Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Configuration / System / Configuration / ConfigurationSchemaErrors.cs / 1305376 / ConfigurationSchemaErrors.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Text; internal class ConfigurationSchemaErrors { // Errors with ExceptionAction.Local are logged to this list. // This list is reset when processing of a section is complete. // Errors on this list may be added to the _errorsAll list // when RetrieveAndResetLocalErrors is called. private List_errorsLocal; // Errors with ExceptionAction.Global are logged to this list. private List _errorsGlobal; // All errors related to a config file are logged to this list. // This includes all global errors, all non-specific errors, // and local errors for input that applies to this config file. private List _errorsAll; internal ConfigurationSchemaErrors() {} internal bool HasLocalErrors { get { return ErrorsHelper.GetHasErrors(_errorsLocal); } } internal bool HasGlobalErrors { get { return ErrorsHelper.GetHasErrors(_errorsGlobal); } } private bool HasAllErrors { get { return ErrorsHelper.GetHasErrors(_errorsAll); } } internal int GlobalErrorCount { get { return ErrorsHelper.GetErrorCount(_errorsGlobal); } } // // Add a configuration Error. // internal void AddError(ConfigurationException ce, ExceptionAction action) { switch (action) { case ExceptionAction.Global: ErrorsHelper.AddError(ref _errorsAll, ce); ErrorsHelper.AddError(ref _errorsGlobal, ce); break; case ExceptionAction.NonSpecific: ErrorsHelper.AddError(ref _errorsAll, ce); break; case ExceptionAction.Local: ErrorsHelper.AddError(ref _errorsLocal, ce); break; } } internal void SetSingleGlobalError(ConfigurationException ce) { _errorsAll = null; _errorsLocal = null; _errorsGlobal = null; AddError(ce, ExceptionAction.Global); } internal bool HasErrors(bool ignoreLocal) { if (ignoreLocal) { return HasGlobalErrors; } else { return HasAllErrors; } } // ThrowIfErrors // // Throw if Errors were detected and remembered. // // Parameters: // IgnoreLocal - Should we be using the local errors also to // detemine if we should throw? // // Note: We will always return all the errors, no matter what // IgnoreLocal is. // internal void ThrowIfErrors(bool ignoreLocal) { if (HasErrors(ignoreLocal)) { if (HasGlobalErrors) { // Throw just the global errors, as they invalidate // all other config file parsing. throw new ConfigurationErrorsException(_errorsGlobal); } else { // Throw all errors no matter what throw new ConfigurationErrorsException(_errorsAll); } } } // RetrieveAndResetLocalErrors // // Retrieve the Local Errors, and Reset them to none. // internal List RetrieveAndResetLocalErrors(bool keepLocalErrors) { List list = _errorsLocal; _errorsLocal = null; if (keepLocalErrors) { ErrorsHelper.AddErrors(ref _errorsAll, list); } return list; } // // Add errors that have been saved for a specific section. // internal void AddSavedLocalErrors(ICollection coll) { ErrorsHelper.AddErrors(ref _errorsAll, coll); } // ResetLocalErrors // // Remove all the Local Errors, so we can start from scratch // internal void ResetLocalErrors() { RetrieveAndResetLocalErrors(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Text; internal class ConfigurationSchemaErrors { // Errors with ExceptionAction.Local are logged to this list. // This list is reset when processing of a section is complete. // Errors on this list may be added to the _errorsAll list // when RetrieveAndResetLocalErrors is called. private List_errorsLocal; // Errors with ExceptionAction.Global are logged to this list. private List _errorsGlobal; // All errors related to a config file are logged to this list. // This includes all global errors, all non-specific errors, // and local errors for input that applies to this config file. private List _errorsAll; internal ConfigurationSchemaErrors() {} internal bool HasLocalErrors { get { return ErrorsHelper.GetHasErrors(_errorsLocal); } } internal bool HasGlobalErrors { get { return ErrorsHelper.GetHasErrors(_errorsGlobal); } } private bool HasAllErrors { get { return ErrorsHelper.GetHasErrors(_errorsAll); } } internal int GlobalErrorCount { get { return ErrorsHelper.GetErrorCount(_errorsGlobal); } } // // Add a configuration Error. // internal void AddError(ConfigurationException ce, ExceptionAction action) { switch (action) { case ExceptionAction.Global: ErrorsHelper.AddError(ref _errorsAll, ce); ErrorsHelper.AddError(ref _errorsGlobal, ce); break; case ExceptionAction.NonSpecific: ErrorsHelper.AddError(ref _errorsAll, ce); break; case ExceptionAction.Local: ErrorsHelper.AddError(ref _errorsLocal, ce); break; } } internal void SetSingleGlobalError(ConfigurationException ce) { _errorsAll = null; _errorsLocal = null; _errorsGlobal = null; AddError(ce, ExceptionAction.Global); } internal bool HasErrors(bool ignoreLocal) { if (ignoreLocal) { return HasGlobalErrors; } else { return HasAllErrors; } } // ThrowIfErrors // // Throw if Errors were detected and remembered. // // Parameters: // IgnoreLocal - Should we be using the local errors also to // detemine if we should throw? // // Note: We will always return all the errors, no matter what // IgnoreLocal is. // internal void ThrowIfErrors(bool ignoreLocal) { if (HasErrors(ignoreLocal)) { if (HasGlobalErrors) { // Throw just the global errors, as they invalidate // all other config file parsing. throw new ConfigurationErrorsException(_errorsGlobal); } else { // Throw all errors no matter what throw new ConfigurationErrorsException(_errorsAll); } } } // RetrieveAndResetLocalErrors // // Retrieve the Local Errors, and Reset them to none. // internal List RetrieveAndResetLocalErrors(bool keepLocalErrors) { List list = _errorsLocal; _errorsLocal = null; if (keepLocalErrors) { ErrorsHelper.AddErrors(ref _errorsAll, list); } return list; } // // Add errors that have been saved for a specific section. // internal void AddSavedLocalErrors(ICollection coll) { ErrorsHelper.AddErrors(ref _errorsAll, coll); } // ResetLocalErrors // // Remove all the Local Errors, so we can start from scratch // internal void ResetLocalErrors() { RetrieveAndResetLocalErrors(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
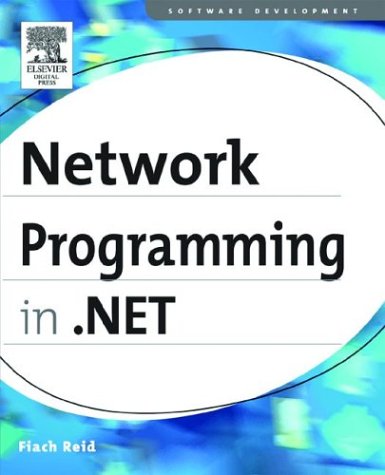
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymmetricAlgorithm.cs
- ParameterCollection.cs
- RequestCacheEntry.cs
- SQLCharsStorage.cs
- TextRangeEditLists.cs
- GatewayDefinition.cs
- ResourceExpressionBuilder.cs
- XmlAnyAttributeAttribute.cs
- RichTextBox.cs
- BrushMappingModeValidation.cs
- DSACryptoServiceProvider.cs
- UxThemeWrapper.cs
- DataServiceQueryOfT.cs
- RTTypeWrapper.cs
- StringAnimationBase.cs
- ScrollContentPresenter.cs
- ButtonBase.cs
- ProcessThread.cs
- PasswordRecovery.cs
- ColorConverter.cs
- DBNull.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- DateTimeSerializationSection.cs
- DTCTransactionManager.cs
- SafeRightsManagementHandle.cs
- Label.cs
- CustomSignedXml.cs
- EventSourceCreationData.cs
- AttachInfo.cs
- LayoutTable.cs
- XmlCharacterData.cs
- CacheSection.cs
- SynchronizationFilter.cs
- RangeValidator.cs
- Binding.cs
- LoginViewDesigner.cs
- CodeSubDirectory.cs
- SqlUtil.cs
- CodeTypeDeclarationCollection.cs
- UserPreferenceChangingEventArgs.cs
- GridViewColumnHeader.cs
- RemotingException.cs
- TCPListener.cs
- PerformanceCounters.cs
- NamedPipeTransportSecurity.cs
- DeploymentSectionCache.cs
- TemplateApplicationHelper.cs
- AnnotationResourceChangedEventArgs.cs
- XmlDataSourceDesigner.cs
- ControlValuePropertyAttribute.cs
- AutoResetEvent.cs
- FreezableOperations.cs
- BitmapData.cs
- ProcessInfo.cs
- DateTimeEditor.cs
- DataGridViewCell.cs
- CompositeControl.cs
- ShapingWorkspace.cs
- dsa.cs
- TypefaceMap.cs
- SpStreamWrapper.cs
- XPathSingletonIterator.cs
- XmlAttributes.cs
- SchemaAttDef.cs
- WebSysDefaultValueAttribute.cs
- WsdlExporter.cs
- PropertyMap.cs
- ListQueryResults.cs
- ResourceKey.cs
- FormViewDeletedEventArgs.cs
- CreateUserWizard.cs
- FileSystemWatcher.cs
- LayoutEvent.cs
- ConditionalAttribute.cs
- rsa.cs
- VisualStyleInformation.cs
- WebPartAuthorizationEventArgs.cs
- FilterException.cs
- MappingMetadataHelper.cs
- UrlPath.cs
- ScrollEventArgs.cs
- DataServiceOperationContext.cs
- PeerContact.cs
- WebServiceData.cs
- ListViewCommandEventArgs.cs
- ListBindingConverter.cs
- SqlProviderManifest.cs
- CodeIndexerExpression.cs
- CompilerGlobalScopeAttribute.cs
- SecurityState.cs
- HtmlInputText.cs
- XmlWriter.cs
- _OverlappedAsyncResult.cs
- DomainUpDown.cs
- FontStyles.cs
- RefType.cs
- FloatSumAggregationOperator.cs
- ContentDisposition.cs
- xamlnodes.cs
- SamlConditions.cs