Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.WorkflowServices / System / ServiceModel / Description / WorkflowRuntimeBehavior.cs / 1305376 / WorkflowRuntimeBehavior.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Description { using System.Globalization; using System.ServiceModel.Administration; using System.ServiceModel.Dispatcher; using System.Workflow.Runtime; using System.Workflow.Runtime.Configuration; using System.Workflow.Runtime.Hosting; public class WorkflowRuntimeBehavior : IServiceBehavior, IWmiInstanceProvider { internal static readonly TimeSpan DefaultCachedInstanceExpiration = TimeSpan.Parse(DefaultCachedInstanceExpirationString, CultureInfo.InvariantCulture); //default of 10 minutes chosen to be in-parity with SM inactivity timeout for session. internal const string DefaultCachedInstanceExpirationString = "00:10:00"; internal const string defaultName = "WorkflowRuntime"; internal const bool DefaultValidateOnCreate = true; static WorkflowRuntimeSection defaultWorkflowRuntimeSection; TimeSpan cachedInstanceExpiration; bool validateOnCreate; WorkflowRuntime workflowRuntime = null; public WorkflowRuntimeBehavior() : this(null, DefaultCachedInstanceExpiration, DefaultValidateOnCreate) { // empty } internal WorkflowRuntimeBehavior(WorkflowRuntime workflowRuntime, TimeSpan cachedInstanceExpiration, bool validateOnCreate) { this.workflowRuntime = workflowRuntime; this.cachedInstanceExpiration = cachedInstanceExpiration; this.validateOnCreate = validateOnCreate; } public TimeSpan CachedInstanceExpiration { get { return this.cachedInstanceExpiration; } set { if (value < TimeSpan.Zero) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); } this.cachedInstanceExpiration = value; } } public WorkflowRuntime WorkflowRuntime { get { if (this.workflowRuntime == null) { this.workflowRuntime = new WorkflowRuntime(WorkflowRuntimeBehavior.DefaultWorkflowRuntimeSection); } return this.workflowRuntime; } } internal bool ValidateOnCreate { get { return this.validateOnCreate; } } static WorkflowRuntimeSection DefaultWorkflowRuntimeSection { get { if (defaultWorkflowRuntimeSection == null) { WorkflowRuntimeSection tempSection = new WorkflowRuntimeSection(); tempSection.ValidateOnCreate = false; tempSection.EnablePerformanceCounters = true; tempSection.Name = defaultName; defaultWorkflowRuntimeSection = tempSection; } return defaultWorkflowRuntimeSection; } } public void AddBindingParameters(ServiceDescription serviceDescription, ServiceHostBase serviceHostBase, System.Collections.ObjectModel.Collectionendpoints, System.ServiceModel.Channels.BindingParameterCollection bindingParameters) { } public void ApplyDispatchBehavior(ServiceDescription serviceDescription, ServiceHostBase serviceHostBase) { if (serviceHostBase == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("serviceHostBase"); } if (serviceHostBase.Extensions == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("serviceHostBase.Extensions"); } WorkflowInstanceLifetimeManagerExtension cachedInstanceExpirationExtension = new WorkflowInstanceLifetimeManagerExtension( this.WorkflowRuntime, this.CachedInstanceExpiration, this.WorkflowRuntime.GetService () != null); serviceHostBase.Extensions.Add(cachedInstanceExpirationExtension); } void IWmiInstanceProvider.FillInstance(IWmiInstance wmiInstance) { wmiInstance.SetProperty("CachedInstanceExpiration", this.CachedInstanceExpiration.ToString()); } string IWmiInstanceProvider.GetInstanceType() { return "WorkflowRuntimeBehavior"; } public void Validate(ServiceDescription serviceDescription, ServiceHostBase serviceHostBase) { ValidateWorkflowRuntime(this.WorkflowRuntime); } void ValidateWorkflowRuntime(WorkflowRuntime workflowRuntime) { if (workflowRuntime.IsStarted) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.WorkflowRuntimeStartedBeforeHostOpen))); } WorkflowSchedulerService workflowSchedulerService = workflowRuntime.GetService (); if (!(workflowSchedulerService is SynchronizationContextWorkflowSchedulerService)) { if (workflowSchedulerService != null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.WrongSchedulerServiceRegistered))); } workflowRuntime.AddService(new SynchronizationContextWorkflowSchedulerService()); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
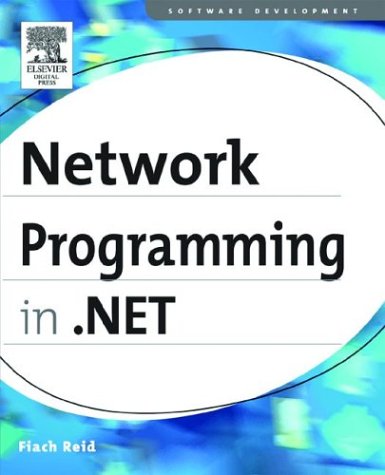
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignBindingValueUIHandler.cs
- ActiveDocumentEvent.cs
- AspProxy.cs
- TcpProcessProtocolHandler.cs
- SqlExpander.cs
- ClientUtils.cs
- CodeFieldReferenceExpression.cs
- ListViewDataItem.cs
- SafeThreadHandle.cs
- InvokeMethodActivity.cs
- CssStyleCollection.cs
- SqlInternalConnection.cs
- RowParagraph.cs
- StylusPointPropertyId.cs
- ResourceReferenceExpression.cs
- BitmapMetadataEnumerator.cs
- GPStream.cs
- OdbcCommandBuilder.cs
- UnicastIPAddressInformationCollection.cs
- EntityViewGenerationConstants.cs
- WebPart.cs
- FormClosingEvent.cs
- SerializationEventsCache.cs
- XamlFilter.cs
- MenuItemBinding.cs
- ToolStripOverflow.cs
- HeaderedContentControl.cs
- VisualTreeHelper.cs
- LateBoundBitmapDecoder.cs
- StorageSetMapping.cs
- ValidationErrorCollection.cs
- HwndKeyboardInputProvider.cs
- QueryOpeningEnumerator.cs
- ColumnClickEvent.cs
- ChangeTracker.cs
- CngKeyBlobFormat.cs
- HtmlSelect.cs
- NamespaceList.cs
- MethodResolver.cs
- PeerNameRecordCollection.cs
- Separator.cs
- GZipDecoder.cs
- ThemeDirectoryCompiler.cs
- PrimitiveType.cs
- Delegate.cs
- FontClient.cs
- GridViewDeleteEventArgs.cs
- CornerRadiusConverter.cs
- QilLoop.cs
- KeyEventArgs.cs
- CachedPathData.cs
- IriParsingElement.cs
- CornerRadiusConverter.cs
- ValidatingReaderNodeData.cs
- Html32TextWriter.cs
- SessionStateUtil.cs
- DataContractSet.cs
- StrongName.cs
- XmlSerializerAssemblyAttribute.cs
- StaticContext.cs
- InstallerTypeAttribute.cs
- DebugHandleTracker.cs
- AnnotationHighlightLayer.cs
- FileSystemInfo.cs
- MsmqProcessProtocolHandler.cs
- DataGridViewLayoutData.cs
- RuleSettingsCollection.cs
- URLMembershipCondition.cs
- SecurityBindingElement.cs
- AsnEncodedData.cs
- PtsCache.cs
- MasterPageCodeDomTreeGenerator.cs
- AsymmetricKeyExchangeDeformatter.cs
- BitmapVisualManager.cs
- HashAlgorithm.cs
- SchemaElementDecl.cs
- StrokeCollection.cs
- SelectedGridItemChangedEvent.cs
- DataShape.cs
- DataStreamFromComStream.cs
- PrimitiveCodeDomSerializer.cs
- DbConnectionHelper.cs
- DataFormat.cs
- XmlSchemaDocumentation.cs
- ReflectionHelper.cs
- HandleCollector.cs
- ISAPIApplicationHost.cs
- XmlWhitespace.cs
- LocalizationParserHooks.cs
- XmlSchemaAttributeGroup.cs
- DisplayNameAttribute.cs
- DataGridViewCellEventArgs.cs
- ControlPropertyNameConverter.cs
- ConfigXmlSignificantWhitespace.cs
- ProtocolImporter.cs
- DataViewSettingCollection.cs
- ConstructorBuilder.cs
- Perspective.cs
- ConnectionManagementElementCollection.cs
- XmlBinaryReader.cs