Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / XmlDocumentViewSchema.cs / 1 / XmlDocumentViewSchema.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System.Collections; using System.Collections.Specialized; using System.Diagnostics; using System.Xml; using System.Xml.XPath; ////// A class to expose hierarchical schema from an XmlDocument object. /// This is used by data source designers to enable data-bound to /// traverse their schema at design time. /// internal sealed class XmlDocumentViewSchema : IDataSourceViewSchema { private string _name; private OrderedDictionary _children; private ArrayList _attrs; private IDataSourceViewSchema[] _viewSchemas; private IDataSourceFieldSchema[] _fieldSchemas; private bool _includeSpecialSchema; public XmlDocumentViewSchema(string name, Pair data, bool includeSpecialSchema) { _includeSpecialSchema = includeSpecialSchema; Debug.Assert(name != null && name.Length > 0); Debug.Assert(data != null); _children = (OrderedDictionary)data.First; Debug.Assert(_children != null); _attrs = (ArrayList)data.Second; Debug.Assert(_attrs != null); _name = name; } public string Name { get { return _name; } } public IDataSourceViewSchema[] GetChildren() { if (_viewSchemas == null) { _viewSchemas = new IDataSourceViewSchema[_children.Count]; int i = 0; foreach (DictionaryEntry de in _children) { _viewSchemas[i] = new XmlDocumentViewSchema((string)de.Key, (Pair)de.Value, _includeSpecialSchema); i++; } } return _viewSchemas; } public IDataSourceFieldSchema[] GetFields() { if (_fieldSchemas == null) { // The three extra slots are for the "special" field names int specialSchemaCount = (_includeSpecialSchema ? 3 : 0); _fieldSchemas = new IDataSourceFieldSchema[_attrs.Count + specialSchemaCount]; if (_includeSpecialSchema) { _fieldSchemas[0] = new XmlDocumentFieldSchema("#Name"); _fieldSchemas[1] = new XmlDocumentFieldSchema("#Value"); _fieldSchemas[2] = new XmlDocumentFieldSchema("#InnerText"); } for (int i = 0; i < _attrs.Count; i++) { _fieldSchemas[i + specialSchemaCount] = new XmlDocumentFieldSchema((string)_attrs[i]); } } return _fieldSchemas; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
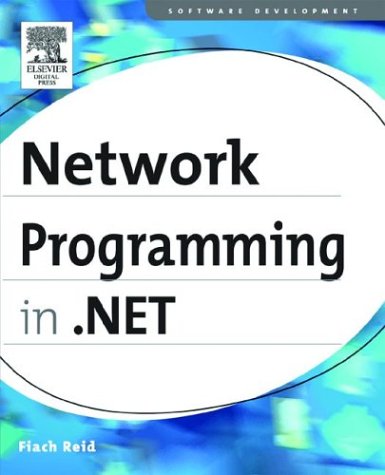
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringUtil.cs
- StylusTip.cs
- Variable.cs
- ExpressionList.cs
- DisplayInformation.cs
- SqlAggregateChecker.cs
- ProviderConnectionPoint.cs
- RegistryKey.cs
- ClockController.cs
- RunInstallerAttribute.cs
- QfeChecker.cs
- DbConnectionOptions.cs
- ZipFileInfoCollection.cs
- MouseBinding.cs
- Section.cs
- ArcSegment.cs
- CodeTryCatchFinallyStatement.cs
- ErrorFormatter.cs
- PackageDigitalSignature.cs
- RectValueSerializer.cs
- PartitionerQueryOperator.cs
- DbProviderFactoriesConfigurationHandler.cs
- _NegoStream.cs
- XmlDownloadManager.cs
- DataGridColumn.cs
- XsltLoader.cs
- ClipboardData.cs
- Brush.cs
- AutoFocusStyle.xaml.cs
- WebBrowser.cs
- RelatedCurrencyManager.cs
- SchemaTableColumn.cs
- DrawListViewSubItemEventArgs.cs
- DropShadowEffect.cs
- UIElementCollection.cs
- Variable.cs
- QuinticEase.cs
- AttachedPropertyMethodSelector.cs
- SkewTransform.cs
- NamedPipeConnectionPoolSettings.cs
- Window.cs
- StatusBarItemAutomationPeer.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- BookmarkInfo.cs
- DependencyPropertyValueSerializer.cs
- CorruptingExceptionCommon.cs
- ParseChildrenAsPropertiesAttribute.cs
- ModelItemDictionaryImpl.cs
- TableLayoutColumnStyleCollection.cs
- HwndHostAutomationPeer.cs
- InkCanvasSelection.cs
- SafeLibraryHandle.cs
- ExpressionList.cs
- DataViewManager.cs
- CodePageEncoding.cs
- Pair.cs
- EnumUnknown.cs
- AddInActivator.cs
- CngKeyBlobFormat.cs
- AttributeCollection.cs
- RequestQueryParser.cs
- DataFormat.cs
- TreeViewHitTestInfo.cs
- Renderer.cs
- PeerCollaborationPermission.cs
- versioninfo.cs
- TransactionFilter.cs
- TrackingRecord.cs
- AbsoluteQuery.cs
- ImageSource.cs
- FrameworkContentElement.cs
- BrowserCapabilitiesCompiler.cs
- DbConnectionPoolGroupProviderInfo.cs
- EntitySetBaseCollection.cs
- ContainerUIElement3D.cs
- ButtonRenderer.cs
- ArrayList.cs
- BitmapEffectGeneralTransform.cs
- securestring.cs
- TreeNodeClickEventArgs.cs
- DispatcherSynchronizationContext.cs
- ConfigurationException.cs
- StyleBamlRecordReader.cs
- CompressionTracing.cs
- SessionEndedEventArgs.cs
- OleDbConnectionFactory.cs
- XPathExpr.cs
- MaterializeFromAtom.cs
- NetworkStream.cs
- Camera.cs
- BindingsCollection.cs
- ChtmlCalendarAdapter.cs
- NavigationProgressEventArgs.cs
- MaterialCollection.cs
- Model3D.cs
- ContractTypeNameCollection.cs
- Trace.cs
- MetadataCacheItem.cs
- EventItfInfo.cs
- Visual.cs