Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / QueryRewriting / FragmentQueryProcessor.cs / 1 / FragmentQueryProcessor.cs
using System; using System.Diagnostics; using System.Collections.Generic; using System.Text; using System.Data.Common.Utils; using System.Data.Common.Utils.Boolean; using System.Data.Mapping.ViewGeneration.Structures; using System.Data.Metadata.Edm; using System.Linq; using System.Globalization; namespace System.Data.Mapping.ViewGeneration.QueryRewriting { using BoolDomainConstraint = DomainConstraint; internal class FragmentQueryProcessor : TileQueryProcessor { private FragmentQueryKB _kb; public FragmentQueryProcessor(FragmentQueryKB kb) { _kb = kb; } internal static FragmentQueryProcessor Merge(FragmentQueryProcessor qp1, FragmentQueryProcessor qp2) { FragmentQueryKB mergedKB = new FragmentQueryKB(); mergedKB.AddKnowledgeBase(qp1.KnowledgeBase); mergedKB.AddKnowledgeBase(qp2.KnowledgeBase); return new FragmentQueryProcessor(mergedKB); } internal FragmentQueryKB KnowledgeBase { get { return _kb; } } // resulting query contains an intersection of attributes internal override FragmentQuery Union(FragmentQuery q1, FragmentQuery q2) { HashSet attributes = new HashSet (q1.Attributes); attributes.IntersectWith(q2.Attributes); BoolExpression condition = BoolExpression.CreateOr(q1.Condition, q2.Condition); return FragmentQuery.Create(attributes, condition); } internal bool IsDisjointFrom(FragmentQuery q1, FragmentQuery q2) { return !IsSatisfiable(Intersect(q1, q2)); } internal bool IsContainedIn(FragmentQuery q1, FragmentQuery q2) { return !IsSatisfiable(Difference(q1, q2)); } internal bool IsEquivalentTo(FragmentQuery q1, FragmentQuery q2) { return IsContainedIn(q1, q2) && IsContainedIn(q2, q1); } internal override FragmentQuery Intersect(FragmentQuery q1, FragmentQuery q2) { HashSet attributes = new HashSet (q1.Attributes); attributes.IntersectWith(q2.Attributes); BoolExpression condition = BoolExpression.CreateAnd(q1.Condition, q2.Condition); return FragmentQuery.Create(attributes, condition); } internal override FragmentQuery Difference(FragmentQuery qA, FragmentQuery qB) { return FragmentQuery.Create(qA.Attributes, BoolExpression.CreateAndNot(qA.Condition, qB.Condition)); } internal override bool IsSatisfiable(FragmentQuery query) { return IsSatisfiable(query.Condition); } private bool IsSatisfiable(BoolExpression condition) { // instantiate conversion context for each check - gives better performance BoolExpression conditionUnderKB = condition.Create( new AndExpr (_kb.KbExpression, condition.Tree)); var context = IdentifierService .Instance.CreateConversionContext(); var converter = new Converter (conditionUnderKB.Tree, context); bool isSatisfiable = converter.Vertex.IsZero() == false; return isSatisfiable; } // creates "derived" views that may be helpful for answering the query // for example, view = SELECT ID WHERE B=2, query = SELECT ID,B WHERE B=2 // Created derived view: SELECT ID,B WHERE B=2 by adding the attribute whose value is determined by the where clause to projected list internal override FragmentQuery CreateDerivedViewBySelectingConstantAttributes(FragmentQuery view) { HashSet newProjectedAttributes = new HashSet (); // collect all variables from the view IEnumerable > variables = view.Condition.Variables; foreach (DomainVariable var in variables) { OneOfConst variableCondition = var.Identifier as OneOfConst; if (variableCondition != null) { // Is this attribute not already projected? MemberPath conditionMember = variableCondition.Slot.MemberPath; // Iterating through the variable domain var.Domain could be wasteful // Instead, consider the actual condition values on the variable. Usually, they don't get repeated (if not, we could cache and check) CellConstantDomain conditionValues = variableCondition.Values; if (false == view.Attributes.Contains(conditionMember)) { foreach (CellConstant value in conditionValues.Values) { // construct constraint: X = value DomainConstraint constraint = new DomainConstraint (var, new Set (new CellConstant[] { value }, CellConstant.EqualityComparer)); // is this constraint implied by the where clause? BoolExpression exclusion = view.Condition.Create( new AndExpr >(view.Condition.Tree, new NotExpr >(new TermExpr >(constraint)))); bool isImplied = false == IsSatisfiable(exclusion); if (isImplied) { // add this variable to the projection, if it is used in the query newProjectedAttributes.Add(conditionMember); } } } } } if (newProjectedAttributes.Count > 0) { newProjectedAttributes.UnionWith(view.Attributes); FragmentQuery derivedView = new FragmentQuery(String.Format(CultureInfo.InvariantCulture, "project({0})", view.Description), view.FromVariable, newProjectedAttributes, view.Condition); return derivedView; } return null; } public override string ToString() { return _kb.ToString(); } #region Private class AttributeSetComparator private class AttributeSetComparator : IEqualityComparer > { internal static readonly AttributeSetComparator DefaultInstance = new AttributeSetComparator(); #region IEqualityComparer > Members public bool Equals(HashSet x, HashSet y) { return x.SetEquals(y); } public int GetHashCode(HashSet attrs) { int hashCode = 123; foreach (MemberPath attr in attrs) { hashCode += MemberPath.EqualityComparer.GetHashCode(attr) * 7; } return hashCode; } #endregion } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Diagnostics; using System.Collections.Generic; using System.Text; using System.Data.Common.Utils; using System.Data.Common.Utils.Boolean; using System.Data.Mapping.ViewGeneration.Structures; using System.Data.Metadata.Edm; using System.Linq; using System.Globalization; namespace System.Data.Mapping.ViewGeneration.QueryRewriting { using BoolDomainConstraint = DomainConstraint ; internal class FragmentQueryProcessor : TileQueryProcessor { private FragmentQueryKB _kb; public FragmentQueryProcessor(FragmentQueryKB kb) { _kb = kb; } internal static FragmentQueryProcessor Merge(FragmentQueryProcessor qp1, FragmentQueryProcessor qp2) { FragmentQueryKB mergedKB = new FragmentQueryKB(); mergedKB.AddKnowledgeBase(qp1.KnowledgeBase); mergedKB.AddKnowledgeBase(qp2.KnowledgeBase); return new FragmentQueryProcessor(mergedKB); } internal FragmentQueryKB KnowledgeBase { get { return _kb; } } // resulting query contains an intersection of attributes internal override FragmentQuery Union(FragmentQuery q1, FragmentQuery q2) { HashSet attributes = new HashSet (q1.Attributes); attributes.IntersectWith(q2.Attributes); BoolExpression condition = BoolExpression.CreateOr(q1.Condition, q2.Condition); return FragmentQuery.Create(attributes, condition); } internal bool IsDisjointFrom(FragmentQuery q1, FragmentQuery q2) { return !IsSatisfiable(Intersect(q1, q2)); } internal bool IsContainedIn(FragmentQuery q1, FragmentQuery q2) { return !IsSatisfiable(Difference(q1, q2)); } internal bool IsEquivalentTo(FragmentQuery q1, FragmentQuery q2) { return IsContainedIn(q1, q2) && IsContainedIn(q2, q1); } internal override FragmentQuery Intersect(FragmentQuery q1, FragmentQuery q2) { HashSet attributes = new HashSet (q1.Attributes); attributes.IntersectWith(q2.Attributes); BoolExpression condition = BoolExpression.CreateAnd(q1.Condition, q2.Condition); return FragmentQuery.Create(attributes, condition); } internal override FragmentQuery Difference(FragmentQuery qA, FragmentQuery qB) { return FragmentQuery.Create(qA.Attributes, BoolExpression.CreateAndNot(qA.Condition, qB.Condition)); } internal override bool IsSatisfiable(FragmentQuery query) { return IsSatisfiable(query.Condition); } private bool IsSatisfiable(BoolExpression condition) { // instantiate conversion context for each check - gives better performance BoolExpression conditionUnderKB = condition.Create( new AndExpr (_kb.KbExpression, condition.Tree)); var context = IdentifierService .Instance.CreateConversionContext(); var converter = new Converter (conditionUnderKB.Tree, context); bool isSatisfiable = converter.Vertex.IsZero() == false; return isSatisfiable; } // creates "derived" views that may be helpful for answering the query // for example, view = SELECT ID WHERE B=2, query = SELECT ID,B WHERE B=2 // Created derived view: SELECT ID,B WHERE B=2 by adding the attribute whose value is determined by the where clause to projected list internal override FragmentQuery CreateDerivedViewBySelectingConstantAttributes(FragmentQuery view) { HashSet newProjectedAttributes = new HashSet (); // collect all variables from the view IEnumerable > variables = view.Condition.Variables; foreach (DomainVariable var in variables) { OneOfConst variableCondition = var.Identifier as OneOfConst; if (variableCondition != null) { // Is this attribute not already projected? MemberPath conditionMember = variableCondition.Slot.MemberPath; // Iterating through the variable domain var.Domain could be wasteful // Instead, consider the actual condition values on the variable. Usually, they don't get repeated (if not, we could cache and check) CellConstantDomain conditionValues = variableCondition.Values; if (false == view.Attributes.Contains(conditionMember)) { foreach (CellConstant value in conditionValues.Values) { // construct constraint: X = value DomainConstraint constraint = new DomainConstraint (var, new Set (new CellConstant[] { value }, CellConstant.EqualityComparer)); // is this constraint implied by the where clause? BoolExpression exclusion = view.Condition.Create( new AndExpr >(view.Condition.Tree, new NotExpr >(new TermExpr >(constraint)))); bool isImplied = false == IsSatisfiable(exclusion); if (isImplied) { // add this variable to the projection, if it is used in the query newProjectedAttributes.Add(conditionMember); } } } } } if (newProjectedAttributes.Count > 0) { newProjectedAttributes.UnionWith(view.Attributes); FragmentQuery derivedView = new FragmentQuery(String.Format(CultureInfo.InvariantCulture, "project({0})", view.Description), view.FromVariable, newProjectedAttributes, view.Condition); return derivedView; } return null; } public override string ToString() { return _kb.ToString(); } #region Private class AttributeSetComparator private class AttributeSetComparator : IEqualityComparer > { internal static readonly AttributeSetComparator DefaultInstance = new AttributeSetComparator(); #region IEqualityComparer > Members public bool Equals(HashSet x, HashSet y) { return x.SetEquals(y); } public int GetHashCode(HashSet attrs) { int hashCode = 123; foreach (MemberPath attr in attrs) { hashCode += MemberPath.EqualityComparer.GetHashCode(attr) * 7; } return hashCode; } #endregion } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
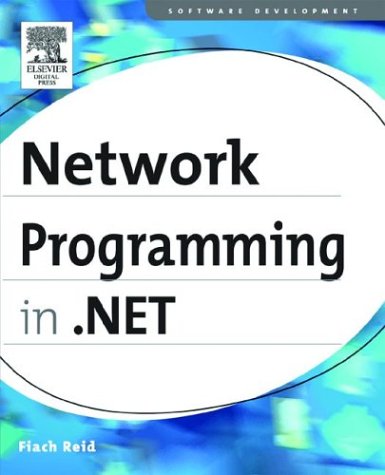
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SinglePageViewer.cs
- PropertyManager.cs
- DecimalStorage.cs
- AlignmentXValidation.cs
- XmlQueryTypeFactory.cs
- TabControl.cs
- NetStream.cs
- ParameterCollection.cs
- ContextStaticAttribute.cs
- CodeTypeReferenceCollection.cs
- storepermissionattribute.cs
- COMException.cs
- NotifyParentPropertyAttribute.cs
- PolicyLevel.cs
- WebControl.cs
- DataRelationPropertyDescriptor.cs
- LinkTarget.cs
- TraceListeners.cs
- ObjectComplexPropertyMapping.cs
- MessageDecoder.cs
- DocumentPageView.cs
- __Filters.cs
- RIPEMD160Managed.cs
- CodeArrayCreateExpression.cs
- ClientTargetSection.cs
- SkinIDTypeConverter.cs
- remotingproxy.cs
- XmlSchemaExternal.cs
- HtmlElementCollection.cs
- ModifierKeysValueSerializer.cs
- ThrowOnMultipleAssignment.cs
- GACMembershipCondition.cs
- ToolStripItemCollection.cs
- StrongNameKeyPair.cs
- _SslStream.cs
- WindowsTokenRoleProvider.cs
- QueryCacheManager.cs
- OdbcParameterCollection.cs
- DialogBaseForm.cs
- MDIClient.cs
- DataGridViewRowEventArgs.cs
- ListViewItemEventArgs.cs
- WsatServiceAddress.cs
- PresentationAppDomainManager.cs
- PersonalizationProviderHelper.cs
- RewritingPass.cs
- JsonObjectDataContract.cs
- GroupItem.cs
- Size.cs
- CollectionTypeElement.cs
- GridViewSortEventArgs.cs
- DataControlFieldCell.cs
- ProxyWebPartManager.cs
- ZipArchive.cs
- AssemblyHash.cs
- SearchForVirtualItemEventArgs.cs
- ParseChildrenAsPropertiesAttribute.cs
- LocalizableAttribute.cs
- ThrowHelper.cs
- versioninfo.cs
- EntityDataSourceContextCreatingEventArgs.cs
- UrlMapping.cs
- StickyNoteHelper.cs
- VisualStateManager.cs
- Image.cs
- COM2ColorConverter.cs
- HexParser.cs
- UpdateRecord.cs
- OrderByQueryOptionExpression.cs
- PopupControlService.cs
- NamespaceQuery.cs
- ImageMapEventArgs.cs
- RecognizerInfo.cs
- ScrollChrome.cs
- ReadOnlyMetadataCollection.cs
- WithStatement.cs
- HwndSourceParameters.cs
- DataPager.cs
- EntityClientCacheKey.cs
- XmlTypeMapping.cs
- RootProfilePropertySettingsCollection.cs
- securestring.cs
- MatrixKeyFrameCollection.cs
- FactoryGenerator.cs
- MetabaseSettings.cs
- XmlName.cs
- OleDragDropHandler.cs
- ElementsClipboardData.cs
- SimpleRecyclingCache.cs
- FormsAuthentication.cs
- ResourceType.cs
- ButtonColumn.cs
- DataPagerField.cs
- StaticTextPointer.cs
- CompilationRelaxations.cs
- MissingManifestResourceException.cs
- SizeValueSerializer.cs
- NameSpaceExtractor.cs
- LinqTreeNodeEvaluator.cs
- JsonReader.cs