Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / FloatSumAggregationOperator.cs / 1305376 / FloatSumAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // FloatSumAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for floats. /// internal sealed class FloatSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal FloatSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override float InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. double sum = 0.0; while (enumerator.MoveNext()) { checked { sum += enumerator.Current; } } return (float)sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new FloatSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class FloatSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal FloatSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref double currentElement) { float element = default(float); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. double tempSum = 0.0f; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); tempSum += element; } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = tempSum; return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // FloatSumAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for floats. /// internal sealed class FloatSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal FloatSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override float InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. double sum = 0.0; while (enumerator.MoveNext()) { checked { sum += enumerator.Current; } } return (float)sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new FloatSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class FloatSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal FloatSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref double currentElement) { float element = default(float); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. double tempSum = 0.0f; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); tempSum += element; } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = tempSum; return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
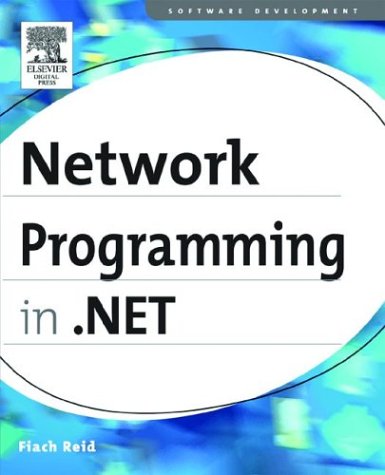
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Pen.cs
- ContextInformation.cs
- GeneralTransform3D.cs
- SqlFlattener.cs
- COM2ICategorizePropertiesHandler.cs
- XsltCompileContext.cs
- ObjectFullSpanRewriter.cs
- ExpressionBuilderCollection.cs
- EastAsianLunisolarCalendar.cs
- CultureInfo.cs
- ProjectionPathBuilder.cs
- EncoderFallback.cs
- TypeElementCollection.cs
- SiteMapNodeCollection.cs
- VisualStyleInformation.cs
- ProfileProvider.cs
- TypeUtil.cs
- MDIWindowDialog.cs
- Misc.cs
- SatelliteContractVersionAttribute.cs
- StringUtil.cs
- StrokeCollectionConverter.cs
- OverrideMode.cs
- XslAst.cs
- MemberMemberBinding.cs
- DependencyPropertyDescriptor.cs
- PathData.cs
- IQueryable.cs
- VirtualPathProvider.cs
- LogLogRecordHeader.cs
- Page.cs
- ColorContextHelper.cs
- ListViewAutomationPeer.cs
- AttributeQuery.cs
- _FixedSizeReader.cs
- ApplicationServiceManager.cs
- DataGridViewColumnEventArgs.cs
- MenuItemBindingCollection.cs
- OperatingSystem.cs
- AudioFileOut.cs
- Timer.cs
- CodeGotoStatement.cs
- ToolStripKeyboardHandlingService.cs
- EntityDataSourceReferenceGroup.cs
- _BasicClient.cs
- Selection.cs
- ProtocolReflector.cs
- SudsWriter.cs
- AppDomainAttributes.cs
- RemoteCryptoRsaServiceProvider.cs
- MenuBindingsEditorForm.cs
- SerializerWriterEventHandlers.cs
- TransformConverter.cs
- DiscoveryClientBindingElement.cs
- SqlResolver.cs
- EntitySetDataBindingList.cs
- HTMLTagNameToTypeMapper.cs
- TriggerActionCollection.cs
- DbFunctionCommandTree.cs
- RestHandler.cs
- Rect3DValueSerializer.cs
- ColumnCollection.cs
- DecimalAnimation.cs
- FormViewUpdatedEventArgs.cs
- XPathExpr.cs
- BuildResult.cs
- ObjectTypeMapping.cs
- BitmapEncoder.cs
- PtsCache.cs
- WindowsSysHeader.cs
- EventSourceCreationData.cs
- DbConnectionPoolOptions.cs
- SelectionEditingBehavior.cs
- AsyncOperationManager.cs
- FirstQueryOperator.cs
- DependencyPropertyHelper.cs
- RuleSetBrowserDialog.cs
- SelectedCellsChangedEventArgs.cs
- TransformPatternIdentifiers.cs
- DescendentsWalker.cs
- ProfileModule.cs
- UriTemplateMatchException.cs
- BoolExpressionVisitors.cs
- Compiler.cs
- SchemaNamespaceManager.cs
- SoundPlayer.cs
- WebPartCloseVerb.cs
- EventListenerClientSide.cs
- ConfigurationManagerHelper.cs
- PageThemeCodeDomTreeGenerator.cs
- Timer.cs
- EditorPart.cs
- Soap.cs
- EmbeddedObject.cs
- BinaryObjectReader.cs
- RegexRunner.cs
- Form.cs
- HtmlElement.cs
- WebPartConnectionsCloseVerb.cs
- XmlNavigatorStack.cs