Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Net / System / Net / Mail / MailHeaderInfo.cs / 1 / MailHeaderInfo.cs
using System; using System.Collections.Specialized; using System.Net.Mail; using System.Globalization; using System.Collections.Generic; namespace System.Net.Mail { // Enumeration of the well-known headers. // If you add to this enum you MUST also add the appropriate initializer in m_HeaderInfo below. internal enum MailHeaderID { Bcc = 0, Cc, Comments, ContentDescription, ContentDisposition, ContentID, ContentLocation, ContentTransferEncoding, ContentType, Date, From, Importance, InReplyTo, Keywords, Max, MessageID, MimeVersion, Priority, References, ReplyTo, ResentBcc, ResentCc, ResentDate, ResentFrom, ResentMessageID, ResentSender, ResentTo, Sender, Subject, To, XPriority, XReceiver, XSender, ZMaxEnumValue = XSender, // Keep this to equal to the last "known" enum entry if you add to the end Unknown = -1 } internal static class MailHeaderInfo { // Structure that wraps information about a single mail header private struct HeaderInfo { public readonly string NormalizedName; public readonly bool IsSingleton; public readonly MailHeaderID ID; public HeaderInfo(MailHeaderID id, string name, bool isSingleton) { ID = id; NormalizedName = name; IsSingleton = isSingleton; } } // Table of well-known mail headers. // Keep the initializers in [....] with the enum above. private static readonly HeaderInfo[] m_HeaderInfo = { // ID NormalizedString IsSingleton new HeaderInfo(MailHeaderID.Bcc, "Bcc", true), new HeaderInfo(MailHeaderID.Cc, "Cc", true), new HeaderInfo(MailHeaderID.Comments, "Comments", false), new HeaderInfo(MailHeaderID.ContentDescription, "Content-Description", true), new HeaderInfo(MailHeaderID.ContentDisposition, "Content-Disposition", true), new HeaderInfo(MailHeaderID.ContentID, "Content-ID", true), new HeaderInfo(MailHeaderID.ContentLocation, "Content-Location", true), new HeaderInfo(MailHeaderID.ContentTransferEncoding, "Content-Transfer-Encoding", true), new HeaderInfo(MailHeaderID.ContentType, "Content-Type", true), new HeaderInfo(MailHeaderID.Date, "Date", true), new HeaderInfo(MailHeaderID.From, "From", true), new HeaderInfo(MailHeaderID.Importance, "Importance", true), new HeaderInfo(MailHeaderID.InReplyTo, "In-Reply-To", true), new HeaderInfo(MailHeaderID.Keywords, "Keywords", false), new HeaderInfo(MailHeaderID.Max, "Max", false), new HeaderInfo(MailHeaderID.MessageID, "Message-ID", true), new HeaderInfo(MailHeaderID.MimeVersion, "MIME-Version", true), new HeaderInfo(MailHeaderID.Priority, "Priority", true), new HeaderInfo(MailHeaderID.References, "References", true), new HeaderInfo(MailHeaderID.ReplyTo, "Reply-To", true), new HeaderInfo(MailHeaderID.ResentBcc, "Resent-Bcc", false), new HeaderInfo(MailHeaderID.ResentCc, "Resent-Cc", false), new HeaderInfo(MailHeaderID.ResentDate, "Resent-Date", false), new HeaderInfo(MailHeaderID.ResentFrom, "Resent-From", false), new HeaderInfo(MailHeaderID.ResentMessageID, "Resent-Message-ID", false), new HeaderInfo(MailHeaderID.ResentSender, "Resent-Sender", false), new HeaderInfo(MailHeaderID.ResentTo, "Resent-To", false), new HeaderInfo(MailHeaderID.Sender, "Sender", true), new HeaderInfo(MailHeaderID.Subject, "Subject", true), new HeaderInfo(MailHeaderID.To, "To", true), new HeaderInfo(MailHeaderID.XPriority, "X-Priority", true), new HeaderInfo(MailHeaderID.XReceiver, "X-Receiver", false), new HeaderInfo(MailHeaderID.XSender, "X-Sender", true) }; private static readonly Dictionarym_HeaderDictionary; static MailHeaderInfo() { #if DEBUG // Check that enum and header info array are in [....] for(int i = 0; i < m_HeaderInfo.Length; i++) { if((int)m_HeaderInfo[i].ID != i) { throw new Exception("Header info data structures are not in [....]"); } } #endif // Create dictionary for string-to-enum lookup. Ordinal and IgnoreCase are intentional. m_HeaderDictionary = new Dictionary ((int)MailHeaderID.ZMaxEnumValue + 1, StringComparer.OrdinalIgnoreCase); for(int i = 0; i < m_HeaderInfo.Length; i++) { m_HeaderDictionary.Add(m_HeaderInfo[i].NormalizedName, i); } } internal static string GetString(MailHeaderID id) { switch(id) { case MailHeaderID.Unknown: case MailHeaderID.ZMaxEnumValue+1: return null; default: return m_HeaderInfo[(int)id].NormalizedName; } } internal static MailHeaderID GetID(string name) { int id; if(m_HeaderDictionary.TryGetValue(name, out id)) { return (MailHeaderID)id; } return MailHeaderID.Unknown; } internal static bool IsWellKnown(string name) { int dummy; return m_HeaderDictionary.TryGetValue(name, out dummy); } internal static bool IsSingleton(string name) { int index; if(m_HeaderDictionary.TryGetValue(name, out index)) { return m_HeaderInfo[index].IsSingleton; } return false; } internal static string NormalizeCase(string name) { int index; if(m_HeaderDictionary.TryGetValue(name, out index)) { return m_HeaderInfo[index].NormalizedName; } return name; } internal static bool IsMatch(string name, MailHeaderID header) { int index; if(m_HeaderDictionary.TryGetValue(name, out index) && (MailHeaderID)index == header) { return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Specialized; using System.Net.Mail; using System.Globalization; using System.Collections.Generic; namespace System.Net.Mail { // Enumeration of the well-known headers. // If you add to this enum you MUST also add the appropriate initializer in m_HeaderInfo below. internal enum MailHeaderID { Bcc = 0, Cc, Comments, ContentDescription, ContentDisposition, ContentID, ContentLocation, ContentTransferEncoding, ContentType, Date, From, Importance, InReplyTo, Keywords, Max, MessageID, MimeVersion, Priority, References, ReplyTo, ResentBcc, ResentCc, ResentDate, ResentFrom, ResentMessageID, ResentSender, ResentTo, Sender, Subject, To, XPriority, XReceiver, XSender, ZMaxEnumValue = XSender, // Keep this to equal to the last "known" enum entry if you add to the end Unknown = -1 } internal static class MailHeaderInfo { // Structure that wraps information about a single mail header private struct HeaderInfo { public readonly string NormalizedName; public readonly bool IsSingleton; public readonly MailHeaderID ID; public HeaderInfo(MailHeaderID id, string name, bool isSingleton) { ID = id; NormalizedName = name; IsSingleton = isSingleton; } } // Table of well-known mail headers. // Keep the initializers in [....] with the enum above. private static readonly HeaderInfo[] m_HeaderInfo = { // ID NormalizedString IsSingleton new HeaderInfo(MailHeaderID.Bcc, "Bcc", true), new HeaderInfo(MailHeaderID.Cc, "Cc", true), new HeaderInfo(MailHeaderID.Comments, "Comments", false), new HeaderInfo(MailHeaderID.ContentDescription, "Content-Description", true), new HeaderInfo(MailHeaderID.ContentDisposition, "Content-Disposition", true), new HeaderInfo(MailHeaderID.ContentID, "Content-ID", true), new HeaderInfo(MailHeaderID.ContentLocation, "Content-Location", true), new HeaderInfo(MailHeaderID.ContentTransferEncoding, "Content-Transfer-Encoding", true), new HeaderInfo(MailHeaderID.ContentType, "Content-Type", true), new HeaderInfo(MailHeaderID.Date, "Date", true), new HeaderInfo(MailHeaderID.From, "From", true), new HeaderInfo(MailHeaderID.Importance, "Importance", true), new HeaderInfo(MailHeaderID.InReplyTo, "In-Reply-To", true), new HeaderInfo(MailHeaderID.Keywords, "Keywords", false), new HeaderInfo(MailHeaderID.Max, "Max", false), new HeaderInfo(MailHeaderID.MessageID, "Message-ID", true), new HeaderInfo(MailHeaderID.MimeVersion, "MIME-Version", true), new HeaderInfo(MailHeaderID.Priority, "Priority", true), new HeaderInfo(MailHeaderID.References, "References", true), new HeaderInfo(MailHeaderID.ReplyTo, "Reply-To", true), new HeaderInfo(MailHeaderID.ResentBcc, "Resent-Bcc", false), new HeaderInfo(MailHeaderID.ResentCc, "Resent-Cc", false), new HeaderInfo(MailHeaderID.ResentDate, "Resent-Date", false), new HeaderInfo(MailHeaderID.ResentFrom, "Resent-From", false), new HeaderInfo(MailHeaderID.ResentMessageID, "Resent-Message-ID", false), new HeaderInfo(MailHeaderID.ResentSender, "Resent-Sender", false), new HeaderInfo(MailHeaderID.ResentTo, "Resent-To", false), new HeaderInfo(MailHeaderID.Sender, "Sender", true), new HeaderInfo(MailHeaderID.Subject, "Subject", true), new HeaderInfo(MailHeaderID.To, "To", true), new HeaderInfo(MailHeaderID.XPriority, "X-Priority", true), new HeaderInfo(MailHeaderID.XReceiver, "X-Receiver", false), new HeaderInfo(MailHeaderID.XSender, "X-Sender", true) }; private static readonly Dictionary m_HeaderDictionary; static MailHeaderInfo() { #if DEBUG // Check that enum and header info array are in [....] for(int i = 0; i < m_HeaderInfo.Length; i++) { if((int)m_HeaderInfo[i].ID != i) { throw new Exception("Header info data structures are not in [....]"); } } #endif // Create dictionary for string-to-enum lookup. Ordinal and IgnoreCase are intentional. m_HeaderDictionary = new Dictionary ((int)MailHeaderID.ZMaxEnumValue + 1, StringComparer.OrdinalIgnoreCase); for(int i = 0; i < m_HeaderInfo.Length; i++) { m_HeaderDictionary.Add(m_HeaderInfo[i].NormalizedName, i); } } internal static string GetString(MailHeaderID id) { switch(id) { case MailHeaderID.Unknown: case MailHeaderID.ZMaxEnumValue+1: return null; default: return m_HeaderInfo[(int)id].NormalizedName; } } internal static MailHeaderID GetID(string name) { int id; if(m_HeaderDictionary.TryGetValue(name, out id)) { return (MailHeaderID)id; } return MailHeaderID.Unknown; } internal static bool IsWellKnown(string name) { int dummy; return m_HeaderDictionary.TryGetValue(name, out dummy); } internal static bool IsSingleton(string name) { int index; if(m_HeaderDictionary.TryGetValue(name, out index)) { return m_HeaderInfo[index].IsSingleton; } return false; } internal static string NormalizeCase(string name) { int index; if(m_HeaderDictionary.TryGetValue(name, out index)) { return m_HeaderInfo[index].NormalizedName; } return name; } internal static bool IsMatch(string name, MailHeaderID header) { int index; if(m_HeaderDictionary.TryGetValue(name, out index) && (MailHeaderID)index == header) { return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
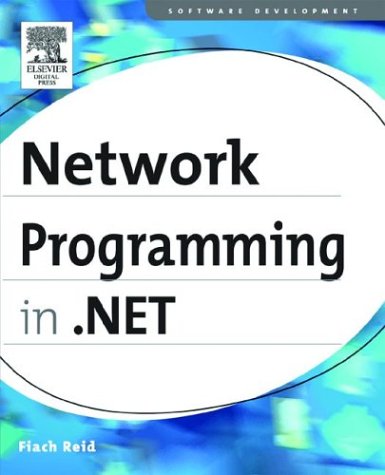
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataKey.cs
- BitmapSizeOptions.cs
- RawAppCommandInputReport.cs
- AggregatePushdown.cs
- CompilerGeneratedAttribute.cs
- Crypto.cs
- ByteRangeDownloader.cs
- NoResizeHandleGlyph.cs
- ObjectIDGenerator.cs
- ByteConverter.cs
- WebRequest.cs
- HttpCachePolicyElement.cs
- ClonableStack.cs
- HttpCacheVary.cs
- ListBox.cs
- ConfigurationStrings.cs
- TagMapCollection.cs
- NullEntityWrapper.cs
- TranslateTransform.cs
- CompilerTypeWithParams.cs
- ObjectListCommandsPage.cs
- ConfigurationSectionGroupCollection.cs
- MsmqProcessProtocolHandler.cs
- CatalogZoneDesigner.cs
- SHA512Managed.cs
- SelfIssuedSamlTokenFactory.cs
- PeerName.cs
- StorageSetMapping.cs
- ListViewTableCell.cs
- SoapInteropTypes.cs
- KeyedQueue.cs
- ErrorRuntimeConfig.cs
- XmlEnumAttribute.cs
- ImmutableCommunicationTimeouts.cs
- sqlstateclientmanager.cs
- DesignerAutoFormatStyle.cs
- FileDialog_Vista_Interop.cs
- PolicyValidator.cs
- StatusBarDrawItemEvent.cs
- OdbcParameter.cs
- ImageProxy.cs
- CreateUserWizard.cs
- Buffer.cs
- WeakEventTable.cs
- FamilyTypeface.cs
- VirtualizingPanel.cs
- ProtectedProviderSettings.cs
- BaseServiceProvider.cs
- ColumnTypeConverter.cs
- EntityContainerEntitySet.cs
- BaseDataList.cs
- IteratorDescriptor.cs
- OpacityConverter.cs
- SettingsPropertyValueCollection.cs
- XmlDictionaryWriter.cs
- BrowserCapabilitiesFactory.cs
- GridToolTip.cs
- IConvertible.cs
- Table.cs
- WsdlInspector.cs
- VectorAnimation.cs
- AddingNewEventArgs.cs
- Freezable.cs
- MaskDescriptor.cs
- ControlParameter.cs
- NestPullup.cs
- GraphicsContext.cs
- OdbcStatementHandle.cs
- ServiceOperation.cs
- CLSCompliantAttribute.cs
- AuthenticatedStream.cs
- XmlSchemaAttributeGroupRef.cs
- AssertFilter.cs
- GenericTypeParameterBuilder.cs
- EntryIndex.cs
- ToolStripTemplateNode.cs
- X509Utils.cs
- ProviderException.cs
- TypedTableBaseExtensions.cs
- SynchronousReceiveElement.cs
- ProcessModuleCollection.cs
- X509CertificateChain.cs
- OperationCanceledException.cs
- SingletonConnectionReader.cs
- IndentedWriter.cs
- CharAnimationUsingKeyFrames.cs
- Codec.cs
- Cursors.cs
- DateTimeSerializationSection.cs
- StringDictionaryWithComparer.cs
- UrlPath.cs
- ExitEventArgs.cs
- LassoHelper.cs
- ActivityCodeDomReferenceService.cs
- RequestCacheEntry.cs
- SystemIPv4InterfaceProperties.cs
- GridEntryCollection.cs
- NativeRecognizer.cs
- UIElement.cs
- ToolStripOverflow.cs