cs
- ArrayWithOffset.cs
- DictionaryTraceRecord.cs
- SignerInfo.cs
- XmlQuerySequence.cs
- WsrmFault.cs
- ArrayConverter.cs
- SiteMapDesignerDataSourceView.cs
- SrgsElementFactory.cs
- BoundField.cs
- DiscriminatorMap.cs
- PrinterResolution.cs
- AnnotationDocumentPaginator.cs
- XmlSchemaExporter.cs
- TypePropertyEditor.cs
- SourceFileInfo.cs
- ViewStateModeByIdAttribute.cs
- ValidatingPropertiesEventArgs.cs
- Receive.cs
- RSAProtectedConfigurationProvider.cs
- XmlSchemaExternal.cs
- HtmlHistory.cs
- __ConsoleStream.cs
- CreateUserWizard.cs
- Stack.cs
- GraphicsPathIterator.cs
- SqlInternalConnectionTds.cs
- StreamReader.cs
- ToolStripSplitButton.cs
- ScriptingScriptResourceHandlerSection.cs
- XPathBinder.cs
- ConditionalAttribute.cs
- MediaPlayerState.cs
- GenerateTemporaryAssemblyTask.cs
- PersistenceIOParticipant.cs
- CharConverter.cs
- ObjectConverter.cs
- TrustExchangeException.cs
- XmlDocumentFragment.cs
- WhitespaceRuleReader.cs
- StructuralCache.cs
- AutomationProperties.cs
- UntypedNullExpression.cs
- InertiaExpansionBehavior.cs
- ErrorWrapper.cs
- EmptyEnumerable.cs
- WeakReferenceList.cs
- MouseButtonEventArgs.cs
- Emitter.cs
- WebPartRestoreVerb.cs
- TrustManagerPromptUI.cs
- MenuAutomationPeer.cs
- MemoryFailPoint.cs
- odbcmetadatafactory.cs
- XXXInfos.cs
- CharEnumerator.cs
- Polyline.cs
- Stylus.cs
- ApplicationServiceHelper.cs
- PathGradientBrush.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- UserControl.cs
- ProcessInfo.cs
- AssemblyCollection.cs
- DataListItemCollection.cs
- XmlAnyElementAttribute.cs
- MobileListItemCollection.cs
- CodeDirectoryCompiler.cs
- TriState.cs
- PeerNameRegistration.cs
- filewebrequest.cs
- ThemeDirectoryCompiler.cs
- CodeObject.cs
- RenderTargetBitmap.cs
- ListViewPagedDataSource.cs
- ClientBuildManagerTypeDescriptionProviderBridge.cs
- Message.cs
- UdpUtility.cs
- CheckBoxRenderer.cs
- RequestBringIntoViewEventArgs.cs
- XMLSyntaxException.cs
- IFlowDocumentViewer.cs
- WebPartConnectionsDisconnectVerb.cs
- RSAPKCS1KeyExchangeFormatter.cs
- UserInitiatedRoutedEventPermission.cs
- QueryStringParameter.cs
- BitmapCodecInfoInternal.cs
- PrintEvent.cs
- PreProcessInputEventArgs.cs
- InterleavedZipPartStream.cs
- Clause.cs
- OneToOneMappingSerializer.cs
- WebPartChrome.cs