Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / InputOutput / Registration.cs / 1 / Registration.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file implements registration-related messaging using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.ServiceModel; using System.ServiceModel.Security; using System.ServiceModel.Transactions; using System.Xml; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; using Microsoft.Transactions.Wsat.StateMachines; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; using Fault = Microsoft.Transactions.Wsat.Messaging.Fault; namespace Microsoft.Transactions.Wsat.InputOutput { class RegistrationCoordinator : IRegistrationCoordinator { ProtocolState state; public RegistrationCoordinator (ProtocolState state) { this.state = state; } // // IRegistrationCoordinator // public void Register (Message message, RequestAsyncResult result) { // Unmarshal the message Register register = new Register (message, this.state.ProtocolVersion); EndpointAddress to = register.ParticipantProtocolService; WsatRegistrationHeader registrationHeader = WsatRegistrationHeader.ReadFrom(message); if (registrationHeader == null) { if (DebugTrace.Warning) { DebugTrace.Trace(TraceLevel.Warning, "Rejecting Register message with no registration header"); } this.SendFault(result, this.state.Faults.InvalidParameters); return; } switch (register.Protocol) { case ControlProtocol.Completion: // We only accept a registration for completion if we have an enlistment already TransactionEnlistment enlistment; enlistment = state.Lookup.FindEnlistment(registrationHeader.TransactionId); CompletionEnlistment completion = enlistment as CompletionEnlistment; if (completion == null) { if (DebugTrace.Warning) { DebugTrace.Trace(TraceLevel.Warning, "Rejecting uncorrelated Register message for completion"); } this.SendFault(result, this.state.Faults.UnknownCompletionEnlistment); return; } CompletionParticipantProxy completionProxy = state.TryCreateCompletionParticipantProxy (to); if (completionProxy == null) { if (DebugTrace.Warning) { DebugTrace.Trace(TraceLevel.Warning, "Rejecting Register message for completion on no completion enlistment"); } this.SendFault(result, this.state.Faults.InvalidParameters); return; } try { completion.StateMachine.Enqueue(new MsgRegisterCompletionEvent(completion, ref register, result, completionProxy)); } finally { completionProxy.Release(); } break; case ControlProtocol.Durable2PC: case ControlProtocol.Volatile2PC: // If OutboundTransactions are disabled, we don't accept new participants if (!state.TransactionManager.Settings.NetworkOutboundAccess) { if (DebugTrace.Warning) { DebugTrace.Trace(TraceLevel.Warning, "Rejecting Register message because outbound transactions are disabled"); } this.SendFault(result, this.state.Faults.ParticipantRegistrationNetAccessDisabled); return; } // Check for loopback (i.e., we sent a register message to ourselves) if (register.Loopback == state.ProcessId) { if (DebugTrace.Warning) { DebugTrace.Trace(TraceLevel.Warning, "Rejecting recursive Register message from self"); } this.SendFault(result, this.state.Faults.ParticipantRegistrationLoopback); return; } // Attempt to resolve the proxy TwoPhaseCommitParticipantProxy proxy = state.TryCreateTwoPhaseCommitParticipantProxy (to); if (proxy == null) { if (DebugTrace.Warning) { DebugTrace.Trace(TraceLevel.Warning, "Rejecting Register message because 2PC proxy could not be created"); } this.SendFault(result, this.state.Faults.InvalidParameters); return; } try { // Create a new participant enlistment ParticipantEnlistment participant = new ParticipantEnlistment(state, registrationHeader, register.Protocol, proxy); state.TransactionManagerSend.Register(participant, new MsgRegisterEvent(participant, ref register, result)); } finally { proxy.Release(); } break; default: // An invalid Enum value on this internal code path indicates // a product bug and violates assumptions about // valid values in MSDTC. DiagnosticUtility.FailFast("Registration protocol should have been validated"); break; } } // // Sending messages // public void SendRegisterResponse (TransactionEnlistment enlistment, RequestAsyncResult result, ControlProtocol protocol, EndpointAddress coordinatorService) { RegisterResponse response = new RegisterResponse(this.state.ProtocolVersion); response.CoordinatorProtocolService = coordinatorService; if (DebugTrace.Info) { DebugTrace.TxTrace ( TraceLevel.Info, enlistment.EnlistmentId, "Sending RegisterResponse for {0}", protocol ); } RegistrationProxy.SendRegisterResponse (result, ref response); } public void SendFault (RequestAsyncResult result, Fault fault) { if (DebugTrace.Warning) { DebugTrace.Trace(TraceLevel.Warning, "Sending {0} fault to registration participant", fault.Code.Name); } state.Perf.FaultsSentCountPerInterval.Increment(); RegistrationProxy.SendFaultResponse(result, fault); } } class RegistrationParticipant { ProtocolState state; AsyncCallback sendDurableRegisterComplete; AsyncCallback sendVolatileRegisterComplete; public RegistrationParticipant (ProtocolState state) { this.state = state; this.sendDurableRegisterComplete = DiagnosticUtility.ThunkAsyncCallback(new AsyncCallback(OnSendDurableRegisterComplete)); this.sendVolatileRegisterComplete = DiagnosticUtility.ThunkAsyncCallback(new AsyncCallback(OnSendVolatileRegisterComplete)); } // // Sending messages // void OnSendDurableRegisterComplete (IAsyncResult ar) { if (!ar.CompletedSynchronously) { OnSendRegisterComplete((CoordinatorEnlistment) ar.AsyncState, ControlProtocol.Durable2PC, ar); } } void OnSendVolatileRegisterComplete (IAsyncResult ar) { if (!ar.CompletedSynchronously) { VolatileCoordinatorEnlistment volatileCoordinator = (VolatileCoordinatorEnlistment) ar.AsyncState; OnSendRegisterComplete(volatileCoordinator.Coordinator, ControlProtocol.Volatile2PC, ar); } } void OnSendRegisterComplete(CoordinatorEnlistment coordinator, ControlProtocol protocol, IAsyncResult ar) { SynchronizationEvent newEvent; EndpointAddress coordinatorService = null; try { RegisterResponse response = coordinator.RegistrationProxy.EndSendRegister (ar); coordinatorService = response.CoordinatorProtocolService; TwoPhaseCommitCoordinatorProxy proxy; proxy = state.TryCreateTwoPhaseCommitCoordinatorProxy(coordinatorService); if (proxy == null) { if (RegistrationCoordinatorResponseInvalidMetadataRecord.ShouldTrace) { RegistrationCoordinatorResponseInvalidMetadataRecord.Trace( coordinator.EnlistmentId, coordinator.SuperiorContext, protocol, coordinatorService, null, this.state.ProtocolVersion ); } newEvent = new MsgRegistrationCoordinatorSendFailureEvent(coordinator); } else { try { if (protocol == ControlProtocol.Durable2PC) { newEvent = new MsgRegisterDurableResponseEvent(coordinator, response, proxy); } else { VolatileCoordinatorEnlistment volatileCoordinator = (VolatileCoordinatorEnlistment)ar.AsyncState; newEvent = new MsgRegisterVolatileResponseEvent(volatileCoordinator, response, proxy); } } finally { proxy.Release(); } } } catch (WsatFaultException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); newEvent = new MsgRegistrationCoordinatorFaultEvent (coordinator, protocol, e.Fault); if (RegistrationCoordinatorFaultedRecord.ShouldTrace) { RegistrationCoordinatorFaultedRecord.Trace( coordinator.EnlistmentId, coordinator.SuperiorContext, protocol, e.Fault ); } } catch (WsatMessagingException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); state.Perf.MessageSendFailureCountPerInterval.Increment(); if (RegistrationCoordinatorFailedRecord.ShouldTrace) { RegistrationCoordinatorFailedRecord.Trace( coordinator.EnlistmentId, coordinator.SuperiorContext, protocol, e ); } DebugTrace.TraceSendFailure(coordinator.EnlistmentId, e); newEvent = new MsgRegistrationCoordinatorSendFailureEvent(coordinator); } coordinator.StateMachine.Enqueue (newEvent); } public void SendDurableRegister(CoordinatorEnlistment coordinator) { SendRegister (coordinator, ControlProtocol.Durable2PC, coordinator.ParticipantService, this.sendDurableRegisterComplete, coordinator); } public void SendVolatileRegister(VolatileCoordinatorEnlistment volatileCoordinator) { SendRegister (volatileCoordinator.Coordinator, ControlProtocol.Volatile2PC, volatileCoordinator.ParticipantService, this.sendVolatileRegisterComplete, volatileCoordinator); } void SendRegister(CoordinatorEnlistment coordinator, ControlProtocol protocol, EndpointAddress protocolService, AsyncCallback callback, object callbackState) { Register register = new Register(this.state.ProtocolVersion); register.Protocol = protocol; register.Loopback = state.ProcessId; register.ParticipantProtocolService = protocolService; register.SupportingToken = coordinator.SuperiorIssuedToken; if (DebugTrace.Info) { DebugTrace.TxTrace ( TraceLevel.Info, coordinator.EnlistmentId, "Sending Register for {0} to {1}", protocol, Ports.TryGetAddress (coordinator.RegistrationProxy)); } IAsyncResult ar = coordinator.RegistrationProxy.BeginSendRegister (ref register, callback, callbackState); if (ar.CompletedSynchronously) { OnSendRegisterComplete(coordinator, protocol, ar); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
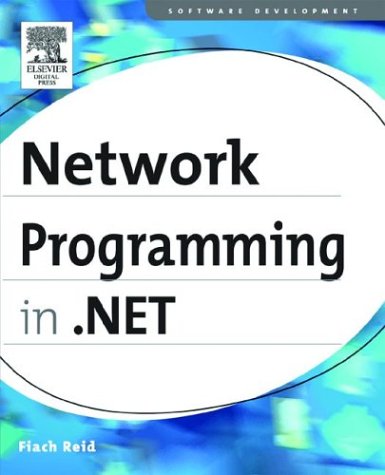
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- hebrewshape.cs
- HebrewNumber.cs
- RefreshEventArgs.cs
- ApplicationSecurityManager.cs
- MultipartContentParser.cs
- ToolStripContainer.cs
- InputMethodStateChangeEventArgs.cs
- ParseChildrenAsPropertiesAttribute.cs
- Model3DCollection.cs
- RoleGroup.cs
- WebBrowserHelper.cs
- relpropertyhelper.cs
- InputQueue.cs
- DispatcherObject.cs
- Hash.cs
- AssemblyFilter.cs
- Activator.cs
- ServicePoint.cs
- HelloOperationAsyncResult.cs
- HttpResponseWrapper.cs
- TreeViewAutomationPeer.cs
- WorkerRequest.cs
- DefaultObjectMappingItemCollection.cs
- BinaryFormatterWriter.cs
- UnaryExpression.cs
- TimeEnumHelper.cs
- ActiveXContainer.cs
- _OverlappedAsyncResult.cs
- SqlExpressionNullability.cs
- ContainsRowNumberChecker.cs
- SqlPersonalizationProvider.cs
- HyperLink.cs
- DataRowCollection.cs
- AdapterUtil.cs
- StrokeNodeEnumerator.cs
- ApplicationSecurityManager.cs
- PlanCompilerUtil.cs
- XhtmlTextWriter.cs
- DeviceContext.cs
- ExpandCollapsePattern.cs
- MDIWindowDialog.cs
- ByteStack.cs
- RichTextBoxAutomationPeer.cs
- BitmapImage.cs
- RowTypePropertyElement.cs
- BaseCodeDomTreeGenerator.cs
- GZipStream.cs
- XamlInt32CollectionSerializer.cs
- Effect.cs
- ArgIterator.cs
- AttachedProperty.cs
- WebPartTransformer.cs
- AdapterSwitches.cs
- SqlDataSource.cs
- PresentationAppDomainManager.cs
- XmlTextAttribute.cs
- BitmapInitialize.cs
- XmlWrappingReader.cs
- XPathNavigator.cs
- EntityObject.cs
- FrameworkElement.cs
- HostedNamedPipeTransportManager.cs
- BindingCompleteEventArgs.cs
- GridErrorDlg.cs
- TypeSchema.cs
- InternalCache.cs
- SystemColors.cs
- PopOutPanel.cs
- RedirectionProxy.cs
- ByteStreamMessageEncodingElement.cs
- SimpleMailWebEventProvider.cs
- Misc.cs
- CompiledQuery.cs
- AnimationClock.cs
- DbConnectionFactory.cs
- _AutoWebProxyScriptWrapper.cs
- DesignerActionKeyboardBehavior.cs
- ErrorView.xaml.cs
- DateTimeFormat.cs
- ExpressionBinding.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- SqlNodeTypeOperators.cs
- UnhandledExceptionEventArgs.cs
- DataViewSettingCollection.cs
- InheritanceRules.cs
- SchemaComplexType.cs
- CFStream.cs
- OleDbReferenceCollection.cs
- TextDataBindingHandler.cs
- DataTableNewRowEvent.cs
- CollectionView.cs
- CalendarDateChangedEventArgs.cs
- RoleService.cs
- FormViewDeleteEventArgs.cs
- Stack.cs
- EastAsianLunisolarCalendar.cs
- DialogDivider.cs
- ThaiBuddhistCalendar.cs
- Parallel.cs
- CategoryState.cs