Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / BezierSegment.cs / 1305600 / BezierSegment.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: BezierSegment.cs //----------------------------------------------------------------------------- using System; using MS.Internal; using MS.Internal.PresentationCore; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows; using System.Text.RegularExpressions; using System.Windows.Media.Composition; using System.Windows.Media.Animation; using System.Diagnostics; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// BezierSegment /// public sealed partial class BezierSegment : PathSegment { #region Constructors ////// /// public BezierSegment() : base() { } ////// /// public BezierSegment(Point point1, Point point2, Point point3, bool isStroked) { Point1 = point1; Point2 = point2; Point3 = point3; IsStroked = isStroked; } // Internal constructor supporting smooth joins between segments internal BezierSegment(Point point1, Point point2, Point point3, bool isStroked, bool isSmoothJoin) { Point1 = point1; Point2 = point2; Point3 = point3; IsStroked = isStroked; IsSmoothJoin = isSmoothJoin; } #endregion #region AddToFigure internal override void AddToFigure( Matrix matrix, // The transformation matrid PathFigure figure, // The figure to add to ref Point current) // Out: Segment endpoint, not transformed { current = Point3; if (matrix.IsIdentity) { figure.Segments.Add(this); } else { Point pt1 = Point1; pt1 *= matrix; Point pt2 = Point2; pt2 *= matrix; Point pt3 = current; pt3 *= matrix; figure.Segments.Add(new BezierSegment(pt1, pt2, pt3, IsStroked, IsSmoothJoin)); } } #endregion #region Resource ////// SerializeData - Serialize the contents of this Segment to the provided context. /// internal override void SerializeData(StreamGeometryContext ctx) { ctx.BezierTo(Point1, Point2, Point3, IsStroked, IsSmoothJoin); } internal override bool IsCurved() { return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "C{1:" + format + "}{0}{2:" + format + "}{0}{3:" + format + "}", separator, Point1, Point2, Point3 ); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: BezierSegment.cs //----------------------------------------------------------------------------- using System; using MS.Internal; using MS.Internal.PresentationCore; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows; using System.Text.RegularExpressions; using System.Windows.Media.Composition; using System.Windows.Media.Animation; using System.Diagnostics; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// BezierSegment /// public sealed partial class BezierSegment : PathSegment { #region Constructors ////// /// public BezierSegment() : base() { } ////// /// public BezierSegment(Point point1, Point point2, Point point3, bool isStroked) { Point1 = point1; Point2 = point2; Point3 = point3; IsStroked = isStroked; } // Internal constructor supporting smooth joins between segments internal BezierSegment(Point point1, Point point2, Point point3, bool isStroked, bool isSmoothJoin) { Point1 = point1; Point2 = point2; Point3 = point3; IsStroked = isStroked; IsSmoothJoin = isSmoothJoin; } #endregion #region AddToFigure internal override void AddToFigure( Matrix matrix, // The transformation matrid PathFigure figure, // The figure to add to ref Point current) // Out: Segment endpoint, not transformed { current = Point3; if (matrix.IsIdentity) { figure.Segments.Add(this); } else { Point pt1 = Point1; pt1 *= matrix; Point pt2 = Point2; pt2 *= matrix; Point pt3 = current; pt3 *= matrix; figure.Segments.Add(new BezierSegment(pt1, pt2, pt3, IsStroked, IsSmoothJoin)); } } #endregion #region Resource ////// SerializeData - Serialize the contents of this Segment to the provided context. /// internal override void SerializeData(StreamGeometryContext ctx) { ctx.BezierTo(Point1, Point2, Point3, IsStroked, IsSmoothJoin); } internal override bool IsCurved() { return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "C{1:" + format + "}{0}{2:" + format + "}{0}{3:" + format + "}", separator, Point1, Point2, Point3 ); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
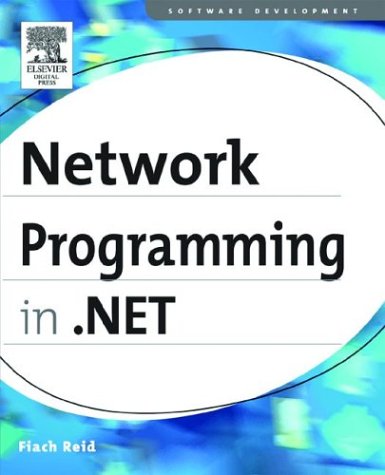
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientTarget.cs
- TrustManager.cs
- XsltFunctions.cs
- PageTheme.cs
- ObjectDataSourceStatusEventArgs.cs
- StylusPointCollection.cs
- SHA512Cng.cs
- PointCollectionValueSerializer.cs
- TreeNodeMouseHoverEvent.cs
- MultiTouchSystemGestureLogic.cs
- IntSecurity.cs
- mansign.cs
- BrowserCapabilitiesCompiler.cs
- SqlCommandBuilder.cs
- ExceptionHandlers.cs
- UnsupportedPolicyOptionsException.cs
- TimelineGroup.cs
- AlphabetConverter.cs
- WizardPanelChangingEventArgs.cs
- Floater.cs
- BitmapCodecInfoInternal.cs
- ArrangedElement.cs
- EmptyElement.cs
- SocketInformation.cs
- TransformCollection.cs
- DataListCommandEventArgs.cs
- RNGCryptoServiceProvider.cs
- CriticalFinalizerObject.cs
- XmlQueryRuntime.cs
- ServiceDescriptionImporter.cs
- JoinCqlBlock.cs
- FacetEnabledSchemaElement.cs
- PrintPreviewDialog.cs
- ItemsPresenter.cs
- RootBrowserWindowAutomationPeer.cs
- BitHelper.cs
- TaiwanLunisolarCalendar.cs
- UseAttributeSetsAction.cs
- RootProfilePropertySettingsCollection.cs
- SqlClientPermission.cs
- FilteredXmlReader.cs
- AttachedPropertiesService.cs
- FixedSOMTextRun.cs
- SymbolTable.cs
- _KerberosClient.cs
- TextUtf8RawTextWriter.cs
- ZipIOLocalFileBlock.cs
- FastPropertyAccessor.cs
- GridViewSortEventArgs.cs
- CapabilitiesPattern.cs
- TextFormatter.cs
- XmlIlTypeHelper.cs
- SoapEnumAttribute.cs
- SetterBaseCollection.cs
- UnmanagedMemoryStreamWrapper.cs
- TypefaceCollection.cs
- _RequestCacheProtocol.cs
- XmlException.cs
- EditorPart.cs
- CommandBindingCollection.cs
- MailSettingsSection.cs
- FlowThrottle.cs
- StaticTextPointer.cs
- XPathPatternBuilder.cs
- TimersDescriptionAttribute.cs
- ContextMenuAutomationPeer.cs
- InProcStateClientManager.cs
- Content.cs
- EventMap.cs
- XmlDomTextWriter.cs
- InvalidPrinterException.cs
- ItemsControl.cs
- AnimationStorage.cs
- CodeChecksumPragma.cs
- _ListenerRequestStream.cs
- DefaultExpression.cs
- AppDomainGrammarProxy.cs
- TreeNodeEventArgs.cs
- Size3D.cs
- WindowsScroll.cs
- SecurityKeyUsage.cs
- PropertyStore.cs
- Separator.cs
- TemplatedWizardStep.cs
- Logging.cs
- TableItemProviderWrapper.cs
- DbConnectionPoolOptions.cs
- HttpHostedTransportConfiguration.cs
- BaseTemplateBuildProvider.cs
- LinearGradientBrush.cs
- ResourcesGenerator.cs
- Group.cs
- AppSettingsExpressionBuilder.cs
- BamlVersionHeader.cs
- CatalogPartCollection.cs
- TimelineCollection.cs
- PingReply.cs
- LoadedEvent.cs
- MimeWriter.cs
- SemanticValue.cs