Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Automation / AutomationProperties.cs / 1 / AutomationProperties.cs
using System; namespace System.Windows.Automation { /// public static class AutomationProperties { #region AutomationId ////// AutomationId Property /// public static readonly DependencyProperty AutomationIdProperty = DependencyProperty.RegisterAttached( "AutomationId", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AutomationId property on a DependencyObject. /// public static void SetAutomationId(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AutomationIdProperty, value); } ////// Helper for reading AutomationId property from a DependencyObject. /// public static string GetAutomationId(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AutomationIdProperty)); } #endregion AutomationId #region Name ////// Name Property /// public static readonly DependencyProperty NameProperty = DependencyProperty.RegisterAttached( "Name", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting Name property on a DependencyObject. /// public static void SetName(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(NameProperty, value); } ////// Helper for reading Name property from a DependencyObject. /// public static string GetName(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(NameProperty)); } #endregion Name #region HelpText ////// HelpText Property /// public static readonly DependencyProperty HelpTextProperty = DependencyProperty.RegisterAttached( "HelpText", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting HelpText property on a DependencyObject. /// public static void SetHelpText(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HelpTextProperty, value); } ////// Helper for reading HelpText property from a DependencyObject. /// public static string GetHelpText(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(HelpTextProperty)); } #endregion HelpText #region AcceleratorKey ////// AcceleratorKey Property /// public static readonly DependencyProperty AcceleratorKeyProperty = DependencyProperty.RegisterAttached( "AcceleratorKey", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AcceleratorKey property on a DependencyObject. /// public static void SetAcceleratorKey(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AcceleratorKeyProperty, value); } ////// Helper for reading AcceleratorKey property from a DependencyObject. /// public static string GetAcceleratorKey(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AcceleratorKeyProperty)); } #endregion AcceleratorKey #region AccessKey ////// AccessKey Property /// public static readonly DependencyProperty AccessKeyProperty = DependencyProperty.RegisterAttached( "AccessKey", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AccessKey property on a DependencyObject. /// public static void SetAccessKey(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AccessKeyProperty, value); } ////// Helper for reading AccessKey property from a DependencyObject. /// public static string GetAccessKey(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AccessKeyProperty)); } #endregion AccessKey #region ItemStatus ////// ItemStatus Property /// public static readonly DependencyProperty ItemStatusProperty = DependencyProperty.RegisterAttached( "ItemStatus", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting ItemStatus property on a DependencyObject. /// public static void SetItemStatus(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ItemStatusProperty, value); } ////// Helper for reading ItemStatus property from a DependencyObject. /// public static string GetItemStatus(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(ItemStatusProperty)); } #endregion ItemStatus #region ItemType ////// ItemType Property /// public static readonly DependencyProperty ItemTypeProperty = DependencyProperty.RegisterAttached( "ItemType", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting ItemType property on a DependencyObject. /// public static void SetItemType(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ItemTypeProperty, value); } ////// Helper for reading ItemType property from a DependencyObject. /// public static string GetItemType(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(ItemTypeProperty)); } #endregion ItemType #region IsColumnHeader ////// IsColumnHeader Property /// public static readonly DependencyProperty IsColumnHeaderProperty = DependencyProperty.RegisterAttached( "IsColumnHeader", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsColumnHeader property on a DependencyObject. /// public static void SetIsColumnHeader(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsColumnHeaderProperty, value); } ////// Helper for reading IsColumnHeader property from a DependencyObject. /// public static bool GetIsColumnHeader(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsColumnHeaderProperty)); } #endregion IsColumnHeader #region IsRowHeader ////// IsRowHeader Property /// public static readonly DependencyProperty IsRowHeaderProperty = DependencyProperty.RegisterAttached( "IsRowHeader", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsRowHeader property on a DependencyObject. /// public static void SetIsRowHeader(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsRowHeaderProperty, value); } ////// Helper for reading IsRowHeader property from a DependencyObject. /// public static bool GetIsRowHeader(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsRowHeaderProperty)); } #endregion IsRowHeader #region IsRequiredForForm ////// IsRequiredForForm Property /// public static readonly DependencyProperty IsRequiredForFormProperty = DependencyProperty.RegisterAttached( "IsRequiredForForm", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsRequiredForForm property on a DependencyObject. /// public static void SetIsRequiredForForm(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsRequiredForFormProperty, value); } ////// Helper for reading IsRequiredForForm property from a DependencyObject. /// public static bool GetIsRequiredForForm(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsRequiredForFormProperty)); } #endregion IsRequiredForForm #region LabeledBy ////// LabeledBy Property /// public static readonly DependencyProperty LabeledByProperty = DependencyProperty.RegisterAttached( "LabeledBy", typeof(UIElement), typeof(AutomationProperties), new UIPropertyMetadata((UIElement)null)); ////// Helper for setting LabeledBy property on a DependencyObject. /// public static void SetLabeledBy(DependencyObject element, UIElement value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(LabeledByProperty, value); } ////// Helper for reading LabeledBy property from a DependencyObject. /// public static UIElement GetLabeledBy(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((UIElement)element.GetValue(LabeledByProperty)); } #endregion LabeledBy #region private implementation // Validation callback for string properties private static bool IsNotNull(object value) { return (value != null); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; namespace System.Windows.Automation { /// public static class AutomationProperties { #region AutomationId ////// AutomationId Property /// public static readonly DependencyProperty AutomationIdProperty = DependencyProperty.RegisterAttached( "AutomationId", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AutomationId property on a DependencyObject. /// public static void SetAutomationId(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AutomationIdProperty, value); } ////// Helper for reading AutomationId property from a DependencyObject. /// public static string GetAutomationId(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AutomationIdProperty)); } #endregion AutomationId #region Name ////// Name Property /// public static readonly DependencyProperty NameProperty = DependencyProperty.RegisterAttached( "Name", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting Name property on a DependencyObject. /// public static void SetName(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(NameProperty, value); } ////// Helper for reading Name property from a DependencyObject. /// public static string GetName(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(NameProperty)); } #endregion Name #region HelpText ////// HelpText Property /// public static readonly DependencyProperty HelpTextProperty = DependencyProperty.RegisterAttached( "HelpText", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting HelpText property on a DependencyObject. /// public static void SetHelpText(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HelpTextProperty, value); } ////// Helper for reading HelpText property from a DependencyObject. /// public static string GetHelpText(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(HelpTextProperty)); } #endregion HelpText #region AcceleratorKey ////// AcceleratorKey Property /// public static readonly DependencyProperty AcceleratorKeyProperty = DependencyProperty.RegisterAttached( "AcceleratorKey", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AcceleratorKey property on a DependencyObject. /// public static void SetAcceleratorKey(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AcceleratorKeyProperty, value); } ////// Helper for reading AcceleratorKey property from a DependencyObject. /// public static string GetAcceleratorKey(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AcceleratorKeyProperty)); } #endregion AcceleratorKey #region AccessKey ////// AccessKey Property /// public static readonly DependencyProperty AccessKeyProperty = DependencyProperty.RegisterAttached( "AccessKey", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AccessKey property on a DependencyObject. /// public static void SetAccessKey(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AccessKeyProperty, value); } ////// Helper for reading AccessKey property from a DependencyObject. /// public static string GetAccessKey(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AccessKeyProperty)); } #endregion AccessKey #region ItemStatus ////// ItemStatus Property /// public static readonly DependencyProperty ItemStatusProperty = DependencyProperty.RegisterAttached( "ItemStatus", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting ItemStatus property on a DependencyObject. /// public static void SetItemStatus(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ItemStatusProperty, value); } ////// Helper for reading ItemStatus property from a DependencyObject. /// public static string GetItemStatus(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(ItemStatusProperty)); } #endregion ItemStatus #region ItemType ////// ItemType Property /// public static readonly DependencyProperty ItemTypeProperty = DependencyProperty.RegisterAttached( "ItemType", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting ItemType property on a DependencyObject. /// public static void SetItemType(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ItemTypeProperty, value); } ////// Helper for reading ItemType property from a DependencyObject. /// public static string GetItemType(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(ItemTypeProperty)); } #endregion ItemType #region IsColumnHeader ////// IsColumnHeader Property /// public static readonly DependencyProperty IsColumnHeaderProperty = DependencyProperty.RegisterAttached( "IsColumnHeader", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsColumnHeader property on a DependencyObject. /// public static void SetIsColumnHeader(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsColumnHeaderProperty, value); } ////// Helper for reading IsColumnHeader property from a DependencyObject. /// public static bool GetIsColumnHeader(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsColumnHeaderProperty)); } #endregion IsColumnHeader #region IsRowHeader ////// IsRowHeader Property /// public static readonly DependencyProperty IsRowHeaderProperty = DependencyProperty.RegisterAttached( "IsRowHeader", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsRowHeader property on a DependencyObject. /// public static void SetIsRowHeader(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsRowHeaderProperty, value); } ////// Helper for reading IsRowHeader property from a DependencyObject. /// public static bool GetIsRowHeader(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsRowHeaderProperty)); } #endregion IsRowHeader #region IsRequiredForForm ////// IsRequiredForForm Property /// public static readonly DependencyProperty IsRequiredForFormProperty = DependencyProperty.RegisterAttached( "IsRequiredForForm", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsRequiredForForm property on a DependencyObject. /// public static void SetIsRequiredForForm(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsRequiredForFormProperty, value); } ////// Helper for reading IsRequiredForForm property from a DependencyObject. /// public static bool GetIsRequiredForForm(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsRequiredForFormProperty)); } #endregion IsRequiredForForm #region LabeledBy ////// LabeledBy Property /// public static readonly DependencyProperty LabeledByProperty = DependencyProperty.RegisterAttached( "LabeledBy", typeof(UIElement), typeof(AutomationProperties), new UIPropertyMetadata((UIElement)null)); ////// Helper for setting LabeledBy property on a DependencyObject. /// public static void SetLabeledBy(DependencyObject element, UIElement value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(LabeledByProperty, value); } ////// Helper for reading LabeledBy property from a DependencyObject. /// public static UIElement GetLabeledBy(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((UIElement)element.GetValue(LabeledByProperty)); } #endregion LabeledBy #region private implementation // Validation callback for string properties private static bool IsNotNull(object value) { return (value != null); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
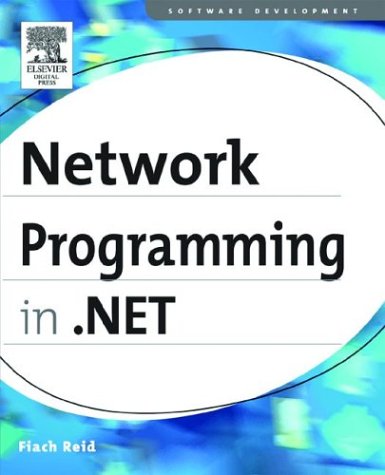
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SID.cs
- RuleRefElement.cs
- SessionPageStateSection.cs
- ExecutionScope.cs
- DataSourceControl.cs
- SchemaHelper.cs
- XmlSchemaGroupRef.cs
- UICuesEvent.cs
- TraceEventCache.cs
- ValidationResult.cs
- GenericWebPart.cs
- XmlSchemaDatatype.cs
- GPPOINTF.cs
- DrawingAttributeSerializer.cs
- KeyMatchBuilder.cs
- XmlWriterSettings.cs
- SchemaElementLookUpTable.cs
- TraceUtils.cs
- PermissionAttributes.cs
- MSG.cs
- KeyGesture.cs
- AddressAccessDeniedException.cs
- HtmlInputSubmit.cs
- DataMemberFieldConverter.cs
- Completion.cs
- SetterBaseCollection.cs
- CheckedPointers.cs
- UTF8Encoding.cs
- tooltip.cs
- CodeEventReferenceExpression.cs
- X509Certificate2.cs
- DatagramAdapter.cs
- ScriptingJsonSerializationSection.cs
- ModelService.cs
- DataGridViewBindingCompleteEventArgs.cs
- WpfXamlMember.cs
- Mutex.cs
- BindToObject.cs
- FilteredDataSetHelper.cs
- EntityDataSource.cs
- DataGridRelationshipRow.cs
- OrderByBuilder.cs
- OdbcConnectionPoolProviderInfo.cs
- DataGridViewCellValidatingEventArgs.cs
- listitem.cs
- Clipboard.cs
- DataBinder.cs
- WebUtil.cs
- SimpleMailWebEventProvider.cs
- Invariant.cs
- TreeNodeCollection.cs
- MobileControlDesigner.cs
- CodeObjectCreateExpression.cs
- CachedFontFace.cs
- DbSourceCommand.cs
- SRGSCompiler.cs
- EntityDataSourceWrapperCollection.cs
- QuadraticBezierSegment.cs
- BindValidationContext.cs
- HeaderedContentControl.cs
- CaseStatement.cs
- DoubleLinkListEnumerator.cs
- SqlBooleanizer.cs
- TextEditorThreadLocalStore.cs
- XamlWriter.cs
- Compiler.cs
- WebPartZoneCollection.cs
- TextDecorations.cs
- SchemaTableOptionalColumn.cs
- DataGridViewCellConverter.cs
- PopupControlService.cs
- MenuBindingsEditor.cs
- SqlCharStream.cs
- IDictionary.cs
- SplineKeyFrames.cs
- TPLETWProvider.cs
- UnsafeCollabNativeMethods.cs
- WindowsAuthenticationModule.cs
- ToolTipAutomationPeer.cs
- SpinWait.cs
- CellTreeNode.cs
- WindowsSysHeader.cs
- LongCountAggregationOperator.cs
- DeploymentExceptionMapper.cs
- RijndaelManagedTransform.cs
- DataGridColumnDropSeparator.cs
- XmlLoader.cs
- UnsafeNetInfoNativeMethods.cs
- DoWorkEventArgs.cs
- DeferredSelectedIndexReference.cs
- ScrollProperties.cs
- Compilation.cs
- WindowAutomationPeer.cs
- WebPartConnectionsConfigureVerb.cs
- SerializationInfo.cs
- CacheOutputQuery.cs
- SecurityUtils.cs
- SqlPersonalizationProvider.cs
- CalendarDay.cs
- tabpagecollectioneditor.cs