Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Metadata / StoreItemCollection.cs / 2 / StoreItemCollection.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Common; using System.Data.Common.Utils; using System.Diagnostics; using System.Xml; using System.Data.Entity; using System.Linq; namespace System.Data.Metadata.Edm { ////// Class for representing a collection of items in Store space. /// public sealed partial class StoreItemCollection : ItemCollection { #region Fields // Cache for primitive type maps for Edm to provider private readonly CacheForPrimitiveTypes _primitiveTypeMaps = new CacheForPrimitiveTypes(); private readonly Memoizer_cachedCTypeFunction; private readonly DbProviderManifest _providerManifest; private readonly DbProviderFactory _providerFactory; // Storing the query cache manager in the store item collection since all queries are currently bound to the // store. So storing it in StoreItemCollection makes sense. Also, since query cache requires version and other // stuff of the provider, we can assume that the connection is always open and we have the store metadata. // Also we can use the same cache manager both for Entity Client and Object Query, since query cache has // no reference to any metadata in OSpace. Also we assume that ObjectMaterializer loads the assembly // before it tries to do object materialization, since we might not have loaded an assembly in another workspace // where this store item collection is getting reused private readonly System.Data.Common.QueryCache.QueryCacheManager _queryCacheManager = System.Data.Common.QueryCache.QueryCacheManager.Create(); #endregion #region Constructors // used by EntityStoreSchemaGenerator to start with an empty (primitive types only) StoreItemCollection and // add types discovered from the database internal StoreItemCollection(DbProviderFactory factory, DbProviderManifest manifest) : base(DataSpace.SSpace) { Debug.Assert(factory != null, "factory is null"); Debug.Assert(manifest != null, "manifest is null"); _providerFactory = factory; _providerManifest = manifest; _cachedCTypeFunction = new Memoizer (ConvertFunctionParameterToCType, null); LoadProviderManifest(_providerManifest, true /*checkForSystemNamespace*/); } /// /// constructor that loads the metadata files from the specified xmlReaders, and returns the list of errors /// encountered during load as the out parameter errors. /// /// Publicly available from System.Data.Entity.Desgin.dll /// /// xmlReaders where the CDM schemas are loaded /// the paths where the files can be found that match the xml readers collection /// An out parameter to return the collection of errors encountered while loading [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] // referenced by System.Data.Entity.Design.dll internal StoreItemCollection(IEnumerablexmlReaders, System.Collections.ObjectModel.ReadOnlyCollection filePaths, out IList errors) : base(DataSpace.SSpace) { EntityUtil.CheckArgumentNull(xmlReaders, "xmlReaders"); EntityUtil.CheckArgumentEmpty(ref xmlReaders, Strings.StoreItemCollectionMustHaveOneArtifact, "xmlReader"); errors = this.Init(xmlReaders, filePaths, false, out _providerManifest, out _providerFactory, out _cachedCTypeFunction); } /// /// constructor that loads the metadata files from the specified xmlReaders, and returns the list of errors /// encountered during load as the out parameter errors. /// /// Publicly available from System.Data.Entity.Desgin.dll /// /// xmlReaders where the CDM schemas are loaded /// the paths where the files can be found that match the xml readers collection internal StoreItemCollection(IEnumerablexmlReaders, IEnumerable filePaths) : base(DataSpace.SSpace) { EntityUtil.CheckArgumentNull(filePaths, "filePaths"); EntityUtil.CheckArgumentEmpty(ref xmlReaders, Strings.StoreItemCollectionMustHaveOneArtifact, "xmlReader"); this.Init(xmlReaders, filePaths, true, out _providerManifest, out _providerFactory, out _cachedCTypeFunction); } /// /// Public constructor that loads the metadata files from the specified xmlReaders. /// Throws when encounter errors. /// /// xmlReaders where the CDM schemas are loaded public StoreItemCollection(IEnumerablexmlReaders) : base(DataSpace.SSpace) { EntityUtil.CheckArgumentNull(xmlReaders, "xmlReaders"); EntityUtil.CheckArgumentEmpty(ref xmlReaders, Strings.StoreItemCollectionMustHaveOneArtifact, "xmlReader"); MetadataArtifactLoader composite = MetadataArtifactLoader.CreateCompositeFromXmlReaders(xmlReaders); this.Init(composite.GetReaders(), composite.GetPaths(), true, out _providerManifest, out _providerFactory, out _cachedCTypeFunction); } /// /// Constructs the new instance of StoreItemCollection /// with the list of CDM files provided. /// /// paths where the CDM schemas are loaded ///Thrown if path name is not valid ///thrown if paths argument is null ///For errors related to invalid schemas. public StoreItemCollection(params string[] filePaths) : base(DataSpace.SSpace) { EntityUtil.CheckArgumentNull(filePaths, "filePaths"); IEnumerableenumerableFilePaths = filePaths; EntityUtil.CheckArgumentEmpty(ref enumerableFilePaths, Strings.StoreItemCollectionMustHaveOneArtifact, "filePaths"); // Wrap the file paths in instances of the MetadataArtifactLoader class, which provides // an abstraction and a uniform interface over a diverse set of metadata artifacts. // MetadataArtifactLoader composite = null; List readers = null; try { composite = MetadataArtifactLoader.CreateCompositeFromFilePaths(enumerableFilePaths, XmlConstants.SSpaceSchemaExtension); readers = composite.CreateReaders(DataSpace.SSpace); IEnumerable ieReaders = readers.AsEnumerable(); EntityUtil.CheckArgumentEmpty(ref ieReaders, Strings.StoreItemCollectionMustHaveOneArtifact, "filePaths"); this.Init(readers, composite.GetPaths(DataSpace.SSpace), true, out _providerManifest, out _providerFactory, out _cachedCTypeFunction); } finally { if (readers != null) { Helper.DisposeXmlReaders(readers); } } } private IList Init(IEnumerable xmlReaders, IEnumerable filePaths, bool throwOnError, out DbProviderManifest providerManifest, out DbProviderFactory providerFactory, out Memoizer cachedCTypeFunction) { EntityUtil.CheckArgumentNull(xmlReaders, "xmlReaders"); // 'filePaths' can be null cachedCTypeFunction = new Memoizer (ConvertFunctionParameterToCType, null); Loader loader = new Loader(xmlReaders, filePaths, throwOnError); providerFactory = loader.ProviderFactory; providerManifest = loader.ProviderManifest; // load the items into the colleciton if (!loader.HasNonWarningErrors) { LoadProviderManifest(loader.ProviderManifest, true /* check for system namespace */); List errorList = EdmItemCollection.LoadItems(_providerManifest, loader.Schemas, this); foreach (var error in errorList) { loader.Errors.Add(error); } if (throwOnError && errorList.Count != 0) loader.ThrowOnNonWarningErrors(); } return loader.Errors; } #endregion #region Properties /// /// Returns the query cache manager /// internal System.Data.Common.QueryCache.QueryCacheManager QueryCacheManager { get { return _queryCacheManager; } } internal DbProviderFactory StoreProviderFactory { get { return _providerFactory; } } internal DbProviderManifest StoreProviderManifest { get { return _providerManifest; } } #endregion #region Methods ////// Get the list of primitive types for the given space /// ///public System.Collections.ObjectModel.ReadOnlyCollection GetPrimitiveTypes() { return _primitiveTypeMaps.GetTypes(); } /// /// Given the canonical primitive type, get the mapping primitive type in the given dataspace /// /// canonical primitive type ///The mapped scalar type internal override PrimitiveType GetMappedPrimitiveType(PrimitiveTypeKind primitiveTypeKind) { PrimitiveType type = null; _primitiveTypeMaps.TryGetType(primitiveTypeKind, null, out type); return type; } ////// checks if the schemaKey refers to the provider manifest schema key /// and if true, loads the provider manifest /// /// The connection where the store manifest is loaded from /// Check for System namespace ///The provider manifest object that was loaded private void LoadProviderManifest(DbProviderManifest storeManifest, bool checkForSystemNamespace) { foreach (PrimitiveType primitiveType in storeManifest.GetStoreTypes()) { //Add it to the collection and the primitive type maps this.AddInternal(primitiveType); _primitiveTypeMaps.Add(primitiveType); } foreach (EdmFunction function in storeManifest.GetStoreFunctions()) { AddInternal(function); } } #endregion ////// Get all the overloads of the function with the given name, this method is used for internal perspective /// /// The full name of the function /// true for case-insensitive lookup ///A collection of all the functions with the given name in the given data space ///Thrown if functionaName argument passed in is null internal System.Collections.ObjectModel.ReadOnlyCollectionGetCTypeFunctions(string functionName, bool ignoreCase) { System.Collections.ObjectModel.ReadOnlyCollection functionOverloads; if (this.FunctionLookUpTable.TryGetValue(functionName, out functionOverloads)) { functionOverloads = ConvertToCTypeFunctions(functionOverloads); if (ignoreCase) { return functionOverloads; } return GetCaseSensitiveFunctions(functionOverloads, functionName); } return Helper.EmptyEdmFunctionReadOnlyCollection; } private System.Collections.ObjectModel.ReadOnlyCollection ConvertToCTypeFunctions( System.Collections.ObjectModel.ReadOnlyCollection functionOverloads) { List cTypeFunctions = new List (); foreach (var sTypeFunction in functionOverloads) { cTypeFunctions.Add(this._cachedCTypeFunction.Evaluate(sTypeFunction)); } return cTypeFunctions.AsReadOnly(); } /// /// Convert the S type function parameters and returnType to C type /// /// ///private EdmFunction ConvertFunctionParameterToCType(EdmFunction sTypeFunction) { if (sTypeFunction.IsFromProviderManifest) { return sTypeFunction; } FunctionParameter returnParameter = null; if (sTypeFunction.ReturnParameter != null) { TypeUsage edmTypeUsageReturnParameter = MetadataHelper.ConvertStoreTypeUsageToEdmTypeUsage(sTypeFunction.ReturnParameter.TypeUsage); returnParameter = new FunctionParameter( sTypeFunction.ReturnParameter.Name, edmTypeUsageReturnParameter, sTypeFunction.ReturnParameter.GetParameterMode()); } List parameters = new List (); if (sTypeFunction.Parameters.Count > 0) { foreach (var parameter in sTypeFunction.Parameters) { TypeUsage edmTypeUsage = MetadataHelper.ConvertStoreTypeUsageToEdmTypeUsage(parameter.TypeUsage); FunctionParameter edmTypeParameter = new FunctionParameter(parameter.Name, edmTypeUsage, parameter.GetParameterMode()); parameters.Add(edmTypeParameter); } } EdmFunction edmFunction = new EdmFunction(sTypeFunction.Name, sTypeFunction.NamespaceName, DataSpace.CSpace, new EdmFunctionPayload { Schema = sTypeFunction.Schema, StoreFunctionName = sTypeFunction.StoreFunctionNameAttribute, CommandText = sTypeFunction.CommandTextAttribute, IsAggregate = sTypeFunction.AggregateAttribute, IsBuiltIn = sTypeFunction.BuiltInAttribute, IsNiladic = sTypeFunction.NiladicFunctionAttribute, IsComposable = sTypeFunction.IsComposableAttribute, IsFromProviderManifest = sTypeFunction.IsFromProviderManifest, IsCachedStoreFunction = true, ReturnParameter = returnParameter, Parameters = parameters.ToArray(), ParameterTypeSemantics = sTypeFunction.ParameterTypeSemanticsAttribute, }); edmFunction.SetReadOnly(); return edmFunction; } }//---- ItemCollection }//---- // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Common; using System.Data.Common.Utils; using System.Diagnostics; using System.Xml; using System.Data.Entity; using System.Linq; namespace System.Data.Metadata.Edm { ////// Class for representing a collection of items in Store space. /// public sealed partial class StoreItemCollection : ItemCollection { #region Fields // Cache for primitive type maps for Edm to provider private readonly CacheForPrimitiveTypes _primitiveTypeMaps = new CacheForPrimitiveTypes(); private readonly Memoizer_cachedCTypeFunction; private readonly DbProviderManifest _providerManifest; private readonly DbProviderFactory _providerFactory; // Storing the query cache manager in the store item collection since all queries are currently bound to the // store. So storing it in StoreItemCollection makes sense. Also, since query cache requires version and other // stuff of the provider, we can assume that the connection is always open and we have the store metadata. // Also we can use the same cache manager both for Entity Client and Object Query, since query cache has // no reference to any metadata in OSpace. Also we assume that ObjectMaterializer loads the assembly // before it tries to do object materialization, since we might not have loaded an assembly in another workspace // where this store item collection is getting reused private readonly System.Data.Common.QueryCache.QueryCacheManager _queryCacheManager = System.Data.Common.QueryCache.QueryCacheManager.Create(); #endregion #region Constructors // used by EntityStoreSchemaGenerator to start with an empty (primitive types only) StoreItemCollection and // add types discovered from the database internal StoreItemCollection(DbProviderFactory factory, DbProviderManifest manifest) : base(DataSpace.SSpace) { Debug.Assert(factory != null, "factory is null"); Debug.Assert(manifest != null, "manifest is null"); _providerFactory = factory; _providerManifest = manifest; _cachedCTypeFunction = new Memoizer (ConvertFunctionParameterToCType, null); LoadProviderManifest(_providerManifest, true /*checkForSystemNamespace*/); } /// /// constructor that loads the metadata files from the specified xmlReaders, and returns the list of errors /// encountered during load as the out parameter errors. /// /// Publicly available from System.Data.Entity.Desgin.dll /// /// xmlReaders where the CDM schemas are loaded /// the paths where the files can be found that match the xml readers collection /// An out parameter to return the collection of errors encountered while loading [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] // referenced by System.Data.Entity.Design.dll internal StoreItemCollection(IEnumerablexmlReaders, System.Collections.ObjectModel.ReadOnlyCollection filePaths, out IList errors) : base(DataSpace.SSpace) { EntityUtil.CheckArgumentNull(xmlReaders, "xmlReaders"); EntityUtil.CheckArgumentEmpty(ref xmlReaders, Strings.StoreItemCollectionMustHaveOneArtifact, "xmlReader"); errors = this.Init(xmlReaders, filePaths, false, out _providerManifest, out _providerFactory, out _cachedCTypeFunction); } /// /// constructor that loads the metadata files from the specified xmlReaders, and returns the list of errors /// encountered during load as the out parameter errors. /// /// Publicly available from System.Data.Entity.Desgin.dll /// /// xmlReaders where the CDM schemas are loaded /// the paths where the files can be found that match the xml readers collection internal StoreItemCollection(IEnumerablexmlReaders, IEnumerable filePaths) : base(DataSpace.SSpace) { EntityUtil.CheckArgumentNull(filePaths, "filePaths"); EntityUtil.CheckArgumentEmpty(ref xmlReaders, Strings.StoreItemCollectionMustHaveOneArtifact, "xmlReader"); this.Init(xmlReaders, filePaths, true, out _providerManifest, out _providerFactory, out _cachedCTypeFunction); } /// /// Public constructor that loads the metadata files from the specified xmlReaders. /// Throws when encounter errors. /// /// xmlReaders where the CDM schemas are loaded public StoreItemCollection(IEnumerablexmlReaders) : base(DataSpace.SSpace) { EntityUtil.CheckArgumentNull(xmlReaders, "xmlReaders"); EntityUtil.CheckArgumentEmpty(ref xmlReaders, Strings.StoreItemCollectionMustHaveOneArtifact, "xmlReader"); MetadataArtifactLoader composite = MetadataArtifactLoader.CreateCompositeFromXmlReaders(xmlReaders); this.Init(composite.GetReaders(), composite.GetPaths(), true, out _providerManifest, out _providerFactory, out _cachedCTypeFunction); } /// /// Constructs the new instance of StoreItemCollection /// with the list of CDM files provided. /// /// paths where the CDM schemas are loaded ///Thrown if path name is not valid ///thrown if paths argument is null ///For errors related to invalid schemas. public StoreItemCollection(params string[] filePaths) : base(DataSpace.SSpace) { EntityUtil.CheckArgumentNull(filePaths, "filePaths"); IEnumerableenumerableFilePaths = filePaths; EntityUtil.CheckArgumentEmpty(ref enumerableFilePaths, Strings.StoreItemCollectionMustHaveOneArtifact, "filePaths"); // Wrap the file paths in instances of the MetadataArtifactLoader class, which provides // an abstraction and a uniform interface over a diverse set of metadata artifacts. // MetadataArtifactLoader composite = null; List readers = null; try { composite = MetadataArtifactLoader.CreateCompositeFromFilePaths(enumerableFilePaths, XmlConstants.SSpaceSchemaExtension); readers = composite.CreateReaders(DataSpace.SSpace); IEnumerable ieReaders = readers.AsEnumerable(); EntityUtil.CheckArgumentEmpty(ref ieReaders, Strings.StoreItemCollectionMustHaveOneArtifact, "filePaths"); this.Init(readers, composite.GetPaths(DataSpace.SSpace), true, out _providerManifest, out _providerFactory, out _cachedCTypeFunction); } finally { if (readers != null) { Helper.DisposeXmlReaders(readers); } } } private IList Init(IEnumerable xmlReaders, IEnumerable filePaths, bool throwOnError, out DbProviderManifest providerManifest, out DbProviderFactory providerFactory, out Memoizer cachedCTypeFunction) { EntityUtil.CheckArgumentNull(xmlReaders, "xmlReaders"); // 'filePaths' can be null cachedCTypeFunction = new Memoizer (ConvertFunctionParameterToCType, null); Loader loader = new Loader(xmlReaders, filePaths, throwOnError); providerFactory = loader.ProviderFactory; providerManifest = loader.ProviderManifest; // load the items into the colleciton if (!loader.HasNonWarningErrors) { LoadProviderManifest(loader.ProviderManifest, true /* check for system namespace */); List errorList = EdmItemCollection.LoadItems(_providerManifest, loader.Schemas, this); foreach (var error in errorList) { loader.Errors.Add(error); } if (throwOnError && errorList.Count != 0) loader.ThrowOnNonWarningErrors(); } return loader.Errors; } #endregion #region Properties /// /// Returns the query cache manager /// internal System.Data.Common.QueryCache.QueryCacheManager QueryCacheManager { get { return _queryCacheManager; } } internal DbProviderFactory StoreProviderFactory { get { return _providerFactory; } } internal DbProviderManifest StoreProviderManifest { get { return _providerManifest; } } #endregion #region Methods ////// Get the list of primitive types for the given space /// ///public System.Collections.ObjectModel.ReadOnlyCollection GetPrimitiveTypes() { return _primitiveTypeMaps.GetTypes(); } /// /// Given the canonical primitive type, get the mapping primitive type in the given dataspace /// /// canonical primitive type ///The mapped scalar type internal override PrimitiveType GetMappedPrimitiveType(PrimitiveTypeKind primitiveTypeKind) { PrimitiveType type = null; _primitiveTypeMaps.TryGetType(primitiveTypeKind, null, out type); return type; } ////// checks if the schemaKey refers to the provider manifest schema key /// and if true, loads the provider manifest /// /// The connection where the store manifest is loaded from /// Check for System namespace ///The provider manifest object that was loaded private void LoadProviderManifest(DbProviderManifest storeManifest, bool checkForSystemNamespace) { foreach (PrimitiveType primitiveType in storeManifest.GetStoreTypes()) { //Add it to the collection and the primitive type maps this.AddInternal(primitiveType); _primitiveTypeMaps.Add(primitiveType); } foreach (EdmFunction function in storeManifest.GetStoreFunctions()) { AddInternal(function); } } #endregion ////// Get all the overloads of the function with the given name, this method is used for internal perspective /// /// The full name of the function /// true for case-insensitive lookup ///A collection of all the functions with the given name in the given data space ///Thrown if functionaName argument passed in is null internal System.Collections.ObjectModel.ReadOnlyCollectionGetCTypeFunctions(string functionName, bool ignoreCase) { System.Collections.ObjectModel.ReadOnlyCollection functionOverloads; if (this.FunctionLookUpTable.TryGetValue(functionName, out functionOverloads)) { functionOverloads = ConvertToCTypeFunctions(functionOverloads); if (ignoreCase) { return functionOverloads; } return GetCaseSensitiveFunctions(functionOverloads, functionName); } return Helper.EmptyEdmFunctionReadOnlyCollection; } private System.Collections.ObjectModel.ReadOnlyCollection ConvertToCTypeFunctions( System.Collections.ObjectModel.ReadOnlyCollection functionOverloads) { List cTypeFunctions = new List (); foreach (var sTypeFunction in functionOverloads) { cTypeFunctions.Add(this._cachedCTypeFunction.Evaluate(sTypeFunction)); } return cTypeFunctions.AsReadOnly(); } /// /// Convert the S type function parameters and returnType to C type /// /// ///private EdmFunction ConvertFunctionParameterToCType(EdmFunction sTypeFunction) { if (sTypeFunction.IsFromProviderManifest) { return sTypeFunction; } FunctionParameter returnParameter = null; if (sTypeFunction.ReturnParameter != null) { TypeUsage edmTypeUsageReturnParameter = MetadataHelper.ConvertStoreTypeUsageToEdmTypeUsage(sTypeFunction.ReturnParameter.TypeUsage); returnParameter = new FunctionParameter( sTypeFunction.ReturnParameter.Name, edmTypeUsageReturnParameter, sTypeFunction.ReturnParameter.GetParameterMode()); } List parameters = new List (); if (sTypeFunction.Parameters.Count > 0) { foreach (var parameter in sTypeFunction.Parameters) { TypeUsage edmTypeUsage = MetadataHelper.ConvertStoreTypeUsageToEdmTypeUsage(parameter.TypeUsage); FunctionParameter edmTypeParameter = new FunctionParameter(parameter.Name, edmTypeUsage, parameter.GetParameterMode()); parameters.Add(edmTypeParameter); } } EdmFunction edmFunction = new EdmFunction(sTypeFunction.Name, sTypeFunction.NamespaceName, DataSpace.CSpace, new EdmFunctionPayload { Schema = sTypeFunction.Schema, StoreFunctionName = sTypeFunction.StoreFunctionNameAttribute, CommandText = sTypeFunction.CommandTextAttribute, IsAggregate = sTypeFunction.AggregateAttribute, IsBuiltIn = sTypeFunction.BuiltInAttribute, IsNiladic = sTypeFunction.NiladicFunctionAttribute, IsComposable = sTypeFunction.IsComposableAttribute, IsFromProviderManifest = sTypeFunction.IsFromProviderManifest, IsCachedStoreFunction = true, ReturnParameter = returnParameter, Parameters = parameters.ToArray(), ParameterTypeSemantics = sTypeFunction.ParameterTypeSemanticsAttribute, }); edmFunction.SetReadOnly(); return edmFunction; } }//---- ItemCollection }//---- // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
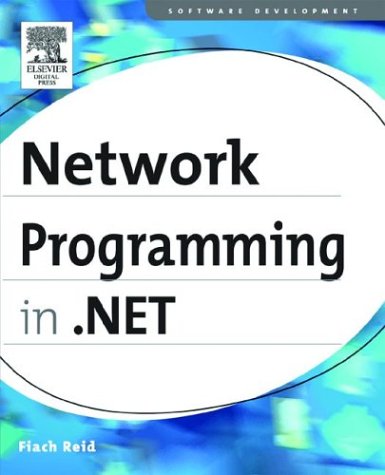
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolboxItemCollection.cs
- IisTraceWebEventProvider.cs
- CodeMethodReturnStatement.cs
- ServicesExceptionNotHandledEventArgs.cs
- UDPClient.cs
- ADConnectionHelper.cs
- LayoutUtils.cs
- PenThread.cs
- TraceData.cs
- MarshalDirectiveException.cs
- SplashScreenNativeMethods.cs
- TrustSection.cs
- ByteStreamGeometryContext.cs
- QilDataSource.cs
- MarginCollapsingState.cs
- Vector3D.cs
- ReferentialConstraintRoleElement.cs
- FunctionGenerator.cs
- TrustLevelCollection.cs
- HttpInputStream.cs
- ScopelessEnumAttribute.cs
- Transaction.cs
- ApplicationBuildProvider.cs
- ExecutedRoutedEventArgs.cs
- ToolStripHighContrastRenderer.cs
- SiteMapPath.cs
- GroupBoxRenderer.cs
- TextBounds.cs
- CalendarAutomationPeer.cs
- ProcessHostConfigUtils.cs
- KeyManager.cs
- Icon.cs
- ModelPerspective.cs
- Positioning.cs
- ImageList.cs
- EncoderFallback.cs
- PeerNameRecordCollection.cs
- XmlSignatureManifest.cs
- EdmItemError.cs
- X509DefaultServiceCertificateElement.cs
- ConfigurationElementCollection.cs
- ToolStripDropDown.cs
- DataGridColumnStyleMappingNameEditor.cs
- ToolBarTray.cs
- XmlSchemaSimpleTypeUnion.cs
- TextCollapsingProperties.cs
- UserControlAutomationPeer.cs
- SqlNode.cs
- BrowserDefinition.cs
- CodeAssignStatement.cs
- HttpResponseHeader.cs
- ConnectorMovedEventArgs.cs
- EntityDataSourceUtil.cs
- FloatAverageAggregationOperator.cs
- ComplexTypeEmitter.cs
- CodeGenerator.cs
- MaterialGroup.cs
- IndependentAnimationStorage.cs
- TextEffectResolver.cs
- DataGridViewSortCompareEventArgs.cs
- SimpleTableProvider.cs
- Directory.cs
- PathGeometry.cs
- TypedTableBaseExtensions.cs
- PauseStoryboard.cs
- EntitySetBase.cs
- LockedActivityGlyph.cs
- ResourceReferenceExpressionConverter.cs
- FixedDocument.cs
- SerializationFieldInfo.cs
- _Win32.cs
- MailWriter.cs
- QilStrConcat.cs
- _ContextAwareResult.cs
- MarginCollapsingState.cs
- MergablePropertyAttribute.cs
- CheckoutException.cs
- SqlFacetAttribute.cs
- XXXOnTypeBuilderInstantiation.cs
- TypefaceMetricsCache.cs
- ApplicationContext.cs
- HttpListener.cs
- TerminateDesigner.cs
- MessageQueuePermission.cs
- ToolZone.cs
- ObjectListItemCollection.cs
- CodeVariableDeclarationStatement.cs
- HuffmanTree.cs
- SqlAggregateChecker.cs
- Parsers.cs
- SourceElementsCollection.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- FileSystemInfo.cs
- FileDialog_Vista_Interop.cs
- MetadataException.cs
- AbstractDataSvcMapFileLoader.cs
- InputEventArgs.cs
- NavigatorInput.cs
- DetailsViewDeleteEventArgs.cs
- ListViewGroup.cs