Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / DataBoundLiteralControl.cs / 1 / DataBoundLiteralControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Control that holds databinding expressions and literals * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.IO; using System.Text; using System.Security.Permissions; using System.Web.Util; internal class DataBoundLiteralControlBuilder : ControlBuilder { internal DataBoundLiteralControlBuilder() { } internal void AddLiteralString(string s) { Debug.Assert(!InDesigner, "!InDesigner"); // Make sure strings and databinding expressions alternate object lastBuilder = GetLastBuilder(); if (lastBuilder != null && lastBuilder is string) { AddSubBuilder(null); } AddSubBuilder(s); } internal void AddDataBindingExpression(CodeBlockBuilder codeBlockBuilder) { Debug.Assert(!InDesigner, "!InDesigner"); // Make sure strings and databinding expressions alternate object lastBuilder = GetLastBuilder(); if (lastBuilder == null || lastBuilder is CodeBlockBuilder) { AddSubBuilder(null); } AddSubBuilder(codeBlockBuilder); } internal int GetStaticLiteralsCount() { // it's divided by 2 because half are strings and half are databinding // expressions). '+1' because we always start with a literal string. return (SubBuilders.Count+1) / 2; } internal int GetDataBoundLiteralCount() { // it's divided by 2 because half are strings and half are databinding // expressions) return SubBuilders.Count / 2; } } ////// [ ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DataBoundLiteralControl : Control, ITextControl { private string[] _staticLiterals; private string[] _dataBoundLiteral; private bool _hasDataBoundStrings; ///Defines the properties and methods of the DataBoundLiteralControl class. ///public DataBoundLiteralControl(int staticLiteralsCount, int dataBoundLiteralCount) { _staticLiterals = new string[staticLiteralsCount]; _dataBoundLiteral = new string[dataBoundLiteralCount]; PreventAutoID(); } /// public void SetStaticString(int index, string s) { _staticLiterals[index] = s; } /// public void SetDataBoundString(int index, string s) { _dataBoundLiteral[index] = s; _hasDataBoundStrings = true; } /// /// public string Text { get { StringBuilder sb = new StringBuilder(); int dataBoundLiteralCount = _dataBoundLiteral.Length; // Append literal and databound strings alternatively for (int i=0; i<_staticLiterals.Length; i++) { if (_staticLiterals[i] != null) sb.Append(_staticLiterals[i]); // Could be null if DataBind() was not called if (i < dataBoundLiteralCount && _dataBoundLiteral[i] != null) sb.Append(_dataBoundLiteral[i]); } return sb.ToString(); } } ///Gets the text content of the data-bound literal control. ///protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } /// /// /// protected override void LoadViewState(object savedState) { if (savedState != null) { _dataBoundLiteral = (string[]) savedState; _hasDataBoundStrings = true; } } ///Loads the previously saved state. Overridden to synchronize Text property with /// LiteralContent. ////// /// protected override object SaveViewState() { // Return null if we didn't get any databound strings if (!_hasDataBoundStrings) return null; // Only save the databound literals to the view state return _dataBoundLiteral; } ///The object that contains the state changes. ///protected internal override void Render(HtmlTextWriter output) { int dataBoundLiteralCount = _dataBoundLiteral.Length; // Render literal and databound strings alternatively for (int i=0; i<_staticLiterals.Length; i++) { if (_staticLiterals[i] != null) output.Write(_staticLiterals[i]); // Could be null if DataBind() was not called if (i < dataBoundLiteralCount && _dataBoundLiteral[i] != null) output.Write(_dataBoundLiteral[i]); } } /// /// /// string ITextControl.Text { get { return Text; } set { throw new NotSupportedException(); } } } ///Implementation of TextControl.Text property. ////// [ DataBindingHandler("System.Web.UI.Design.TextDataBindingHandler, " + AssemblyRef.SystemDesign), ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DesignerDataBoundLiteralControl : Control { private string _text; public DesignerDataBoundLiteralControl() { PreventAutoID(); } ///Simpler version of DataBoundLiteralControlBuilder, used at design time. ////// public string Text { get { return _text; } set { _text = (value != null) ? value : String.Empty; } } protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } ///Gets or sets the text content of the data-bound literal control. ////// protected override void LoadViewState(object savedState) { if (savedState != null) _text = (string) savedState; } ///Loads the previously saved state. Overridden to synchronize Text property with /// LiteralContent. ////// protected internal override void Render(HtmlTextWriter output) { output.Write(_text); } ///Saves any state that was modified after the control began monitoring state changes. ////// protected override object SaveViewState() { return _text; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //The object that contains the state changes. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Control that holds databinding expressions and literals * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.IO; using System.Text; using System.Security.Permissions; using System.Web.Util; internal class DataBoundLiteralControlBuilder : ControlBuilder { internal DataBoundLiteralControlBuilder() { } internal void AddLiteralString(string s) { Debug.Assert(!InDesigner, "!InDesigner"); // Make sure strings and databinding expressions alternate object lastBuilder = GetLastBuilder(); if (lastBuilder != null && lastBuilder is string) { AddSubBuilder(null); } AddSubBuilder(s); } internal void AddDataBindingExpression(CodeBlockBuilder codeBlockBuilder) { Debug.Assert(!InDesigner, "!InDesigner"); // Make sure strings and databinding expressions alternate object lastBuilder = GetLastBuilder(); if (lastBuilder == null || lastBuilder is CodeBlockBuilder) { AddSubBuilder(null); } AddSubBuilder(codeBlockBuilder); } internal int GetStaticLiteralsCount() { // it's divided by 2 because half are strings and half are databinding // expressions). '+1' because we always start with a literal string. return (SubBuilders.Count+1) / 2; } internal int GetDataBoundLiteralCount() { // it's divided by 2 because half are strings and half are databinding // expressions) return SubBuilders.Count / 2; } } ////// [ ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DataBoundLiteralControl : Control, ITextControl { private string[] _staticLiterals; private string[] _dataBoundLiteral; private bool _hasDataBoundStrings; ///Defines the properties and methods of the DataBoundLiteralControl class. ///public DataBoundLiteralControl(int staticLiteralsCount, int dataBoundLiteralCount) { _staticLiterals = new string[staticLiteralsCount]; _dataBoundLiteral = new string[dataBoundLiteralCount]; PreventAutoID(); } /// public void SetStaticString(int index, string s) { _staticLiterals[index] = s; } /// public void SetDataBoundString(int index, string s) { _dataBoundLiteral[index] = s; _hasDataBoundStrings = true; } /// /// public string Text { get { StringBuilder sb = new StringBuilder(); int dataBoundLiteralCount = _dataBoundLiteral.Length; // Append literal and databound strings alternatively for (int i=0; i<_staticLiterals.Length; i++) { if (_staticLiterals[i] != null) sb.Append(_staticLiterals[i]); // Could be null if DataBind() was not called if (i < dataBoundLiteralCount && _dataBoundLiteral[i] != null) sb.Append(_dataBoundLiteral[i]); } return sb.ToString(); } } ///Gets the text content of the data-bound literal control. ///protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } /// /// /// protected override void LoadViewState(object savedState) { if (savedState != null) { _dataBoundLiteral = (string[]) savedState; _hasDataBoundStrings = true; } } ///Loads the previously saved state. Overridden to synchronize Text property with /// LiteralContent. ////// /// protected override object SaveViewState() { // Return null if we didn't get any databound strings if (!_hasDataBoundStrings) return null; // Only save the databound literals to the view state return _dataBoundLiteral; } ///The object that contains the state changes. ///protected internal override void Render(HtmlTextWriter output) { int dataBoundLiteralCount = _dataBoundLiteral.Length; // Render literal and databound strings alternatively for (int i=0; i<_staticLiterals.Length; i++) { if (_staticLiterals[i] != null) output.Write(_staticLiterals[i]); // Could be null if DataBind() was not called if (i < dataBoundLiteralCount && _dataBoundLiteral[i] != null) output.Write(_dataBoundLiteral[i]); } } /// /// /// string ITextControl.Text { get { return Text; } set { throw new NotSupportedException(); } } } ///Implementation of TextControl.Text property. ////// [ DataBindingHandler("System.Web.UI.Design.TextDataBindingHandler, " + AssemblyRef.SystemDesign), ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DesignerDataBoundLiteralControl : Control { private string _text; public DesignerDataBoundLiteralControl() { PreventAutoID(); } ///Simpler version of DataBoundLiteralControlBuilder, used at design time. ////// public string Text { get { return _text; } set { _text = (value != null) ? value : String.Empty; } } protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } ///Gets or sets the text content of the data-bound literal control. ////// protected override void LoadViewState(object savedState) { if (savedState != null) _text = (string) savedState; } ///Loads the previously saved state. Overridden to synchronize Text property with /// LiteralContent. ////// protected internal override void Render(HtmlTextWriter output) { output.Write(_text); } ///Saves any state that was modified after the control began monitoring state changes. ////// protected override object SaveViewState() { return _text; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.The object that contains the state changes. ///
Link Menu
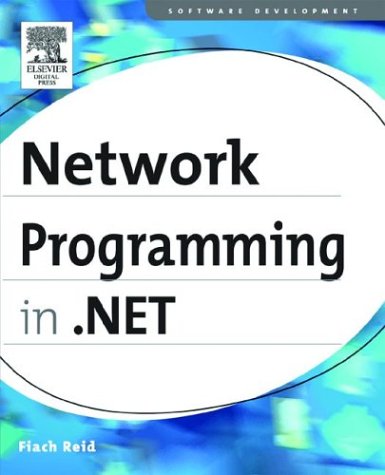
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EndpointConfigContainer.cs
- SqlGatherProducedAliases.cs
- XsltCompileContext.cs
- HtmlInputCheckBox.cs
- TextRangeBase.cs
- Debug.cs
- XmlMapping.cs
- ListControlActionList.cs
- Events.cs
- Timer.cs
- BindingWorker.cs
- DirectionalLight.cs
- ExpressionVisitor.cs
- DynamicDocumentPaginator.cs
- MessageBodyDescription.cs
- Visual3D.cs
- EqualityComparer.cs
- Claim.cs
- TiffBitmapDecoder.cs
- Separator.cs
- QueryableFilterUserControl.cs
- Token.cs
- Win32.cs
- HttpCachePolicyWrapper.cs
- EncoderFallback.cs
- LineSegment.cs
- TimeSpan.cs
- DynamicQueryableWrapper.cs
- XmlDocumentFieldSchema.cs
- RuntimeResourceSet.cs
- oledbmetadatacolumnnames.cs
- WebEventTraceProvider.cs
- KeyManager.cs
- DocumentApplicationJournalEntry.cs
- XmlSerializationReader.cs
- WebPartMinimizeVerb.cs
- SequenceDesigner.cs
- AssociationType.cs
- DuplicateDetector.cs
- ObjectDataSource.cs
- DataObject.cs
- DataServiceRequest.cs
- XMLDiffLoader.cs
- UnsafeNativeMethods.cs
- OrderingQueryOperator.cs
- ManagedWndProcTracker.cs
- LazyLoadBehavior.cs
- FillRuleValidation.cs
- BufferedWebEventProvider.cs
- ImageField.cs
- WeakRefEnumerator.cs
- TypeBrowser.xaml.cs
- ModifyActivitiesPropertyDescriptor.cs
- AddInStore.cs
- OlePropertyStructs.cs
- WindowsProgressbar.cs
- Size3DValueSerializer.cs
- SqlNode.cs
- MdiWindowListItemConverter.cs
- IisTraceListener.cs
- RuleSettingsCollection.cs
- WebPartMenu.cs
- Visitor.cs
- QilReplaceVisitor.cs
- MDIWindowDialog.cs
- FrameworkElement.cs
- sqlnorm.cs
- WS2007HttpBindingCollectionElement.cs
- TextInfo.cs
- SessionPageStateSection.cs
- CollectionChangeEventArgs.cs
- TcpClientSocketManager.cs
- TemplatedControlDesigner.cs
- InkCanvasFeedbackAdorner.cs
- XmlTextReaderImplHelpers.cs
- IDQuery.cs
- CancellationToken.cs
- RegexMatchCollection.cs
- SHA384Managed.cs
- ParentQuery.cs
- PointUtil.cs
- HashAlgorithm.cs
- Win32KeyboardDevice.cs
- HtmlInputHidden.cs
- Utils.cs
- FigureParagraph.cs
- GregorianCalendar.cs
- Double.cs
- MailBnfHelper.cs
- RunInstallerAttribute.cs
- listitem.cs
- SafeFileMapViewHandle.cs
- StringUtil.cs
- Win32Exception.cs
- DateTimeFormatInfoScanner.cs
- XmlSchemaCompilationSettings.cs
- FontClient.cs
- AuthenticationService.cs
- DropSource.cs
- Repeater.cs