Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / WebPartsSection.cs / 3 / WebPartsSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Configuration; using System.Collections; using System.Collections.Specialized; using System.Security.Principal; using System.Web; using System.Web.Compilation; using System.Web.Configuration; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.Util; using System.Xml; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WebPartsSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnableExport = new ConfigurationProperty("enableExport", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propPersonalization = new ConfigurationProperty("personalization", typeof(WebPartsPersonalization), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propTransformers = new ConfigurationProperty("transformers", typeof(TransformerInfoCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); static WebPartsSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnableExport); _properties.Add(_propPersonalization); _properties.Add(_propTransformers); } public WebPartsSection() { } /* protected override void InitializeDefault() { /* Don't Add to Basicmap AuthorizationRule rule0 = new AuthorizationRule(AuthorizationRuleAction.Deny); rule0.Users.Add("*"); rule0.Verbs.Add("enterSharedScope"); Personalization.Authorization.Rules.Add(rule0); AuthorizationRule rule1 = new AuthorizationRule(AuthorizationRuleAction.Allow); rule1.Users.Add("*"); rule1.Verbs.Add("modifyState"); Personalization.Authorization.Rules.Add(rule1); */ /* } */ [ConfigurationProperty("enableExport", DefaultValue = false)] public bool EnableExport { get { return (bool)base[_propEnableExport]; } set { base[_propEnableExport] = value; } } [ConfigurationProperty("personalization")] public WebPartsPersonalization Personalization { get { return (WebPartsPersonalization)base[_propPersonalization]; } } /// protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("transformers")] public TransformerInfoCollection Transformers { get { return (TransformerInfoCollection)base[_propTransformers]; } } protected override object GetRuntimeObject() { Personalization.ValidateAuthorization(); return base.GetRuntimeObject(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Configuration; using System.Collections; using System.Collections.Specialized; using System.Security.Principal; using System.Web; using System.Web.Compilation; using System.Web.Configuration; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.Util; using System.Xml; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WebPartsSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnableExport = new ConfigurationProperty("enableExport", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propPersonalization = new ConfigurationProperty("personalization", typeof(WebPartsPersonalization), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propTransformers = new ConfigurationProperty("transformers", typeof(TransformerInfoCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); static WebPartsSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnableExport); _properties.Add(_propPersonalization); _properties.Add(_propTransformers); } public WebPartsSection() { } /* protected override void InitializeDefault() { /* Don't Add to Basicmap AuthorizationRule rule0 = new AuthorizationRule(AuthorizationRuleAction.Deny); rule0.Users.Add("*"); rule0.Verbs.Add("enterSharedScope"); Personalization.Authorization.Rules.Add(rule0); AuthorizationRule rule1 = new AuthorizationRule(AuthorizationRuleAction.Allow); rule1.Users.Add("*"); rule1.Verbs.Add("modifyState"); Personalization.Authorization.Rules.Add(rule1); */ /* } */ [ConfigurationProperty("enableExport", DefaultValue = false)] public bool EnableExport { get { return (bool)base[_propEnableExport]; } set { base[_propEnableExport] = value; } } [ConfigurationProperty("personalization")] public WebPartsPersonalization Personalization { get { return (WebPartsPersonalization)base[_propPersonalization]; } } /// protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("transformers")] public TransformerInfoCollection Transformers { get { return (TransformerInfoCollection)base[_propTransformers]; } } protected override object GetRuntimeObject() { Personalization.ValidateAuthorization(); return base.GetRuntimeObject(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
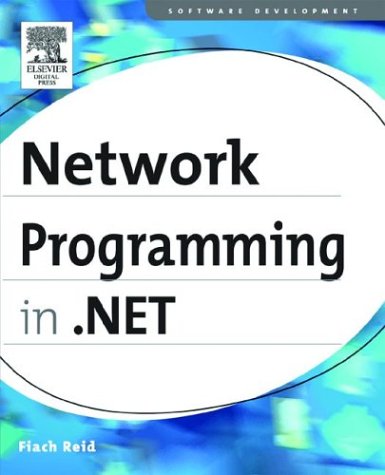
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WrappedIUnknown.cs
- DbParameterCollection.cs
- ChannelDispatcherBase.cs
- NativeRightsManagementAPIsStructures.cs
- ImmComposition.cs
- XmlAttributes.cs
- SqlInternalConnectionSmi.cs
- TextOnlyOutput.cs
- MembershipPasswordException.cs
- XmlNodeChangedEventArgs.cs
- CompilationLock.cs
- AnimationLayer.cs
- XmlCollation.cs
- GenericEnumConverter.cs
- Int32Rect.cs
- IDQuery.cs
- DetailsViewInsertedEventArgs.cs
- activationcontext.cs
- RawKeyboardInputReport.cs
- NamespaceInfo.cs
- DataTrigger.cs
- WebPartMinimizeVerb.cs
- DefaultProxySection.cs
- UIHelper.cs
- SqlCharStream.cs
- CqlBlock.cs
- HandleCollector.cs
- InputManager.cs
- StreamUpdate.cs
- MessageBox.cs
- ProfileSettings.cs
- GeneralTransform2DTo3DTo2D.cs
- ColorEditor.cs
- GeometryHitTestResult.cs
- XmlNullResolver.cs
- RegexNode.cs
- SecurityState.cs
- FormViewDeletedEventArgs.cs
- EntityTypeEmitter.cs
- PersonalizationAdministration.cs
- TextContainerChangedEventArgs.cs
- TripleDESCryptoServiceProvider.cs
- TemplateColumn.cs
- TextMetrics.cs
- SendActivityDesignerTheme.cs
- WindowsGraphics2.cs
- IPPacketInformation.cs
- RawAppCommandInputReport.cs
- PrintingPermissionAttribute.cs
- WorkflowQueue.cs
- StringAnimationUsingKeyFrames.cs
- DataContractFormatAttribute.cs
- CoreSwitches.cs
- KeyValuePair.cs
- ComplexTypeEmitter.cs
- TreePrinter.cs
- WebPartTransformerAttribute.cs
- XPathDescendantIterator.cs
- Guid.cs
- DebuggerAttributes.cs
- ComboBoxRenderer.cs
- DataGridViewAccessibleObject.cs
- TypeViewSchema.cs
- MailMessageEventArgs.cs
- CodeComment.cs
- Stopwatch.cs
- ExternalException.cs
- Vector3DKeyFrameCollection.cs
- ObjectFullSpanRewriter.cs
- PageThemeCodeDomTreeGenerator.cs
- AdPostCacheSubstitution.cs
- SecUtil.cs
- IndentedWriter.cs
- nulltextcontainer.cs
- ProxyHelper.cs
- LineServicesRun.cs
- PatternMatcher.cs
- ReflectionHelper.cs
- handlecollector.cs
- WebPartTracker.cs
- ValueTypeFieldReference.cs
- DataChangedEventManager.cs
- UInt64Storage.cs
- SharedPerformanceCounter.cs
- ScriptModule.cs
- X509Utils.cs
- TCPClient.cs
- NTAccount.cs
- OdbcConnectionHandle.cs
- BaseTemplateBuildProvider.cs
- OdbcInfoMessageEvent.cs
- DatatypeImplementation.cs
- QueryInterceptorAttribute.cs
- DataIdProcessor.cs
- AmbiguousMatchException.cs
- ToggleButtonAutomationPeer.cs
- COAUTHIDENTITY.cs
- DataControlLinkButton.cs
- WeakReferenceEnumerator.cs
- SqlBuffer.cs