Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / internal / materialization / util.cs / 1305376 / util.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Data.Mapping; namespace System.Data.Common.Internal.Materialization { static class Util { ////// Retrieves a mapping to CLR type for the given EDM type. Assumes the MetadataWorkspace has no /// internal static ObjectTypeMapping GetObjectMapping(EdmType type, MetadataWorkspace workspace) { // Check if the workspace has cspace item collection registered with it. If not, then its a case // of public materializer trying to create objects from PODR or EntityDataReader with no context. ItemCollection collection; if (workspace.TryGetItemCollection(DataSpace.CSpace, out collection)) { return (ObjectTypeMapping)workspace.GetMap(type, DataSpace.OCSpace); } else { EdmType ospaceType; EdmType cspaceType; // If its a case of EntityDataReader with no context, the typeUsage which is passed in must contain // a cspace type. We need to look up an OSpace type in the ospace item collection and then create // ocMapping if (type.DataSpace == DataSpace.CSpace) { // if its a primitive type, then the names will be different for CSpace type and OSpace type if (Helper.IsPrimitiveType(type)) { ospaceType = workspace.GetMappedPrimitiveType(((PrimitiveType)type).PrimitiveTypeKind, DataSpace.OSpace); } else { // Metadata will throw if there is no item with this identity present. // Is this exception fine or does object materializer code wants to wrap and throw a new exception ospaceType = workspace.GetItem(type.FullName, DataSpace.OSpace); } cspaceType = type; } else { // In case of PODR, there is no cspace at all. We must create a fake ocmapping, with ospace types // on both the ends ospaceType = type; cspaceType = type; } // This condition must be hit only when someone is trying to materialize a legacy data reader and we // don't have the CSpace metadata. if (!Helper.IsPrimitiveType(ospaceType) && !Helper.IsEntityType(ospaceType) && !Helper.IsComplexType(ospaceType)) { throw EntityUtil.MaterializerUnsupportedType(); } ObjectTypeMapping typeMapping; if (Helper.IsPrimitiveType(ospaceType)) { typeMapping = new ObjectTypeMapping(ospaceType, cspaceType); } else { typeMapping = DefaultObjectMappingItemCollection.LoadObjectMapping(cspaceType, ospaceType, null); } return typeMapping; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Data.Mapping; namespace System.Data.Common.Internal.Materialization { static class Util { ////// Retrieves a mapping to CLR type for the given EDM type. Assumes the MetadataWorkspace has no /// internal static ObjectTypeMapping GetObjectMapping(EdmType type, MetadataWorkspace workspace) { // Check if the workspace has cspace item collection registered with it. If not, then its a case // of public materializer trying to create objects from PODR or EntityDataReader with no context. ItemCollection collection; if (workspace.TryGetItemCollection(DataSpace.CSpace, out collection)) { return (ObjectTypeMapping)workspace.GetMap(type, DataSpace.OCSpace); } else { EdmType ospaceType; EdmType cspaceType; // If its a case of EntityDataReader with no context, the typeUsage which is passed in must contain // a cspace type. We need to look up an OSpace type in the ospace item collection and then create // ocMapping if (type.DataSpace == DataSpace.CSpace) { // if its a primitive type, then the names will be different for CSpace type and OSpace type if (Helper.IsPrimitiveType(type)) { ospaceType = workspace.GetMappedPrimitiveType(((PrimitiveType)type).PrimitiveTypeKind, DataSpace.OSpace); } else { // Metadata will throw if there is no item with this identity present. // Is this exception fine or does object materializer code wants to wrap and throw a new exception ospaceType = workspace.GetItem(type.FullName, DataSpace.OSpace); } cspaceType = type; } else { // In case of PODR, there is no cspace at all. We must create a fake ocmapping, with ospace types // on both the ends ospaceType = type; cspaceType = type; } // This condition must be hit only when someone is trying to materialize a legacy data reader and we // don't have the CSpace metadata. if (!Helper.IsPrimitiveType(ospaceType) && !Helper.IsEntityType(ospaceType) && !Helper.IsComplexType(ospaceType)) { throw EntityUtil.MaterializerUnsupportedType(); } ObjectTypeMapping typeMapping; if (Helper.IsPrimitiveType(ospaceType)) { typeMapping = new ObjectTypeMapping(ospaceType, cspaceType); } else { typeMapping = DefaultObjectMappingItemCollection.LoadObjectMapping(cspaceType, ospaceType, null); } return typeMapping; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
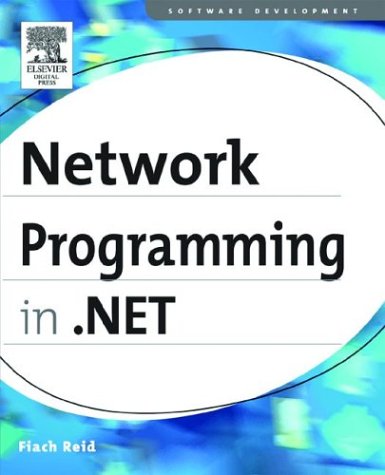
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NetworkAddressChange.cs
- AddressingProperty.cs
- InlinedAggregationOperator.cs
- DiscriminatorMap.cs
- FrameworkPropertyMetadata.cs
- Grid.cs
- CommandConverter.cs
- ToolStripItemCollection.cs
- ScrollData.cs
- XmlQueryContext.cs
- ClientFormsIdentity.cs
- EntityTypeBase.cs
- ComponentDispatcher.cs
- CertificateManager.cs
- ReaderContextStackData.cs
- EventMap.cs
- XmlException.cs
- ComponentEvent.cs
- ToolStripLabel.cs
- ArgumentValidation.cs
- BamlBinaryReader.cs
- XmlSchemaSimpleTypeList.cs
- DbProviderSpecificTypePropertyAttribute.cs
- ComboBoxHelper.cs
- KeyedCollection.cs
- ParagraphResult.cs
- ToolStripOverflowButton.cs
- DrawingContextDrawingContextWalker.cs
- DesignRelationCollection.cs
- UnhandledExceptionEventArgs.cs
- MarshalDirectiveException.cs
- HtmlFormAdapter.cs
- QueryOutputWriter.cs
- TypedTableHandler.cs
- HTMLTextWriter.cs
- FontCollection.cs
- AnimationStorage.cs
- EventDescriptor.cs
- Profiler.cs
- EncryptedType.cs
- TaskExtensions.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- MetadataProperty.cs
- PathFigure.cs
- RadioButtonPopupAdapter.cs
- Stackframe.cs
- SemaphoreSecurity.cs
- RectConverter.cs
- CodeMemberField.cs
- EventData.cs
- TableItemProviderWrapper.cs
- AutoResizedEvent.cs
- ISO2022Encoding.cs
- ScrollableControl.cs
- XPathItem.cs
- Rules.cs
- XmlIgnoreAttribute.cs
- Parallel.cs
- BufferedGraphicsContext.cs
- XmlBaseWriter.cs
- CFGGrammar.cs
- MessageSecurityOverHttpElement.cs
- PrintDocument.cs
- tabpagecollectioneditor.cs
- FixedSOMTable.cs
- WebServiceReceive.cs
- DataSourceHelper.cs
- CLSCompliantAttribute.cs
- ConfigXmlText.cs
- SplitterPanel.cs
- DataGridItemEventArgs.cs
- autovalidator.cs
- ListBindableAttribute.cs
- TypeViewSchema.cs
- WebPartPersonalization.cs
- WizardForm.cs
- TreeNodeEventArgs.cs
- ThrowOnMultipleAssignment.cs
- XamlGridLengthSerializer.cs
- LeafCellTreeNode.cs
- SqlBooleanizer.cs
- DataGridViewAddColumnDialog.cs
- RefreshEventArgs.cs
- DatePicker.cs
- DragEvent.cs
- HeaderUtility.cs
- AsyncPostBackTrigger.cs
- ToolStripRendererSwitcher.cs
- TriggerBase.cs
- ScrollChrome.cs
- SrgsText.cs
- BamlTreeMap.cs
- ButtonChrome.cs
- SqlProfileProvider.cs
- CollectionViewSource.cs
- MLangCodePageEncoding.cs
- ClientRolePrincipal.cs
- XmlSchemaAppInfo.cs
- ScrollPattern.cs
- SerializerProvider.cs