Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Input / InputEventArgs.cs / 1 / InputEventArgs.cs
using System.Collections; using System; using System.Security; using MS.Internal.PresentationCore; // for FriendAccessAllowed namespace System.Windows.Input { ////// The InputEventArgs class represents a type of RoutedEventArgs that /// are relevant to all input events. /// [FriendAccessAllowed ] // expose UserInitiated public class InputEventArgs : RoutedEventArgs { ////// Initializes a new instance of the InputEventArgs class. /// /// /// The input device to associate with this event. /// /// /// The time when the input occured. /// public InputEventArgs(InputDevice inputDevice, int timestamp) { /* inputDevice parameter being null is valid*/ /* timestamp parameter is valuetype, need not be checked */ _inputDevice = inputDevice; _timestamp = timestamp; } ////// Read-only access to the input device that initiated this /// event. /// public InputDevice Device { get {return _inputDevice;} internal set {_inputDevice = value;} } ////// Read-only access to the input timestamp. /// public int Timestamp { get {return _timestamp;} } ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { InputEventHandler handler = (InputEventHandler) genericHandler; handler(genericTarget, this); } private InputDevice _inputDevice; private static int _timestamp; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Collections; using System; using System.Security; using MS.Internal.PresentationCore; // for FriendAccessAllowed namespace System.Windows.Input { ////// The InputEventArgs class represents a type of RoutedEventArgs that /// are relevant to all input events. /// [FriendAccessAllowed ] // expose UserInitiated public class InputEventArgs : RoutedEventArgs { ////// Initializes a new instance of the InputEventArgs class. /// /// /// The input device to associate with this event. /// /// /// The time when the input occured. /// public InputEventArgs(InputDevice inputDevice, int timestamp) { /* inputDevice parameter being null is valid*/ /* timestamp parameter is valuetype, need not be checked */ _inputDevice = inputDevice; _timestamp = timestamp; } ////// Read-only access to the input device that initiated this /// event. /// public InputDevice Device { get {return _inputDevice;} internal set {_inputDevice = value;} } ////// Read-only access to the input timestamp. /// public int Timestamp { get {return _timestamp;} } ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { InputEventHandler handler = (InputEventHandler) genericHandler; handler(genericTarget, this); } private InputDevice _inputDevice; private static int _timestamp; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
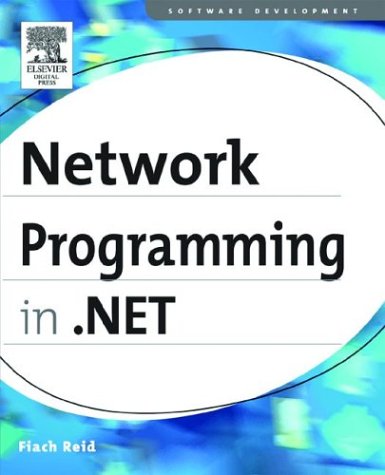
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeSnippetExpression.cs
- Panel.cs
- DataService.cs
- ToolStripRendererSwitcher.cs
- HtmlToClrEventProxy.cs
- WpfKnownTypeInvoker.cs
- CompilerParameters.cs
- CqlQuery.cs
- ExpressionPrefixAttribute.cs
- ByeOperation11AsyncResult.cs
- PageCodeDomTreeGenerator.cs
- EventProviderWriter.cs
- BitmapDecoder.cs
- InvalidCastException.cs
- ProfileEventArgs.cs
- MenuRenderer.cs
- EdmType.cs
- FileSystemWatcher.cs
- Options.cs
- CodeAttributeArgumentCollection.cs
- Section.cs
- EntityContainerEntitySetDefiningQuery.cs
- ToolboxCategory.cs
- XmlSerializerFactory.cs
- RayMeshGeometry3DHitTestResult.cs
- XmlDocumentViewSchema.cs
- CompilerState.cs
- InlineCollection.cs
- Rectangle.cs
- TaskForm.cs
- X509CertificateChain.cs
- AdjustableArrowCap.cs
- EventHandlerList.cs
- CodeDelegateInvokeExpression.cs
- DesignerAttribute.cs
- ScrollEvent.cs
- SoapReflectionImporter.cs
- SecurityTokenProvider.cs
- GroupDescription.cs
- DbProviderFactory.cs
- X509Certificate2.cs
- CharacterMetrics.cs
- WebResourceAttribute.cs
- ObjectDataSourceEventArgs.cs
- DataGridViewColumnConverter.cs
- CharEnumerator.cs
- DataServiceException.cs
- AsyncOperation.cs
- ReferenceSchema.cs
- Imaging.cs
- DataColumnMapping.cs
- BoolExpr.cs
- MenuItemBindingCollection.cs
- Int64Storage.cs
- BitmapEffectDrawingContent.cs
- XmlSerializationReader.cs
- SqlTypesSchemaImporter.cs
- AppDomainFactory.cs
- DataError.cs
- PnrpPeerResolver.cs
- SynchronizationContext.cs
- DelayedRegex.cs
- DefaultPropertyAttribute.cs
- DoubleCollectionConverter.cs
- TagNameToTypeMapper.cs
- InternalSafeNativeMethods.cs
- Token.cs
- ImageListUtils.cs
- Debugger.cs
- ISAPIApplicationHost.cs
- BmpBitmapEncoder.cs
- Debugger.cs
- SystemDiagnosticsSection.cs
- DataPagerField.cs
- MatchingStyle.cs
- DecimalStorage.cs
- WebPart.cs
- EnumType.cs
- XmlDictionaryReader.cs
- DataServiceQueryProvider.cs
- DataDocumentXPathNavigator.cs
- AsyncOperationManager.cs
- Emitter.cs
- DbDataReader.cs
- ExceptionUtil.cs
- Dispatcher.cs
- EntityClientCacheEntry.cs
- XmlNodeChangedEventManager.cs
- InfoCardCryptoHelper.cs
- DataGridViewRowPrePaintEventArgs.cs
- ChangePasswordDesigner.cs
- SqlServices.cs
- SchemaTableColumn.cs
- RolePrincipal.cs
- NamespaceList.cs
- DataGridTableCollection.cs
- BinaryNode.cs
- SchemaManager.cs
- Int32Rect.cs
- BuildProviderUtils.cs