Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / ExpressionBuilder / EdmFunctions.cs / 1305376 / EdmFunctions.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Globalization; using System.IO; using System.Diagnostics; using System.Text; using System.Text.RegularExpressions; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Data.Common.CommandTrees.ExpressionBuilder.Internal; using System.Reflection; namespace System.Data.Common.CommandTrees.ExpressionBuilder { ////// Provides an API to construct [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] #if PUBLIC_DBEXPRESSIONBUILDER public #endif static class EdmFunctions { #region Private Implementation private static EdmFunction ResolveCanonicalFunction(string functionName, TypeUsage[] argumentTypes) { Debug.Assert(!string.IsNullOrEmpty(functionName), "Function name must not be null"); Lists that invoke canonical EDM functions, and allows that API to be accessed as extension methods on the expression type itself. /// functions = new List ( System.Linq.Enumerable.Where( EdmProviderManifest.Instance.GetStoreFunctions(), func => string.Equals(func.Name, functionName, StringComparison.Ordinal)) ); EdmFunction foundFunction = null; bool ambiguous = false; if (functions.Count > 0) { foundFunction = EntitySql.FunctionOverloadResolver.ResolveFunctionOverloads(functions, argumentTypes, false, out ambiguous); if (ambiguous) { throw EntityUtil.Argument(Entity.Strings.Cqt_Function_CanonicalFunction_AmbiguousMatch(functionName)); } } if (foundFunction == null) { throw EntityUtil.Argument(Entity.Strings.Cqt_Function_CanonicalFunction_NotFound(functionName)); } return foundFunction; } private static DbFunctionExpression InvokeCanonicalFunction(string functionName, params DbExpression[] arguments) { TypeUsage[] argumentTypes = new TypeUsage[arguments.Length]; for (int idx = 0; idx < arguments.Length; idx++) { Debug.Assert(arguments[idx] != null, "Ensure arguments are non-null before calling InvokeCanonicalFunction"); argumentTypes[idx] = arguments[idx].ResultType; } EdmFunction foundFunction = ResolveCanonicalFunction(functionName, argumentTypes); return DbExpressionBuilder.Invoke(foundFunction, arguments); } #endregion #if PUBLIC_DBEXPRESSIONBUILDER #region Aggregate functions - Average, Count, LongCount, Max, Min, Sum, StDev, StDevP, Var, VarP /// /// Creates a /// An expression that specifies the collection from which the average value should be computed ///that invokes the canonical 'Avg' function over the /// specified collection. The result type of the expression is the same as the element type of the collection. /// A new DbFunctionExpression that produces the average value. ////// is null. No overload of the canonical 'Avg' function accepts an argument with the result type of public static DbFunctionExpression Average(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Avg", collection); } ///. /// Creates a /// An expression that specifies the collection over which the count value should be computed. ///that invokes the canonical 'Count' function over the /// specified collection. The result type of the expression is Edm.Int32. /// A new DbFunctionExpression that produces the count value. ////// is null. No overload of the canonical 'Count' function accepts an argument with the result type of public static DbFunctionExpression Count(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Count", collection); } ///. /// Creates a /// An expression that specifies the collection over which the count value should be computed. ///that invokes the canonical 'BigCount' function over the /// specified collection. The result type of the expression is Edm.Int64. /// A new DbFunctionExpression that produces the count value. ////// is null. No overload of the canonical 'BigCount' function accepts an argument with the result type of public static DbFunctionExpression LongCount(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("BigCount", collection); } ///. /// Creates a /// An expression that specifies the collection from which the maximum value should be retrieved ///that invokes the canonical 'Max' function over the /// specified collection. The result type of the expression is the same as the element type of the collection. /// A new DbFunctionExpression that produces the maximum value. ////// is null. No overload of the canonical 'Max' function accepts an argument with the result type of public static DbFunctionExpression Max(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Max", collection); } ///. /// Creates a /// An expression that specifies the collection from which the minimum value should be retrieved ///that invokes the canonical 'Min' function over the /// specified collection. The result type of the expression is the same as the element type of the collection. /// A new DbFunctionExpression that produces the minimum value. ////// is null. No overload of the canonical 'Min' function accepts an argument with the result type of public static DbFunctionExpression Min(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Min", collection); } ///. /// Creates a /// An expression that specifies the collection from which the sum should be computed ///that invokes the canonical 'Sum' function over the /// specified collection. The result type of the expression is the same as the element type of the collection. /// A new DbFunctionExpression that produces the sum. ////// is null. No overload of the canonical 'Sum' function accepts an argument with the result type of public static DbFunctionExpression Sum(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Sum", collection); } ///. /// Creates a /// An expression that specifies the collection for which the standard deviation should be computed ///that invokes the canonical 'StDev' function over the /// non-null members of the specified collection. The result type of the expression is Edm.Double. /// A new DbFunctionExpression that produces the standard deviation value over non-null members of the collection. ////// is null. No overload of the canonical 'StDev' function accepts an argument with the result type of [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "St")] public static DbFunctionExpression StDev(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("StDev", collection); } ///. /// Creates a /// An expression that specifies the collection for which the standard deviation should be computed ///that invokes the canonical 'StDevP' function over the /// population of the specified collection. The result type of the expression is Edm.Double. /// A new DbFunctionExpression that produces the standard deviation value. ////// is null. No overload of the canonical 'StDevP' function accepts an argument with the result type of [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "St")] public static DbFunctionExpression StDevP(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("StDevP", collection); } ///. /// Creates a /// An expression that specifies the collection for which the statistical variance should be computed ///that invokes the canonical 'Var' function over the /// non-null members of the specified collection. The result type of the expression is Edm.Double. /// A new DbFunctionExpression that produces the statistical variance value for the non-null members of the collection. ////// is null. No overload of the canonical 'Var' function accepts an argument with the result type of public static DbFunctionExpression Var(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Var", collection); } ///. /// Creates a /// An expression that specifies the collection for which the statistical variance should be computed ///that invokes the canonical 'VarP' function over the /// population of the specified collection. The result type of the expression Edm.Double. /// A new DbFunctionExpression that produces the statistical variance value. ////// is null. No overload of the canonical 'VarP' function accepts an argument with the result type of public static DbFunctionExpression VarP(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("VarP", collection); } #endregion #region String functions - Concat, Contains, EndsWith, IndexOf, Left, Length, LTrim, Replace, Reverse, Right, RTrim, StartsWith, Substring, ToUpper, ToLower, Trim ///. /// Creates a /// An expression that specifies the string that should appear first in the concatenated result string. /// An expression that specifies the string that should appear second in the concatenated result string. ///that invokes the canonical 'Concat' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// string. /// A new DbFunctionExpression that produces the concatenated string. ////// or is null. No overload of the canonical 'Concat' function accepts arguments with the result types of public static DbFunctionExpression Concat(this DbExpression string1, DbExpression string2) { EntityUtil.CheckArgumentNull(string1, "string1"); EntityUtil.CheckArgumentNull(string2, "string2"); return InvokeCanonicalFunction("Concat", string1, string2); } // ///and . /// Creates a /// An expression that specifies the string to search for any occurence ofthat invokes the canonical 'Contains' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// Boolean. /// . /// An expression that specifies the string to search for in . /// A new DbFunctionExpression that returns a Boolean value indicating whether or not ///occurs within . /// or is null. No overload of the canonical 'Contains' function accepts arguments with the result types of public static DbExpression Contains(this DbExpression searchedString, DbExpression searchedForString) { EntityUtil.CheckArgumentNull(searchedString, "searchedString"); EntityUtil.CheckArgumentNull(searchedForString, "searchedForString"); return InvokeCanonicalFunction("Contains", searchedString, searchedForString); } ///and . /// Creates a /// An expression that specifies the string to check for the specified . /// An expression that specifies the suffix for whichthat invokes the canonical 'EndsWith' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// Boolean. /// should be checked. /// A new DbFunctionExpression that indicates whether ///ends with . /// or is null. No overload of the canonical 'EndsWith' function accepts arguments with the result types of public static DbFunctionExpression EndsWith(this DbExpression stringArgument, DbExpression suffix) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(suffix, "suffix"); return InvokeCanonicalFunction("EndsWith", stringArgument, suffix); } ///and . /// Creates a ///that invokes the canonical 'IndexOf' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// Edm.Int32. /// The index returned by IndexOf is 1-based. /// An expression that specifies the string to search for. /// An expression that specifies the string to locate within should be checked. /// A new DbFunctionExpression that returns the first index of ///in . /// or is null. No overload of the canonical 'IndexOf' function accepts arguments with the result types of public static DbFunctionExpression IndexOf(this DbExpression searchString, DbExpression stringToFind) { EntityUtil.CheckArgumentNull(searchString, "searchString"); EntityUtil.CheckArgumentNull(stringToFind, "stringToFind"); return InvokeCanonicalFunction("IndexOf", stringToFind, searchString); } ///and . /// Creates a /// An expression that specifies the string from which to extract the leftmost substring. /// An expression that specifies the length of the leftmost substring to extract fromthat invokes the canonical 'Left' function with the /// specified arguments, which must have a string and integer numeric result type. The result type of the expression is /// string. /// . /// A new DbFunctionExpression that returns the the leftmost substring of length ///from . /// or is null. No overload of the canonical 'Left' function accepts arguments with the result types of public static DbFunctionExpression Left(this DbExpression stringArgument, DbExpression length) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(length, "length"); return InvokeCanonicalFunction("Left", stringArgument, length); } ///. /// Creates a /// An expression that specifies the string for which the length should be computed. ///that invokes the canonical 'Length' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns the the length of ///. /// is null. No overload of the canonical 'Length' function accepts an argument with the result type of public static DbFunctionExpression Length(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("Length", stringArgument); } ///. /// Creates a /// An expression that specifies the string in which to perform the replacement operation /// An expression that specifies the string to replace /// An expression that specifies the replacement string ///that invokes the canonical 'Replace' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression than returns a new string based on ///where every occurence of is replaced by . /// , or is null. No overload of the canonical 'Length' function accepts arguments with the result types of public static DbFunctionExpression Replace(this DbExpression stringArgument, DbExpression toReplace, DbExpression replacement) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(toReplace, "toReplace"); EntityUtil.CheckArgumentNull(replacement, "replacement"); return InvokeCanonicalFunction("Replace", stringArgument, toReplace, replacement); } ///, and . /// Creates a /// An expression that specifies the string to reverse. ///that invokes the canonical 'Reverse' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that produces the reversed value of ///. /// is null. No overload of the canonical 'Reverse' function accepts an argument with the result type of public static DbFunctionExpression Reverse(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("Reverse", stringArgument); } ///. /// Creates a /// An expression that specifies the string from which to extract the rightmost substring. /// An expression that specifies the length of the rightmost substring to extract fromthat invokes the canonical 'Right' function with the /// specified arguments, which must have a string and integer numeric result type. The result type of the expression is /// string. /// . /// A new DbFunctionExpression that returns the the rightmost substring of length ///from . /// or is null. No overload of the canonical 'Right' function accepts arguments with the result types of public static DbFunctionExpression Right(this DbExpression stringArgument, DbExpression length) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(length, "length"); return InvokeCanonicalFunction("Right", stringArgument, length); } ///. /// Creates a /// An expression that specifies the string to check for the specified . /// An expression that specifies the prefix for whichthat invokes the canonical 'StartsWith' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// Boolean. /// should be checked. /// A new DbFunctionExpression that indicates whether ///starts with . /// or is null. No overload of the canonical 'StartsWith' function accepts arguments with the result types of public static DbFunctionExpression StartsWith(this DbExpression stringArgument, DbExpression prefix) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(prefix, "prefix"); return InvokeCanonicalFunction("StartsWith", stringArgument, prefix); } // ///and . /// Creates a ///that invokes the canonical 'Substring' function with the /// specified arguments, which must have a string and integer numeric result types. The result type of the /// expression is string. /// Substring requires that the index specified by /// An expression that specifies the string from which to extract the substring. /// An expression that specifies the starting index from which the substring should be taken. /// An expression that specifies the length of the substring. ///be 1-based. A new DbFunctionExpression that returns the substring of length ///from starting at . /// , or is null. No overload of the canonical 'Substring' function accepts arguments with the result types of public static DbFunctionExpression Substring(this DbExpression stringArgument, DbExpression start, DbExpression length) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(start, "start"); EntityUtil.CheckArgumentNull(length, "length"); return InvokeCanonicalFunction("Substring", stringArgument, start, length); } ///, and . /// Creates a /// An expression that specifies the string that should be converted to lower case. ///that invokes the canonical 'ToLower' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns value of ///converted to lower case. /// is null. No overload of the canonical 'ToLower' function accepts an argument with the result type of public static DbFunctionExpression ToLower(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("ToLower", stringArgument); } ///. /// Creates a /// An expression that specifies the string that should be converted to upper case. ///that invokes the canonical 'ToUpper' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns value of ///converted to upper case. /// is null. No overload of the canonical 'ToUpper' function accepts an argument with the result type of public static DbFunctionExpression ToUpper(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("ToUpper", stringArgument); } ///. /// Creates a /// An expression that specifies the string from which leading and trailing space should be removed. ///that invokes the canonical 'Trim' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns value of ///with leading and trailing space removed. /// is null. No overload of the canonical 'Trim' function accepts an argument with the result type of public static DbFunctionExpression Trim(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("Trim", stringArgument); } ///. /// Creates a /// An expression that specifies the string from which trailing space should be removed. ///that invokes the canonical 'RTrim' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns value of ///with trailing space removed. /// is null. No overload of the canonical 'RTrim' function accepts an argument with the result type of public static DbFunctionExpression TrimEnd(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("RTrim", stringArgument); } ///. /// Creates a /// An expression that specifies the string from which leading space should be removed. ///that invokes the canonical 'LTrim' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns value of ///with leading space removed. /// is null. No overload of the canonical 'LTrim' function accepts an argument with the result type of public static DbFunctionExpression TrimStart(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("LTrim", stringArgument); } #endregion #region Date/Time member access methods - Year, Month, Day, DayOfYear, Hour, Minute, Second, Millisecond, GetTotalOffsetMinutes ///. /// Creates a /// An expression that specifies the value from which the year should be retrieved. ///that invokes the canonical 'Year' function with the /// specified argument, which must have a DateTime or DateTimeOffset result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer year value from ///. /// is null. No overload of the canonical 'Year' function accepts an argument with the result type of public static DbFunctionExpression Year(this DbExpression dateValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); return InvokeCanonicalFunction("Year", dateValue); } ///. /// Creates a /// An expression that specifies the value from which the month should be retrieved. ///that invokes the canonical 'Month' function with the /// specified argument, which must have a DateTime or DateTimeOffset result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer month value from ///. /// is null. No overload of the canonical 'Month' function accepts an argument with the result type of public static DbFunctionExpression Month(this DbExpression dateValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); return InvokeCanonicalFunction("Month", dateValue); } ///. /// Creates a /// An expression that specifies the value from which the day should be retrieved. ///that invokes the canonical 'Day' function with the /// specified argument, which must have a DateTime or DateTimeOffset result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer day value from ///. /// is null. No overload of the canonical 'Day' function accepts an argument with the result type of public static DbFunctionExpression Day(this DbExpression dateValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); return InvokeCanonicalFunction("Day", dateValue); } ///. /// Creates a /// An expression that specifies the value from which the day within the year should be retrieved. ///that invokes the canonical 'DayOfYear' function with the /// specified argument, which must have a DateTime or DateTimeOffset result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer day of year value from ///. /// is null. No overload of the canonical 'DayOfYear' function accepts an argument with the result type of public static DbFunctionExpression DayOfYear(this DbExpression dateValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); return InvokeCanonicalFunction("DayOfYear", dateValue); } ///. /// Creates a /// An expression that specifies the value from which the hour should be retrieved. ///that invokes the canonical 'Hour' function with the /// specified argument, which must have a DateTime, DateTimeOffset or Time result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer hour value from ///. /// is null. No overload of the canonical 'Hour' function accepts an argument with the result type of public static DbFunctionExpression Hour(this DbExpression timeValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); return InvokeCanonicalFunction("Hour", timeValue); } ///. /// Creates a /// An expression that specifies the value from which the minute should be retrieved. ///that invokes the canonical 'Minute' function with the /// specified argument, which must have a DateTime, DateTimeOffset or Time result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer minute value from ///. /// is null. No overload of the canonical 'Minute' function accepts an argument with the result type of public static DbFunctionExpression Minute(this DbExpression timeValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); return InvokeCanonicalFunction("Minute", timeValue); } ///. /// Creates a /// An expression that specifies the value from which the second should be retrieved. ///that invokes the canonical 'Second' function with the /// specified argument, which must have a DateTime, DateTimeOffset or Time result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer second value from ///. /// is null. No overload of the canonical 'Second' function accepts an argument with the result type of public static DbFunctionExpression Second(this DbExpression timeValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); return InvokeCanonicalFunction("Second", timeValue); } ///. /// Creates a /// An expression that specifies the value from which the millisecond should be retrieved. ///that invokes the canonical 'Millisecond' function with the /// specified argument, which must have a DateTime, DateTimeOffset or Time result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer millisecond value from ///. /// is null. No overload of the canonical 'Millisecond' function accepts an argument with the result type of public static DbFunctionExpression Millisecond(this DbExpression timeValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); return InvokeCanonicalFunction("Millisecond", timeValue); } ///. /// Creates a /// An expression that specifies the DateTimeOffset value from which the minute offset from GMT should be retrieved. ///that invokes the canonical 'GetTotalOffsetMinutes' function with the /// specified argument, which must have a DateTimeOffset result type. The result type of the expression is Edm.Int32. /// A new DbFunctionExpression that returns the number of minutes ///is offset from GMT. /// is null. No overload of the canonical 'GetTotalOffsetMinutes' function accepts an argument with the result type of public static DbFunctionExpression GetTotalOffsetMinutes(this DbExpression dateTimeOffsetArgument) { EntityUtil.CheckArgumentNull(dateTimeOffsetArgument, "dateTimeOffsetArgument"); return InvokeCanonicalFunction("GetTotalOffsetMinutes", dateTimeOffsetArgument); } #endregion #region Date/Time creation methods - CurrentDateTime, CurrentDateTimeOffset, CurrentUtcDateTime, CreateDateTime, CreateDateTimeOffset, CreateTime, TruncateTime ///. /// Creates a ///that invokes the canonical 'CurrentDateTime' function. /// A new DbFunctionExpression that returns the current date and time as an Edm.DateTime instance. public static DbFunctionExpression CurrentDateTime() { return InvokeCanonicalFunction("CurrentDateTime"); } ////// Creates a ///that invokes the canonical 'CurrentDateTimeOffset' function. /// A new DbFunctionExpression that returns the current date and time as an Edm.DateTimeOffset instance. public static DbFunctionExpression CurrentDateTimeOffset() { return InvokeCanonicalFunction("CurrentDateTimeOffset"); } ////// Creates a ///that invokes the canonical 'CurrentUtcDateTime' function. /// A new DbFunctionExpression that returns the current UTC date and time as an Edm.DateTime instance. public static DbFunctionExpression CurrentUtcDateTime() { return InvokeCanonicalFunction("CurrentUtcDateTime"); } ////// Creates a /// An expression that specifies the value for which the time component should be truncated. ///that invokes the canonical 'TruncateTime' function with the /// specified argument, which must have a DateTime or DateTimeOffset result type. The result type of the /// expression is the same as the result type of . /// A new DbFunctionExpression that returns the value of ///with time set to zero. /// is null. No overload of the canonical 'TruncateTime' function accepts an argument with the result type of public static DbFunctionExpression TruncateTime(this DbExpression dateValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); return InvokeCanonicalFunction("TruncateTime", dateValue); } ///. /// Creates a /// An expression that provides the year component value for the new DateTime instance. /// An expression that provides the month component value for the new DateTime instance. /// An expression that provides the day component value for the new DateTime instance. /// An expression that provides the hour component value for the new DateTime instance. /// An expression that provides the minute component value for the new DateTime instance. /// An expression that provides the second component value for the new DateTime instance. ///that invokes the canonical 'CreateDateTime' function with the /// specified arguments. must have a result type of Edm.Double, while all other arguments /// must have a result type of Edm.Int32. The result type of the expression is Edm.DateTime. /// A new DbFunctionExpression that returns a new DateTime based on the specified component values. ////// , , , , , or is null. No overload of the canonical 'CreateDateTime' function accepts arguments with the result types of public static DbFunctionExpression CreateDateTime(DbExpression year, DbExpression month, DbExpression day, DbExpression hour, DbExpression minute, DbExpression second) { EntityUtil.CheckArgumentNull(year, "year"); EntityUtil.CheckArgumentNull(month, "month"); EntityUtil.CheckArgumentNull(day, "day"); EntityUtil.CheckArgumentNull(hour, "hour"); EntityUtil.CheckArgumentNull(minute, "minute"); EntityUtil.CheckArgumentNull(second, "second"); return InvokeCanonicalFunction("CreateDateTime", year, month, day, hour, minute, second); } ///, , , , , and . /// Creates a /// An expression that provides the year component value for the new DateTimeOffset instance. /// An expression that provides the month component value for the new DateTimeOffset instance. /// An expression that provides the day component value for the new DateTimeOffset instance. /// An expression that provides the hour component value for the new DateTimeOffset instance. /// An expression that provides the minute component value for the new DateTimeOffset instance. /// An expression that provides the second component value for the new DateTimeOffset instance. /// An expression that provides the number of minutes in the time zone offset component value for the new DateTimeOffset instance. ///that invokes the canonical 'CreateDateTimeOffset' function with the /// specified arguments. must have a result type of Edm.Double, while all other arguments /// must have a result type of Edm.Int32. The result type of the expression is Edm.DateTimeOffset. /// A new DbFunctionExpression that returns a new DateTimeOffset based on the specified component values. ////// , , , , , or is null. No overload of the canonical 'CreateDateTimeOffset' function accepts arguments with the result types of public static DbFunctionExpression CreateDateTimeOffset(DbExpression year, DbExpression month, DbExpression day, DbExpression hour, DbExpression minute, DbExpression second, DbExpression timeZoneOffset) { EntityUtil.CheckArgumentNull(year, "year"); EntityUtil.CheckArgumentNull(month, "month"); EntityUtil.CheckArgumentNull(day, "day"); EntityUtil.CheckArgumentNull(hour, "hour"); EntityUtil.CheckArgumentNull(minute, "minute"); EntityUtil.CheckArgumentNull(second, "second"); EntityUtil.CheckArgumentNull(timeZoneOffset, "timeZoneOffset"); return InvokeCanonicalFunction("CreateDateTimeOffset", year, month, day, hour, minute, second, timeZoneOffset); } ///, , , , , and . /// Creates a /// An expression that provides the hour component value for the new DateTime instance. /// An expression that provides the minute component value for the new DateTime instance. /// An expression that provides the second component value for the new DateTime instance. ///that invokes the canonical 'CreateTime' function with the /// specified arguments. must have a result type of Edm.Double, while all other arguments /// must have a result type of Edm.Int32. The result type of the expression is Edm.Time. /// A new DbFunctionExpression that returns a new Time based on the specified component values. ////// , , or is null. No overload of the canonical 'CreateTime' function accepts arguments with the result types of public static DbFunctionExpression CreateTime(DbExpression hour, DbExpression minute, DbExpression second) { EntityUtil.CheckArgumentNull(hour, "hour"); EntityUtil.CheckArgumentNull(minute, "minute"); EntityUtil.CheckArgumentNull(second, "second"); return InvokeCanonicalFunction("CreateTime", hour, minute, second); } #endregion #region Date/Time addition - AddYears, AddMonths, AddDays, AddHours, AddMinutes, AddSeconds, AddMilliseconds, AddMicroseconds, AddNanoseconds ///, , and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddYears' function with the /// specified arguments, which must have DateTime or DateTimeOffset and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of years to add to . /// A new DbFunctionExpression that adds the number of years specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddYears' function accepts arguments with the result types of public static DbFunctionExpression AddYears(this DbExpression dateValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddYears", dateValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddMonths' function with the /// specified arguments, which must have DateTime or DateTimeOffset and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of months to add to . /// A new DbFunctionExpression that adds the number of months specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddMonths' function accepts arguments with the result types of public static DbFunctionExpression AddMonths(this DbExpression dateValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddMonths", dateValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddDays' function with the /// specified arguments, which must have DateTime or DateTimeOffset and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of days to add to . /// A new DbFunctionExpression that adds the number of days specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddDays' function accepts arguments with the result types of public static DbFunctionExpression AddDays(this DbExpression dateValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddDays", dateValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddHours' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of hours to add to . /// A new DbFunctionExpression that adds the number of hours specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddHours' function accepts arguments with the result types of public static DbFunctionExpression AddHours(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddHours", timeValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddMinutes' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of minutes to add to . /// A new DbFunctionExpression that adds the number of minutes specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddMinutes' function accepts arguments with the result types of public static DbFunctionExpression AddMinutes(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddMinutes", timeValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddSeconds' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of seconds to add to . /// A new DbFunctionExpression that adds the number of seconds specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddSeconds' function accepts arguments with the result types of public static DbFunctionExpression AddSeconds(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddSeconds", timeValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddMilliseconds' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of milliseconds to add to . /// A new DbFunctionExpression that adds the number of milliseconds specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddMilliseconds' function accepts arguments with the result types of public static DbFunctionExpression AddMilliseconds(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddMilliseconds", timeValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddMicroseconds' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of microseconds to add to . /// A new DbFunctionExpression that adds the number of microseconds specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddMicroseconds' function accepts arguments with the result types of public static DbFunctionExpression AddMicroseconds(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddMicroseconds", timeValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddNanoseconds' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of nanoseconds to add to . /// A new DbFunctionExpression that adds the number of nanoseconds specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddNanoseconds' function accepts arguments with the result types of public static DbFunctionExpression AddNanoseconds(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddNanoseconds", timeValue, addValue); } #endregion #region Date/Time difference - DiffYears, DiffMonths, DiffDays, DiffHours, DiffMinutes, DiffSeconds, DiffMilliseconds, DiffMicroseconds, DiffNanoseconds ///and . /// Creates a /// An expression that specifies the first DateTime or DateTimeOffset value. /// An expression that specifies the DateTime or DateTimeOffset for which the year difference fromthat invokes the canonical 'DiffYears' function with the /// specified arguments, which must each have a DateTime or DateTimeOffset result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the year difference between and . ////// or is null. No overload of the canonical 'DiffYears' function accepts arguments with the result types of public static DbFunctionExpression DiffYears(this DbExpression dateValue1, DbExpression dateValue2) { EntityUtil.CheckArgumentNull(dateValue1, "dateValue1"); EntityUtil.CheckArgumentNull(dateValue2, "dateValue2"); return InvokeCanonicalFunction("DiffYears", dateValue1, dateValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime or DateTimeOffset value. /// An expression that specifies the DateTime or DateTimeOffset for which the month difference fromthat invokes the canonical 'DiffMonths' function with the /// specified arguments, which must each have a DateTime or DateTimeOffset result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the month difference between and . ////// or is null. No overload of the canonical 'DiffMonths' function accepts arguments with the result types of public static DbFunctionExpression DiffMonths(this DbExpression dateValue1, DbExpression dateValue2) { EntityUtil.CheckArgumentNull(dateValue1, "dateValue1"); EntityUtil.CheckArgumentNull(dateValue2, "dateValue2"); return InvokeCanonicalFunction("DiffMonths", dateValue1, dateValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime or DateTimeOffset value. /// An expression that specifies the DateTime or DateTimeOffset for which the day difference fromthat invokes the canonical 'DiffDays' function with the /// specified arguments, which must each have a DateTime or DateTimeOffset result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the day difference between and . ////// or is null. No overload of the canonical 'DiffDays' function accepts arguments with the result types of public static DbFunctionExpression DiffDays(this DbExpression dateValue1, DbExpression dateValue2) { EntityUtil.CheckArgumentNull(dateValue1, "dateValue1"); EntityUtil.CheckArgumentNull(dateValue2, "dateValue2"); return InvokeCanonicalFunction("DiffDays", dateValue1, dateValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the hour difference fromthat invokes the canonical 'DiffHours' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the hour difference between and . ////// or is null. No overload of the canonical 'DiffHours' function accepts arguments with the result types of public static DbFunctionExpression DiffHours(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffHours", timeValue1, timeValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the minute difference fromthat invokes the canonical 'DiffMinutes' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the minute difference between and . ////// or is null. No overload of the canonical 'DiffMinutes' function accepts arguments with the result types of public static DbFunctionExpression DiffMinutes(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffMinutes", timeValue1, timeValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the second difference fromthat invokes the canonical 'DiffSeconds' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the second difference between and . ////// or is null. No overload of the canonical 'DiffSeconds' function accepts arguments with the result types of public static DbFunctionExpression DiffSeconds(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffSeconds", timeValue1, timeValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the millisecond difference fromthat invokes the canonical 'DiffMilliseconds' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the millisecond difference between and . ////// or is null. No overload of the canonical 'DiffMilliseconds' function accepts arguments with the result types of public static DbFunctionExpression DiffMilliseconds(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffMilliseconds", timeValue1, timeValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the microsecond difference fromthat invokes the canonical 'DiffMicroseconds' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the microsecond difference between and . ////// or is null. No overload of the canonical 'DiffMicroseconds' function accepts arguments with the result types of public static DbFunctionExpression DiffMicroseconds(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffMicroseconds", timeValue1, timeValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the nanosecond difference fromthat invokes the canonical 'DiffNanoseconds' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the nanosecond difference between and . ////// or is null. No overload of the canonical 'DiffNanoseconds' function accepts arguments with the result types of public static DbFunctionExpression DiffNanoseconds(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffNanoseconds", timeValue1, timeValue2); } #endregion #region Math functions - Floor, Ceiling, Round, Truncate, Abs, Power ///and . /// Creates a /// An expression that specifies the numeric value to round. ///that invokes the canonical 'Round' function with the /// specified argument, which must each have a single, double or decimal result type. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that rounds the specified argument to the nearest integer value. ////// is null. No overload of the canonical 'Round' function accepts an argument with the result type of public static DbFunctionExpression Round(this DbExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return InvokeCanonicalFunction("Round", value); } ///. /// Creates a /// An expression that specifies the numeric value to round. /// An expression that specifies the number of digits of precision to use when rounding. ///that invokes the canonical 'Round' function with the /// specified arguments, which must have a single, double or decimal, and integer result types. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that rounds the specified argument to the nearest integer value, with precision as specified by ///. /// or is null. No overload of the canonical 'Round' function accepts arguments with the result types of public static DbFunctionExpression Round(this DbExpression value, DbExpression digits) { EntityUtil.CheckArgumentNull(value, "value"); EntityUtil.CheckArgumentNull(digits, "digits"); return InvokeCanonicalFunction("Round", value, digits); } ///and . /// Creates a /// An expression that specifies the numeric value. ///that invokes the canonical 'Floor' function with the /// specified argument, which must each have a single, double or decimal result type. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that returns the largest integer value not greater than ///. /// is null. No overload of the canonical 'Floor' function accepts an argument with the result type of public static DbFunctionExpression Floor(this DbExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return InvokeCanonicalFunction("Floor", value); } ///. /// Creates a /// An expression that specifies the numeric value. ///that invokes the canonical 'Ceiling' function with the /// specified argument, which must each have a single, double or decimal result type. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that returns the smallest integer value not less than than ///. /// is null. No overload of the canonical 'Ceiling' function accepts an argument with the result type of public static DbFunctionExpression Ceiling(this DbExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return InvokeCanonicalFunction("Ceiling", value); } ///. /// Creates a /// An expression that specifies the numeric value. ///that invokes the canonical 'Abs' function with the /// specified argument, which must each have a numeric result type. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that returns the absolute value of ///. /// is null. No overload of the canonical 'Abs' function accepts an argument with the result type of public static DbFunctionExpression Abs(this DbExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return InvokeCanonicalFunction("Abs", value); } ///. /// Creates a /// An expression that specifies the numeric value to truncate. /// An expression that specifies the number of digits of precision to use when truncating. ///that invokes the canonical 'Truncate' function with the /// specified arguments, which must have a single, double or decimal, and integer result types. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that truncates the specified argument to the nearest integer value, with precision as specified by ///. /// is null. No overload of the canonical 'Truncate' function accepts arguments with the result types of public static DbFunctionExpression Truncate(this DbExpression value, DbExpression digits) { EntityUtil.CheckArgumentNull(value, "value"); EntityUtil.CheckArgumentNull(digits, "digits"); return InvokeCanonicalFunction("Truncate", value, digits); } #endregion #endif ///and . /// Creates a /// An expression that specifies the numeric value to raise to the given power. /// An expression that specifies the power to whichthat invokes the canonical 'Power' function with the /// specified arguments, which must have numeric result types. The result type of the expression is /// the same as the result type of . /// should be raised. /// A new DbFunctionExpression that returns the value of ///raised to the power specified by . /// is null. No overload of the canonical 'Power' function accepts arguments with the result types of public static DbFunctionExpression Power(this DbExpression baseArgument, DbExpression exponent) { EntityUtil.CheckArgumentNull(baseArgument, "baseArgument"); EntityUtil.CheckArgumentNull(exponent, "exponent"); return InvokeCanonicalFunction("Power", baseArgument, exponent); } #if PUBLIC_DBEXPRESSIONBUILDER #region Bitwise functions - And, Or, Not, Xor ///and . /// Creates a /// An expression that specifies the first operand. /// An expression that specifies the second operand. ///that invokes the canonical 'BitwiseAnd' function with the /// specified arguments, which must have the same integer numeric result type. The result type of the /// expression is this same type. /// A new DbFunctionExpression that returns the value produced by performing the bitwise AND of ///and . /// is null. No overload of the canonical 'BitwiseAnd' function accepts arguments with the result types of public static DbFunctionExpression BitwiseAnd(this DbExpression value1, DbExpression value2) { EntityUtil.CheckArgumentNull(value1, "value1"); EntityUtil.CheckArgumentNull(value2, "value2"); return InvokeCanonicalFunction("BitwiseAnd", value1, value2); } ///and . /// Creates a /// An expression that specifies the first operand. /// An expression that specifies the second operand. ///that invokes the canonical 'BitwiseOr' function with the /// specified arguments, which must have the same integer numeric result type. The result type of the /// expression is this same type. /// A new DbFunctionExpression that returns the value produced by performing the bitwise OR of ///and . /// is null. No overload of the canonical 'BitwiseOr' function accepts arguments with the result types of public static DbFunctionExpression BitwiseOr(this DbExpression value1, DbExpression value2) { EntityUtil.CheckArgumentNull(value1, "value1"); EntityUtil.CheckArgumentNull(value2, "value2"); return InvokeCanonicalFunction("BitwiseOr", value1, value2); } ///and . /// Creates a /// An expression that specifies the first operand. ///that invokes the canonical 'BitwiseNot' function with the /// specified argument, which must have an integer numeric result type. The result type of the expression /// is this same type. /// A new DbFunctionExpression that returns the value produced by performing the bitwise NOT of ///. /// is null. No overload of the canonical 'BitwiseNot' function accepts an argument with the result type of public static DbFunctionExpression BitwiseNot(this DbExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return InvokeCanonicalFunction("BitwiseNot", value); } ///. /// Creates a /// An expression that specifies the first operand. /// An expression that specifies the second operand. ///that invokes the canonical 'BitwiseXor' function with the /// specified arguments, which must have the same integer numeric result type. The result type of the /// expression is this same type. /// A new DbFunctionExpression that returns the value produced by performing the bitwise XOR (exclusive OR) of ///and . /// is null. No overload of the canonical 'BitwiseXor' function accepts arguments with the result types of public static DbFunctionExpression BitwiseXor(this DbExpression value1, DbExpression value2) { EntityUtil.CheckArgumentNull(value1, "value1"); EntityUtil.CheckArgumentNull(value2, "value2"); return InvokeCanonicalFunction("BitwiseXor", value1, value2); } #endregion #endif #region GUID Generation - NewGuid ///and . /// Creates a ///that invokes the canonical 'NewGuid' function. /// A new DbFunctionExpression that returns a new GUID value. public static DbFunctionExpression NewGuid() { return InvokeCanonicalFunction("NewGuid"); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Globalization; using System.IO; using System.Diagnostics; using System.Text; using System.Text.RegularExpressions; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Data.Common.CommandTrees.ExpressionBuilder.Internal; using System.Reflection; namespace System.Data.Common.CommandTrees.ExpressionBuilder { ////// Provides an API to construct [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] #if PUBLIC_DBEXPRESSIONBUILDER public #endif static class EdmFunctions { #region Private Implementation private static EdmFunction ResolveCanonicalFunction(string functionName, TypeUsage[] argumentTypes) { Debug.Assert(!string.IsNullOrEmpty(functionName), "Function name must not be null"); Lists that invoke canonical EDM functions, and allows that API to be accessed as extension methods on the expression type itself. /// functions = new List ( System.Linq.Enumerable.Where( EdmProviderManifest.Instance.GetStoreFunctions(), func => string.Equals(func.Name, functionName, StringComparison.Ordinal)) ); EdmFunction foundFunction = null; bool ambiguous = false; if (functions.Count > 0) { foundFunction = EntitySql.FunctionOverloadResolver.ResolveFunctionOverloads(functions, argumentTypes, false, out ambiguous); if (ambiguous) { throw EntityUtil.Argument(Entity.Strings.Cqt_Function_CanonicalFunction_AmbiguousMatch(functionName)); } } if (foundFunction == null) { throw EntityUtil.Argument(Entity.Strings.Cqt_Function_CanonicalFunction_NotFound(functionName)); } return foundFunction; } private static DbFunctionExpression InvokeCanonicalFunction(string functionName, params DbExpression[] arguments) { TypeUsage[] argumentTypes = new TypeUsage[arguments.Length]; for (int idx = 0; idx < arguments.Length; idx++) { Debug.Assert(arguments[idx] != null, "Ensure arguments are non-null before calling InvokeCanonicalFunction"); argumentTypes[idx] = arguments[idx].ResultType; } EdmFunction foundFunction = ResolveCanonicalFunction(functionName, argumentTypes); return DbExpressionBuilder.Invoke(foundFunction, arguments); } #endregion #if PUBLIC_DBEXPRESSIONBUILDER #region Aggregate functions - Average, Count, LongCount, Max, Min, Sum, StDev, StDevP, Var, VarP /// /// Creates a /// An expression that specifies the collection from which the average value should be computed ///that invokes the canonical 'Avg' function over the /// specified collection. The result type of the expression is the same as the element type of the collection. /// A new DbFunctionExpression that produces the average value. ////// is null. No overload of the canonical 'Avg' function accepts an argument with the result type of public static DbFunctionExpression Average(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Avg", collection); } ///. /// Creates a /// An expression that specifies the collection over which the count value should be computed. ///that invokes the canonical 'Count' function over the /// specified collection. The result type of the expression is Edm.Int32. /// A new DbFunctionExpression that produces the count value. ////// is null. No overload of the canonical 'Count' function accepts an argument with the result type of public static DbFunctionExpression Count(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Count", collection); } ///. /// Creates a /// An expression that specifies the collection over which the count value should be computed. ///that invokes the canonical 'BigCount' function over the /// specified collection. The result type of the expression is Edm.Int64. /// A new DbFunctionExpression that produces the count value. ////// is null. No overload of the canonical 'BigCount' function accepts an argument with the result type of public static DbFunctionExpression LongCount(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("BigCount", collection); } ///. /// Creates a /// An expression that specifies the collection from which the maximum value should be retrieved ///that invokes the canonical 'Max' function over the /// specified collection. The result type of the expression is the same as the element type of the collection. /// A new DbFunctionExpression that produces the maximum value. ////// is null. No overload of the canonical 'Max' function accepts an argument with the result type of public static DbFunctionExpression Max(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Max", collection); } ///. /// Creates a /// An expression that specifies the collection from which the minimum value should be retrieved ///that invokes the canonical 'Min' function over the /// specified collection. The result type of the expression is the same as the element type of the collection. /// A new DbFunctionExpression that produces the minimum value. ////// is null. No overload of the canonical 'Min' function accepts an argument with the result type of public static DbFunctionExpression Min(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Min", collection); } ///. /// Creates a /// An expression that specifies the collection from which the sum should be computed ///that invokes the canonical 'Sum' function over the /// specified collection. The result type of the expression is the same as the element type of the collection. /// A new DbFunctionExpression that produces the sum. ////// is null. No overload of the canonical 'Sum' function accepts an argument with the result type of public static DbFunctionExpression Sum(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Sum", collection); } ///. /// Creates a /// An expression that specifies the collection for which the standard deviation should be computed ///that invokes the canonical 'StDev' function over the /// non-null members of the specified collection. The result type of the expression is Edm.Double. /// A new DbFunctionExpression that produces the standard deviation value over non-null members of the collection. ////// is null. No overload of the canonical 'StDev' function accepts an argument with the result type of [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "St")] public static DbFunctionExpression StDev(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("StDev", collection); } ///. /// Creates a /// An expression that specifies the collection for which the standard deviation should be computed ///that invokes the canonical 'StDevP' function over the /// population of the specified collection. The result type of the expression is Edm.Double. /// A new DbFunctionExpression that produces the standard deviation value. ////// is null. No overload of the canonical 'StDevP' function accepts an argument with the result type of [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "St")] public static DbFunctionExpression StDevP(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("StDevP", collection); } ///. /// Creates a /// An expression that specifies the collection for which the statistical variance should be computed ///that invokes the canonical 'Var' function over the /// non-null members of the specified collection. The result type of the expression is Edm.Double. /// A new DbFunctionExpression that produces the statistical variance value for the non-null members of the collection. ////// is null. No overload of the canonical 'Var' function accepts an argument with the result type of public static DbFunctionExpression Var(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("Var", collection); } ///. /// Creates a /// An expression that specifies the collection for which the statistical variance should be computed ///that invokes the canonical 'VarP' function over the /// population of the specified collection. The result type of the expression Edm.Double. /// A new DbFunctionExpression that produces the statistical variance value. ////// is null. No overload of the canonical 'VarP' function accepts an argument with the result type of public static DbFunctionExpression VarP(this DbExpression collection) { EntityUtil.CheckArgumentNull(collection, "collection"); return InvokeCanonicalFunction("VarP", collection); } #endregion #region String functions - Concat, Contains, EndsWith, IndexOf, Left, Length, LTrim, Replace, Reverse, Right, RTrim, StartsWith, Substring, ToUpper, ToLower, Trim ///. /// Creates a /// An expression that specifies the string that should appear first in the concatenated result string. /// An expression that specifies the string that should appear second in the concatenated result string. ///that invokes the canonical 'Concat' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// string. /// A new DbFunctionExpression that produces the concatenated string. ////// or is null. No overload of the canonical 'Concat' function accepts arguments with the result types of public static DbFunctionExpression Concat(this DbExpression string1, DbExpression string2) { EntityUtil.CheckArgumentNull(string1, "string1"); EntityUtil.CheckArgumentNull(string2, "string2"); return InvokeCanonicalFunction("Concat", string1, string2); } // ///and . /// Creates a /// An expression that specifies the string to search for any occurence ofthat invokes the canonical 'Contains' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// Boolean. /// . /// An expression that specifies the string to search for in . /// A new DbFunctionExpression that returns a Boolean value indicating whether or not ///occurs within . /// or is null. No overload of the canonical 'Contains' function accepts arguments with the result types of public static DbExpression Contains(this DbExpression searchedString, DbExpression searchedForString) { EntityUtil.CheckArgumentNull(searchedString, "searchedString"); EntityUtil.CheckArgumentNull(searchedForString, "searchedForString"); return InvokeCanonicalFunction("Contains", searchedString, searchedForString); } ///and . /// Creates a /// An expression that specifies the string to check for the specified . /// An expression that specifies the suffix for whichthat invokes the canonical 'EndsWith' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// Boolean. /// should be checked. /// A new DbFunctionExpression that indicates whether ///ends with . /// or is null. No overload of the canonical 'EndsWith' function accepts arguments with the result types of public static DbFunctionExpression EndsWith(this DbExpression stringArgument, DbExpression suffix) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(suffix, "suffix"); return InvokeCanonicalFunction("EndsWith", stringArgument, suffix); } ///and . /// Creates a ///that invokes the canonical 'IndexOf' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// Edm.Int32. /// The index returned by IndexOf is 1-based. /// An expression that specifies the string to search for. /// An expression that specifies the string to locate within should be checked. /// A new DbFunctionExpression that returns the first index of ///in . /// or is null. No overload of the canonical 'IndexOf' function accepts arguments with the result types of public static DbFunctionExpression IndexOf(this DbExpression searchString, DbExpression stringToFind) { EntityUtil.CheckArgumentNull(searchString, "searchString"); EntityUtil.CheckArgumentNull(stringToFind, "stringToFind"); return InvokeCanonicalFunction("IndexOf", stringToFind, searchString); } ///and . /// Creates a /// An expression that specifies the string from which to extract the leftmost substring. /// An expression that specifies the length of the leftmost substring to extract fromthat invokes the canonical 'Left' function with the /// specified arguments, which must have a string and integer numeric result type. The result type of the expression is /// string. /// . /// A new DbFunctionExpression that returns the the leftmost substring of length ///from . /// or is null. No overload of the canonical 'Left' function accepts arguments with the result types of public static DbFunctionExpression Left(this DbExpression stringArgument, DbExpression length) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(length, "length"); return InvokeCanonicalFunction("Left", stringArgument, length); } ///. /// Creates a /// An expression that specifies the string for which the length should be computed. ///that invokes the canonical 'Length' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns the the length of ///. /// is null. No overload of the canonical 'Length' function accepts an argument with the result type of public static DbFunctionExpression Length(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("Length", stringArgument); } ///. /// Creates a /// An expression that specifies the string in which to perform the replacement operation /// An expression that specifies the string to replace /// An expression that specifies the replacement string ///that invokes the canonical 'Replace' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression than returns a new string based on ///where every occurence of is replaced by . /// , or is null. No overload of the canonical 'Length' function accepts arguments with the result types of public static DbFunctionExpression Replace(this DbExpression stringArgument, DbExpression toReplace, DbExpression replacement) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(toReplace, "toReplace"); EntityUtil.CheckArgumentNull(replacement, "replacement"); return InvokeCanonicalFunction("Replace", stringArgument, toReplace, replacement); } ///, and . /// Creates a /// An expression that specifies the string to reverse. ///that invokes the canonical 'Reverse' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that produces the reversed value of ///. /// is null. No overload of the canonical 'Reverse' function accepts an argument with the result type of public static DbFunctionExpression Reverse(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("Reverse", stringArgument); } ///. /// Creates a /// An expression that specifies the string from which to extract the rightmost substring. /// An expression that specifies the length of the rightmost substring to extract fromthat invokes the canonical 'Right' function with the /// specified arguments, which must have a string and integer numeric result type. The result type of the expression is /// string. /// . /// A new DbFunctionExpression that returns the the rightmost substring of length ///from . /// or is null. No overload of the canonical 'Right' function accepts arguments with the result types of public static DbFunctionExpression Right(this DbExpression stringArgument, DbExpression length) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(length, "length"); return InvokeCanonicalFunction("Right", stringArgument, length); } ///. /// Creates a /// An expression that specifies the string to check for the specified . /// An expression that specifies the prefix for whichthat invokes the canonical 'StartsWith' function with the /// specified arguments, which must each have a string result type. The result type of the expression is /// Boolean. /// should be checked. /// A new DbFunctionExpression that indicates whether ///starts with . /// or is null. No overload of the canonical 'StartsWith' function accepts arguments with the result types of public static DbFunctionExpression StartsWith(this DbExpression stringArgument, DbExpression prefix) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(prefix, "prefix"); return InvokeCanonicalFunction("StartsWith", stringArgument, prefix); } // ///and . /// Creates a ///that invokes the canonical 'Substring' function with the /// specified arguments, which must have a string and integer numeric result types. The result type of the /// expression is string. /// Substring requires that the index specified by /// An expression that specifies the string from which to extract the substring. /// An expression that specifies the starting index from which the substring should be taken. /// An expression that specifies the length of the substring. ///be 1-based. A new DbFunctionExpression that returns the substring of length ///from starting at . /// , or is null. No overload of the canonical 'Substring' function accepts arguments with the result types of public static DbFunctionExpression Substring(this DbExpression stringArgument, DbExpression start, DbExpression length) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); EntityUtil.CheckArgumentNull(start, "start"); EntityUtil.CheckArgumentNull(length, "length"); return InvokeCanonicalFunction("Substring", stringArgument, start, length); } ///, and . /// Creates a /// An expression that specifies the string that should be converted to lower case. ///that invokes the canonical 'ToLower' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns value of ///converted to lower case. /// is null. No overload of the canonical 'ToLower' function accepts an argument with the result type of public static DbFunctionExpression ToLower(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("ToLower", stringArgument); } ///. /// Creates a /// An expression that specifies the string that should be converted to upper case. ///that invokes the canonical 'ToUpper' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns value of ///converted to upper case. /// is null. No overload of the canonical 'ToUpper' function accepts an argument with the result type of public static DbFunctionExpression ToUpper(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("ToUpper", stringArgument); } ///. /// Creates a /// An expression that specifies the string from which leading and trailing space should be removed. ///that invokes the canonical 'Trim' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns value of ///with leading and trailing space removed. /// is null. No overload of the canonical 'Trim' function accepts an argument with the result type of public static DbFunctionExpression Trim(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("Trim", stringArgument); } ///. /// Creates a /// An expression that specifies the string from which trailing space should be removed. ///that invokes the canonical 'RTrim' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns value of ///with trailing space removed. /// is null. No overload of the canonical 'RTrim' function accepts an argument with the result type of public static DbFunctionExpression TrimEnd(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("RTrim", stringArgument); } ///. /// Creates a /// An expression that specifies the string from which leading space should be removed. ///that invokes the canonical 'LTrim' function with the /// specified argument, which must have a string result type. The result type of the expression is /// also string. /// A new DbFunctionExpression that returns value of ///with leading space removed. /// is null. No overload of the canonical 'LTrim' function accepts an argument with the result type of public static DbFunctionExpression TrimStart(this DbExpression stringArgument) { EntityUtil.CheckArgumentNull(stringArgument, "stringArgument"); return InvokeCanonicalFunction("LTrim", stringArgument); } #endregion #region Date/Time member access methods - Year, Month, Day, DayOfYear, Hour, Minute, Second, Millisecond, GetTotalOffsetMinutes ///. /// Creates a /// An expression that specifies the value from which the year should be retrieved. ///that invokes the canonical 'Year' function with the /// specified argument, which must have a DateTime or DateTimeOffset result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer year value from ///. /// is null. No overload of the canonical 'Year' function accepts an argument with the result type of public static DbFunctionExpression Year(this DbExpression dateValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); return InvokeCanonicalFunction("Year", dateValue); } ///. /// Creates a /// An expression that specifies the value from which the month should be retrieved. ///that invokes the canonical 'Month' function with the /// specified argument, which must have a DateTime or DateTimeOffset result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer month value from ///. /// is null. No overload of the canonical 'Month' function accepts an argument with the result type of public static DbFunctionExpression Month(this DbExpression dateValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); return InvokeCanonicalFunction("Month", dateValue); } ///. /// Creates a /// An expression that specifies the value from which the day should be retrieved. ///that invokes the canonical 'Day' function with the /// specified argument, which must have a DateTime or DateTimeOffset result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer day value from ///. /// is null. No overload of the canonical 'Day' function accepts an argument with the result type of public static DbFunctionExpression Day(this DbExpression dateValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); return InvokeCanonicalFunction("Day", dateValue); } ///. /// Creates a /// An expression that specifies the value from which the day within the year should be retrieved. ///that invokes the canonical 'DayOfYear' function with the /// specified argument, which must have a DateTime or DateTimeOffset result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer day of year value from ///. /// is null. No overload of the canonical 'DayOfYear' function accepts an argument with the result type of public static DbFunctionExpression DayOfYear(this DbExpression dateValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); return InvokeCanonicalFunction("DayOfYear", dateValue); } ///. /// Creates a /// An expression that specifies the value from which the hour should be retrieved. ///that invokes the canonical 'Hour' function with the /// specified argument, which must have a DateTime, DateTimeOffset or Time result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer hour value from ///. /// is null. No overload of the canonical 'Hour' function accepts an argument with the result type of public static DbFunctionExpression Hour(this DbExpression timeValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); return InvokeCanonicalFunction("Hour", timeValue); } ///. /// Creates a /// An expression that specifies the value from which the minute should be retrieved. ///that invokes the canonical 'Minute' function with the /// specified argument, which must have a DateTime, DateTimeOffset or Time result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer minute value from ///. /// is null. No overload of the canonical 'Minute' function accepts an argument with the result type of public static DbFunctionExpression Minute(this DbExpression timeValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); return InvokeCanonicalFunction("Minute", timeValue); } ///. /// Creates a /// An expression that specifies the value from which the second should be retrieved. ///that invokes the canonical 'Second' function with the /// specified argument, which must have a DateTime, DateTimeOffset or Time result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer second value from ///. /// is null. No overload of the canonical 'Second' function accepts an argument with the result type of public static DbFunctionExpression Second(this DbExpression timeValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); return InvokeCanonicalFunction("Second", timeValue); } ///. /// Creates a /// An expression that specifies the value from which the millisecond should be retrieved. ///that invokes the canonical 'Millisecond' function with the /// specified argument, which must have a DateTime, DateTimeOffset or Time result type. The result type of /// the expression is Edm.Int32. /// A new DbFunctionExpression that returns the integer millisecond value from ///. /// is null. No overload of the canonical 'Millisecond' function accepts an argument with the result type of public static DbFunctionExpression Millisecond(this DbExpression timeValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); return InvokeCanonicalFunction("Millisecond", timeValue); } ///. /// Creates a /// An expression that specifies the DateTimeOffset value from which the minute offset from GMT should be retrieved. ///that invokes the canonical 'GetTotalOffsetMinutes' function with the /// specified argument, which must have a DateTimeOffset result type. The result type of the expression is Edm.Int32. /// A new DbFunctionExpression that returns the number of minutes ///is offset from GMT. /// is null. No overload of the canonical 'GetTotalOffsetMinutes' function accepts an argument with the result type of public static DbFunctionExpression GetTotalOffsetMinutes(this DbExpression dateTimeOffsetArgument) { EntityUtil.CheckArgumentNull(dateTimeOffsetArgument, "dateTimeOffsetArgument"); return InvokeCanonicalFunction("GetTotalOffsetMinutes", dateTimeOffsetArgument); } #endregion #region Date/Time creation methods - CurrentDateTime, CurrentDateTimeOffset, CurrentUtcDateTime, CreateDateTime, CreateDateTimeOffset, CreateTime, TruncateTime ///. /// Creates a ///that invokes the canonical 'CurrentDateTime' function. /// A new DbFunctionExpression that returns the current date and time as an Edm.DateTime instance. public static DbFunctionExpression CurrentDateTime() { return InvokeCanonicalFunction("CurrentDateTime"); } ////// Creates a ///that invokes the canonical 'CurrentDateTimeOffset' function. /// A new DbFunctionExpression that returns the current date and time as an Edm.DateTimeOffset instance. public static DbFunctionExpression CurrentDateTimeOffset() { return InvokeCanonicalFunction("CurrentDateTimeOffset"); } ////// Creates a ///that invokes the canonical 'CurrentUtcDateTime' function. /// A new DbFunctionExpression that returns the current UTC date and time as an Edm.DateTime instance. public static DbFunctionExpression CurrentUtcDateTime() { return InvokeCanonicalFunction("CurrentUtcDateTime"); } ////// Creates a /// An expression that specifies the value for which the time component should be truncated. ///that invokes the canonical 'TruncateTime' function with the /// specified argument, which must have a DateTime or DateTimeOffset result type. The result type of the /// expression is the same as the result type of . /// A new DbFunctionExpression that returns the value of ///with time set to zero. /// is null. No overload of the canonical 'TruncateTime' function accepts an argument with the result type of public static DbFunctionExpression TruncateTime(this DbExpression dateValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); return InvokeCanonicalFunction("TruncateTime", dateValue); } ///. /// Creates a /// An expression that provides the year component value for the new DateTime instance. /// An expression that provides the month component value for the new DateTime instance. /// An expression that provides the day component value for the new DateTime instance. /// An expression that provides the hour component value for the new DateTime instance. /// An expression that provides the minute component value for the new DateTime instance. /// An expression that provides the second component value for the new DateTime instance. ///that invokes the canonical 'CreateDateTime' function with the /// specified arguments. must have a result type of Edm.Double, while all other arguments /// must have a result type of Edm.Int32. The result type of the expression is Edm.DateTime. /// A new DbFunctionExpression that returns a new DateTime based on the specified component values. ////// , , , , , or is null. No overload of the canonical 'CreateDateTime' function accepts arguments with the result types of public static DbFunctionExpression CreateDateTime(DbExpression year, DbExpression month, DbExpression day, DbExpression hour, DbExpression minute, DbExpression second) { EntityUtil.CheckArgumentNull(year, "year"); EntityUtil.CheckArgumentNull(month, "month"); EntityUtil.CheckArgumentNull(day, "day"); EntityUtil.CheckArgumentNull(hour, "hour"); EntityUtil.CheckArgumentNull(minute, "minute"); EntityUtil.CheckArgumentNull(second, "second"); return InvokeCanonicalFunction("CreateDateTime", year, month, day, hour, minute, second); } ///, , , , , and . /// Creates a /// An expression that provides the year component value for the new DateTimeOffset instance. /// An expression that provides the month component value for the new DateTimeOffset instance. /// An expression that provides the day component value for the new DateTimeOffset instance. /// An expression that provides the hour component value for the new DateTimeOffset instance. /// An expression that provides the minute component value for the new DateTimeOffset instance. /// An expression that provides the second component value for the new DateTimeOffset instance. /// An expression that provides the number of minutes in the time zone offset component value for the new DateTimeOffset instance. ///that invokes the canonical 'CreateDateTimeOffset' function with the /// specified arguments. must have a result type of Edm.Double, while all other arguments /// must have a result type of Edm.Int32. The result type of the expression is Edm.DateTimeOffset. /// A new DbFunctionExpression that returns a new DateTimeOffset based on the specified component values. ////// , , , , , or is null. No overload of the canonical 'CreateDateTimeOffset' function accepts arguments with the result types of public static DbFunctionExpression CreateDateTimeOffset(DbExpression year, DbExpression month, DbExpression day, DbExpression hour, DbExpression minute, DbExpression second, DbExpression timeZoneOffset) { EntityUtil.CheckArgumentNull(year, "year"); EntityUtil.CheckArgumentNull(month, "month"); EntityUtil.CheckArgumentNull(day, "day"); EntityUtil.CheckArgumentNull(hour, "hour"); EntityUtil.CheckArgumentNull(minute, "minute"); EntityUtil.CheckArgumentNull(second, "second"); EntityUtil.CheckArgumentNull(timeZoneOffset, "timeZoneOffset"); return InvokeCanonicalFunction("CreateDateTimeOffset", year, month, day, hour, minute, second, timeZoneOffset); } ///, , , , , and . /// Creates a /// An expression that provides the hour component value for the new DateTime instance. /// An expression that provides the minute component value for the new DateTime instance. /// An expression that provides the second component value for the new DateTime instance. ///that invokes the canonical 'CreateTime' function with the /// specified arguments. must have a result type of Edm.Double, while all other arguments /// must have a result type of Edm.Int32. The result type of the expression is Edm.Time. /// A new DbFunctionExpression that returns a new Time based on the specified component values. ////// , , or is null. No overload of the canonical 'CreateTime' function accepts arguments with the result types of public static DbFunctionExpression CreateTime(DbExpression hour, DbExpression minute, DbExpression second) { EntityUtil.CheckArgumentNull(hour, "hour"); EntityUtil.CheckArgumentNull(minute, "minute"); EntityUtil.CheckArgumentNull(second, "second"); return InvokeCanonicalFunction("CreateTime", hour, minute, second); } #endregion #region Date/Time addition - AddYears, AddMonths, AddDays, AddHours, AddMinutes, AddSeconds, AddMilliseconds, AddMicroseconds, AddNanoseconds ///, , and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddYears' function with the /// specified arguments, which must have DateTime or DateTimeOffset and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of years to add to . /// A new DbFunctionExpression that adds the number of years specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddYears' function accepts arguments with the result types of public static DbFunctionExpression AddYears(this DbExpression dateValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddYears", dateValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddMonths' function with the /// specified arguments, which must have DateTime or DateTimeOffset and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of months to add to . /// A new DbFunctionExpression that adds the number of months specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddMonths' function accepts arguments with the result types of public static DbFunctionExpression AddMonths(this DbExpression dateValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddMonths", dateValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddDays' function with the /// specified arguments, which must have DateTime or DateTimeOffset and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of days to add to . /// A new DbFunctionExpression that adds the number of days specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddDays' function accepts arguments with the result types of public static DbFunctionExpression AddDays(this DbExpression dateValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(dateValue, "dateValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddDays", dateValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddHours' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of hours to add to . /// A new DbFunctionExpression that adds the number of hours specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddHours' function accepts arguments with the result types of public static DbFunctionExpression AddHours(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddHours", timeValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddMinutes' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of minutes to add to . /// A new DbFunctionExpression that adds the number of minutes specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddMinutes' function accepts arguments with the result types of public static DbFunctionExpression AddMinutes(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddMinutes", timeValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddSeconds' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of seconds to add to . /// A new DbFunctionExpression that adds the number of seconds specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddSeconds' function accepts arguments with the result types of public static DbFunctionExpression AddSeconds(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddSeconds", timeValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddMilliseconds' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of milliseconds to add to . /// A new DbFunctionExpression that adds the number of milliseconds specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddMilliseconds' function accepts arguments with the result types of public static DbFunctionExpression AddMilliseconds(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddMilliseconds", timeValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddMicroseconds' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of microseconds to add to . /// A new DbFunctionExpression that adds the number of microseconds specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddMicroseconds' function accepts arguments with the result types of public static DbFunctionExpression AddMicroseconds(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddMicroseconds", timeValue, addValue); } ///and . /// Creates a /// An expression that specifies the value to whichthat invokes the canonical 'AddNanoseconds' function with the /// specified arguments, which must have DateTime, DateTimeOffset or Time, and integer result types. The result /// type of the expression is the same as the result type of . /// should be added. /// An expression that specifies the number of nanoseconds to add to . /// A new DbFunctionExpression that adds the number of nanoseconds specified by ///to the value specified by . /// or is null. No overload of the canonical 'AddNanoseconds' function accepts arguments with the result types of public static DbFunctionExpression AddNanoseconds(this DbExpression timeValue, DbExpression addValue) { EntityUtil.CheckArgumentNull(timeValue, "timeValue"); EntityUtil.CheckArgumentNull(addValue, "addValue"); return InvokeCanonicalFunction("AddNanoseconds", timeValue, addValue); } #endregion #region Date/Time difference - DiffYears, DiffMonths, DiffDays, DiffHours, DiffMinutes, DiffSeconds, DiffMilliseconds, DiffMicroseconds, DiffNanoseconds ///and . /// Creates a /// An expression that specifies the first DateTime or DateTimeOffset value. /// An expression that specifies the DateTime or DateTimeOffset for which the year difference fromthat invokes the canonical 'DiffYears' function with the /// specified arguments, which must each have a DateTime or DateTimeOffset result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the year difference between and . ////// or is null. No overload of the canonical 'DiffYears' function accepts arguments with the result types of public static DbFunctionExpression DiffYears(this DbExpression dateValue1, DbExpression dateValue2) { EntityUtil.CheckArgumentNull(dateValue1, "dateValue1"); EntityUtil.CheckArgumentNull(dateValue2, "dateValue2"); return InvokeCanonicalFunction("DiffYears", dateValue1, dateValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime or DateTimeOffset value. /// An expression that specifies the DateTime or DateTimeOffset for which the month difference fromthat invokes the canonical 'DiffMonths' function with the /// specified arguments, which must each have a DateTime or DateTimeOffset result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the month difference between and . ////// or is null. No overload of the canonical 'DiffMonths' function accepts arguments with the result types of public static DbFunctionExpression DiffMonths(this DbExpression dateValue1, DbExpression dateValue2) { EntityUtil.CheckArgumentNull(dateValue1, "dateValue1"); EntityUtil.CheckArgumentNull(dateValue2, "dateValue2"); return InvokeCanonicalFunction("DiffMonths", dateValue1, dateValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime or DateTimeOffset value. /// An expression that specifies the DateTime or DateTimeOffset for which the day difference fromthat invokes the canonical 'DiffDays' function with the /// specified arguments, which must each have a DateTime or DateTimeOffset result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the day difference between and . ////// or is null. No overload of the canonical 'DiffDays' function accepts arguments with the result types of public static DbFunctionExpression DiffDays(this DbExpression dateValue1, DbExpression dateValue2) { EntityUtil.CheckArgumentNull(dateValue1, "dateValue1"); EntityUtil.CheckArgumentNull(dateValue2, "dateValue2"); return InvokeCanonicalFunction("DiffDays", dateValue1, dateValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the hour difference fromthat invokes the canonical 'DiffHours' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the hour difference between and . ////// or is null. No overload of the canonical 'DiffHours' function accepts arguments with the result types of public static DbFunctionExpression DiffHours(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffHours", timeValue1, timeValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the minute difference fromthat invokes the canonical 'DiffMinutes' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the minute difference between and . ////// or is null. No overload of the canonical 'DiffMinutes' function accepts arguments with the result types of public static DbFunctionExpression DiffMinutes(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffMinutes", timeValue1, timeValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the second difference fromthat invokes the canonical 'DiffSeconds' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the second difference between and . ////// or is null. No overload of the canonical 'DiffSeconds' function accepts arguments with the result types of public static DbFunctionExpression DiffSeconds(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffSeconds", timeValue1, timeValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the millisecond difference fromthat invokes the canonical 'DiffMilliseconds' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the millisecond difference between and . ////// or is null. No overload of the canonical 'DiffMilliseconds' function accepts arguments with the result types of public static DbFunctionExpression DiffMilliseconds(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffMilliseconds", timeValue1, timeValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the microsecond difference fromthat invokes the canonical 'DiffMicroseconds' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the microsecond difference between and . ////// or is null. No overload of the canonical 'DiffMicroseconds' function accepts arguments with the result types of public static DbFunctionExpression DiffMicroseconds(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffMicroseconds", timeValue1, timeValue2); } ///and . /// Creates a /// An expression that specifies the first DateTime, DateTimeOffset or Time value. /// An expression that specifies the DateTime, DateTimeOffset or Time for which the nanosecond difference fromthat invokes the canonical 'DiffNanoseconds' function with the /// specified arguments, which must each have a DateTime, DateTimeOffset or Time result type. The result type of /// must match the result type of . /// The result type of the expression is Edm.Int32. /// should be calculated. /// A new DbFunctionExpression that returns the nanosecond difference between and . ////// or is null. No overload of the canonical 'DiffNanoseconds' function accepts arguments with the result types of public static DbFunctionExpression DiffNanoseconds(this DbExpression timeValue1, DbExpression timeValue2) { EntityUtil.CheckArgumentNull(timeValue1, "timeValue1"); EntityUtil.CheckArgumentNull(timeValue2, "timeValue2"); return InvokeCanonicalFunction("DiffNanoseconds", timeValue1, timeValue2); } #endregion #region Math functions - Floor, Ceiling, Round, Truncate, Abs, Power ///and . /// Creates a /// An expression that specifies the numeric value to round. ///that invokes the canonical 'Round' function with the /// specified argument, which must each have a single, double or decimal result type. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that rounds the specified argument to the nearest integer value. ////// is null. No overload of the canonical 'Round' function accepts an argument with the result type of public static DbFunctionExpression Round(this DbExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return InvokeCanonicalFunction("Round", value); } ///. /// Creates a /// An expression that specifies the numeric value to round. /// An expression that specifies the number of digits of precision to use when rounding. ///that invokes the canonical 'Round' function with the /// specified arguments, which must have a single, double or decimal, and integer result types. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that rounds the specified argument to the nearest integer value, with precision as specified by ///. /// or is null. No overload of the canonical 'Round' function accepts arguments with the result types of public static DbFunctionExpression Round(this DbExpression value, DbExpression digits) { EntityUtil.CheckArgumentNull(value, "value"); EntityUtil.CheckArgumentNull(digits, "digits"); return InvokeCanonicalFunction("Round", value, digits); } ///and . /// Creates a /// An expression that specifies the numeric value. ///that invokes the canonical 'Floor' function with the /// specified argument, which must each have a single, double or decimal result type. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that returns the largest integer value not greater than ///. /// is null. No overload of the canonical 'Floor' function accepts an argument with the result type of public static DbFunctionExpression Floor(this DbExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return InvokeCanonicalFunction("Floor", value); } ///. /// Creates a /// An expression that specifies the numeric value. ///that invokes the canonical 'Ceiling' function with the /// specified argument, which must each have a single, double or decimal result type. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that returns the smallest integer value not less than than ///. /// is null. No overload of the canonical 'Ceiling' function accepts an argument with the result type of public static DbFunctionExpression Ceiling(this DbExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return InvokeCanonicalFunction("Ceiling", value); } ///. /// Creates a /// An expression that specifies the numeric value. ///that invokes the canonical 'Abs' function with the /// specified argument, which must each have a numeric result type. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that returns the absolute value of ///. /// is null. No overload of the canonical 'Abs' function accepts an argument with the result type of public static DbFunctionExpression Abs(this DbExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return InvokeCanonicalFunction("Abs", value); } ///. /// Creates a /// An expression that specifies the numeric value to truncate. /// An expression that specifies the number of digits of precision to use when truncating. ///that invokes the canonical 'Truncate' function with the /// specified arguments, which must have a single, double or decimal, and integer result types. The result /// type of the expression is the same as the result type of . /// A new DbFunctionExpression that truncates the specified argument to the nearest integer value, with precision as specified by ///. /// is null. No overload of the canonical 'Truncate' function accepts arguments with the result types of public static DbFunctionExpression Truncate(this DbExpression value, DbExpression digits) { EntityUtil.CheckArgumentNull(value, "value"); EntityUtil.CheckArgumentNull(digits, "digits"); return InvokeCanonicalFunction("Truncate", value, digits); } #endregion #endif ///and . /// Creates a /// An expression that specifies the numeric value to raise to the given power. /// An expression that specifies the power to whichthat invokes the canonical 'Power' function with the /// specified arguments, which must have numeric result types. The result type of the expression is /// the same as the result type of . /// should be raised. /// A new DbFunctionExpression that returns the value of ///raised to the power specified by . /// is null. No overload of the canonical 'Power' function accepts arguments with the result types of public static DbFunctionExpression Power(this DbExpression baseArgument, DbExpression exponent) { EntityUtil.CheckArgumentNull(baseArgument, "baseArgument"); EntityUtil.CheckArgumentNull(exponent, "exponent"); return InvokeCanonicalFunction("Power", baseArgument, exponent); } #if PUBLIC_DBEXPRESSIONBUILDER #region Bitwise functions - And, Or, Not, Xor ///and . /// Creates a /// An expression that specifies the first operand. /// An expression that specifies the second operand. ///that invokes the canonical 'BitwiseAnd' function with the /// specified arguments, which must have the same integer numeric result type. The result type of the /// expression is this same type. /// A new DbFunctionExpression that returns the value produced by performing the bitwise AND of ///and . /// is null. No overload of the canonical 'BitwiseAnd' function accepts arguments with the result types of public static DbFunctionExpression BitwiseAnd(this DbExpression value1, DbExpression value2) { EntityUtil.CheckArgumentNull(value1, "value1"); EntityUtil.CheckArgumentNull(value2, "value2"); return InvokeCanonicalFunction("BitwiseAnd", value1, value2); } ///and . /// Creates a /// An expression that specifies the first operand. /// An expression that specifies the second operand. ///that invokes the canonical 'BitwiseOr' function with the /// specified arguments, which must have the same integer numeric result type. The result type of the /// expression is this same type. /// A new DbFunctionExpression that returns the value produced by performing the bitwise OR of ///and . /// is null. No overload of the canonical 'BitwiseOr' function accepts arguments with the result types of public static DbFunctionExpression BitwiseOr(this DbExpression value1, DbExpression value2) { EntityUtil.CheckArgumentNull(value1, "value1"); EntityUtil.CheckArgumentNull(value2, "value2"); return InvokeCanonicalFunction("BitwiseOr", value1, value2); } ///and . /// Creates a /// An expression that specifies the first operand. ///that invokes the canonical 'BitwiseNot' function with the /// specified argument, which must have an integer numeric result type. The result type of the expression /// is this same type. /// A new DbFunctionExpression that returns the value produced by performing the bitwise NOT of ///. /// is null. No overload of the canonical 'BitwiseNot' function accepts an argument with the result type of public static DbFunctionExpression BitwiseNot(this DbExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return InvokeCanonicalFunction("BitwiseNot", value); } ///. /// Creates a /// An expression that specifies the first operand. /// An expression that specifies the second operand. ///that invokes the canonical 'BitwiseXor' function with the /// specified arguments, which must have the same integer numeric result type. The result type of the /// expression is this same type. /// A new DbFunctionExpression that returns the value produced by performing the bitwise XOR (exclusive OR) of ///and . /// is null. No overload of the canonical 'BitwiseXor' function accepts arguments with the result types of public static DbFunctionExpression BitwiseXor(this DbExpression value1, DbExpression value2) { EntityUtil.CheckArgumentNull(value1, "value1"); EntityUtil.CheckArgumentNull(value2, "value2"); return InvokeCanonicalFunction("BitwiseXor", value1, value2); } #endregion #endif #region GUID Generation - NewGuid ///and . /// Creates a ///that invokes the canonical 'NewGuid' function. /// A new DbFunctionExpression that returns a new GUID value. public static DbFunctionExpression NewGuid() { return InvokeCanonicalFunction("NewGuid"); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
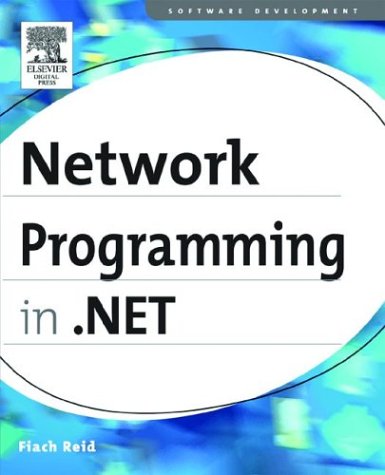
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NativeMethodsOther.cs
- WebMessageEncodingBindingElement.cs
- ChameleonKey.cs
- XmlSchemaAny.cs
- CodeDomConfigurationHandler.cs
- SerializationEventsCache.cs
- entityreference_tresulttype.cs
- DataBoundControlHelper.cs
- LogicalExpressionTypeConverter.cs
- VisualStateGroup.cs
- SafeMemoryMappedViewHandle.cs
- KeySpline.cs
- SymbolTable.cs
- EnumUnknown.cs
- PointF.cs
- ExpressionEvaluator.cs
- RowType.cs
- DictionaryBase.cs
- BatchParser.cs
- DecimalConstantAttribute.cs
- IdentityHolder.cs
- SchemaTableColumn.cs
- BindingsCollection.cs
- PostBackOptions.cs
- TransformCollection.cs
- BitConverter.cs
- SynchronizationHandlesCodeDomSerializer.cs
- TreeNodeSelectionProcessor.cs
- SQLDoubleStorage.cs
- ConnectionsZoneDesigner.cs
- PageThemeCodeDomTreeGenerator.cs
- IdentityReference.cs
- SelfIssuedSamlTokenFactory.cs
- SettingsProviderCollection.cs
- webclient.cs
- TextOnlyOutput.cs
- OleDbCommandBuilder.cs
- ProfessionalColorTable.cs
- ImageBrush.cs
- ComPlusInstanceContextInitializer.cs
- PrintPreviewDialog.cs
- Authorization.cs
- XmlWriterDelegator.cs
- GeneralTransform.cs
- BitmapDecoder.cs
- OdbcHandle.cs
- Empty.cs
- Fx.cs
- UIElement3D.cs
- EncodingInfo.cs
- ControlTemplate.cs
- RecordBuilder.cs
- HasCopySemanticsAttribute.cs
- AttributeCollection.cs
- NaturalLanguageHyphenator.cs
- AnnotationStore.cs
- DataFormat.cs
- NTAccount.cs
- QuaternionAnimation.cs
- FormViewDeletedEventArgs.cs
- SmiXetterAccessMap.cs
- EnumMember.cs
- StatementContext.cs
- ResourcesBuildProvider.cs
- ArraySegment.cs
- SkinBuilder.cs
- MatchingStyle.cs
- TokenBasedSet.cs
- ThreadInterruptedException.cs
- _RequestCacheProtocol.cs
- ComponentEditorPage.cs
- ComboBoxRenderer.cs
- Queue.cs
- XmlSerializerNamespaces.cs
- RegexRunner.cs
- BoundColumn.cs
- SizeFConverter.cs
- ScriptIgnoreAttribute.cs
- PasswordTextNavigator.cs
- BitmapData.cs
- TextSearch.cs
- CompositeDesignerAccessibleObject.cs
- RenderDataDrawingContext.cs
- GridViewCommandEventArgs.cs
- ClientScriptItemCollection.cs
- ControlType.cs
- ListItemConverter.cs
- MdImport.cs
- DictionaryBase.cs
- shaperfactoryquerycachekey.cs
- DataColumn.cs
- XmlSchemaAnnotation.cs
- ContextQuery.cs
- FrameDimension.cs
- ToolStripButton.cs
- KeyToListMap.cs
- StreamResourceInfo.cs
- ACE.cs
- VectorAnimation.cs
- DesignTimeResourceProviderFactoryAttribute.cs