Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / UIAutomation / UIAutomationClient / MS / Internal / Automation / SafeHandles.cs / 1 / SafeHandles.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Various SafeHandles used by UIA // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Automation; using System.Windows.Automation.Provider; // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace MS.Internal.Automation { internal sealed class SafeNodeHandle : SafeHandle { // Called by P/Invoke when returning SafeHandles // (Also used by UiaCoreApi to create invalid handles.) internal SafeNodeHandle() : base(IntPtr.Zero, true) { } // No need to provide a finalizer - SafeHandle's critical finalizer will // call ReleaseHandle for you. public override bool IsInvalid { get { return handle == IntPtr.Zero; } } override protected bool ReleaseHandle() { return UiaCoreApi.UiaNodeRelease(handle); } } // Internal Class that wraps the IntPtr to the Pattern internal sealed class SafePatternHandle : SafeHandle { // Called by P/Invoke when returning SafeHandles // (Also used by UiaCoreApi to create invalid handles.) internal SafePatternHandle() : base(IntPtr.Zero, true) { } // No need to provide a finalizer - SafeHandle's critical finalizer will // call ReleaseHandle for you. public override bool IsInvalid { get { return handle == IntPtr.Zero; } } override protected bool ReleaseHandle() { return UiaCoreApi.UiaPatternRelease(handle); } } // Internal Class that wraps the IntPtr to the Event internal sealed class SafeEventHandle : SafeHandle { internal SafeEventHandle() : base(IntPtr.Zero, true) { } public override bool IsInvalid { get { return handle == IntPtr.Zero; } } override protected bool ReleaseHandle() { UiaCoreApi.UiaRemoveEvent(handle); return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Various SafeHandles used by UIA // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Automation; using System.Windows.Automation.Provider; // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace MS.Internal.Automation { internal sealed class SafeNodeHandle : SafeHandle { // Called by P/Invoke when returning SafeHandles // (Also used by UiaCoreApi to create invalid handles.) internal SafeNodeHandle() : base(IntPtr.Zero, true) { } // No need to provide a finalizer - SafeHandle's critical finalizer will // call ReleaseHandle for you. public override bool IsInvalid { get { return handle == IntPtr.Zero; } } override protected bool ReleaseHandle() { return UiaCoreApi.UiaNodeRelease(handle); } } // Internal Class that wraps the IntPtr to the Pattern internal sealed class SafePatternHandle : SafeHandle { // Called by P/Invoke when returning SafeHandles // (Also used by UiaCoreApi to create invalid handles.) internal SafePatternHandle() : base(IntPtr.Zero, true) { } // No need to provide a finalizer - SafeHandle's critical finalizer will // call ReleaseHandle for you. public override bool IsInvalid { get { return handle == IntPtr.Zero; } } override protected bool ReleaseHandle() { return UiaCoreApi.UiaPatternRelease(handle); } } // Internal Class that wraps the IntPtr to the Event internal sealed class SafeEventHandle : SafeHandle { internal SafeEventHandle() : base(IntPtr.Zero, true) { } public override bool IsInvalid { get { return handle == IntPtr.Zero; } } override protected bool ReleaseHandle() { UiaCoreApi.UiaRemoveEvent(handle); return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
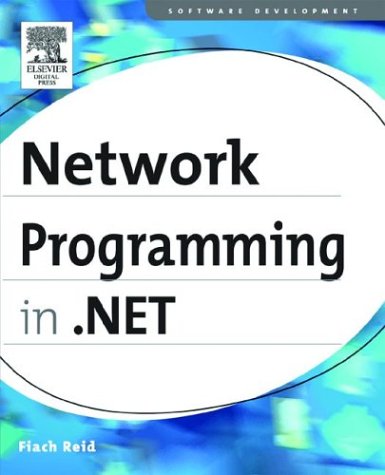
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializationSectionGroup.cs
- RangeBase.cs
- CompiledIdentityConstraint.cs
- TrueReadOnlyCollection.cs
- TemplateBaseAction.cs
- Base64Encoder.cs
- BufferedOutputStream.cs
- followingsibling.cs
- BitmapEffectRenderDataResource.cs
- UIElement3DAutomationPeer.cs
- CustomTypeDescriptor.cs
- XamlInterfaces.cs
- EndpointAddress.cs
- CompatibleComparer.cs
- FunctionUpdateCommand.cs
- PackageRelationshipCollection.cs
- StorageConditionPropertyMapping.cs
- HMACSHA256.cs
- DataContractSerializerElement.cs
- OrderingQueryOperator.cs
- AccessText.cs
- ListDictionary.cs
- DtrList.cs
- fixedPageContentExtractor.cs
- CommonProperties.cs
- CellTreeNodeVisitors.cs
- Transform.cs
- ListQueryResults.cs
- NamedObject.cs
- IdentifierService.cs
- cryptoapiTransform.cs
- NameTable.cs
- ADRoleFactoryConfiguration.cs
- SqlServer2KCompatibilityCheck.cs
- WebPartConnectionsConnectVerb.cs
- ElementUtil.cs
- NamespaceList.cs
- ConfigsHelper.cs
- PackUriHelper.cs
- OpenTypeLayoutCache.cs
- ValidatorUtils.cs
- SmiTypedGetterSetter.cs
- CqlGenerator.cs
- FrameworkContentElement.cs
- NetworkInterface.cs
- SqlNotificationRequest.cs
- DockPattern.cs
- HelpFileFileNameEditor.cs
- EventSinkHelperWriter.cs
- ExclusiveTcpListener.cs
- ObjectDataSourceSelectingEventArgs.cs
- InputScopeConverter.cs
- BinaryObjectReader.cs
- XPSSignatureDefinition.cs
- CalendarDay.cs
- ComponentEditorPage.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- InheritanceAttribute.cs
- Axis.cs
- ClientSession.cs
- CodeGeneratorOptions.cs
- CopyNamespacesAction.cs
- RegexRunnerFactory.cs
- Rotation3DKeyFrameCollection.cs
- FrameworkContextData.cs
- TableLayout.cs
- TableCell.cs
- HttpCookiesSection.cs
- __ConsoleStream.cs
- altserialization.cs
- AuthenticationException.cs
- MobileTemplatedControlDesigner.cs
- ResizingMessageFilter.cs
- TextEditorCharacters.cs
- SymmetricKey.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- SoapElementAttribute.cs
- remotingproxy.cs
- FixedStringLookup.cs
- URLEditor.cs
- SecureEnvironment.cs
- DateTimeParse.cs
- ListViewInsertedEventArgs.cs
- SmiEventStream.cs
- SplitterPanel.cs
- SecurityCriticalDataForSet.cs
- DesignerActionUIStateChangeEventArgs.cs
- XmlEnumAttribute.cs
- XmlElementCollection.cs
- XmlValidatingReaderImpl.cs
- MachineKeySection.cs
- ToolStripGrip.cs
- NTAccount.cs
- ColumnMap.cs
- KeyManager.cs
- ObjectCloneHelper.cs
- NotifyInputEventArgs.cs
- ToolStripLabel.cs
- HttpPostedFile.cs
- DataMisalignedException.cs