Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / WebControls / EditCommandColumn.cs / 1 / EditCommandColumn.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class EditCommandColumn : DataGridColumn { ///Creates a special column with buttons for ///, /// , and commands to edit items /// within the selected row. /// public EditCommandColumn() { } ///Initializes a new instance of an ///class. /// [ DefaultValue(ButtonColumnType.LinkButton) ] public virtual ButtonColumnType ButtonType { get { object o = ViewState["ButtonType"]; if (o != null) return(ButtonColumnType)o; return ButtonColumnType.LinkButton; } set { if (value < ButtonColumnType.LinkButton || value > ButtonColumnType.PushButton) { throw new ArgumentOutOfRangeException("value"); } ViewState["ButtonType"] = value; OnColumnChanged(); } } ///Indicates the button type for the column. ////// [ Localizable(true), DefaultValue("") ] public virtual string CancelText { get { object o = ViewState["CancelText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["CancelText"] = value; OnColumnChanged(); } } [ DefaultValue(true), ] public virtual bool CausesValidation { get { object o = ViewState["CausesValidation"]; if (o != null) { return (bool)o; } return true; } set { ViewState["CausesValidation"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button /// in the column. /// [ Localizable(true), DefaultValue("") ] public virtual string EditText { get { object o = ViewState["EditText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["EditText"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button in /// the column. /// [ Localizable(true), DefaultValue("") ] public virtual string UpdateText { get { object o = ViewState["UpdateText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["UpdateText"] = value; OnColumnChanged(); } } [ DefaultValue(""), ] public virtual string ValidationGroup { get { object o = ViewState["ValidationGroup"]; if (o != null) { return (string)o; } return String.Empty; } set { ViewState["ValidationGroup"] = value; OnColumnChanged(); } } private void AddButtonToCell(TableCell cell, string commandName, string buttonText, bool causesValidation, string validationGroup) { WebControl buttonControl = null; ControlCollection controls = cell.Controls; ButtonColumnType buttonType = ButtonType; if (buttonType == ButtonColumnType.LinkButton) { LinkButton button = new DataGridLinkButton(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } else { Button button = new Button(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } controls.Add(buttonControl); } ///Indicates the text to display for the ///command button /// in the column. /// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); bool causesValidation = CausesValidation; if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { if (itemType == ListItemType.EditItem) { ControlCollection controls = cell.Controls; AddButtonToCell(cell, DataGrid.UpdateCommandName, UpdateText, causesValidation, ValidationGroup); LiteralControl spaceControl = new LiteralControl(" "); controls.Add(spaceControl); AddButtonToCell(cell, DataGrid.CancelCommandName, CancelText, false, String.Empty); } else { AddButtonToCell(cell, DataGrid.EditCommandName, EditText, false, String.Empty); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Initializes a cell within the column. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class EditCommandColumn : DataGridColumn { ///Creates a special column with buttons for ///, /// , and commands to edit items /// within the selected row. /// public EditCommandColumn() { } ///Initializes a new instance of an ///class. /// [ DefaultValue(ButtonColumnType.LinkButton) ] public virtual ButtonColumnType ButtonType { get { object o = ViewState["ButtonType"]; if (o != null) return(ButtonColumnType)o; return ButtonColumnType.LinkButton; } set { if (value < ButtonColumnType.LinkButton || value > ButtonColumnType.PushButton) { throw new ArgumentOutOfRangeException("value"); } ViewState["ButtonType"] = value; OnColumnChanged(); } } ///Indicates the button type for the column. ////// [ Localizable(true), DefaultValue("") ] public virtual string CancelText { get { object o = ViewState["CancelText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["CancelText"] = value; OnColumnChanged(); } } [ DefaultValue(true), ] public virtual bool CausesValidation { get { object o = ViewState["CausesValidation"]; if (o != null) { return (bool)o; } return true; } set { ViewState["CausesValidation"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button /// in the column. /// [ Localizable(true), DefaultValue("") ] public virtual string EditText { get { object o = ViewState["EditText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["EditText"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button in /// the column. /// [ Localizable(true), DefaultValue("") ] public virtual string UpdateText { get { object o = ViewState["UpdateText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["UpdateText"] = value; OnColumnChanged(); } } [ DefaultValue(""), ] public virtual string ValidationGroup { get { object o = ViewState["ValidationGroup"]; if (o != null) { return (string)o; } return String.Empty; } set { ViewState["ValidationGroup"] = value; OnColumnChanged(); } } private void AddButtonToCell(TableCell cell, string commandName, string buttonText, bool causesValidation, string validationGroup) { WebControl buttonControl = null; ControlCollection controls = cell.Controls; ButtonColumnType buttonType = ButtonType; if (buttonType == ButtonColumnType.LinkButton) { LinkButton button = new DataGridLinkButton(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } else { Button button = new Button(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } controls.Add(buttonControl); } ///Indicates the text to display for the ///command button /// in the column. /// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); bool causesValidation = CausesValidation; if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { if (itemType == ListItemType.EditItem) { ControlCollection controls = cell.Controls; AddButtonToCell(cell, DataGrid.UpdateCommandName, UpdateText, causesValidation, ValidationGroup); LiteralControl spaceControl = new LiteralControl(" "); controls.Add(spaceControl); AddButtonToCell(cell, DataGrid.CancelCommandName, CancelText, false, String.Empty); } else { AddButtonToCell(cell, DataGrid.EditCommandName, EditText, false, String.Empty); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Initializes a cell within the column. ///
Link Menu
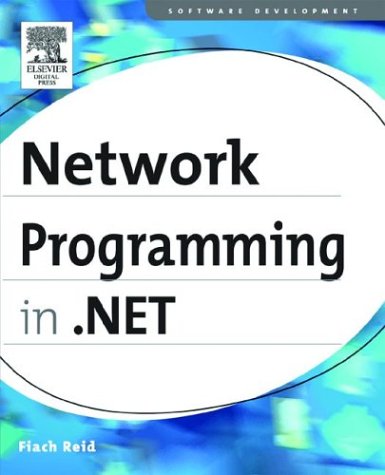
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataSourceGeneratorException.cs
- Msmq4PoisonHandler.cs
- DbDataAdapter.cs
- ObjectParameterCollection.cs
- SecurityTokenResolver.cs
- ErrorView.xaml.cs
- TextParagraphView.cs
- RepeaterCommandEventArgs.cs
- ObjectTag.cs
- CodeExpressionStatement.cs
- ISAPIApplicationHost.cs
- DocumentOrderQuery.cs
- TransformGroup.cs
- DiscoveryClientOutputChannel.cs
- PolyBezierSegment.cs
- WebUtil.cs
- Match.cs
- AuthorizationRuleCollection.cs
- UserControl.cs
- RIPEMD160.cs
- GlobalEventManager.cs
- GeneralTransform3DGroup.cs
- ServiceDocumentFormatter.cs
- TransactionScope.cs
- ScrollItemPattern.cs
- ObjectView.cs
- XmlSchemaExporter.cs
- WorkflowWebHostingModule.cs
- DiscoveryClientOutputChannel.cs
- DataObjectMethodAttribute.cs
- Encoding.cs
- BoundsDrawingContextWalker.cs
- FontFamily.cs
- CacheEntry.cs
- DynamicValueConverter.cs
- InspectionWorker.cs
- FormDocumentDesigner.cs
- WindowProviderWrapper.cs
- ManipulationDevice.cs
- WindowsAltTab.cs
- Types.cs
- MailWebEventProvider.cs
- DesignerValidatorAdapter.cs
- ActivityDesignerAccessibleObject.cs
- RSAOAEPKeyExchangeDeformatter.cs
- CellQuery.cs
- HTMLTextWriter.cs
- Directory.cs
- AttributeData.cs
- XmlSignificantWhitespace.cs
- MD5HashHelper.cs
- TextMetrics.cs
- AnnotationAdorner.cs
- StringComparer.cs
- BinaryObjectInfo.cs
- DefaultSettingsSection.cs
- ChannelParameterCollection.cs
- WindowsTab.cs
- CheckBox.cs
- TabletDevice.cs
- HwndStylusInputProvider.cs
- ExtendedPropertyDescriptor.cs
- DropSource.cs
- ComponentCommands.cs
- ResourcePermissionBase.cs
- SoapAttributeAttribute.cs
- DesignTimeValidationFeature.cs
- InputLanguage.cs
- Viewport3DAutomationPeer.cs
- QueryConverter.cs
- Splitter.cs
- DbDataReader.cs
- PngBitmapDecoder.cs
- ThicknessConverter.cs
- ImpersonationContext.cs
- MenuTracker.cs
- Stack.cs
- ConfigurationSchemaErrors.cs
- ConfigXmlWhitespace.cs
- ConfigurationValidatorBase.cs
- AssemblyCache.cs
- DictationGrammar.cs
- XmlSerializationGeneratedCode.cs
- ContextMarshalException.cs
- CustomSignedXml.cs
- PropertyTab.cs
- ApplicationException.cs
- ArrayTypeMismatchException.cs
- TreeView.cs
- PackageFilter.cs
- GregorianCalendar.cs
- MachineKeyValidationConverter.cs
- WeakEventManager.cs
- FormViewPageEventArgs.cs
- UserNamePasswordClientCredential.cs
- TypedDataSourceCodeGenerator.cs
- DuplexChannelFactory.cs
- MyContact.cs
- LocalValueEnumerator.cs
- Image.cs