Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / RawAppCommandInputReport.cs / 1 / RawAppCommandInputReport.cs
using System; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Win32; using System.Windows; namespace System.Windows.Input { ////// The RawAppCommandInputReport class encapsulates the raw input provided from WM_APPCOMMAND message. /// This WM_APPCOMMAND message gets generated when the DefWindowProc processes the WM_XBUTTONUP or /// WM_NCXBUTTONUP message, or when the user types an application command key. /// /// ////// It is important to note that the InputReport class only contains /// blittable types. This is required so that the report can be marshalled across application domains. /// /// To get the coordinates of the cursor if the message was generated /// by a button click on the mouse, the application can call GetMessagePos. /// An application can test whether the message was generated by the mouse by checking whether Device contains FAPPCOMMAND_MOUSE. /// Unlike other windows messages, an application should return TRUE from this message if it processes it. /// internal class RawAppCommandInputReport : InputReport { ////// Constructs ad instance of the RawAppCommandInputReport class. /// /// /// The input source that provided this input. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The Application Command associated. /// /// /// The device that generated the app command. /// /// the input device that generated the input event internal RawAppCommandInputReport( PresentationSource inputSource, InputMode mode, int timestamp, int appCommand, InputType device, InputType inputType) : base(inputSource, inputType, mode, timestamp) { _appCommand = appCommand; _device = device; } ////// Read-only access to the AppCommand that was reported. /// internal int AppCommand { get { return _appCommand; } } ////// Read-only access to the device that generated the AppCommand /// internal InputType Device { get { return _device; } } private int _appCommand; private InputType _device; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Win32; using System.Windows; namespace System.Windows.Input { ////// The RawAppCommandInputReport class encapsulates the raw input provided from WM_APPCOMMAND message. /// This WM_APPCOMMAND message gets generated when the DefWindowProc processes the WM_XBUTTONUP or /// WM_NCXBUTTONUP message, or when the user types an application command key. /// /// ////// It is important to note that the InputReport class only contains /// blittable types. This is required so that the report can be marshalled across application domains. /// /// To get the coordinates of the cursor if the message was generated /// by a button click on the mouse, the application can call GetMessagePos. /// An application can test whether the message was generated by the mouse by checking whether Device contains FAPPCOMMAND_MOUSE. /// Unlike other windows messages, an application should return TRUE from this message if it processes it. /// internal class RawAppCommandInputReport : InputReport { ////// Constructs ad instance of the RawAppCommandInputReport class. /// /// /// The input source that provided this input. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The Application Command associated. /// /// /// The device that generated the app command. /// /// the input device that generated the input event internal RawAppCommandInputReport( PresentationSource inputSource, InputMode mode, int timestamp, int appCommand, InputType device, InputType inputType) : base(inputSource, inputType, mode, timestamp) { _appCommand = appCommand; _device = device; } ////// Read-only access to the AppCommand that was reported. /// internal int AppCommand { get { return _appCommand; } } ////// Read-only access to the device that generated the AppCommand /// internal InputType Device { get { return _device; } } private int _appCommand; private InputType _device; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
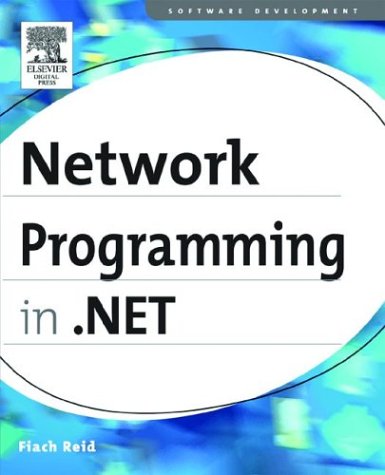
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SkinIDTypeConverter.cs
- ClientClassGenerator.cs
- OutKeywords.cs
- HtmlInputSubmit.cs
- StringConverter.cs
- StartFileNameEditor.cs
- OleDbConnectionFactory.cs
- OdbcConnectionOpen.cs
- WebUtil.cs
- NaturalLanguageHyphenator.cs
- Message.cs
- EventBuilder.cs
- TreeNodeClickEventArgs.cs
- SymLanguageType.cs
- Pen.cs
- PositiveTimeSpanValidatorAttribute.cs
- typedescriptorpermission.cs
- ResourcePermissionBase.cs
- FaultConverter.cs
- CodeTypeMember.cs
- TextEndOfParagraph.cs
- ClientSponsor.cs
- MaskedTextProvider.cs
- WindowsSlider.cs
- AssemblyAssociatedContentFileAttribute.cs
- InfoCardConstants.cs
- _KerberosClient.cs
- ListViewTableRow.cs
- DataBoundControlHelper.cs
- XXXInfos.cs
- SafeProcessHandle.cs
- CrossContextChannel.cs
- AnimatedTypeHelpers.cs
- SafeLocalAllocation.cs
- ToolStripLocationCancelEventArgs.cs
- Stackframe.cs
- CommentGlyph.cs
- XmlResolver.cs
- BitmapEncoder.cs
- HMACSHA1.cs
- EndCreateSecurityTokenRequest.cs
- DBParameter.cs
- ViewBase.cs
- BitmapEffectvisualstate.cs
- StateRuntime.cs
- DelegatingConfigHost.cs
- EntityParameter.cs
- LocalValueEnumerator.cs
- CodeSnippetStatement.cs
- WorkflowApplicationCompletedEventArgs.cs
- UnsafeNativeMethods.cs
- DataFieldCollectionEditor.cs
- ViewBase.cs
- ImageAutomationPeer.cs
- Int64KeyFrameCollection.cs
- AssemblyBuilder.cs
- CodeChecksumPragma.cs
- ArgumentValue.cs
- DataGridDesigner.cs
- ColorMatrix.cs
- DataGridCellsPresenter.cs
- TransactedBatchingBehavior.cs
- PanelStyle.cs
- Int32Rect.cs
- RoleManagerModule.cs
- DataBindingCollection.cs
- __ConsoleStream.cs
- SynchronizationContextHelper.cs
- LessThan.cs
- ImplicitInputBrush.cs
- BooleanKeyFrameCollection.cs
- FilterQuery.cs
- JsonFormatWriterGenerator.cs
- SessionPageStateSection.cs
- String.cs
- SmiContextFactory.cs
- WindowsListViewItemStartMenu.cs
- BitmapSource.cs
- InputBinder.cs
- OdbcTransaction.cs
- SrgsRulesCollection.cs
- LazyInitializer.cs
- AuthenticationConfig.cs
- ExcCanonicalXml.cs
- ApplicationActivator.cs
- ValueOfAction.cs
- ObjectKeyFrameCollection.cs
- _DisconnectOverlappedAsyncResult.cs
- DataPagerFieldCollection.cs
- ListItemCollection.cs
- BCLDebug.cs
- ConditionalAttribute.cs
- XamlFigureLengthSerializer.cs
- SocketInformation.cs
- XamlVector3DCollectionSerializer.cs
- CopyCodeAction.cs
- DataListDesigner.cs
- PeerCredential.cs
- DataSourceExpression.cs
- ReadOnlyDictionary.cs