Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / InfoCardConstants.cs / 1 / InfoCardConstants.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.Text; using System.Xml; using System.IdentityModel.Claims; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using Microsoft.Win32; // // Summary: // This class contains the dictionary for the claims associated with the // infocards. // internal static class InfoCardConstants { // // Indigo message limits, managed through the registry. // private static int s_maxMessageSize; private static int s_maxMexChainLength; private const int DEFAULT_MAX_MESSAGE_SIZE = 20 * 1024 * 1024; // in bytes private const int DEFAULT_MAX_MEX_CHAIN = 100; private const string MAX_MESSAGE_KEY = "MaximumMessageSize"; private const string MAX_MEX_CHAIN = "MaximumMexChain"; private const string INFOCARD_REGISTRY_KEY = @"software\microsoft\infocard"; static public int MaximumMessageSize { get { return s_maxMessageSize; } } static public int MaximumMexChainLength { get { return s_maxMexChainLength; } } static public string RegistryKey { get { return INFOCARD_REGISTRY_KEY; } } static public string GivenName { get { return ClaimTypes.GivenName; } } static public string EmailAddress { get { return ClaimTypes.Email; } } static public string Surname { get { return ClaimTypes.Surname; } } static public string StreetAddress { get { return ClaimTypes.StreetAddress; } } static public string Locality { get { return ClaimTypes.Locality; } } static public string StateOrProvince { get { return ClaimTypes.StateOrProvince; } } /* SSS_WARNINGS_OFF */ static public string PostalCode { get { return ClaimTypes.PostalCode; } } static public string Country { get { return ClaimTypes.Country; } } /* SSS_WARNINGS_ON */ static public string HomePhone { get { return ClaimTypes.HomePhone; } } static public string OtherPhone { get { return ClaimTypes.OtherPhone; } } static public string MobilePhone { get { return ClaimTypes.MobilePhone; } } static public string DateOfBirth { get { return ClaimTypes.DateOfBirth; } } static public string Gender { get { return ClaimTypes.Gender; } } static public string PPID { get { return ClaimTypes.PPID; } } static public string Webpage{ get { return ClaimTypes.Webpage; } } private static string[ ] s_SelfIssuedClaims = { GivenName, Surname, EmailAddress, StreetAddress, Locality, StateOrProvince, /* SSS_WARNINGS_OFF */ PostalCode, Country, /* SSS_WARNINGS_ON */ HomePhone, OtherPhone, MobilePhone, DateOfBirth, Gender, Webpage }; private static Dictionarys_claimDisplayTags = new Dictionary (); private static Dictionary s_claimDescription = new Dictionary (); /* SSS_WARNINGS_OFF */ private static XmlDictionaryReaderQuotas s_defaultQuotas = new XmlDictionaryReaderQuotas(); private static List s_localTokenFactoryWhiteList = new List (); /* SSS_WARNINGS_ON */ private static List s_policyElementsToBeProcessed = new List (); // // Summary // Checks if the input values are in the list that the local token factory supports // // Parameters // nameSpaceValue - NameSpace for the xml element // value -the xml element name // /* SSS_WARNINGS_OFF */ public static bool DoesLocalTokenFactoryWhiteListContain( string nameSpaceValue, string value ) { return s_localTokenFactoryWhiteList.Contains( nameSpaceValue + "/" + value ); /* SSS_WARNINGS_ON */ } // // Summary // Checks if the input values are in the list of elements that infocard processes // // Parameters // nameSpaceValue - NameSpace for the xml element // value -the xml element name // public static bool DoesPolicyElementsToBeProcessedListContain( string nameSpaceValue, string value ) { return s_policyElementsToBeProcessed.Contains( nameSpaceValue + "/" + value ); } public static XmlDictionaryReaderQuotas DefaultQuotas { get { return s_defaultQuotas; } } public static string[ ] SelfIssuedClaimsUris { get { return s_SelfIssuedClaims; } } public static string PPIDClaimsUri { get { return PPID; } } public static string ClaimDisplayTag( string key ) { string displayTag = null; if( s_claimDisplayTags.ContainsKey( key ) ) { displayTag = SR.GetString( s_claimDisplayTags[ key ] ); } else { throw IDT.ThrowHelperWarning( new ArgumentOutOfRangeException( SR.GetString( SR.InvalidSelfIssuedUri ) ) ); } return displayTag; } public static string ClaimsDescription( string key ) { string description = null; if( s_claimDescription.ContainsKey( key ) ) { description = SR.GetString( s_claimDescription[ key ] ); } else { throw IDT.ThrowHelperWarning( new ArgumentOutOfRangeException( SR.GetString( SR.InvalidSelfIssuedUri ) ) ); } return description; } static InfoCardConstants() { s_claimDisplayTags.Add( GivenName, SR.GivenNameText ); /* SSS_WARNINGS_OFF */ s_claimDisplayTags.Add( EmailAddress, SR.EmailAddressText ); s_claimDisplayTags.Add( Country, SR.CountryText ); /* SSS_WARNINGS_ON */ s_claimDisplayTags.Add( DateOfBirth, SR.DateOfBirthText ); s_claimDisplayTags.Add( Gender, SR.GenderText); s_claimDisplayTags.Add( HomePhone, SR.HomePhoneText ); s_claimDisplayTags.Add( Locality, SR.LocalityText ); s_claimDisplayTags.Add( MobilePhone, SR.MobilePhoneText ); s_claimDisplayTags.Add( OtherPhone, SR.OtherPhoneText ); s_claimDisplayTags.Add( PostalCode, SR.PostalCodeText ); s_claimDisplayTags.Add( StateOrProvince, SR.StateOrProvinceText ); s_claimDisplayTags.Add( StreetAddress, SR.StreetAddressText ); s_claimDisplayTags.Add( Surname, SR.SurnameText ); s_claimDisplayTags.Add( PPID, SR.PPIDText ); s_claimDisplayTags.Add( Webpage, SR.WebPageText ); s_claimDescription.Add( GivenName, SR.GivenNameDescription ); /* SSS_WARNINGS_OFF */ s_claimDescription.Add( EmailAddress, SR.EmailAddressDescription ); s_claimDescription.Add( Country, SR.CountryDescription ); /* SSS_WARNINGS_ON */ s_claimDescription.Add( DateOfBirth, SR.DateOfBirthDescription ); s_claimDescription.Add( Gender, SR.GenderDescription ); s_claimDescription.Add( HomePhone, SR.HomePhoneDescription ); s_claimDescription.Add( Locality, SR.LocalityDescription ); s_claimDescription.Add( MobilePhone, SR.MobilePhoneDescription ); s_claimDescription.Add( OtherPhone, SR.OtherPhoneDescription ); s_claimDescription.Add( PostalCode, SR.PostalCodeDescription ); s_claimDescription.Add( StateOrProvince, SR.StateOrProvinceDescription ); s_claimDescription.Add( StreetAddress, SR.StreetAddressDescription ); s_claimDescription.Add( Surname, SR.SurnameDescription ); s_claimDescription.Add( PPID, SR.PPIDDescription ); s_claimDescription.Add( Webpage, SR.WebPageDescription ); // // Quotas for xml readers // s_defaultQuotas.MaxDepth = 32; // max depth of elements s_defaultQuotas.MaxStringContentLength = 8192; // maximum string read s_defaultQuotas.MaxArrayLength = 20 * 1024 * 1024; // maximum byte array s_defaultQuotas.MaxBytesPerRead = 4096; // max start element tag s_defaultQuotas.MaxNameTableCharCount = 16384; // max size of name table // // Message limits // s_maxMessageSize = DEFAULT_MAX_MESSAGE_SIZE; s_maxMexChainLength = DEFAULT_MAX_MEX_CHAIN; using( SystemIdentity system = new SystemIdentity( false ) ) { using( RegistryKey rk = Registry.LocalMachine.OpenSubKey( INFOCARD_REGISTRY_KEY, false ) ) { if( null != rk ) { object maxMessage = rk.GetValue( MAX_MESSAGE_KEY, DEFAULT_MAX_MESSAGE_SIZE ); // // Registry is in kbs. Indigo properties in bytes // if( maxMessage is int && (int)maxMessage > 0 && (int)maxMessage * 1024 > 0 ) { s_maxMessageSize = (int) maxMessage * 1024; } object maxHops = rk.GetValue( MAX_MEX_CHAIN, DEFAULT_MAX_MEX_CHAIN ); if( maxHops is int && (int)maxHops > 0 ) { s_maxMexChainLength = (int) maxHops; } } } } // // Add the allowed elements from WSTrust Feb 2005 // /* SSS_WARNINGS_OFF */ s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustXmlSoap2005.c_Namespace + "/" + XmlNames.WSTrustXmlSoap2005.c_SignatureAlgorithm ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustXmlSoap2005.c_Namespace + "/" + XmlNames.WSTrustXmlSoap2005.c_EncryptionAlgorithm ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustXmlSoap2005.c_Namespace + "/" + XmlNames.WSTrustXmlSoap2005.c_CanonicalizationAlgorithm ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustXmlSoap2005.c_Namespace + "/" + XmlNames.WSTrustXmlSoap2005.c_SignWith ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustXmlSoap2005.c_Namespace + "/" + XmlNames.WSTrustXmlSoap2005.c_ClaimsElement ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustXmlSoap2005.c_Namespace + "/" + XmlNames.WSTrustXmlSoap2005.c_TokenType ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustXmlSoap2005.c_Namespace + "/" + XmlNames.WSTrustXmlSoap2005.c_KeyType ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustXmlSoap2005.c_Namespace + "/" + XmlNames.WSTrustXmlSoap2005.c_KeySize ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustXmlSoap2005.c_Namespace + "/" + XmlNames.WSTrustXmlSoap2005.c_EncryptWith ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustXmlSoap2005.c_Namespace + "/" + XmlNames.WSTrustXmlSoap2005.c_RequestType ); // // Add the allowed elements from WSTrust Oasis 2007 (1.3) // s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_SignatureAlgorithm ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_EncryptionAlgorithm ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_CanonicalizationAlgorithm ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_SignWith ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_ClaimsElement ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_TokenType ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_KeyType ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_KeySize ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_EncryptWith ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_RequestType ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_SecondaryParameters ); s_localTokenFactoryWhiteList.Add( XmlNames.WSTrustOasis2007.c_Namespace + "/" + XmlNames.WSTrustOasis2007.c_KeyWrapAlgorithm ); s_localTokenFactoryWhiteList.Add( XmlNames.WSSecurityPolicyXmlSoap2005.c_Namespace + "/" + XmlNames.WSSecurityPolicyXmlSoap2005.c_RequestSecurityTokenTemplate ); s_localTokenFactoryWhiteList.Add( XmlNames.WSSecurityPolicyOasis2007.c_Namespace + "/" + XmlNames.WSSecurityPolicyOasis2007.c_RequestSecurityTokenTemplate ); s_policyElementsToBeProcessed.AddRange( s_localTokenFactoryWhiteList ); /* SSS_WARNINGS_ON */ s_policyElementsToBeProcessed.Add( XmlNames.WSPolicyXmlSoap2004.c_Namespace + "/" + XmlNames.WSPolicyXmlSoap2004.c_AppliesTo ); s_policyElementsToBeProcessed.Add( XmlNames.WSPolicyW32007.c_Namespace + "/" + XmlNames.WSPolicyW32007.c_AppliesTo ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
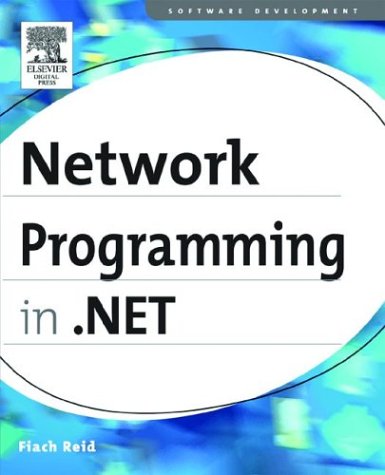
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MsmqChannelFactoryBase.cs
- Triangle.cs
- MulticastNotSupportedException.cs
- RemoteWebConfigurationHostServer.cs
- TypeUtil.cs
- ProxyGenerationError.cs
- TextEncodedRawTextWriter.cs
- PageAsyncTaskManager.cs
- TransactionContextValidator.cs
- ThreadAbortException.cs
- IPCCacheManager.cs
- PersonalizationProviderHelper.cs
- isolationinterop.cs
- SettingsAttributeDictionary.cs
- Error.cs
- AuthenticateEventArgs.cs
- RowTypeElement.cs
- Variant.cs
- RequestTimeoutManager.cs
- SoapSchemaExporter.cs
- WebPermission.cs
- XmlStringTable.cs
- DataConnectionHelper.cs
- BasicBrowserDialog.designer.cs
- TraceListeners.cs
- WebPartTransformerCollection.cs
- XamlStyleSerializer.cs
- DbQueryCommandTree.cs
- XPathExpr.cs
- PrivilegedConfigurationManager.cs
- WebServiceTypeData.cs
- LifetimeServices.cs
- WSHttpBindingBaseElement.cs
- ValueType.cs
- DateTimeStorage.cs
- HitTestResult.cs
- ObjectHandle.cs
- ValidationErrorCollection.cs
- FixedPageProcessor.cs
- Condition.cs
- DataContractJsonSerializerOperationFormatter.cs
- XmlSerializerVersionAttribute.cs
- HttpResponseWrapper.cs
- Formatter.cs
- UniqueEventHelper.cs
- TextBounds.cs
- FunctionGenerator.cs
- MethodToken.cs
- IssuanceLicense.cs
- RemotingClientProxy.cs
- WindowsContainer.cs
- QueryProcessor.cs
- Event.cs
- Dictionary.cs
- Regex.cs
- DecoderBestFitFallback.cs
- SelectionItemPattern.cs
- CompressEmulationStream.cs
- IImplicitResourceProvider.cs
- FontUnit.cs
- PieceNameHelper.cs
- MultipleViewPatternIdentifiers.cs
- TcpHostedTransportConfiguration.cs
- XmlIncludeAttribute.cs
- ObjectPropertyMapping.cs
- TdsEnums.cs
- ToolStripDropDownClosedEventArgs.cs
- WindowsAuthenticationEventArgs.cs
- PriorityRange.cs
- XmlSerializationGeneratedCode.cs
- CallContext.cs
- SpellerStatusTable.cs
- ServiceModelConfigurationSectionGroup.cs
- NativeMethods.cs
- XmlSchemaGroup.cs
- HttpWebResponse.cs
- OleDbErrorCollection.cs
- WindowsListViewSubItem.cs
- ObjectParameterCollection.cs
- CharacterShapingProperties.cs
- XmlWriterDelegator.cs
- QilStrConcatenator.cs
- ParallelTimeline.cs
- FixedDocumentSequencePaginator.cs
- SequentialWorkflowRootDesigner.cs
- XamlTreeBuilder.cs
- SingleStorage.cs
- WindowsListViewItemStartMenu.cs
- SoapEnumAttribute.cs
- ColumnResizeAdorner.cs
- JsonFormatWriterGenerator.cs
- TableLayoutPanelResizeGlyph.cs
- SqlGenerator.cs
- Menu.cs
- WebPartMinimizeVerb.cs
- TimerElapsedEvenArgs.cs
- ColumnWidthChangingEvent.cs
- EncoderParameters.cs
- columnmapfactory.cs
- ConfigurationCollectionAttribute.cs