Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DLinq / Dlinq / SqlClient / Common / SqlNodeTypeOperators.cs / 1305376 / SqlNodeTypeOperators.cs
using System; using System.Collections.Generic; using System.Text; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq.SqlClient { internal static class SqlNodeTypeOperators { ////// Determines whether the given unary operator node type returns a value that /// is predicate. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateUnaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: return true; case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Convert: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given unary operator expects a predicate as input. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsUnaryOperatorExpectingPredicateOperand(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: return true; case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Convert: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateBinaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether this operator is a binary comparison operator (i.e. >, =>, ==, etc) /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification = "These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsComparisonOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: return true; case SqlNodeType.And: case SqlNodeType.Or: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsBinaryOperatorExpectingPredicateOperands(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.EQ: case SqlNodeType.EQ2V: case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.NE: case SqlNodeType.NE2V: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given node requires support on the client for evaluation. /// For example, LINK nodes may be delay-executed only when the user requests the result. /// internal static bool IsClientAidedExpression(this SqlExpression expr) { switch (expr.NodeType) { case SqlNodeType.Link: case SqlNodeType.Element: case SqlNodeType.Multiset: case SqlNodeType.ClientQuery: case SqlNodeType.TypeCase: case SqlNodeType.New: return true; default: return false; }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Text; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq.SqlClient { internal static class SqlNodeTypeOperators { ////// Determines whether the given unary operator node type returns a value that /// is predicate. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateUnaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: return true; case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Convert: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given unary operator expects a predicate as input. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsUnaryOperatorExpectingPredicateOperand(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.Not: case SqlNodeType.Not2V: return true; case SqlNodeType.IsNull: case SqlNodeType.IsNotNull: case SqlNodeType.Negate: case SqlNodeType.BitNot: case SqlNodeType.Count: case SqlNodeType.LongCount: case SqlNodeType.Max: case SqlNodeType.Min: case SqlNodeType.Sum: case SqlNodeType.Avg: case SqlNodeType.Stddev: case SqlNodeType.Convert: case SqlNodeType.ValueOf: case SqlNodeType.OuterJoinedValue: case SqlNodeType.ClrLength: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsPredicateBinaryOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether this operator is a binary comparison operator (i.e. >, =>, ==, etc) /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification = "These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsComparisonOperator(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.EQ: case SqlNodeType.NE: case SqlNodeType.EQ2V: case SqlNodeType.NE2V: return true; case SqlNodeType.And: case SqlNodeType.Or: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given binary operator node type returns a value that /// is a predicate boolean. /// [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification="These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] internal static bool IsBinaryOperatorExpectingPredicateOperands(this SqlNodeType nodeType) { switch (nodeType) { case SqlNodeType.And: case SqlNodeType.Or: return true; case SqlNodeType.EQ: case SqlNodeType.EQ2V: case SqlNodeType.GE: case SqlNodeType.GT: case SqlNodeType.LE: case SqlNodeType.LT: case SqlNodeType.NE: case SqlNodeType.NE2V: case SqlNodeType.Add: case SqlNodeType.Sub: case SqlNodeType.Mul: case SqlNodeType.Div: case SqlNodeType.Mod: case SqlNodeType.BitAnd: case SqlNodeType.BitOr: case SqlNodeType.BitXor: case SqlNodeType.Concat: case SqlNodeType.Coalesce: return false; default: throw Error.UnexpectedNode(nodeType); } } ////// Determines whether the given node requires support on the client for evaluation. /// For example, LINK nodes may be delay-executed only when the user requests the result. /// internal static bool IsClientAidedExpression(this SqlExpression expr) { switch (expr.NodeType) { case SqlNodeType.Link: case SqlNodeType.Element: case SqlNodeType.Multiset: case SqlNodeType.ClientQuery: case SqlNodeType.TypeCase: case SqlNodeType.New: return true; default: return false; }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
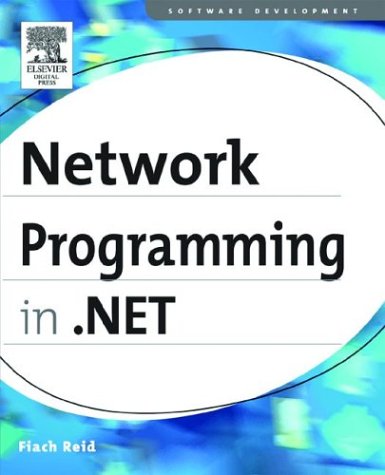
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BindableTemplateBuilder.cs
- FileEnumerator.cs
- WindowsHyperlink.cs
- BmpBitmapDecoder.cs
- ObjectItemNoOpAssemblyLoader.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- LayoutTable.cs
- MobileUserControlDesigner.cs
- URI.cs
- ISAPIApplicationHost.cs
- httpserverutility.cs
- ConsoleCancelEventArgs.cs
- TypedReference.cs
- CompositeFontFamily.cs
- XmlUtilWriter.cs
- PrintEvent.cs
- AttributeXamlType.cs
- PreDigestedSignedInfo.cs
- ExcCanonicalXml.cs
- MobileUserControl.cs
- AddingNewEventArgs.cs
- TextBoxBase.cs
- SettingsPropertyNotFoundException.cs
- KnownBoxes.cs
- HitTestParameters.cs
- ContentTextAutomationPeer.cs
- OdbcInfoMessageEvent.cs
- ObjectView.cs
- StrokeSerializer.cs
- TypeGeneratedEventArgs.cs
- JoinCqlBlock.cs
- GPPOINT.cs
- TextContainerChangedEventArgs.cs
- TypeHelpers.cs
- StatusStrip.cs
- CodeMemberEvent.cs
- TextParagraphProperties.cs
- FilteredAttributeCollection.cs
- ModelFunctionTypeElement.cs
- ImageMetadata.cs
- baseshape.cs
- PageBreakRecord.cs
- GPPOINT.cs
- WindowsFormsHostPropertyMap.cs
- OpenTypeLayout.cs
- SecurityManager.cs
- Win32Interop.cs
- ProcessThread.cs
- SqlUserDefinedTypeAttribute.cs
- BooleanToVisibilityConverter.cs
- SingleSelectRootGridEntry.cs
- RC2.cs
- Decorator.cs
- ScriptIgnoreAttribute.cs
- RsaKeyGen.cs
- X509Utils.cs
- DashStyle.cs
- ErrorActivity.cs
- HtmlHistory.cs
- AlternateView.cs
- DebuggerAttributes.cs
- FileInfo.cs
- ValidatorCompatibilityHelper.cs
- HandlerFactoryWrapper.cs
- ImageAttributes.cs
- TraceData.cs
- MultiDataTrigger.cs
- Screen.cs
- XmlHierarchyData.cs
- ChangeBlockUndoRecord.cs
- WindowsSolidBrush.cs
- ListViewDesigner.cs
- Point3DCollectionConverter.cs
- HttpProcessUtility.cs
- StringUtil.cs
- listitem.cs
- CheckBoxBaseAdapter.cs
- HwndStylusInputProvider.cs
- StringUtil.cs
- SqlInfoMessageEvent.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- StaticSiteMapProvider.cs
- figurelengthconverter.cs
- SoapSchemaImporter.cs
- WebConfigurationHost.cs
- InsufficientMemoryException.cs
- CustomPeerResolverService.cs
- XmlNamespaceDeclarationsAttribute.cs
- FileController.cs
- AddingNewEventArgs.cs
- WebProxyScriptElement.cs
- RecognizedAudio.cs
- OptimalBreakSession.cs
- WebServiceHandler.cs
- BatchStream.cs
- DataSetSchema.cs
- LeftCellWrapper.cs
- VirtualPathProvider.cs
- DataGridTable.cs
- FrameworkContentElement.cs