Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / GlobalEventManager.cs / 1305600 / GlobalEventManager.cs
using System; using System.Collections; using System.Diagnostics; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { internal static class GlobalEventManager { #region Operations // Registers a RoutedEvent with the given details // NOTE: The Name must be unique within the given OwnerType internal static RoutedEvent RegisterRoutedEvent( string name, RoutingStrategy routingStrategy, Type handlerType, Type ownerType) { Debug.Assert(GetRoutedEventFromName(name, ownerType, false) == null, "RoutedEvent name must be unique within a given OwnerType"); lock (Synchronized) { // Create a new RoutedEvent // Requires GlobalLock to access _countRoutedEvents RoutedEvent routedEvent = new RoutedEvent( name, routingStrategy, handlerType, ownerType); // Increment the count for registered RoutedEvents // Requires GlobalLock to access _countRoutedEvents _countRoutedEvents++; AddOwner(routedEvent, ownerType); return routedEvent; } } // Register a Class Handler // NOTE: Handler Type must be the // same as the one specified when // registering the corresponding RoutedEvent internal static void RegisterClassHandler( Type classType, RoutedEvent routedEvent, Delegate handler, bool handledEventsToo) { Debug.Assert( typeof(UIElement).IsAssignableFrom(classType) || typeof(ContentElement).IsAssignableFrom(classType) || typeof(UIElement3D).IsAssignableFrom(classType), "Class Handlers can be registered only for UIElement/ContentElement/UIElement3D and their sub types"); Debug.Assert(routedEvent.IsLegalHandler(handler), "Handler Type mismatch"); ClassHandlersStore classListenersLists; int index; // We map the classType to a DType use DTypeMap for storage DependencyObjectType dType = DependencyObjectType.FromSystemTypeInternal(classType); // Get the updated EventHandlersStore for the given DType GetDTypedClassListeners(dType, routedEvent, out classListenersLists, out index); // Reuired to update storage lock (Synchronized) { // Add new routed event handler and get the updated set of handlers RoutedEventHandlerInfoList updatedClassListeners = classListenersLists.AddToExistingHandlers(index, handler, handledEventsToo); // Update Sub Classes ItemStructListkeys = _dTypedClassListeners.ActiveDTypes; for (int i=0; i keys = _dTypedRoutedEventList.ActiveDTypes; int destIndex = 0; for (int i=0; i dTypedRoutedEventList = (FrugalObjectList )_dTypedRoutedEventList[keys.List[i]]; for(int j = 0; j < dTypedRoutedEventList.Count; j++) { RoutedEvent routedEvent = dTypedRoutedEventList[j]; if(Array.IndexOf(routedEvents, routedEvent) < 0) { routedEvents[destIndex++] = routedEvent; } } } // Enumerate through all of the RoutedEvents in the Hashtable // Requires GlobalLock to access _ownerTypedRoutedEventList IDictionaryEnumerator htEnumerator = _ownerTypedRoutedEventList.GetEnumerator(); while(htEnumerator.MoveNext() == true) { FrugalObjectList ownerRoutedEventList = (FrugalObjectList )htEnumerator.Value; for(int j = 0; j < ownerRoutedEventList.Count; j++) { RoutedEvent routedEvent = ownerRoutedEventList[j]; if(Array.IndexOf(routedEvents, routedEvent) < 0) { routedEvents[destIndex++] = routedEvent; } } } } return routedEvents; } internal static void AddOwner(RoutedEvent routedEvent, Type ownerType) { // If the ownerType is a subclass of DependencyObject // we map it to a DType use DTypeMap for storage else // we use the more generic Hashtable. if ((ownerType == typeof(DependencyObject)) || ownerType.IsSubclassOf(typeof(DependencyObject))) { DependencyObjectType dType = DependencyObjectType.FromSystemTypeInternal(ownerType); // Get the ItemList of RoutedEvents for the given OwnerType // Requires GlobalLock to access _dTypedRoutedEventList object ownerRoutedEventListObj = _dTypedRoutedEventList[dType]; FrugalObjectList ownerRoutedEventList; if (ownerRoutedEventListObj == null) { // Create an ItemList of RoutedEvents for the // given OwnerType if one does not already exist ownerRoutedEventList = new FrugalObjectList (1); _dTypedRoutedEventList[dType] = ownerRoutedEventList; } else { ownerRoutedEventList = (FrugalObjectList )ownerRoutedEventListObj; } // Add the newly created // RoutedEvent to the ItemList // Requires GlobalLock to access ownerRoutedEventList if(!ownerRoutedEventList.Contains(routedEvent)) { ownerRoutedEventList.Add(routedEvent); } } else { // Get the ItemList of RoutedEvents for the given OwnerType // Requires GlobalLock to access _ownerTypedRoutedEventList object ownerRoutedEventListObj = _ownerTypedRoutedEventList[ownerType]; FrugalObjectList ownerRoutedEventList; if (ownerRoutedEventListObj == null) { // Create an ItemList of RoutedEvents for the // given OwnerType if one does not already exist ownerRoutedEventList = new FrugalObjectList (1); _ownerTypedRoutedEventList[ownerType] = ownerRoutedEventList; } else { ownerRoutedEventList = (FrugalObjectList )ownerRoutedEventListObj; } // Add the newly created // RoutedEvent to the ItemList // Requires GlobalLock to access ownerRoutedEventList if(!ownerRoutedEventList.Contains(routedEvent)) { ownerRoutedEventList.Add(routedEvent); } } } // Returns a RoutedEvents that match // the ownerType input param // If not found returns null internal static RoutedEvent[] GetRoutedEventsForOwner(Type ownerType) { if ((ownerType == typeof(DependencyObject)) || ownerType.IsSubclassOf(typeof(DependencyObject))) { // Search DTypeMap DependencyObjectType dType = DependencyObjectType.FromSystemTypeInternal(ownerType); // Get the ItemList of RoutedEvents for the given DType FrugalObjectList ownerRoutedEventList = (FrugalObjectList )_dTypedRoutedEventList[dType]; if (ownerRoutedEventList != null) { return ownerRoutedEventList.ToArray(); } } else // Search Hashtable { // Get the ItemList of RoutedEvents for the given OwnerType FrugalObjectList ownerRoutedEventList = (FrugalObjectList )_ownerTypedRoutedEventList[ownerType]; if (ownerRoutedEventList != null) { return ownerRoutedEventList.ToArray(); } } // No match found return null; } // Returns a RoutedEvents that match // the name and ownerType input params // If not found returns null internal static RoutedEvent GetRoutedEventFromName( string name, Type ownerType, bool includeSupers) { if ((ownerType == typeof(DependencyObject)) || ownerType.IsSubclassOf(typeof(DependencyObject))) { // Search DTypeMap DependencyObjectType dType = DependencyObjectType.FromSystemTypeInternal(ownerType); while (dType != null) { // Get the ItemList of RoutedEvents for the given DType FrugalObjectList ownerRoutedEventList = (FrugalObjectList )_dTypedRoutedEventList[dType]; if (ownerRoutedEventList != null) { // Check for RoutedEvent with matching name in the ItemList for (int i=0; i ownerRoutedEventList = (FrugalObjectList )_ownerTypedRoutedEventList[ownerType]; if (ownerRoutedEventList != null) { // Check for RoutedEvent with matching name in the ItemList for (int i=0; i = Int32.MaxValue) { throw new InvalidOperationException(SR.Get(SRID.TooManyRoutedEvents)); } index = _globalIndexToEventMap.Add(value); } return index; } // Must be called from within a lock of GlobalEventManager.Synchronized internal static object EventFromGlobalIndex(int globalIndex) { return _globalIndexToEventMap[globalIndex]; } // must be used within a lock of GlobalEventManager.Synchronized private static ArrayList _globalIndexToEventMap = new ArrayList(100); // #endregion #region Data // This is an efficient Hashtable of ItemLists keyed on DType // Each ItemList holds the registered RoutedEvents for that OwnerType private static DTypeMap _dTypedRoutedEventList = new DTypeMap(10); // Initialization sizes based on typical MSN scenario // This is a Hashtable of ItemLists keyed on OwnerType // Each ItemList holds the registered RoutedEvents for that OwnerType private static Hashtable _ownerTypedRoutedEventList = new Hashtable(10); // Initialization sizes based on typical MSN scenario // This member keeps a count of the total number of Routed Events registered so far // The member also serves as the internally used ComputedEventIndex that indexes // EventListenersListss that store class handler information for a class type private static int _countRoutedEvents = 0; // This is an efficient Hashtable of ItemLists keyed on DType // Each ItemList holds the registered RoutedEvent class handlers for that ClassType private static DTypeMap _dTypedClassListeners = new DTypeMap(100); // Initialization sizes based on typical Expression Blend startup scenario // This is the cached value for the DType of DependencyObject private static DependencyObjectType _dependencyObjectType = DependencyObjectType.FromSystemTypeInternal(typeof(DependencyObject)); internal static object Synchronized = new object(); #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
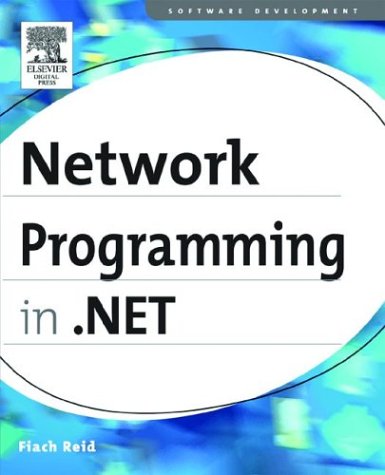
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MailMessage.cs
- CultureMapper.cs
- PathData.cs
- MsmqIntegrationSecurityElement.cs
- IsolatedStorageSecurityState.cs
- Model3DGroup.cs
- SqlFacetAttribute.cs
- WSAddressing10ProblemHeaderQNameFault.cs
- SQLRoleProvider.cs
- Control.cs
- X509SecurityTokenAuthenticator.cs
- ElementAction.cs
- ImageSource.cs
- LayoutManager.cs
- ConstantCheck.cs
- ClientRuntimeConfig.cs
- ImageCollectionEditor.cs
- ComponentCollection.cs
- SevenBitStream.cs
- MemberInfoSerializationHolder.cs
- CryptographicAttribute.cs
- Point.cs
- SqlServices.cs
- SQLInt64Storage.cs
- FragmentQueryProcessor.cs
- TypeDescriptionProvider.cs
- Internal.cs
- SafeHandles.cs
- ExpandCollapsePattern.cs
- WebPartsSection.cs
- ProfileSection.cs
- WebServiceTypeData.cs
- ActivityCodeDomReferenceService.cs
- ElementAction.cs
- EventMemberCodeDomSerializer.cs
- ListControlConvertEventArgs.cs
- SafeMILHandle.cs
- BitmapEffectInput.cs
- Effect.cs
- WebUtility.cs
- ErrorTableItemStyle.cs
- ScalarType.cs
- LogicalExpr.cs
- InvokePatternIdentifiers.cs
- DataGridViewRowHeaderCell.cs
- ListViewItem.cs
- DataControlField.cs
- XmlNotation.cs
- ObjectStorage.cs
- ImageSource.cs
- TableChangeProcessor.cs
- PropertyMapper.cs
- Pair.cs
- Metafile.cs
- ActivationWorker.cs
- OrderByExpression.cs
- PackagingUtilities.cs
- HostedElements.cs
- ApplicationId.cs
- Freezable.cs
- ClientSponsor.cs
- ObjectAssociationEndMapping.cs
- Size.cs
- OpacityConverter.cs
- ComponentCodeDomSerializer.cs
- RunInstallerAttribute.cs
- SqlCommand.cs
- DataGridViewRowCollection.cs
- ObfuscateAssemblyAttribute.cs
- XmlAnyElementAttributes.cs
- xsdvalidator.cs
- RemotingSurrogateSelector.cs
- TaskDesigner.cs
- WebPartConnectionsConfigureVerb.cs
- InvalidateEvent.cs
- EncoderReplacementFallback.cs
- SymmetricAlgorithm.cs
- _NestedMultipleAsyncResult.cs
- BuildProviderCollection.cs
- EncodingNLS.cs
- UniformGrid.cs
- FirstMatchCodeGroup.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- BitmapEffectCollection.cs
- TextAnchor.cs
- TransactionManager.cs
- EmptyCollection.cs
- WeakHashtable.cs
- GridViewColumnCollectionChangedEventArgs.cs
- FixUp.cs
- RoutedPropertyChangedEventArgs.cs
- ColumnMapVisitor.cs
- Sentence.cs
- FixedLineResult.cs
- PersonalizationDictionary.cs
- WriteableBitmap.cs
- AuthorizationRuleCollection.cs
- UntrustedRecipientException.cs
- HttpModulesSection.cs
- NetTcpSecurityElement.cs