Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Services / Monitoring / system / Diagnosticts / ProcessThread.cs / 1 / ProcessThread.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Diagnostics { using System.Threading; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Collections; using System.IO; using Microsoft.Win32; using System.Security.Permissions; using Microsoft.Win32.SafeHandles; // using System.Windows.Forms; ////// [ Designer("System.Diagnostics.Design.ProcessThreadDesigner, " + AssemblyRef.SystemDesign), HostProtection(SelfAffectingProcessMgmt=true, SelfAffectingThreading=true) ] public class ProcessThread : Component { // // FIELDS // ThreadInfo threadInfo; bool isRemoteMachine; bool priorityBoostEnabled; bool havePriorityBoostEnabled; ThreadPriorityLevel priorityLevel; bool havePriorityLevel; // // CONSTRUCTORS // ////// Represents a Win32 thread. This can be used to obtain /// information about the thread, such as it's performance characteristics. This is /// returned from the System.Diagnostics.Process.ProcessThread property of the System.Diagnostics.Process component. /// ////// I don't understand /// the following comment associated with the previous sentence: "property of /// Process component " Rather than just "processTHread". There is no such /// member on Process. Do we mean 'threads'? /// ////// Internal constructor. /// ///internal ProcessThread(bool isRemoteMachine, ThreadInfo threadInfo) { this.isRemoteMachine = isRemoteMachine; this.threadInfo = threadInfo; GC.SuppressFinalize(this); } // // PROPERTIES // /// /// Returns the base priority of the thread which is computed by combining the /// process priority class with the priority level of the associated thread. /// [MonitoringDescription(SR.ThreadBasePriority)] public int BasePriority { get { return threadInfo.basePriority; } } ////// The current priority indicates the actual priority of the associated thread, /// which may deviate from the base priority based on how the OS is currently /// scheduling the thread. /// [MonitoringDescription(SR.ThreadCurrentPriority)] public int CurrentPriority { get { return threadInfo.currentPriority; } } ////// Returns the unique identifier for the associated thread. /// [MonitoringDescription(SR.ThreadId)] public int Id { get { return threadInfo.threadId; } } ////// [Browsable(false)] public int IdealProcessor { set { SafeThreadHandle threadHandle = null; try { threadHandle = OpenThreadHandle(NativeMethods.THREAD_SET_INFORMATION); if (NativeMethods.SetThreadIdealProcessor(threadHandle, value) < 0) { throw new Win32Exception(); } } finally { CloseThreadHandle(threadHandle); } } } ////// Sets the processor that this thread would ideally like to run on. /// ////// Returns or sets whether this thread would like a priority boost if the user interacts /// with user interface associated with this thread. /// [MonitoringDescription(SR.ThreadPriorityBoostEnabled)] public bool PriorityBoostEnabled { get { if (!havePriorityBoostEnabled) { SafeThreadHandle threadHandle = null; try { threadHandle = OpenThreadHandle(NativeMethods.THREAD_QUERY_INFORMATION); bool disabled = false; if (!NativeMethods.GetThreadPriorityBoost(threadHandle, out disabled)) { throw new Win32Exception(); } priorityBoostEnabled = !disabled; havePriorityBoostEnabled = true; } finally { CloseThreadHandle(threadHandle); } } return priorityBoostEnabled; } set { SafeThreadHandle threadHandle = null; try { threadHandle = OpenThreadHandle(NativeMethods.THREAD_SET_INFORMATION); if (!NativeMethods.SetThreadPriorityBoost(threadHandle, !value)) throw new Win32Exception(); priorityBoostEnabled = value; havePriorityBoostEnabled = true; } finally { CloseThreadHandle(threadHandle); } } } ////// Returns or sets the priority level of the associated thread. The priority level is /// not an absolute level, but instead contributes to the actual thread priority by /// considering the priority class of the process. /// [MonitoringDescription(SR.ThreadPriorityLevel)] public ThreadPriorityLevel PriorityLevel { get { if (!havePriorityLevel) { SafeThreadHandle threadHandle = null; try { threadHandle = OpenThreadHandle(NativeMethods.THREAD_QUERY_INFORMATION); int value = NativeMethods.GetThreadPriority(threadHandle); if (value == 0x7fffffff) { throw new Win32Exception(); } priorityLevel = (ThreadPriorityLevel)value; havePriorityLevel = true; } finally { CloseThreadHandle(threadHandle); } } return priorityLevel; } set { SafeThreadHandle threadHandle = null; try { threadHandle = OpenThreadHandle(NativeMethods.THREAD_SET_INFORMATION); if (!NativeMethods.SetThreadPriority(threadHandle, (int)value)) { throw new Win32Exception(); } priorityLevel = value; } finally { CloseThreadHandle(threadHandle); } } } ////// Returns the amount of time the thread has spent running code inside the operating /// system core. /// [MonitoringDescription(SR.ThreadPrivilegedProcessorTime)] public TimeSpan PrivilegedProcessorTime { get { EnsureState(State.IsNt); return GetThreadTimes().PrivilegedProcessorTime; } } ////// Returns the memory address of the function that was called when the associated /// thread was started. /// [MonitoringDescription(SR.ThreadStartAddress)] public IntPtr StartAddress { get { EnsureState(State.IsNt); return threadInfo.startAddress; } } ////// Returns the time the associated thread was started. /// [MonitoringDescription(SR.ThreadStartTime)] public DateTime StartTime { get { EnsureState(State.IsNt); return GetThreadTimes().StartTime; } } ////// Returns the current state of the associated thread, e.g. is it running, waiting, etc. /// [MonitoringDescription(SR.ThreadThreadState)] public ThreadState ThreadState { get { EnsureState(State.IsNt); return threadInfo.threadState; } } ////// Returns the amount of time the associated thread has spent utilizing the CPU. /// It is the sum of the System.Diagnostics.ProcessThread.UserProcessorTime and /// System.Diagnostics.ProcessThread.PrivilegedProcessorTime. /// [MonitoringDescription(SR.ThreadTotalProcessorTime)] public TimeSpan TotalProcessorTime { get { EnsureState(State.IsNt); return GetThreadTimes().TotalProcessorTime; } } ////// Returns the amount of time the associated thread has spent running code /// inside the application (not the operating system core). /// [MonitoringDescription(SR.ThreadUserProcessorTime)] public TimeSpan UserProcessorTime { get { EnsureState(State.IsNt); return GetThreadTimes().UserProcessorTime; } } ////// Returns the reason the associated thread is waiting, if any. /// [MonitoringDescription(SR.ThreadWaitReason)] public ThreadWaitReason WaitReason { get { EnsureState(State.IsNt); if (threadInfo.threadState != ThreadState.Wait) { throw new InvalidOperationException(SR.GetString(SR.WaitReasonUnavailable)); } return threadInfo.threadWaitReason; } } // // METHODS // ////// Helper to close a thread handle. /// ///private static void CloseThreadHandle(SafeThreadHandle handle) { if (handle != null) { handle.Close(); } } /// /// Helper to check preconditions for property access. /// void EnsureState(State state) { if ( ((state & State.IsLocal) != (State)0) && isRemoteMachine) { throw new NotSupportedException(SR.GetString(SR.NotSupportedRemoteThread)); } if ((state & State.IsNt) != (State)0) { if (Environment.OSVersion.Platform != PlatformID.Win32NT) { throw new PlatformNotSupportedException(SR.GetString(SR.WinNTRequired)); } } } ////// Helper to open a thread handle. /// ///SafeThreadHandle OpenThreadHandle(int access) { EnsureState(State.IsLocal); return ProcessManager.OpenThread(threadInfo.threadId, access); } /// /// Resets the ideal processor so there is no ideal processor for this thread (e.g. /// any processor is ideal). /// public void ResetIdealProcessor() { // 32 means "any processor is fine" IdealProcessor = 32; } ////// Sets which processors the associated thread is allowed to be scheduled to run on. /// Each processor is represented as a bit: bit 0 is processor one, bit 1 is processor /// two, etc. For example, the value 1 means run on processor one, 2 means run on /// processor two, 3 means run on processor one or two. /// [Browsable(false)] public IntPtr ProcessorAffinity { set { SafeThreadHandle threadHandle = null; try { threadHandle = OpenThreadHandle(NativeMethods.THREAD_SET_INFORMATION | NativeMethods.THREAD_QUERY_INFORMATION); if (NativeMethods.SetThreadAffinityMask(threadHandle, new HandleRef(this, value)) == IntPtr.Zero) { throw new Win32Exception(); } } finally { CloseThreadHandle(threadHandle); } } } private ProcessThreadTimes GetThreadTimes() { ProcessThreadTimes threadTimes = new ProcessThreadTimes(); SafeThreadHandle threadHandle = null; try { threadHandle = OpenThreadHandle(NativeMethods.THREAD_QUERY_INFORMATION); if (!NativeMethods.GetThreadTimes(threadHandle, out threadTimes.create, out threadTimes.exit, out threadTimes.kernel, out threadTimes.user)) { throw new Win32Exception(); } } finally { CloseThreadHandle(threadHandle); } return threadTimes; } ////// Preconditions for accessing properties. /// ///enum State { IsLocal = 0x2, IsNt = 0x4 } } }
Link Menu
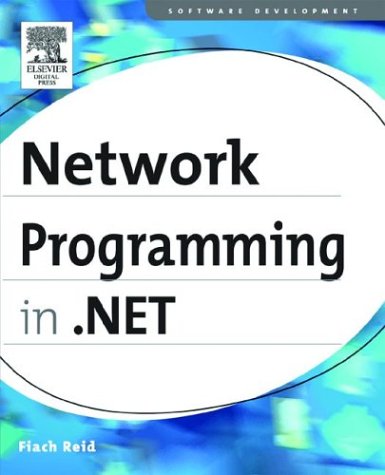
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseTemplateParser.cs
- ObjectDataProvider.cs
- BrowserDefinitionCollection.cs
- InitializationEventAttribute.cs
- Popup.cs
- AttributeInfo.cs
- FlagsAttribute.cs
- PasswordBoxAutomationPeer.cs
- EntityPropertyMappingAttribute.cs
- CircleHotSpot.cs
- OpacityConverter.cs
- StoryFragments.cs
- ParserStack.cs
- SystemIPGlobalStatistics.cs
- CheckBoxField.cs
- PackageRelationship.cs
- MailFileEditor.cs
- UndirectedGraph.cs
- CommandConverter.cs
- TextBoxRenderer.cs
- MonthCalendar.cs
- FontUnitConverter.cs
- WebPartChrome.cs
- IChannel.cs
- WebBrowserUriTypeConverter.cs
- COM2FontConverter.cs
- RoutedEventValueSerializer.cs
- SerializationObjectManager.cs
- WebColorConverter.cs
- DataSet.cs
- ChannelToken.cs
- DataSetUtil.cs
- InputScopeConverter.cs
- ArgumentNullException.cs
- LocalBuilder.cs
- ClientSettingsSection.cs
- RuleSettings.cs
- ImpersonationContext.cs
- FontFamilyIdentifier.cs
- TileBrush.cs
- ListViewGroupConverter.cs
- URL.cs
- DataTableTypeConverter.cs
- HttpStaticObjectsCollectionWrapper.cs
- DataGridViewCellValidatingEventArgs.cs
- EventDriven.cs
- ItemList.cs
- InputProviderSite.cs
- QueryCacheManager.cs
- SafeRightsManagementEnvironmentHandle.cs
- XPathChildIterator.cs
- XsdBuilder.cs
- FormCollection.cs
- TemplateColumn.cs
- NestedContainer.cs
- RsaSecurityTokenParameters.cs
- Faults.cs
- AxisAngleRotation3D.cs
- MaskInputRejectedEventArgs.cs
- FigureParaClient.cs
- Viewport3DAutomationPeer.cs
- PrintDialog.cs
- SubclassTypeValidatorAttribute.cs
- COMException.cs
- Point3DValueSerializer.cs
- StandardCommands.cs
- CreateUserWizard.cs
- Span.cs
- RectAnimationBase.cs
- HttpHandler.cs
- TerminateSequence.cs
- ResXResourceWriter.cs
- VariableQuery.cs
- SmtpReplyReaderFactory.cs
- UriTemplateMatchException.cs
- UnsafeNativeMethods.cs
- ObjectSecurityT.cs
- XsdCachingReader.cs
- CellConstant.cs
- FlowDocument.cs
- MimeMapping.cs
- FormatterConverter.cs
- TypeBuilder.cs
- BamlBinaryWriter.cs
- ToolStripSystemRenderer.cs
- ChannelManager.cs
- ThreadExceptionDialog.cs
- NullPackagingPolicy.cs
- StickyNoteContentControl.cs
- XmlAtomicValue.cs
- Comparer.cs
- IndexingContentUnit.cs
- AvTrace.cs
- EntityDataSourceDesigner.cs
- HttpRequest.cs
- ScaleTransform.cs
- MatrixCamera.cs
- externdll.cs
- Quaternion.cs
- OdbcStatementHandle.cs