Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlInputHidden.cs / 1305376 / HtmlInputHidden.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputHidden.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System.ComponentModel; using System; using System.Collections; using System.Collections.Specialized; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ DefaultEvent("ServerChange"), SupportsEventValidation, ] public class HtmlInputHidden : HtmlInputControl, IPostBackDataHandler { private static readonly object EventServerChange = new object(); /* * Creates an intrinsic Html INPUT type=hidden control. */ public HtmlInputHidden() : base("hidden") { } ////// The ///class defines the methods, properties, /// and events of the HtmlInputHidden control. This class allows programmatic access /// to the HTML <input type=hidden> element on the server. /// /// [ WebCategory("Action"), WebSysDescription(SR.HtmlInputHidden_OnServerChange) ] public event EventHandler ServerChange { add { Events.AddHandler(EventServerChange, value); } remove { Events.RemoveHandler(EventServerChange, value); } } /* * Method used to raise the OnServerChange event. */ ////// Occurs when the ///control /// is changed on the server. /// /// protected virtual void OnServerChange(EventArgs e) { EventHandler handler = (EventHandler)Events[EventServerChange]; if (handler != null) handler(this, e); } /* * */ ////// Raised on the server when the ///control /// changes between postback requests. /// /// /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); // if no change handler, no need to save posted property if (!Disabled) { if (Events[EventServerChange] == null) { ViewState.SetItemDirty("value",false); } if (Page != null) { Page.RegisterEnabledControl(this); } } } /* * Method of IPostBackDataHandler interface to process posted data. * InputText process a newly posted value. */ ////// /// bool IPostBackDataHandler.LoadPostData(string postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } protected virtual bool LoadPostData(string postDataKey, NameValueCollection postCollection) { string current = Value; string text = postCollection.GetValues(postDataKey)[0]; if (!current.Equals(text)) { ValidateEvent(postDataKey); Value = text; return true; } return false; } protected override void RenderAttributes(HtmlTextWriter writer) { base.RenderAttributes(writer); if (Page != null) { Page.ClientScript.RegisterForEventValidation(RenderedNameAttribute); } } /* * Method of IPostBackDataHandler interface which is invoked whenever posted data * for a control has changed. TextBox fires an OnTextChanged event. */ ////// /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ////// /// protected virtual void RaisePostDataChangedEvent() { OnServerChange(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
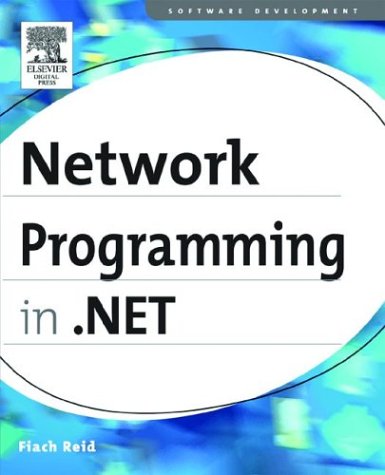
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Constant.cs
- SecurityTokenProvider.cs
- XmlTextWriter.cs
- ArraySortHelper.cs
- BrowserDefinition.cs
- DataBindingList.cs
- WebPartManager.cs
- Documentation.cs
- ApplicationProxyInternal.cs
- CultureTableRecord.cs
- SqlDataSource.cs
- SystemIPv4InterfaceProperties.cs
- DrawingServices.cs
- MenuBase.cs
- ToolZone.cs
- CaseExpr.cs
- NotifyInputEventArgs.cs
- Scheduler.cs
- StringInfo.cs
- SqlVersion.cs
- ConnectionAcceptor.cs
- WindowsListViewGroupSubsetLink.cs
- ErrorFormatterPage.cs
- HideDisabledControlAdapter.cs
- OutOfMemoryException.cs
- ComponentGlyph.cs
- SimpleRecyclingCache.cs
- Int32KeyFrameCollection.cs
- Baml6ConstructorInfo.cs
- ToolStripDropDownButton.cs
- TagPrefixAttribute.cs
- CompilationUnit.cs
- OdbcConnectionString.cs
- TableLayoutCellPaintEventArgs.cs
- TagElement.cs
- WebReference.cs
- SQLDoubleStorage.cs
- MappingItemCollection.cs
- ConfigurationStrings.cs
- SQLBytesStorage.cs
- TemplatePartAttribute.cs
- EntityViewContainer.cs
- Logging.cs
- SiteMembershipCondition.cs
- SeekStoryboard.cs
- WindowProviderWrapper.cs
- AsymmetricSignatureFormatter.cs
- CapabilitiesAssignment.cs
- ArglessEventHandlerProxy.cs
- ListBoxDesigner.cs
- ConfigXmlCDataSection.cs
- DesignerSerializationOptionsAttribute.cs
- BitmapEffectCollection.cs
- FloaterParaClient.cs
- PropertyEmitter.cs
- WriteTimeStream.cs
- XPathScanner.cs
- Char.cs
- AsmxEndpointPickerExtension.cs
- SafeSecurityHelper.cs
- SequenceRangeCollection.cs
- GridViewRowCollection.cs
- AppDomainGrammarProxy.cs
- EasingKeyFrames.cs
- MarshalDirectiveException.cs
- GroupLabel.cs
- CustomAttribute.cs
- AspNetHostingPermission.cs
- UnicastIPAddressInformationCollection.cs
- ObjectAssociationEndMapping.cs
- AnnotationStore.cs
- TypeLoader.cs
- XamlBrushSerializer.cs
- InputElement.cs
- OdbcParameterCollection.cs
- TreeWalkHelper.cs
- DesignTimeValidationFeature.cs
- TextElementEnumerator.cs
- AuthenticationModulesSection.cs
- ToolboxItemAttribute.cs
- NeutralResourcesLanguageAttribute.cs
- SqlNodeAnnotation.cs
- FtpWebRequest.cs
- OdbcConnectionPoolProviderInfo.cs
- HitTestWithPointDrawingContextWalker.cs
- PropertyToken.cs
- DebuggerAttributes.cs
- EditableRegion.cs
- BindingBase.cs
- OpenTypeMethods.cs
- XmlSecureResolver.cs
- AsymmetricSignatureDeformatter.cs
- SecurityContext.cs
- Exceptions.cs
- NeutralResourcesLanguageAttribute.cs
- Geometry.cs
- XmlSchemaGroupRef.cs
- OleServicesContext.cs
- PrinterResolution.cs
- SQLGuid.cs