Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / EventRouteFactory.cs / 1305600 / EventRouteFactory.cs
using System; using System.Windows; using MS.Utility; namespace System.Windows { ////// Creates and recycles instance of EventRoute /// internal static class EventRouteFactory { #region Operations ////// Fetch a recycled object if available /// else create a new instance /// internal static EventRoute FetchObject(RoutedEvent routedEvent) { EventRoute eventRoute = Pop(); if (eventRoute == null) { eventRoute = new EventRoute(routedEvent); } else { eventRoute.RoutedEvent = routedEvent; } return eventRoute; } ////// Recycle the given instance of EventRoute /// internal static void RecycleObject(EventRoute eventRoute) { // Cleanup all refernces held eventRoute.Clear(); // Push instance on to the stack Push(eventRoute); } #endregion Operations #region HelperMethods ////// Push the given instance of EventRoute on to the stack /// private static void Push(EventRoute eventRoute) { lock (_synchronized) { // In a normal scenario it is extremely rare to // require more than 2 EventRoutes at the same time if (_eventRouteStack == null) { _eventRouteStack = new EventRoute[2]; _stackTop = 0; } if (_stackTop < 2) { _eventRouteStack[_stackTop++] = eventRoute; } } } ////// Pop off the last instance of EventRoute in the stack /// private static EventRoute Pop() { lock (_synchronized) { if (_stackTop > 0) { EventRoute eventRoute = _eventRouteStack[--_stackTop]; _eventRouteStack[_stackTop] = null; return eventRoute; } } return null; } #endregion HelperMethods #region Data private static EventRoute[] _eventRouteStack; private static int _stackTop; private static object _synchronized = new object(); #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
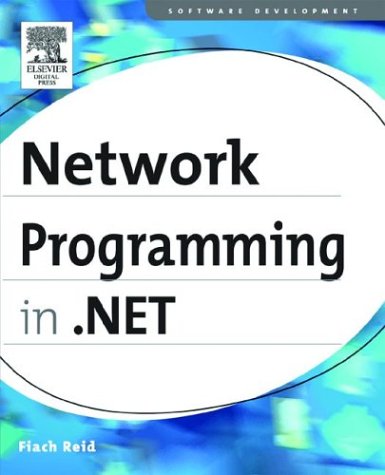
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServiceProviders.cs
- ContentFileHelper.cs
- SqlClientWrapperSmiStream.cs
- PrimitiveSchema.cs
- DBSchemaRow.cs
- DataGridViewRowPrePaintEventArgs.cs
- CornerRadius.cs
- EncodedStreamFactory.cs
- PageThemeBuildProvider.cs
- PageSetupDialog.cs
- SmuggledIUnknown.cs
- Point4DConverter.cs
- UniqueIdentifierService.cs
- TextParentUndoUnit.cs
- _SSPISessionCache.cs
- nulltextnavigator.cs
- MenuItemStyleCollection.cs
- ScrollItemPattern.cs
- TextEndOfParagraph.cs
- StringReader.cs
- DataGridViewColumnCollection.cs
- Literal.cs
- XsltContext.cs
- RegexBoyerMoore.cs
- ManifestResourceInfo.cs
- PrintPreviewGraphics.cs
- TreeViewAutomationPeer.cs
- InfoCardProofToken.cs
- CompilerErrorCollection.cs
- HostedAspNetEnvironment.cs
- Graph.cs
- RecognizedPhrase.cs
- ComponentDispatcherThread.cs
- SerTrace.cs
- Object.cs
- PanelDesigner.cs
- MsmqInputChannelListener.cs
- LinkUtilities.cs
- DesignerValidatorAdapter.cs
- MobileDeviceCapabilitiesSectionHandler.cs
- Lock.cs
- AuthenticatingEventArgs.cs
- ListControlActionList.cs
- Overlapped.cs
- X509Chain.cs
- URLEditor.cs
- RangeValueProviderWrapper.cs
- SelectionEditingBehavior.cs
- JournalEntry.cs
- SecurityElement.cs
- WeakReference.cs
- ConcurrentDictionary.cs
- HttpClientProtocol.cs
- Wizard.cs
- ProjectionPathBuilder.cs
- WebContext.cs
- CharKeyFrameCollection.cs
- TextSpan.cs
- TabControlAutomationPeer.cs
- FormViewInsertedEventArgs.cs
- CompilerGeneratedAttribute.cs
- ForceCopyBuildProvider.cs
- SqlTypesSchemaImporter.cs
- DataServiceEntityAttribute.cs
- ProjectedSlot.cs
- Cursor.cs
- Point3DAnimation.cs
- EtwProvider.cs
- ZipIOLocalFileBlock.cs
- DiagnosticsConfigurationHandler.cs
- GeneralTransform3DGroup.cs
- ThreadStaticAttribute.cs
- DataObjectAttribute.cs
- ValidationSummary.cs
- BrowserCapabilitiesCodeGenerator.cs
- WebPartsSection.cs
- WindowsTokenRoleProvider.cs
- AuthenticateEventArgs.cs
- Internal.cs
- GlyphRunDrawing.cs
- Timeline.cs
- SHA384Managed.cs
- TreeNode.cs
- dsa.cs
- WebUtil.cs
- InstanceOwnerException.cs
- PlanCompiler.cs
- NetPeerTcpBinding.cs
- SQLSingle.cs
- CustomLineCap.cs
- URLMembershipCondition.cs
- InputLanguageProfileNotifySink.cs
- DesignerActionTextItem.cs
- DefaultValidator.cs
- DataObjectPastingEventArgs.cs
- GlyphTypeface.cs
- XmlLoader.cs
- AbsoluteQuery.cs
- TogglePatternIdentifiers.cs
- OleDbPropertySetGuid.cs