Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / SQLTypes / SqlCharStream.cs / 1 / SqlCharStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SqlStreamChars.cs // // Create by: JunFang // // Description: // // Notes: // // History: // // 04/17/01 JunFang Created. // // @EndHeader@ //************************************************************************* namespace System.Data.SqlTypes { using System; using System.IO; using System.Runtime.InteropServices; using System.Data.SqlTypes; #if WINFSInternalOnly public #else internal #endif abstract class SqlStreamChars: System.Data.SqlTypes.INullable, IDisposable { public abstract bool IsNull { get; } public abstract bool CanRead { get; } public abstract bool CanSeek { get; } public abstract bool CanWrite { get; } public abstract long Length { get; } public abstract long Position { get; set; } // -------------------------------------------------------------- // Public methods // ------------------------------------------------------------- public abstract int Read (char[] buffer, int offset, int count); public abstract void Write (char[] buffer, int offset, int count); public abstract long Seek (long offset, SeekOrigin origin); public abstract void SetLength (long value); public abstract void Flush (); public virtual void Close(){ Dispose(true); } void IDisposable.Dispose() { Dispose(true); } protected virtual void Dispose(bool disposing) { } public virtual int ReadChar() { // Reads one char from the stream by calling Read(char[], int, int). // Will return an char cast to an int or -1 on end of stream. // The performance of the default implementation on Stream is bad, // and any subclass with an internal buffer should override this method. char[] oneCharArray = new char[1]; int r = Read(oneCharArray, 0, 1); if (r==0) return -1; return oneCharArray[0]; } public virtual void WriteChar(char value) { // Writes one char from the stream by calling Write(char[], int, int). // The performance of the default implementation on Stream is bad, // and any subclass with an internal buffer should override this method. char[] oneCharArray = new char[1]; oneCharArray[0] = value; Write(oneCharArray, 0, 1); } // Private class: the Null SqlStreamChars private class NullSqlStreamChars : SqlStreamChars { // -------------------------------------------------------------- // Constructor(s) // -------------------------------------------------------------- internal NullSqlStreamChars() { } // ------------------------------------------------------------- // Public properties // -------------------------------------------------------------- public override bool IsNull { get { return true; } } public override bool CanRead { get { return false; } } public override bool CanSeek { get { return false; } } public override bool CanWrite { get { return false; } } public override long Length { get { throw new SqlNullValueException(); } } public override long Position { get { throw new SqlNullValueException(); } set { throw new SqlNullValueException(); } } // ------------------------------------------------------------- // Public methods // ------------------------------------------------------------- public override int Read (char[] buffer, int offset, int count) { throw new SqlNullValueException(); } public override void Write (char[] buffer, int offset, int count) { throw new SqlNullValueException(); } public override long Seek (long offset, SeekOrigin origin) { throw new SqlNullValueException(); } public override void SetLength (long value) { throw new SqlNullValueException(); } public override void Flush () { throw new SqlNullValueException(); } public override void Close () { } } // class NullSqlStreamChars // The Null instance public static SqlStreamChars Null { get { return new NullSqlStreamChars(); } } } // class SqlStreamChars } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
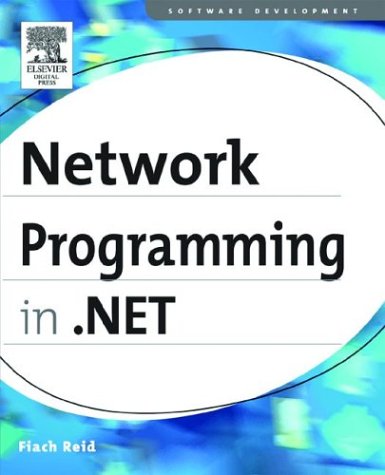
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AbstractDataSvcMapFileLoader.cs
- CellRelation.cs
- Button.cs
- SystemGatewayIPAddressInformation.cs
- OpCodes.cs
- SignatureGenerator.cs
- SafeNativeMethods.cs
- MarkupExtensionSerializer.cs
- DecoderFallback.cs
- OneOfTypeConst.cs
- JoinElimination.cs
- GreenMethods.cs
- QueryTask.cs
- ParserContext.cs
- util.cs
- CommandValueSerializer.cs
- DBDataPermission.cs
- HttpModuleAction.cs
- BitmapEffectInputConnector.cs
- XmlMemberMapping.cs
- TcpTransportManager.cs
- ByteStack.cs
- Directory.cs
- SplitContainer.cs
- EntryIndex.cs
- DetailsViewDeleteEventArgs.cs
- Rotation3D.cs
- EmissiveMaterial.cs
- TextShapeableCharacters.cs
- WebResourceAttribute.cs
- RelOps.cs
- ConfigUtil.cs
- DataBindingCollection.cs
- EntitySetDataBindingList.cs
- X509Certificate.cs
- AdapterUtil.cs
- MinimizableAttributeTypeConverter.cs
- ValidationContext.cs
- ListBoxDesigner.cs
- TextReader.cs
- BulletChrome.cs
- JsonXmlDataContract.cs
- AnnotationElement.cs
- OrderedDictionary.cs
- SchemaElementLookUpTable.cs
- Crc32.cs
- RegionIterator.cs
- InternalConfigConfigurationFactory.cs
- DetailsViewUpdatedEventArgs.cs
- TextServicesDisplayAttribute.cs
- ToolStripPanelRow.cs
- InputReport.cs
- Scene3D.cs
- CustomErrorCollection.cs
- ConstantCheck.cs
- SQLBoolean.cs
- DependencyPropertyHelper.cs
- GlobalEventManager.cs
- BufferedGraphicsManager.cs
- Int32AnimationUsingKeyFrames.cs
- BindingMemberInfo.cs
- ProgressChangedEventArgs.cs
- BaseCodePageEncoding.cs
- PasswordRecovery.cs
- Geometry.cs
- BrowserCapabilitiesCompiler.cs
- OleDbFactory.cs
- CacheChildrenQuery.cs
- ImageBrush.cs
- XamlPointCollectionSerializer.cs
- OperationCanceledException.cs
- ResourceReader.cs
- FlowPosition.cs
- DataRowChangeEvent.cs
- ManipulationVelocities.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- NameValueFileSectionHandler.cs
- SqlCacheDependencyDatabaseCollection.cs
- ToolStripHighContrastRenderer.cs
- PropertyRecord.cs
- WeakReference.cs
- StrokeCollectionConverter.cs
- TrackBar.cs
- ConnectionManagementElement.cs
- IndicShape.cs
- Native.cs
- CacheDependency.cs
- SqlServices.cs
- NativeMethodsOther.cs
- XmlSchemaValidationException.cs
- FileLogRecord.cs
- _Win32.cs
- CompilationUtil.cs
- AuthenticationService.cs
- AttributeXamlType.cs
- LinkConverter.cs
- ObjectViewEntityCollectionData.cs
- TextReader.cs
- RootBuilder.cs
- WindowAutomationPeer.cs