Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / PtsHost / EmbeddedObject.cs / 1 / EmbeddedObject.cs
// // Copyright (C) Microsoft Corporation. All rights reserved. // // File: EmbeddedObject.cs // // Description: Definition for embedded object inside a text paragraph. // // History: // 05/05/2003 : grzegorz - moving from Avalon branch. // //---------------------------------------------------------------------------- using System; using System.Windows; using MS.Internal.Documents; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// EmbeddedObject class stores information about object embedded within /// a text paragraph. /// internal abstract class EmbeddedObject { ////// Constructor. /// /// /// Embedded object's character position. /// protected EmbeddedObject(int dcp) { Dcp = dcp; } ////// Dispose object. /// internal virtual void Dispose() { } ////// Update object using date from another embedded object of the same /// type. /// /// /// Source of updated data. /// internal abstract void Update(EmbeddedObject newObject); ////// Embedded object's owner. /// internal abstract DependencyObject Element { get; } ////// Position within a text paragraph (number of characters from the /// beginning of text paragraph). /// internal int Dcp; } ////// Stores information about attached object embedded within a text paragraph. /// internal class AttachedObject : EmbeddedObject { ////// Constructor. /// /// /// Attached object's character position. /// /// /// Paragraph associated with attached object. /// internal AttachedObject(int dcp, BaseParagraph para) : base(dcp) { Para = para; } ////// Dispose object. /// internal override void Dispose() { Para.Dispose(); Para = null; base.Dispose(); } ////// Update object using date from another attached object of the same /// type. /// /// /// Source of updated data. /// internal override void Update(EmbeddedObject newObject) { AttachedObject newAttachedObject = newObject as AttachedObject; ErrorHandler.Assert(newAttachedObject != null, ErrorHandler.EmbeddedObjectTypeMismatch); ErrorHandler.Assert(newAttachedObject.Element.Equals(Element), ErrorHandler.EmbeddedObjectOwnerMismatch); Dcp = newAttachedObject.Dcp; Para.SetUpdateInfo(PTS.FSKCHANGE.fskchInside, false); } ////// Attached object's owner. /// internal override DependencyObject Element { get { return Para.Element; } } ////// Paragraph associated with attached object. /// internal BaseParagraph Para; } ////// Stores information about inline object embedded within a text line. /// internal sealed class InlineObject : EmbeddedObject { ////// Constructor. /// /// /// Embedded object's character position. /// /// /// UIElementIsland associated with embedded object. /// /// /// TextParagraph associated with embedded object. /// internal InlineObject(int dcp, UIElementIsland uiElementIsland, TextParagraph para) : base(dcp) { _para = para; _uiElementIsland = uiElementIsland; _uiElementIsland.DesiredSizeChanged += new DesiredSizeChangedEventHandler(_para.OnUIElementDesiredSizeChanged); } ////// Dispose object. /// internal override void Dispose() { if (_uiElementIsland != null) { _uiElementIsland.DesiredSizeChanged -= new DesiredSizeChangedEventHandler(_para.OnUIElementDesiredSizeChanged); } base.Dispose(); } ////// Update object using date from another embedded object of the same /// type. /// /// /// Source of updated data. /// internal override void Update(EmbeddedObject newObject) { // These should definitely be the same InlineObject newInline = newObject as InlineObject; ErrorHandler.Assert(newInline != null, ErrorHandler.EmbeddedObjectTypeMismatch); ErrorHandler.Assert(newInline._uiElementIsland == _uiElementIsland, ErrorHandler.EmbeddedObjectOwnerMismatch); // We've ensured ownership is still with the old InlineObject, so we now null this out to prevent dispose from disconnecting. newInline._uiElementIsland = null; } ////// Embedded object's owner. /// internal override DependencyObject Element { get { return _uiElementIsland.Root; } } private UIElementIsland _uiElementIsland; private TextParagraph _para; } ////// Stores information about figure embedded within a text paragraph. /// internal sealed class FigureObject : AttachedObject { ////// Constructor. /// /// /// Figure object's character position. /// /// /// Paragraph associated with figure object. /// internal FigureObject(int dcp, FigureParagraph para) : base(dcp, para) { } } ////// Stores information about floater embedded within a text paragraph. /// internal sealed class FloaterObject : AttachedObject { ////// Constructor. /// /// /// Floater object's character position. /// /// /// Paragraph associated with floater object. /// internal FloaterObject(int dcp, FloaterParagraph para) : base(dcp, para) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: EmbeddedObject.cs // // Description: Definition for embedded object inside a text paragraph. // // History: // 05/05/2003 : grzegorz - moving from Avalon branch. // //---------------------------------------------------------------------------- using System; using System.Windows; using MS.Internal.Documents; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// EmbeddedObject class stores information about object embedded within /// a text paragraph. /// internal abstract class EmbeddedObject { ////// Constructor. /// /// /// Embedded object's character position. /// protected EmbeddedObject(int dcp) { Dcp = dcp; } ////// Dispose object. /// internal virtual void Dispose() { } ////// Update object using date from another embedded object of the same /// type. /// /// /// Source of updated data. /// internal abstract void Update(EmbeddedObject newObject); ////// Embedded object's owner. /// internal abstract DependencyObject Element { get; } ////// Position within a text paragraph (number of characters from the /// beginning of text paragraph). /// internal int Dcp; } ////// Stores information about attached object embedded within a text paragraph. /// internal class AttachedObject : EmbeddedObject { ////// Constructor. /// /// /// Attached object's character position. /// /// /// Paragraph associated with attached object. /// internal AttachedObject(int dcp, BaseParagraph para) : base(dcp) { Para = para; } ////// Dispose object. /// internal override void Dispose() { Para.Dispose(); Para = null; base.Dispose(); } ////// Update object using date from another attached object of the same /// type. /// /// /// Source of updated data. /// internal override void Update(EmbeddedObject newObject) { AttachedObject newAttachedObject = newObject as AttachedObject; ErrorHandler.Assert(newAttachedObject != null, ErrorHandler.EmbeddedObjectTypeMismatch); ErrorHandler.Assert(newAttachedObject.Element.Equals(Element), ErrorHandler.EmbeddedObjectOwnerMismatch); Dcp = newAttachedObject.Dcp; Para.SetUpdateInfo(PTS.FSKCHANGE.fskchInside, false); } ////// Attached object's owner. /// internal override DependencyObject Element { get { return Para.Element; } } ////// Paragraph associated with attached object. /// internal BaseParagraph Para; } ////// Stores information about inline object embedded within a text line. /// internal sealed class InlineObject : EmbeddedObject { ////// Constructor. /// /// /// Embedded object's character position. /// /// /// UIElementIsland associated with embedded object. /// /// /// TextParagraph associated with embedded object. /// internal InlineObject(int dcp, UIElementIsland uiElementIsland, TextParagraph para) : base(dcp) { _para = para; _uiElementIsland = uiElementIsland; _uiElementIsland.DesiredSizeChanged += new DesiredSizeChangedEventHandler(_para.OnUIElementDesiredSizeChanged); } ////// Dispose object. /// internal override void Dispose() { if (_uiElementIsland != null) { _uiElementIsland.DesiredSizeChanged -= new DesiredSizeChangedEventHandler(_para.OnUIElementDesiredSizeChanged); } base.Dispose(); } ////// Update object using date from another embedded object of the same /// type. /// /// /// Source of updated data. /// internal override void Update(EmbeddedObject newObject) { // These should definitely be the same InlineObject newInline = newObject as InlineObject; ErrorHandler.Assert(newInline != null, ErrorHandler.EmbeddedObjectTypeMismatch); ErrorHandler.Assert(newInline._uiElementIsland == _uiElementIsland, ErrorHandler.EmbeddedObjectOwnerMismatch); // We've ensured ownership is still with the old InlineObject, so we now null this out to prevent dispose from disconnecting. newInline._uiElementIsland = null; } ////// Embedded object's owner. /// internal override DependencyObject Element { get { return _uiElementIsland.Root; } } private UIElementIsland _uiElementIsland; private TextParagraph _para; } ////// Stores information about figure embedded within a text paragraph. /// internal sealed class FigureObject : AttachedObject { ////// Constructor. /// /// /// Figure object's character position. /// /// /// Paragraph associated with figure object. /// internal FigureObject(int dcp, FigureParagraph para) : base(dcp, para) { } } ////// Stores information about floater embedded within a text paragraph. /// internal sealed class FloaterObject : AttachedObject { ////// Constructor. /// /// /// Floater object's character position. /// /// /// Paragraph associated with floater object. /// internal FloaterObject(int dcp, FloaterParagraph para) : base(dcp, para) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
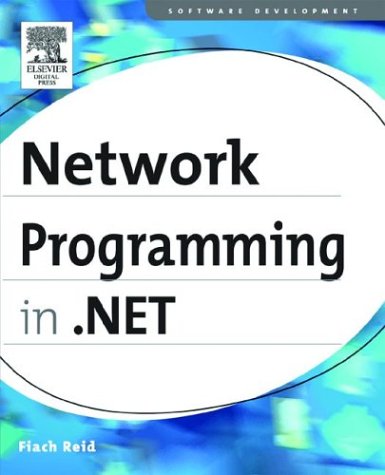
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- controlskin.cs
- MenuItemBinding.cs
- JsonQNameDataContract.cs
- TypeUtil.cs
- LineBreakRecord.cs
- MulticastIPAddressInformationCollection.cs
- UniqueIdentifierService.cs
- ScriptingProfileServiceSection.cs
- ModulesEntry.cs
- ApplicationContext.cs
- TargetException.cs
- MailWriter.cs
- OrderablePartitioner.cs
- BitmapEffectDrawing.cs
- NoneExcludedImageIndexConverter.cs
- nulltextnavigator.cs
- MinimizableAttributeTypeConverter.cs
- QueryRewriter.cs
- ResolveDuplex11AsyncResult.cs
- TextWriterTraceListener.cs
- ServerValidateEventArgs.cs
- XPathNodePointer.cs
- XslTransform.cs
- DataListItemCollection.cs
- DataGridViewDataConnection.cs
- MultiTrigger.cs
- HasCopySemanticsAttribute.cs
- KeyValuePairs.cs
- VisualBasicValue.cs
- MultipleViewPattern.cs
- XPathParser.cs
- InstanceHandleConflictException.cs
- PerformanceCounterPermissionEntry.cs
- StrokeCollection2.cs
- XmlReflectionImporter.cs
- Span.cs
- PropertyGroupDescription.cs
- ModelUIElement3D.cs
- MaterialGroup.cs
- Classification.cs
- XmlSchemaAttributeGroup.cs
- MultiAsyncResult.cs
- Helpers.cs
- LogExtent.cs
- StorageModelBuildProvider.cs
- RootProfilePropertySettingsCollection.cs
- UIntPtr.cs
- InvalidOleVariantTypeException.cs
- DbQueryCommandTree.cs
- TableLayoutRowStyleCollection.cs
- SerializerWriterEventHandlers.cs
- UniqueEventHelper.cs
- DataGridViewTopLeftHeaderCell.cs
- ListViewInsertEventArgs.cs
- UnwrappedTypesXmlSerializerManager.cs
- IDispatchConstantAttribute.cs
- TdsParserHelperClasses.cs
- UInt64Converter.cs
- DataGridViewComboBoxEditingControl.cs
- ClientUtils.cs
- FontFamily.cs
- AppSettingsReader.cs
- WindowsComboBox.cs
- WindowsTooltip.cs
- AssertFilter.cs
- WindowsServiceElement.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- XmlWriterTraceListener.cs
- TraceLevelStore.cs
- FixedTextBuilder.cs
- dataprotectionpermission.cs
- AccessDataSource.cs
- DeflateStream.cs
- SignatureHelper.cs
- RectValueSerializer.cs
- sqlnorm.cs
- StorageEntityTypeMapping.cs
- CommandPlan.cs
- PointAnimationClockResource.cs
- Keywords.cs
- ObjectViewEntityCollectionData.cs
- QueryContinueDragEventArgs.cs
- EmulateRecognizeCompletedEventArgs.cs
- SoapReflectionImporter.cs
- SemanticBasicElement.cs
- LicenseManager.cs
- AbsoluteQuery.cs
- UIElementHelper.cs
- UnsafeNativeMethodsPenimc.cs
- WebPartDescription.cs
- CheckBox.cs
- StylusPointProperty.cs
- WebBrowserEvent.cs
- rsa.cs
- ProtocolElementCollection.cs
- DoubleCollectionConverter.cs
- PerformanceCounterManager.cs
- ProfileSettings.cs
- CheckBox.cs
- CompilerScope.cs