Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media3D / ModelUIElement3D.cs / 1 / ModelUIElement3D.cs
//---------------------------------------------------------------------------- // //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // // History: // 4/12/2007: kurtb - Created // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Media; using MS.Internal.Media3D; using System; using System.Diagnostics; using System.Collections.Specialized; using System.ComponentModel; using System.Windows.Automation.Peers; using System.Windows.Media.Composition; using System.Windows.Markup; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// ModelUIElement3D is a UIElement3D which draws the given Model3D. /// ModelUIElement3D is usable from Xaml. /// [ContentProperty("Model")] public sealed class ModelUIElement3D : UIElement3D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Default ctor /// public ModelUIElement3D() { } #endregion Constructors ////// DependencyProperty which backs the ModelUIElement3D.Content property. /// public static readonly DependencyProperty ModelProperty = DependencyProperty.Register( "Model", /* propertyType = */ typeof(Model3D), /* ownerType = */ typeof(ModelUIElement3D), new PropertyMetadata(ModelPropertyChanged), (ValidateValueCallback) delegate { return MediaContext.CurrentMediaContext.WriteAccessEnabled; }); private static void ModelPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ModelUIElement3D owner = ((ModelUIElement3D) d); // if it's not a subproperty change, then we need to change the protected Model property of Visual3D if (!e.IsASubPropertyChange) { owner.Visual3DModel = (Model3D)e.NewValue; } } ////// The Model3D to render /// public Model3D Model { get { return (Model3D) GetValue(ModelProperty); } set { SetValue(ModelProperty, value); } } ////// Called by the Automation infrastructure when AutomationPeer /// is requested for this element. /// protected override AutomationPeer OnCreateAutomationPeer() { return new UIElement3DAutomationPeer(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // // History: // 4/12/2007: kurtb - Created // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Media; using MS.Internal.Media3D; using System; using System.Diagnostics; using System.Collections.Specialized; using System.ComponentModel; using System.Windows.Automation.Peers; using System.Windows.Media.Composition; using System.Windows.Markup; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// ModelUIElement3D is a UIElement3D which draws the given Model3D. /// ModelUIElement3D is usable from Xaml. /// [ContentProperty("Model")] public sealed class ModelUIElement3D : UIElement3D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Default ctor /// public ModelUIElement3D() { } #endregion Constructors ////// DependencyProperty which backs the ModelUIElement3D.Content property. /// public static readonly DependencyProperty ModelProperty = DependencyProperty.Register( "Model", /* propertyType = */ typeof(Model3D), /* ownerType = */ typeof(ModelUIElement3D), new PropertyMetadata(ModelPropertyChanged), (ValidateValueCallback) delegate { return MediaContext.CurrentMediaContext.WriteAccessEnabled; }); private static void ModelPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ModelUIElement3D owner = ((ModelUIElement3D) d); // if it's not a subproperty change, then we need to change the protected Model property of Visual3D if (!e.IsASubPropertyChange) { owner.Visual3DModel = (Model3D)e.NewValue; } } ////// The Model3D to render /// public Model3D Model { get { return (Model3D) GetValue(ModelProperty); } set { SetValue(ModelProperty, value); } } ////// Called by the Automation infrastructure when AutomationPeer /// is requested for this element. /// protected override AutomationPeer OnCreateAutomationPeer() { return new UIElement3DAutomationPeer(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
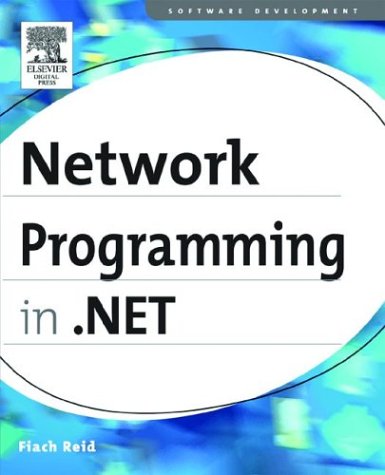
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DirectoryObjectSecurity.cs
- Point4D.cs
- HttpModule.cs
- ManifestSignatureInformation.cs
- NativeMethods.cs
- OAVariantLib.cs
- DataControlCommands.cs
- EntityDesignerDataSourceView.cs
- RectValueSerializer.cs
- GridSplitterAutomationPeer.cs
- SafeNativeMethods.cs
- BitmapEffectCollection.cs
- UnhandledExceptionEventArgs.cs
- SystemInformation.cs
- EncryptedType.cs
- LinkButton.cs
- ArgumentOutOfRangeException.cs
- DetailsViewInsertEventArgs.cs
- NonVisualControlAttribute.cs
- PersistenceProviderBehavior.cs
- RequestCachingSection.cs
- Scheduling.cs
- EntitySet.cs
- PrintController.cs
- BaseProcessor.cs
- QilTernary.cs
- XPathNavigatorException.cs
- DependencyPropertyConverter.cs
- TreeViewItem.cs
- ExpandSegmentCollection.cs
- BuildProviderAppliesToAttribute.cs
- LoginName.cs
- DependencyObjectProvider.cs
- WindowsListViewItemStartMenu.cs
- SizeAnimationBase.cs
- ConfigurationSchemaErrors.cs
- SqlDelegatedTransaction.cs
- DataRecordInternal.cs
- WmlLinkAdapter.cs
- ConfigurationManagerInternalFactory.cs
- PaperSize.cs
- AppDomainAttributes.cs
- RelatedPropertyManager.cs
- BasicHttpBindingElement.cs
- ConfigurationValues.cs
- StorageTypeMapping.cs
- XmlLanguageConverter.cs
- CompilerHelpers.cs
- FaultDesigner.cs
- AdRotatorDesigner.cs
- VisualBrush.cs
- milexports.cs
- XmlBindingWorker.cs
- WebPartDisplayModeEventArgs.cs
- DescendentsWalkerBase.cs
- HtmlUtf8RawTextWriter.cs
- AppLevelCompilationSectionCache.cs
- DependencyObjectProvider.cs
- Msec.cs
- CapacityStreamGeometryContext.cs
- BitmapMetadataEnumerator.cs
- GeometryHitTestResult.cs
- BehaviorEditorPart.cs
- Rules.cs
- WebSysDescriptionAttribute.cs
- WSHttpTransportSecurityElement.cs
- ReferenceEqualityComparer.cs
- ClientReliableChannelBinder.cs
- ConfigXmlAttribute.cs
- Vector3DAnimation.cs
- SqlDataSourceFilteringEventArgs.cs
- NameTable.cs
- EmptyEnumerator.cs
- SQLCharsStorage.cs
- KeyValueConfigurationElement.cs
- CodeTypeReference.cs
- PresentationTraceSources.cs
- Update.cs
- ContextDataSourceView.cs
- DiscoveryClientDocuments.cs
- ListControlActionList.cs
- DelegatedStream.cs
- UpdateEventArgs.cs
- XmlSerializer.cs
- RtfToken.cs
- BoundPropertyEntry.cs
- GcHandle.cs
- NativeRightsManagementAPIsStructures.cs
- localization.cs
- EventMappingSettingsCollection.cs
- ErrorWrapper.cs
- StrongNameMembershipCondition.cs
- PrtCap_Public.cs
- WindowsListBox.cs
- ListViewItem.cs
- StylusButton.cs
- RuleAction.cs
- documentsequencetextpointer.cs
- XmlIncludeAttribute.cs
- CultureData.cs