Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / EntityPropertyMappingAttribute.cs / 1305376 / EntityPropertyMappingAttribute.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// EntityPropertyMapping attribute implementation // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { using System; using System.Data.Services.Client; ////// List of syndication properties settable through ////// /// Potentially the following atom specific elements could also be considered: /// * Author* /// * Category* /// * Content? /// * Contributor* /// * Id /// * Link* /// * Source? /// public enum SyndicationItemProperty { ////// User specified a non-syndication property /// CustomProperty, ////// author/email /// AuthorEmail, ////// author/name /// AuthorName, // Author* ////// author/uri /// AuthorUri, ////// contributor/email /// ContributorEmail, ////// contributor/name /// ContributorName, // Contributor* ////// contributor/uri /// ContributorUri, ////// updated /// Updated, // CategoryTerm, // Category* // CategoryScheme, // CategoryLabel, // LinkHref, // Link* // LinkRel, // LinkType, // LinkHrefLang, // LinkTitle, // LinkLength, ////// published /// Published, ////// rights /// Rights, ////// summary /// Summary, ////// title /// Title } ////// Type of content for a public enum SyndicationTextContentKind { ///if the property /// is of text type /// /// Plaintext /// Plaintext, ////// HTML /// Html, ////// XHTML /// Xhtml } ////// Attribute used for mapping a given property or sub-property of a ResourceType to /// an xml element/attribute with arbitrary nesting /// [AttributeUsage(AttributeTargets.Class, AllowMultiple = true, Inherited = true)] public sealed class EntityPropertyMappingAttribute : Attribute { #region Private Members ///Schema Namespace prefix for atom. private const string AtomNamespacePrefix = "atom"; ////// Source property path /// private readonly String sourcePath; ////// Target Xml element/attribute /// private readonly String targetPath; ////// If mapping to syndication element, the name of syndication item /// private readonly SyndicationItemProperty targetSyndicationItem; ////// If mapping to syndication content, the content type of syndication item /// private readonly SyndicationTextContentKind targetTextContentKind; ////// If mapping to non-syndication element/attribute, the namespace prefix for the /// target element/attribute /// private readonly String targetNamespacePrefix; ////// If mapping to non-syndication element/attribute, the namespace for the /// target element/attribute /// private readonly String targetNamespaceUri; ////// The content can optionally be kept in the original location along with the /// newly mapping location by setting this option to true, false by default /// private readonly bool keepInContent; #endregion #region Constructors ////// Used for mapping a resource property to syndication content /// /// Source property path /// Syndication item to which theis mapped /// Syndication content kind for /// If true the property value is kept in the content section as before, /// when false the property value is only placed at the mapped location public EntityPropertyMappingAttribute(String sourcePath, SyndicationItemProperty targetSyndicationItem, SyndicationTextContentKind targetTextContentKind, bool keepInContent) { if (String.IsNullOrEmpty(sourcePath)) { throw new ArgumentException(Strings.EntityPropertyMapping_EpmAttribute("sourcePath")); } this.sourcePath = sourcePath; this.targetPath = SyndicationItemPropertyToPath(targetSyndicationItem); this.targetSyndicationItem = targetSyndicationItem; this.targetTextContentKind = targetTextContentKind; this.targetNamespacePrefix = EntityPropertyMappingAttribute.AtomNamespacePrefix; this.targetNamespaceUri = XmlConstants.AtomNamespace; this.keepInContent = keepInContent; } /// /// Used for mapping a resource property to arbitrary xml element/attribute /// /// Source property path /// Target element/attribute path /// Namespace prefix for theto which belongs /// Uri of the namespace to which belongs /// If true the property value is kept in the content section as before, /// when false the property value is only placed at the mapped location public EntityPropertyMappingAttribute(String sourcePath, String targetPath, String targetNamespacePrefix, String targetNamespaceUri, bool keepInContent) { if (String.IsNullOrEmpty(sourcePath)) { throw new ArgumentException(Strings.EntityPropertyMapping_EpmAttribute("sourcePath")); } this.sourcePath = sourcePath; if (String.IsNullOrEmpty(targetPath)) { throw new ArgumentException(Strings.EntityPropertyMapping_EpmAttribute("targetPath")); } if (targetPath[0] == '@') { throw new ArgumentException(Strings.EpmTargetTree_InvalidTargetPath(targetPath)); } this.targetPath = targetPath; this.targetSyndicationItem = SyndicationItemProperty.CustomProperty; this.targetTextContentKind = SyndicationTextContentKind.Plaintext; this.targetNamespacePrefix = targetNamespacePrefix; if (String.IsNullOrEmpty(targetNamespaceUri)) { throw new ArgumentException(Strings.EntityPropertyMapping_EpmAttribute("targetNamespaceUri")); } this.targetNamespaceUri = targetNamespaceUri; Uri uri; if (!Uri.TryCreate(targetNamespaceUri, UriKind.Absolute, out uri)) { throw new ArgumentException(Strings.EntityPropertyMapping_TargetNamespaceUriNotValid(targetNamespaceUri)); } this.keepInContent = keepInContent; } #region Properties /// /// Source property path /// public String SourcePath { get { return this.sourcePath; } } ////// Target Xml element/attribute /// public String TargetPath { get { return this.targetPath; } } ////// If mapping to syndication element, the name of syndication item /// public SyndicationItemProperty TargetSyndicationItem { get { return this.targetSyndicationItem; } } ////// If mapping to non-syndication element/attribute, the namespace prefix for the /// target element/attribute /// public String TargetNamespacePrefix { get { return this.targetNamespacePrefix; } } ////// If mapping to non-syndication element/attribute, the namespace for the /// target element/attribute /// public String TargetNamespaceUri { get { return this.targetNamespaceUri; } } ////// If mapping to syndication content, the content type of syndication item /// public SyndicationTextContentKind TargetTextContentKind { get { return this.targetTextContentKind; } } ////// The content can optionally be kept in the original location along with the /// newly mapping location by setting this option to true, false by default /// public bool KeepInContent { get { return this.keepInContent; } } #endregion ////// Maps the enumeration of allowed /// Value of thevalues to their string representations /// given in /// the contstructor /// String representing the xml element path in the syndication property internal static String SyndicationItemPropertyToPath(SyndicationItemProperty targetSyndicationItem) { switch (targetSyndicationItem) { case SyndicationItemProperty.AuthorEmail: return "author/email"; case SyndicationItemProperty.AuthorName: return "author/name"; case SyndicationItemProperty.AuthorUri: return "author/uri"; case SyndicationItemProperty.ContributorEmail: return "contributor/email"; case SyndicationItemProperty.ContributorName: return "contributor/name"; case SyndicationItemProperty.ContributorUri: return "contributor/uri"; case SyndicationItemProperty.Updated: return "updated"; case SyndicationItemProperty.Published: return "published"; case SyndicationItemProperty.Rights: return "rights"; case SyndicationItemProperty.Summary: return "summary"; case SyndicationItemProperty.Title: return "title"; default: throw new ArgumentException(Strings.EntityPropertyMapping_EpmAttribute("targetSyndicationItem")); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// EntityPropertyMapping attribute implementation // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { using System; using System.Data.Services.Client; ////// List of syndication properties settable through ////// /// Potentially the following atom specific elements could also be considered: /// * Author* /// * Category* /// * Content? /// * Contributor* /// * Id /// * Link* /// * Source? /// public enum SyndicationItemProperty { ////// User specified a non-syndication property /// CustomProperty, ////// author/email /// AuthorEmail, ////// author/name /// AuthorName, // Author* ////// author/uri /// AuthorUri, ////// contributor/email /// ContributorEmail, ////// contributor/name /// ContributorName, // Contributor* ////// contributor/uri /// ContributorUri, ////// updated /// Updated, // CategoryTerm, // Category* // CategoryScheme, // CategoryLabel, // LinkHref, // Link* // LinkRel, // LinkType, // LinkHrefLang, // LinkTitle, // LinkLength, ////// published /// Published, ////// rights /// Rights, ////// summary /// Summary, ////// title /// Title } ////// Type of content for a public enum SyndicationTextContentKind { ///if the property /// is of text type /// /// Plaintext /// Plaintext, ////// HTML /// Html, ////// XHTML /// Xhtml } ////// Attribute used for mapping a given property or sub-property of a ResourceType to /// an xml element/attribute with arbitrary nesting /// [AttributeUsage(AttributeTargets.Class, AllowMultiple = true, Inherited = true)] public sealed class EntityPropertyMappingAttribute : Attribute { #region Private Members ///Schema Namespace prefix for atom. private const string AtomNamespacePrefix = "atom"; ////// Source property path /// private readonly String sourcePath; ////// Target Xml element/attribute /// private readonly String targetPath; ////// If mapping to syndication element, the name of syndication item /// private readonly SyndicationItemProperty targetSyndicationItem; ////// If mapping to syndication content, the content type of syndication item /// private readonly SyndicationTextContentKind targetTextContentKind; ////// If mapping to non-syndication element/attribute, the namespace prefix for the /// target element/attribute /// private readonly String targetNamespacePrefix; ////// If mapping to non-syndication element/attribute, the namespace for the /// target element/attribute /// private readonly String targetNamespaceUri; ////// The content can optionally be kept in the original location along with the /// newly mapping location by setting this option to true, false by default /// private readonly bool keepInContent; #endregion #region Constructors ////// Used for mapping a resource property to syndication content /// /// Source property path /// Syndication item to which theis mapped /// Syndication content kind for /// If true the property value is kept in the content section as before, /// when false the property value is only placed at the mapped location public EntityPropertyMappingAttribute(String sourcePath, SyndicationItemProperty targetSyndicationItem, SyndicationTextContentKind targetTextContentKind, bool keepInContent) { if (String.IsNullOrEmpty(sourcePath)) { throw new ArgumentException(Strings.EntityPropertyMapping_EpmAttribute("sourcePath")); } this.sourcePath = sourcePath; this.targetPath = SyndicationItemPropertyToPath(targetSyndicationItem); this.targetSyndicationItem = targetSyndicationItem; this.targetTextContentKind = targetTextContentKind; this.targetNamespacePrefix = EntityPropertyMappingAttribute.AtomNamespacePrefix; this.targetNamespaceUri = XmlConstants.AtomNamespace; this.keepInContent = keepInContent; } /// /// Used for mapping a resource property to arbitrary xml element/attribute /// /// Source property path /// Target element/attribute path /// Namespace prefix for theto which belongs /// Uri of the namespace to which belongs /// If true the property value is kept in the content section as before, /// when false the property value is only placed at the mapped location public EntityPropertyMappingAttribute(String sourcePath, String targetPath, String targetNamespacePrefix, String targetNamespaceUri, bool keepInContent) { if (String.IsNullOrEmpty(sourcePath)) { throw new ArgumentException(Strings.EntityPropertyMapping_EpmAttribute("sourcePath")); } this.sourcePath = sourcePath; if (String.IsNullOrEmpty(targetPath)) { throw new ArgumentException(Strings.EntityPropertyMapping_EpmAttribute("targetPath")); } if (targetPath[0] == '@') { throw new ArgumentException(Strings.EpmTargetTree_InvalidTargetPath(targetPath)); } this.targetPath = targetPath; this.targetSyndicationItem = SyndicationItemProperty.CustomProperty; this.targetTextContentKind = SyndicationTextContentKind.Plaintext; this.targetNamespacePrefix = targetNamespacePrefix; if (String.IsNullOrEmpty(targetNamespaceUri)) { throw new ArgumentException(Strings.EntityPropertyMapping_EpmAttribute("targetNamespaceUri")); } this.targetNamespaceUri = targetNamespaceUri; Uri uri; if (!Uri.TryCreate(targetNamespaceUri, UriKind.Absolute, out uri)) { throw new ArgumentException(Strings.EntityPropertyMapping_TargetNamespaceUriNotValid(targetNamespaceUri)); } this.keepInContent = keepInContent; } #region Properties /// /// Source property path /// public String SourcePath { get { return this.sourcePath; } } ////// Target Xml element/attribute /// public String TargetPath { get { return this.targetPath; } } ////// If mapping to syndication element, the name of syndication item /// public SyndicationItemProperty TargetSyndicationItem { get { return this.targetSyndicationItem; } } ////// If mapping to non-syndication element/attribute, the namespace prefix for the /// target element/attribute /// public String TargetNamespacePrefix { get { return this.targetNamespacePrefix; } } ////// If mapping to non-syndication element/attribute, the namespace for the /// target element/attribute /// public String TargetNamespaceUri { get { return this.targetNamespaceUri; } } ////// If mapping to syndication content, the content type of syndication item /// public SyndicationTextContentKind TargetTextContentKind { get { return this.targetTextContentKind; } } ////// The content can optionally be kept in the original location along with the /// newly mapping location by setting this option to true, false by default /// public bool KeepInContent { get { return this.keepInContent; } } #endregion ////// Maps the enumeration of allowed /// Value of thevalues to their string representations /// given in /// the contstructor /// String representing the xml element path in the syndication property internal static String SyndicationItemPropertyToPath(SyndicationItemProperty targetSyndicationItem) { switch (targetSyndicationItem) { case SyndicationItemProperty.AuthorEmail: return "author/email"; case SyndicationItemProperty.AuthorName: return "author/name"; case SyndicationItemProperty.AuthorUri: return "author/uri"; case SyndicationItemProperty.ContributorEmail: return "contributor/email"; case SyndicationItemProperty.ContributorName: return "contributor/name"; case SyndicationItemProperty.ContributorUri: return "contributor/uri"; case SyndicationItemProperty.Updated: return "updated"; case SyndicationItemProperty.Published: return "published"; case SyndicationItemProperty.Rights: return "rights"; case SyndicationItemProperty.Summary: return "summary"; case SyndicationItemProperty.Title: return "title"; default: throw new ArgumentException(Strings.EntityPropertyMapping_EpmAttribute("targetSyndicationItem")); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
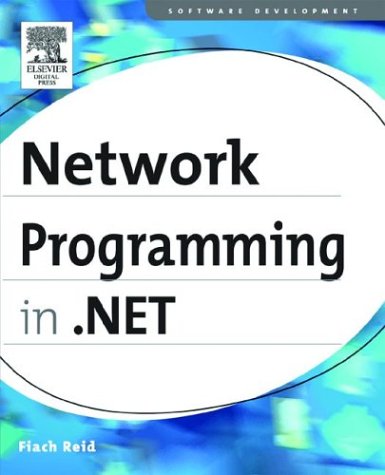
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XPathAncestorIterator.cs
- DrawingServices.cs
- FileDialogPermission.cs
- validationstate.cs
- DigestTraceRecordHelper.cs
- OraclePermission.cs
- XpsLiterals.cs
- ISO2022Encoding.cs
- ApplicationHost.cs
- DataGridTableCollection.cs
- IDispatchConstantAttribute.cs
- HttpBufferlessInputStream.cs
- AttributeEmitter.cs
- CryptoApi.cs
- GridViewPageEventArgs.cs
- SuppressMessageAttribute.cs
- XmlMemberMapping.cs
- ObjectItemAttributeAssemblyLoader.cs
- ViewEvent.cs
- SourceElementsCollection.cs
- wmiprovider.cs
- XmlSchemaElement.cs
- ActivationArguments.cs
- CapabilitiesPattern.cs
- ImageButton.cs
- XmlSchemaObjectTable.cs
- ConfigurationValue.cs
- localization.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- CodeMemberMethod.cs
- Boolean.cs
- Visitors.cs
- SectionInput.cs
- DefaultProxySection.cs
- LinearGradientBrush.cs
- ControlFilterExpression.cs
- FileIOPermission.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- VSWCFServiceContractGenerator.cs
- XmlName.cs
- ExplicitDiscriminatorMap.cs
- VScrollBar.cs
- CellParagraph.cs
- NavigateEvent.cs
- QueryCacheEntry.cs
- ClientConfigurationHost.cs
- CodeSnippetExpression.cs
- MimeMapping.cs
- DbgCompiler.cs
- AdornedElementPlaceholder.cs
- EdmFunctions.cs
- DbConnectionHelper.cs
- WorkflowViewElement.cs
- TypeUnloadedException.cs
- QueryableDataSource.cs
- FontConverter.cs
- TextBoxAutoCompleteSourceConverter.cs
- PagePropertiesChangingEventArgs.cs
- RoleGroup.cs
- FileInfo.cs
- LazyTextWriterCreator.cs
- BindToObject.cs
- RetrieveVirtualItemEventArgs.cs
- CurrentChangingEventArgs.cs
- Localizer.cs
- hebrewshape.cs
- BlobPersonalizationState.cs
- Paragraph.cs
- Dictionary.cs
- ValidationErrorCollection.cs
- BevelBitmapEffect.cs
- FontInfo.cs
- SchemaTableOptionalColumn.cs
- Convert.cs
- ConfigXmlWhitespace.cs
- MsmqOutputChannel.cs
- HashAlgorithm.cs
- SynchronizedPool.cs
- TrackingRecord.cs
- RangeEnumerable.cs
- MD5CryptoServiceProvider.cs
- TraceContextEventArgs.cs
- RTLAwareMessageBox.cs
- ISFTagAndGuidCache.cs
- SimpleHandlerBuildProvider.cs
- TheQuery.cs
- MappingItemCollection.cs
- Sql8ExpressionRewriter.cs
- RecipientInfo.cs
- DrawingContext.cs
- Point3DCollectionConverter.cs
- CharacterShapingProperties.cs
- ToolboxItem.cs
- ErrorTableItemStyle.cs
- CollectionChange.cs
- TemplatedWizardStep.cs
- MatrixAnimationUsingPath.cs
- CellTreeNode.cs
- XmlSchemaAttribute.cs
- ActivityBuilderHelper.cs