Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / FontFace / TypefaceCollection.cs / 1 / TypefaceCollection.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: TypefaceCollection.cs // // Contents: Collection of typefaces // // Created: 5-15-2003 Michael Leonov (mleonov) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Media; using MS.Internal.FontCache; using System.Globalization; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.FontFace { internal unsafe struct TypefaceCollection : ICollection{ private CachedFontFamily _family; private FontFamily _fontFamily; public TypefaceCollection(FontFamily fontFamily, CachedFontFamily family) { _fontFamily = fontFamily; _family = family; } #region ICollection Members public void Add(Typeface item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(Typeface item) { foreach (Typeface t in this) { if (t.Equals(item)) return true; } return false; } public void CopyTo(Typeface[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } foreach (Typeface t in this) { array[arrayIndex++] = t; } } public int Count { get { return _family.NumberOfFaces; } } public bool IsReadOnly { get { return true; } } public bool Remove(Typeface item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { return new Enumerator(this); } #endregion #region IEnumerable Members System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new Enumerator(this); } #endregion private struct Enumerator : IEnumerator { public Enumerator(TypefaceCollection typefaceCollection) { _typefaceCollection = typefaceCollection; // Unfortunately we cannot call Reset() here because not all of the fields are initialized. _familyEnumerator = ((IEnumerable )typefaceCollection._family).GetEnumerator(); } #region IEnumerator Members public Typeface Current { get { CachedFontFace face = _familyEnumerator.Current; return new Typeface(_typefaceCollection._fontFamily, face.Style, face.Weight, face.Stretch); } } #endregion #region IDisposable Members public void Dispose() {} #endregion #region IEnumerator Members object System.Collections.IEnumerator.Current { get { return ((IEnumerator )this).Current; } } public bool MoveNext() { return _familyEnumerator.MoveNext(); } public void Reset() { _familyEnumerator = ((IEnumerable )_typefaceCollection._family).GetEnumerator(); } #endregion private IEnumerator _familyEnumerator; private TypefaceCollection _typefaceCollection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: TypefaceCollection.cs // // Contents: Collection of typefaces // // Created: 5-15-2003 Michael Leonov (mleonov) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Media; using MS.Internal.FontCache; using System.Globalization; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.FontFace { internal unsafe struct TypefaceCollection : ICollection { private CachedFontFamily _family; private FontFamily _fontFamily; public TypefaceCollection(FontFamily fontFamily, CachedFontFamily family) { _fontFamily = fontFamily; _family = family; } #region ICollection Members public void Add(Typeface item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(Typeface item) { foreach (Typeface t in this) { if (t.Equals(item)) return true; } return false; } public void CopyTo(Typeface[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } foreach (Typeface t in this) { array[arrayIndex++] = t; } } public int Count { get { return _family.NumberOfFaces; } } public bool IsReadOnly { get { return true; } } public bool Remove(Typeface item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { return new Enumerator(this); } #endregion #region IEnumerable Members System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new Enumerator(this); } #endregion private struct Enumerator : IEnumerator { public Enumerator(TypefaceCollection typefaceCollection) { _typefaceCollection = typefaceCollection; // Unfortunately we cannot call Reset() here because not all of the fields are initialized. _familyEnumerator = ((IEnumerable )typefaceCollection._family).GetEnumerator(); } #region IEnumerator Members public Typeface Current { get { CachedFontFace face = _familyEnumerator.Current; return new Typeface(_typefaceCollection._fontFamily, face.Style, face.Weight, face.Stretch); } } #endregion #region IDisposable Members public void Dispose() {} #endregion #region IEnumerator Members object System.Collections.IEnumerator.Current { get { return ((IEnumerator )this).Current; } } public bool MoveNext() { return _familyEnumerator.MoveNext(); } public void Reset() { _familyEnumerator = ((IEnumerable )_typefaceCollection._family).GetEnumerator(); } #endregion private IEnumerator _familyEnumerator; private TypefaceCollection _typefaceCollection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
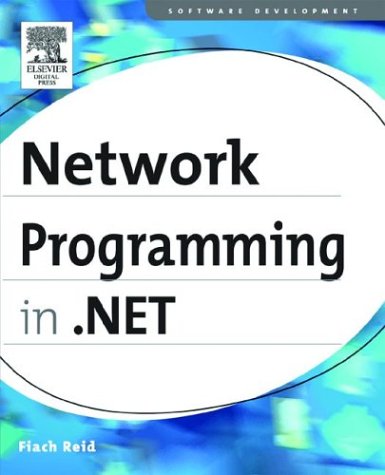
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttributeCollection.cs
- SignatureToken.cs
- ToolStripOverflowButton.cs
- propertytag.cs
- DataGridViewCellValueEventArgs.cs
- EnvelopedPkcs7.cs
- WebPermission.cs
- VisualStyleInformation.cs
- BindingObserver.cs
- CompilationUtil.cs
- HttpWebResponse.cs
- NumberSubstitution.cs
- SdlChannelSink.cs
- Triplet.cs
- TemplatePropertyEntry.cs
- ClickablePoint.cs
- FirstMatchCodeGroup.cs
- MimeTypeAttribute.cs
- WCFModelStrings.Designer.cs
- LinkDesigner.cs
- BitmapVisualManager.cs
- SchemaImporterExtensionElementCollection.cs
- RootProfilePropertySettingsCollection.cs
- SyndicationDeserializer.cs
- CodeSpit.cs
- RecognitionResult.cs
- StringDictionary.cs
- EDesignUtil.cs
- AppPool.cs
- NaturalLanguageHyphenator.cs
- HashAlgorithm.cs
- LongTypeConverter.cs
- NativeMethods.cs
- RootProfilePropertySettingsCollection.cs
- TextProviderWrapper.cs
- DirectoryInfo.cs
- AsyncInvokeOperation.cs
- MetaDataInfo.cs
- StateMachineSubscription.cs
- WebServiceTypeData.cs
- TextTabProperties.cs
- TypeInitializationException.cs
- CriticalFinalizerObject.cs
- RenderDataDrawingContext.cs
- DataMemberFieldConverter.cs
- StatusStrip.cs
- WindowsToolbar.cs
- VerificationAttribute.cs
- EntityDescriptor.cs
- LoginAutoFormat.cs
- HtmlTableRowCollection.cs
- ScriptReferenceBase.cs
- UnknownBitmapDecoder.cs
- OracleDateTime.cs
- RtfToXamlLexer.cs
- RegexRunnerFactory.cs
- DeploymentSection.cs
- DbMetaDataCollectionNames.cs
- EditorZoneBase.cs
- SafeNativeMethodsOther.cs
- FormViewModeEventArgs.cs
- WsdlBuildProvider.cs
- UInt64.cs
- XslAst.cs
- ProgressBarHighlightConverter.cs
- ColorBuilder.cs
- ConnectionStringsExpressionBuilder.cs
- ListViewInsertEventArgs.cs
- XmlSignatureProperties.cs
- FileCodeGroup.cs
- EditCommandColumn.cs
- WindowsToolbarItemAsMenuItem.cs
- sqlinternaltransaction.cs
- Schema.cs
- SystemResourceHost.cs
- DesignerValidatorAdapter.cs
- ModuleConfigurationInfo.cs
- KeyValueConfigurationCollection.cs
- ClrProviderManifest.cs
- LoginView.cs
- SessionStateContainer.cs
- SelectionManager.cs
- ImageConverter.cs
- TaskExceptionHolder.cs
- SafeNativeMethodsCLR.cs
- Win32KeyboardDevice.cs
- TrimSurroundingWhitespaceAttribute.cs
- CheckedListBox.cs
- MultipleCopiesCollection.cs
- PropertyMappingExceptionEventArgs.cs
- CapabilitiesSection.cs
- ConfigurationException.cs
- KoreanCalendar.cs
- ConfigXmlAttribute.cs
- ObsoleteAttribute.cs
- Latin1Encoding.cs
- SerializerWriterEventHandlers.cs
- Tile.cs
- LoginCancelEventArgs.cs
- FixUpCollection.cs