Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / IO / Zip / WriteTimeStream.cs / 1305600 / WriteTimeStream.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // WriteTimeStream - wraps the ArchiveStream in Streaming generation scenarios so that we // can determine current archive stream offset even when working on a stream that is non-seekable // because the Position property is unusable on such streams. // // History: // 03/25/2002: BruceMac: Created. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Windows; using MS.Internal.WindowsBase; namespace MS.Internal.IO.Zip { internal class WriteTimeStream : Stream { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// CanRead - never /// override public bool CanRead { get { return false; } } ////// CanSeek - never /// override public bool CanSeek{ get { return false; } } ////// CanWrite - only if we are not disposed /// override public bool CanWrite { get { return (_baseStream != null); } } ////// Same as Position /// override public long Length { get { CheckDisposed(); return _position; } } ////// Get is supported even on Write-only stream /// override public long Position { get { CheckDisposed(); return _position; } set { CheckDisposed(); IllegalAccess(); // throw exception } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- public override void SetLength(long newLength) { IllegalAccess(); // throw exception } override public long Seek(long offset, SeekOrigin origin) { IllegalAccess(); // throw exception return -1; // keep compiler happy } override public int Read(byte[] buffer, int offset, int count) { IllegalAccess(); // throw exception return -1; // keep compiler happy } override public void Write(byte[] buffer, int offset, int count) { CheckDisposed(); _baseStream.Write(buffer, offset, count); checked{_position += count;} } override public void Flush() { CheckDisposed(); _baseStream.Flush(); } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ internal WriteTimeStream(Stream baseStream) { if (baseStream == null) { throw new ArgumentNullException("baseStream"); } _baseStream = baseStream; // must be based on writable stream if (!_baseStream.CanWrite) throw new ArgumentException(SR.Get(SRID.WriteNotSupported), "baseStream"); } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ protected override void Dispose(bool disposing) { try { if (disposing && (_baseStream != null)) { _baseStream.Close(); } } finally { _baseStream = null; base.Dispose(disposing); } } //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- private static void IllegalAccess() { throw new NotSupportedException(SR.Get(SRID.WriteOnlyStream)); } private void CheckDisposed() { if (_baseStream == null) throw new ObjectDisposedException("Stream"); } // _baseStream doubles as our disposed indicator - it's null if we are disposed private Stream _baseStream; // stream we wrap - needs to only support Write private long _position; // current position } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
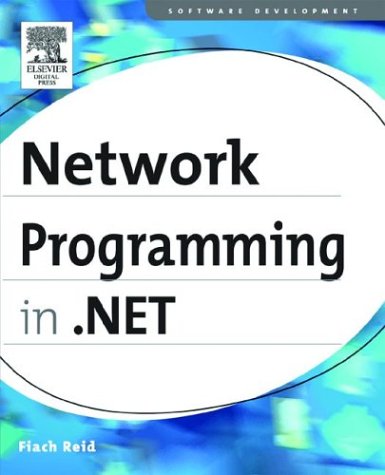
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeGenerator.cs
- ColorAnimationBase.cs
- RuleElement.cs
- TimelineGroup.cs
- SystemParameters.cs
- WebPartEditVerb.cs
- CollectionChangeEventArgs.cs
- FamilyTypeface.cs
- Random.cs
- UnhandledExceptionEventArgs.cs
- TextTreeUndo.cs
- Int64.cs
- IPPacketInformation.cs
- SafeReversePInvokeHandle.cs
- ClientRoleProvider.cs
- ButtonAutomationPeer.cs
- ThreadPool.cs
- MethodRental.cs
- CaseKeyBox.xaml.cs
- Decimal.cs
- DateTimeSerializationSection.cs
- TextParagraph.cs
- UIEndRequest.cs
- BasicHttpMessageSecurity.cs
- Pair.cs
- Monitor.cs
- ManifestResourceInfo.cs
- XmlMembersMapping.cs
- CodeNamespaceImportCollection.cs
- CodeNamespace.cs
- Label.cs
- WriteFileContext.cs
- XamlSerializer.cs
- UnicodeEncoding.cs
- CodeGenerator.cs
- RegisteredScript.cs
- TypeUsageBuilder.cs
- ViewBase.cs
- State.cs
- SmtpMail.cs
- PropertyMapper.cs
- AxisAngleRotation3D.cs
- WindowsToolbarItemAsMenuItem.cs
- EmptyEnumerator.cs
- DescendentsWalker.cs
- ExpressionValueEditor.cs
- Predicate.cs
- GeneralTransform3DTo2DTo3D.cs
- ProtocolsSection.cs
- DataGridViewColumnTypePicker.cs
- ZoneButton.cs
- PrimitiveType.cs
- GridViewCellAutomationPeer.cs
- Touch.cs
- RowToParametersTransformer.cs
- Int32CollectionValueSerializer.cs
- Int32Rect.cs
- HttpPostedFile.cs
- AmbientProperties.cs
- SpeakCompletedEventArgs.cs
- DesignerRegionCollection.cs
- NextPreviousPagerField.cs
- BatchStream.cs
- RequestCacheManager.cs
- IndentTextWriter.cs
- SoundPlayerAction.cs
- DataControlFieldHeaderCell.cs
- InputLanguageCollection.cs
- XPathDocument.cs
- SecurityManager.cs
- _AuthenticationState.cs
- Icon.cs
- CodeBlockBuilder.cs
- IntPtr.cs
- AllMembershipCondition.cs
- XmlIncludeAttribute.cs
- XomlDesignerLoader.cs
- ScriptManagerProxy.cs
- HyperLinkStyle.cs
- InputBindingCollection.cs
- RuleSettings.cs
- BezierSegment.cs
- PasswordRecovery.cs
- IncrementalCompileAnalyzer.cs
- COM2ColorConverter.cs
- DictionarySectionHandler.cs
- LinqDataSourceSelectEventArgs.cs
- WindowsListBox.cs
- ResourceCodeDomSerializer.cs
- SvcMapFileSerializer.cs
- MonthChangedEventArgs.cs
- NullableLongSumAggregationOperator.cs
- XmlUtil.cs
- TreeViewHitTestInfo.cs
- ObjectToIdCache.cs
- baseaxisquery.cs
- TableItemPattern.cs
- IndentTextWriter.cs
- QuadraticBezierSegment.cs
- CalendarButtonAutomationPeer.cs