Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Shapes / Polyline.cs / 1 / Polyline.cs
//---------------------------------------------------------------------------- // File: Polyline.cs // // Description: // Implementation of Polyline shape element. // // History: // 05/30/02 - [....] - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The Polyline shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// public sealed class Polyline : Shape { #region Constructors ////// Instantiates a new instance of a Polyline. /// public Polyline() { } #endregion Constructors #region Dynamic Properties ////// Points property /// public static readonly DependencyProperty PointsProperty = DependencyProperty.Register( "Points", typeof(PointCollection), typeof(Polyline), new FrameworkPropertyMetadata(new FreezableDefaultValueFactory(PointCollection.Empty), FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender)); ////// Points property /// public PointCollection Points { get { return (PointCollection)GetValue(PointsProperty); } set { SetValue(PointsProperty, value); } } ////// FillRule property /// public static readonly DependencyProperty FillRuleProperty = DependencyProperty.Register( "FillRule", typeof(FillRule), typeof(Polyline), new FrameworkPropertyMetadata( FillRule.EvenOdd, FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsFillRuleValid) ); ////// FillRule property /// public FillRule FillRule { get { return (FillRule)GetValue(FillRuleProperty); } set { SetValue(FillRuleProperty, value); } } #endregion Dynamic Properties #region Protected Methods and Properties ////// Get the polyline that defines this shape /// protected override Geometry DefiningGeometry { get { return _polylineGeometry; } } #endregion #region Internal methods internal override void CacheDefiningGeometry() { PointCollection pointCollection = Points; PathFigure pathFigure = new PathFigure(); // Are we degenerate? // Yes, if we don't have data if (pointCollection == null) { _polylineGeometry = Geometry.Empty; return; } // Create the Polyline PathGeometry // if (pointCollection.Count > 0) { pathFigure.StartPoint = pointCollection[0]; if (pointCollection.Count > 1) { Point[] array = new Point[pointCollection.Count - 1]; for (int i = 1; i < pointCollection.Count; i++) { array[i - 1] = pointCollection[i]; } pathFigure.Segments.Add(new PolyLineSegment(array, true)); } } PathGeometry polylineGeometry = new PathGeometry(); polylineGeometry.Figures.Add(pathFigure); // Set FillRule polylineGeometry.FillRule = FillRule; if (polylineGeometry.Bounds == Rect.Empty) { _polylineGeometry = Geometry.Empty; } else { _polylineGeometry = polylineGeometry; } } #endregion Internal methods #region Private Methods and Members private Geometry _polylineGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
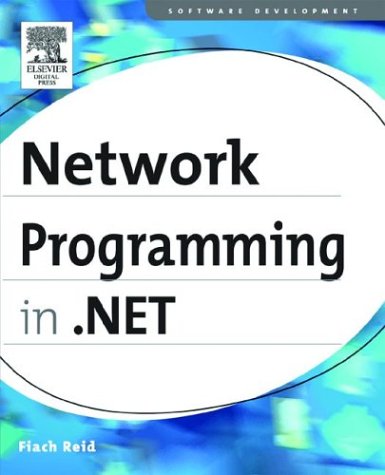
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextEditorTyping.cs
- SliderAutomationPeer.cs
- SerializationEventsCache.cs
- VolatileResourceManager.cs
- ToolStripDropDownClosingEventArgs.cs
- TriggerAction.cs
- dataSvcMapFileLoader.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- DocumentOrderComparer.cs
- BufferCache.cs
- _SslStream.cs
- SrgsRuleRef.cs
- ScriptMethodAttribute.cs
- DataBoundLiteralControl.cs
- WebFaultClientMessageInspector.cs
- TypeDescriptionProviderAttribute.cs
- EdmEntityTypeAttribute.cs
- EntityModelBuildProvider.cs
- WebMessageEncodingElement.cs
- CultureTable.cs
- ChangeDirector.cs
- XD.cs
- ICspAsymmetricAlgorithm.cs
- KeyFrames.cs
- XmlDictionary.cs
- RoutedEventArgs.cs
- xsdvalidator.cs
- CounterSample.cs
- MemoryPressure.cs
- PropertiesTab.cs
- __Filters.cs
- XmlSchemaExporter.cs
- _ConnectStream.cs
- SqlError.cs
- FormCollection.cs
- ZoomPercentageConverter.cs
- Suspend.cs
- CompositeDesignerAccessibleObject.cs
- SchemaMapping.cs
- SqlCommandSet.cs
- Exceptions.cs
- BindingWorker.cs
- BookmarkScopeManager.cs
- GlyphInfoList.cs
- IItemProperties.cs
- StringUtil.cs
- WinEventQueueItem.cs
- BaseCollection.cs
- AssociatedControlConverter.cs
- OdbcInfoMessageEvent.cs
- SimpleColumnProvider.cs
- DesignerActionMethodItem.cs
- SettingsPropertyWrongTypeException.cs
- StylusPointDescription.cs
- DynamicPhysicalDiscoSearcher.cs
- VectorValueSerializer.cs
- UnicodeEncoding.cs
- WebConfigManager.cs
- Thumb.cs
- BamlTreeUpdater.cs
- FixedPageProcessor.cs
- TextRangeBase.cs
- ViewSimplifier.cs
- XmlFormatWriterGenerator.cs
- PolyBezierSegment.cs
- PathTooLongException.cs
- EventManager.cs
- GenericsNotImplementedException.cs
- StateWorkerRequest.cs
- SpanIndex.cs
- ContractMethodParameterInfo.cs
- SqlConnection.cs
- Parser.cs
- DataBoundControlDesigner.cs
- DataDesignUtil.cs
- EventListener.cs
- SqlDataSourceStatusEventArgs.cs
- ServiceBusyException.cs
- Int64Converter.cs
- SingleTagSectionHandler.cs
- RectKeyFrameCollection.cs
- TextModifierScope.cs
- XmlEventCache.cs
- InlinedAggregationOperator.cs
- EmptyEnumerator.cs
- Vars.cs
- ChannelTracker.cs
- WebPartCancelEventArgs.cs
- DataBinder.cs
- TableDetailsCollection.cs
- BindableAttribute.cs
- BinaryObjectInfo.cs
- PageThemeCodeDomTreeGenerator.cs
- LocalizationParserHooks.cs
- PolicyChain.cs
- ObjectRef.cs
- MessageBox.cs
- CommandLibraryHelper.cs
- BitmapFrameEncode.cs
- HtmlEmptyTagControlBuilder.cs