Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / Compilation / SimpleHandlerBuildProvider.cs / 1 / SimpleHandlerBuildProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.IO; using System.Collections; using System.Reflection; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Configuration; using System.Web.Util; using System.Web.UI; #if ORCAS using System.Web.UI.Imaging; #endif [BuildProviderAppliesTo(BuildProviderAppliesTo.Web)] internal abstract class SimpleHandlerBuildProvider: InternalBuildProvider { private SimpleWebHandlerParser _parser; internal override IAssemblyDependencyParser AssemblyDependencyParser { get { return _parser; } } protected abstract SimpleWebHandlerParser CreateParser(); public override CompilerType CodeCompilerType { get { Debug.Assert(_parser == null); _parser = CreateParser(); _parser.SetBuildProvider(this); _parser.IgnoreParseErrors = IgnoreParseErrors; _parser.Parse(ReferencedAssemblies); return _parser.CompilerType; } } protected internal override CodeCompileUnit GetCodeCompileUnit(out IDictionary linePragmasTable) { Debug.Assert(_parser != null); CodeCompileUnit ccu = _parser.GetCodeModel(); linePragmasTable = _parser.GetLinePragmasTable(); return ccu; } public override void GenerateCode(AssemblyBuilder assemblyBuilder) { CodeCompileUnit codeCompileUnit = _parser.GetCodeModel(); // Bail if we have nothing we need to compile if (codeCompileUnit == null) return; assemblyBuilder.AddCodeCompileUnit(this, codeCompileUnit); // Add all the assemblies if (_parser.AssemblyDependencies != null) { foreach (Assembly assembly in _parser.AssemblyDependencies) { assemblyBuilder.AddAssemblyReference(assembly, codeCompileUnit); } } // NOTE: we can't actually generate the fast factory because it would give // a really bad error if the user specifies a classname which doesn't match // the actual class they define. A bit unfortunate, but not that big a deal... // tell the host to generate a fast factory for this type (if any) //string generatedTypeName = _parser.GeneratedTypeName; //if (generatedTypeName != null) // assemblyBuilder.GenerateTypeFactory(generatedTypeName); } public override Type GetGeneratedType(CompilerResults results) { Type t; if (_parser.HasInlineCode) { // This is the case where the asmx/ashx has code in the file, and it // has been compiled. Debug.Assert(results != null); t = _parser.GetTypeToCache(results.CompiledAssembly); } else { // This is the case where the asmx/ashx has no code and is simply // pointing to an existing assembly. Set the UsesExistingAssembly // flag accordingly. t = _parser.GetTypeToCache(null); } return t; } public override ICollection VirtualPathDependencies { get { return _parser.SourceDependencies; } } internal CompilerType GetDefaultCompilerTypeForLanguageInternal(string language) { return GetDefaultCompilerTypeForLanguage(language); } internal CompilerType GetDefaultCompilerTypeInternal() { return GetDefaultCompilerType(); } internal TextReader OpenReaderInternal() { return OpenReader(); } internal override ICollection GetGeneratedTypeNames() { // Note that _parser.TypeName does not necessarily point to the type defined in the handler file, // it could be any type that can be referenced at runtime, App_Code for example. return new SingleObjectCollection(_parser.TypeName); } } #if ORCAS internal class ImageGeneratorBuildProvider: SimpleHandlerBuildProvider { private ImageGeneratorParser _parser; protected override SimpleWebHandlerParser CreateParser() { _parser = new ImageGeneratorParser(VirtualPath); return _parser; } internal override BuildResultCompiledType CreateBuildResult(Type t) { return new ImageGeneratorBuildResultCompiledType(t); } internal override BuildResult CreateBuildResult(CompilerResults results) { ImageGeneratorBuildResultCompiledType result = (ImageGeneratorBuildResultCompiledType)base.CreateBuildResult(results); result.OutputCacheInfo = _parser.OutputCacheDirective; result.CustomErrorImageUrl = _parser.CustomErrorImageUrl; return result; } } #endif internal class WebServiceBuildProvider: SimpleHandlerBuildProvider { protected override SimpleWebHandlerParser CreateParser() { return new WebServiceParser(VirtualPath); } } internal class WebHandlerBuildProvider: SimpleHandlerBuildProvider { protected override SimpleWebHandlerParser CreateParser() { return new WebHandlerParser(VirtualPath); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.IO; using System.Collections; using System.Reflection; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Configuration; using System.Web.Util; using System.Web.UI; #if ORCAS using System.Web.UI.Imaging; #endif [BuildProviderAppliesTo(BuildProviderAppliesTo.Web)] internal abstract class SimpleHandlerBuildProvider: InternalBuildProvider { private SimpleWebHandlerParser _parser; internal override IAssemblyDependencyParser AssemblyDependencyParser { get { return _parser; } } protected abstract SimpleWebHandlerParser CreateParser(); public override CompilerType CodeCompilerType { get { Debug.Assert(_parser == null); _parser = CreateParser(); _parser.SetBuildProvider(this); _parser.IgnoreParseErrors = IgnoreParseErrors; _parser.Parse(ReferencedAssemblies); return _parser.CompilerType; } } protected internal override CodeCompileUnit GetCodeCompileUnit(out IDictionary linePragmasTable) { Debug.Assert(_parser != null); CodeCompileUnit ccu = _parser.GetCodeModel(); linePragmasTable = _parser.GetLinePragmasTable(); return ccu; } public override void GenerateCode(AssemblyBuilder assemblyBuilder) { CodeCompileUnit codeCompileUnit = _parser.GetCodeModel(); // Bail if we have nothing we need to compile if (codeCompileUnit == null) return; assemblyBuilder.AddCodeCompileUnit(this, codeCompileUnit); // Add all the assemblies if (_parser.AssemblyDependencies != null) { foreach (Assembly assembly in _parser.AssemblyDependencies) { assemblyBuilder.AddAssemblyReference(assembly, codeCompileUnit); } } // NOTE: we can't actually generate the fast factory because it would give // a really bad error if the user specifies a classname which doesn't match // the actual class they define. A bit unfortunate, but not that big a deal... // tell the host to generate a fast factory for this type (if any) //string generatedTypeName = _parser.GeneratedTypeName; //if (generatedTypeName != null) // assemblyBuilder.GenerateTypeFactory(generatedTypeName); } public override Type GetGeneratedType(CompilerResults results) { Type t; if (_parser.HasInlineCode) { // This is the case where the asmx/ashx has code in the file, and it // has been compiled. Debug.Assert(results != null); t = _parser.GetTypeToCache(results.CompiledAssembly); } else { // This is the case where the asmx/ashx has no code and is simply // pointing to an existing assembly. Set the UsesExistingAssembly // flag accordingly. t = _parser.GetTypeToCache(null); } return t; } public override ICollection VirtualPathDependencies { get { return _parser.SourceDependencies; } } internal CompilerType GetDefaultCompilerTypeForLanguageInternal(string language) { return GetDefaultCompilerTypeForLanguage(language); } internal CompilerType GetDefaultCompilerTypeInternal() { return GetDefaultCompilerType(); } internal TextReader OpenReaderInternal() { return OpenReader(); } internal override ICollection GetGeneratedTypeNames() { // Note that _parser.TypeName does not necessarily point to the type defined in the handler file, // it could be any type that can be referenced at runtime, App_Code for example. return new SingleObjectCollection(_parser.TypeName); } } #if ORCAS internal class ImageGeneratorBuildProvider: SimpleHandlerBuildProvider { private ImageGeneratorParser _parser; protected override SimpleWebHandlerParser CreateParser() { _parser = new ImageGeneratorParser(VirtualPath); return _parser; } internal override BuildResultCompiledType CreateBuildResult(Type t) { return new ImageGeneratorBuildResultCompiledType(t); } internal override BuildResult CreateBuildResult(CompilerResults results) { ImageGeneratorBuildResultCompiledType result = (ImageGeneratorBuildResultCompiledType)base.CreateBuildResult(results); result.OutputCacheInfo = _parser.OutputCacheDirective; result.CustomErrorImageUrl = _parser.CustomErrorImageUrl; return result; } } #endif internal class WebServiceBuildProvider: SimpleHandlerBuildProvider { protected override SimpleWebHandlerParser CreateParser() { return new WebServiceParser(VirtualPath); } } internal class WebHandlerBuildProvider: SimpleHandlerBuildProvider { protected override SimpleWebHandlerParser CreateParser() { return new WebHandlerParser(VirtualPath); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
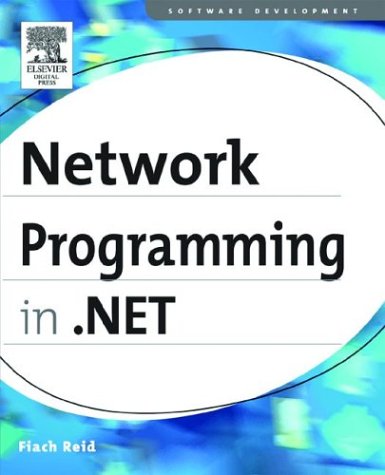
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SizeChangedEventArgs.cs
- TransformationRules.cs
- DataServiceRequestException.cs
- OutputCacheModule.cs
- EdmValidator.cs
- SmtpFailedRecipientsException.cs
- GridErrorDlg.cs
- TrustLevelCollection.cs
- MaskDescriptor.cs
- DataGridState.cs
- DbModificationCommandTree.cs
- ByteAnimation.cs
- DataListItemEventArgs.cs
- SqlConnection.cs
- NavigationService.cs
- XsltArgumentList.cs
- LabelEditEvent.cs
- FolderBrowserDialog.cs
- SqlTriggerAttribute.cs
- XmlHierarchicalEnumerable.cs
- AssociatedControlConverter.cs
- BitmapEffectInput.cs
- DefaultDiscoveryServiceExtension.cs
- FlagsAttribute.cs
- AmbientLight.cs
- QilVisitor.cs
- ReachSerializationCacheItems.cs
- PackWebRequestFactory.cs
- DataSetViewSchema.cs
- DirectoryNotFoundException.cs
- MultiByteCodec.cs
- XmlBuffer.cs
- CriticalFinalizerObject.cs
- PerfCounterSection.cs
- DiscoveryDocument.cs
- DataGridCaption.cs
- OdbcConnectionString.cs
- TlsnegoTokenAuthenticator.cs
- FormatterConverter.cs
- ServicePointManager.cs
- EntityDataSourceState.cs
- SelfIssuedAuthAsymmetricKey.cs
- IsolatedStoragePermission.cs
- NavigationPropertyEmitter.cs
- AdapterDictionary.cs
- DelegateArgument.cs
- XamlSerializerUtil.cs
- CompleteWizardStep.cs
- WebPartAuthorizationEventArgs.cs
- TextParagraphCache.cs
- StringWriter.cs
- MethodAccessException.cs
- XPathNodePointer.cs
- OrderedDictionaryStateHelper.cs
- GuidelineCollection.cs
- MatrixConverter.cs
- MimeWriter.cs
- DataGridViewSortCompareEventArgs.cs
- CompositeControlDesigner.cs
- Int64Storage.cs
- EventLogPermissionEntry.cs
- FamilyMapCollection.cs
- TextBoxLine.cs
- TraceXPathNavigator.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- SizeIndependentAnimationStorage.cs
- UriParserTemplates.cs
- EventProperty.cs
- GeneralTransform3DGroup.cs
- DataGridViewRowPostPaintEventArgs.cs
- ListParagraph.cs
- UInt64.cs
- WorkflowEnvironment.cs
- TemplateControl.cs
- AutoScrollExpandMessageFilter.cs
- ClientTarget.cs
- _SSPISessionCache.cs
- SmtpDateTime.cs
- EditorBrowsableAttribute.cs
- SafeBitVector32.cs
- PathSegmentCollection.cs
- PolyBezierSegment.cs
- BindingParameterCollection.cs
- MulticastNotSupportedException.cs
- ObjectDataSourceView.cs
- MobileControlsSectionHandler.cs
- CodeMemberProperty.cs
- Identity.cs
- _ListenerRequestStream.cs
- EditorAttribute.cs
- ListDependantCardsRequest.cs
- OrderByExpression.cs
- PermissionRequestEvidence.cs
- MimeWriter.cs
- CounterSampleCalculator.cs
- XPathAxisIterator.cs
- UpdateExpressionVisitor.cs
- TileBrush.cs
- BuildManager.cs
- EditorPartCollection.cs