Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / ImageButton.cs / 1305376 / ImageButton.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Drawing.Design; using System.Globalization; using System.Web; using System.Web.UI; using System.Web.Util; ////// [ DefaultEvent("Click"), Designer("System.Web.UI.Design.WebControls.PreviewControlDesigner, " + AssemblyRef.SystemDesign), SupportsEventValidation, ] public class ImageButton : Image, IPostBackDataHandler, IPostBackEventHandler, IButtonControl { private static readonly object EventClick = new object(); private static readonly object EventButtonClick = new object(); private static readonly object EventCommand = new object(); private int x = 0; private int y = 0; ///Creates a control that displays an image, responds to mouse clicks, /// and records the mouse pointer position. ////// public ImageButton() { } ///Initializes a new instance of the ///class. /// [ DefaultValue(""), WebCategory("Behavior"), WebSysDescription(SR.WebControl_CommandName), Themeable(false), ] public string CommandName { get { string s = (string)ViewState["CommandName"]; return((s == null) ? String.Empty : s); } set { ViewState["CommandName"] = value; } } ///Gets or sets the command associated with the ///that is propogated in the event along with the /// property. /// [ Bindable(true), DefaultValue(""), Themeable(false), WebCategory("Behavior"), WebSysDescription(SR.WebControl_CommandArgument), ] public string CommandArgument { get { string s = (string)ViewState["CommandArgument"]; return((s == null) ? String.Empty : s); } set { ViewState["CommandArgument"] = value; } } ///Gets or sets an optional argument that is propogated in /// the ///event with the associated /// /// property. /// [ Themeable(false), DefaultValue(true), WebCategory("Behavior"), WebSysDescription(SR.Button_CausesValidation) ] public virtual bool CausesValidation { get { object b = ViewState["CausesValidation"]; return((b == null) ? true : (bool)b); } set { ViewState["CausesValidation"] = value; } } [ Browsable(true), EditorBrowsableAttribute(EditorBrowsableState.Always), Bindable(true), WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.WebControl_Enabled) ] public override bool Enabled { get { return base.Enabled; } set { base.Enabled = value; } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never), Themeable(false), ] public override bool GenerateEmptyAlternateText { get { return base.GenerateEmptyAlternateText; } set { throw new NotSupportedException(SR.GetString( SR.Property_Set_Not_Supported, "GenerateEmptyAlternateText", this.GetType().ToString())); } } ///Gets or sets whether pressing the button causes page validation to fire. This defaults to True so that when /// using validation controls, the validation state of all controls are updated when the button is clicked, both /// on the client and the server. Setting this to False is useful when defining a cancel or reset button on a page /// that has validators. ////// The script that is executed on a client-side click. /// [ DefaultValue(""), WebCategory("Behavior"), WebSysDescription(SR.Button_OnClientClick), Themeable(false), ] public virtual string OnClientClick { get { string s = (string)ViewState["OnClientClick"]; if (s == null) { return String.Empty; } return s; } set { ViewState["OnClientClick"] = value; } } [ DefaultValue(""), Editor("System.Web.UI.Design.UrlEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), Themeable(false), UrlProperty("*.aspx"), WebCategory("Behavior"), WebSysDescription(SR.Button_PostBackUrl), ] public virtual string PostBackUrl { get { string s = (string)ViewState["PostBackUrl"]; return s == null? String.Empty : s; } set { ViewState["PostBackUrl"] = value; } } public override bool SupportsDisabledAttribute { get { return true; } } ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] protected override HtmlTextWriterTag TagKey { get { return HtmlTextWriterTag.Input; } } [ WebCategory("Behavior"), Themeable(false), DefaultValue(""), WebSysDescription(SR.PostBackControl_ValidationGroup) ] public virtual string ValidationGroup { get { string s = (string)ViewState["ValidationGroup"]; return((s == null) ? String.Empty : s); } set { ViewState["ValidationGroup"] = value; } } ///Gets a value that represents the tag HtmlTextWriterTag.Input. This property is read-only. ////// [ WebCategory("Action"), WebSysDescription(SR.ImageButton_OnClick) ] public event ImageClickEventHandler Click { add { Events.AddHandler(EventClick, value); } remove { Events.RemoveHandler(EventClick, value); } } ///Represents the method that will handle the ///event of an . /// event EventHandler IButtonControl.Click { add { Events.AddHandler(EventButtonClick, value); } remove { Events.RemoveHandler(EventButtonClick, value); } } ///Represents the method that will handle the ///event of an . /// [ WebCategory("Action"), WebSysDescription(SR.ImageButton_OnCommand) ] public event CommandEventHandler Command { add { Events.AddHandler(EventCommand, value); } remove { Events.RemoveHandler(EventCommand, value); } } ///Represents the method that will handle the ///event of an . /// /// protected override void AddAttributesToRender(HtmlTextWriter writer) { Page page = Page; // Make sure we are in a form tag with runat=server. if (page != null) { page.VerifyRenderingInServerForm(this); } writer.AddAttribute(HtmlTextWriterAttribute.Type,"image"); string uniqueID = UniqueID; PostBackOptions options = GetPostBackOptions(); // Don't render Name on a button if __doPostBack is posting back to a different control // because Page will register this control as requiring post back notification even though // it's not the target of the postback. If the TargetControl isn't this control, this control's // RaisePostBackEvent should never get called. See VSWhidbey 477095. if (uniqueID != null && (options == null || options.TargetControl == this)) { writer.AddAttribute(HtmlTextWriterAttribute.Name, uniqueID); } // bool effectiveEnabled = IsEnabled; string onClick = String.Empty; if (effectiveEnabled) { // Need to merge the onclick attribute with the OnClientClick onClick = Util.EnsureEndWithSemiColon(OnClientClick); if (HasAttributes) { string userOnClick = Attributes["onclick"]; if (userOnClick != null) { onClick += Util.EnsureEndWithSemiColon(userOnClick); Attributes.Remove("onclick"); } } } if (Enabled && !effectiveEnabled && SupportsDisabledAttribute) { // We need to do the cascade effect on the server, because the browser // only renders as disabled, but doesn't disable the functionality. writer.AddAttribute(HtmlTextWriterAttribute.Disabled, "disabled"); } base.AddAttributesToRender(writer); if (page != null && options != null) { page.ClientScript.RegisterForEventValidation(options); if (effectiveEnabled) { string postBackEventReference = page.ClientScript.GetPostBackEventReference(options, false); if (!String.IsNullOrEmpty(postBackEventReference)) { onClick = Util.MergeScript(onClick, postBackEventReference); if (options.ClientSubmit) { // Dev10 Bugs 584471 // a derived control may return PostBackOptions with ClientSubmit=true, // such as DataControlImageButton. Without a return false, there would be // a double submit. onClick = Util.EnsureEndWithSemiColon(onClick) + "return false;"; } } } } if (onClick.Length > 0) { writer.AddAttribute(HtmlTextWriterAttribute.Onclick, onClick); if (EnableLegacyRendering) { writer.AddAttribute("language", "javascript", false); } } } // Returns the client post back options. protected virtual PostBackOptions GetPostBackOptions() { PostBackOptions options = new PostBackOptions(this, String.Empty); // The postback script ends up in onclick, not href, // so we shouldn't force the javascript protocol like we do in LinkButton. options.ClientSubmit = false; if (!String.IsNullOrEmpty(PostBackUrl)) { options.ActionUrl = HttpUtility.UrlPathEncode(ResolveClientUrl(PostBackUrl)); } if (CausesValidation && Page != null && Page.GetValidators(ValidationGroup).Count > 0) { options.PerformValidation = true; options.ValidationGroup = ValidationGroup; } return options; } ///Adds the attributes of an ///to the output /// stream for rendering on the client. /// /// bool IPostBackDataHandler.LoadPostData(string postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } ///Processes posted data for the ///control. /// /// protected virtual bool LoadPostData(string postDataKey, NameValueCollection postCollection) { string name = UniqueID; string postX = postCollection[name + ".x"]; string postY = postCollection[name + ".y"]; if (postX != null && postY != null && postX.Length > 0 && postY.Length > 0) { x = Int32.Parse(postX, CultureInfo.InvariantCulture); y = Int32.Parse(postY, CultureInfo.InvariantCulture); if (Page != null) { Page.RegisterRequiresRaiseEvent(this); } } return false; } ///Processes posted data for the ///control. /// protected virtual void OnClick(ImageClickEventArgs e) { ImageClickEventHandler onClickHandler = (ImageClickEventHandler)Events[EventClick]; if (onClickHandler != null) onClickHandler(this,e); EventHandler onButtonClickHandler = (EventHandler)Events[EventButtonClick]; if (onButtonClickHandler != null) onButtonClickHandler(this,(EventArgs)e); } ///Raises the ///event. /// protected virtual void OnCommand(CommandEventArgs e) { CommandEventHandler onCommandHandler = (CommandEventHandler)Events[EventCommand]; if (onCommandHandler != null) onCommandHandler(this,e); // Command events are bubbled up the control heirarchy RaiseBubbleEvent(this, e); } ///Raises the ///event. /// /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null) { Page.RegisterRequiresPostBack(this); if (IsEnabled && ((CausesValidation && Page.GetValidators(ValidationGroup).Count > 0) || !String.IsNullOrEmpty(PostBackUrl))) { Page.RegisterWebFormsScript(); // VSWhidbey 489577 } } } ////// Determine /// if the image has been clicked prior to rendering on the client. ////// /// void IPostBackEventHandler.RaisePostBackEvent(string eventArgument) { RaisePostBackEvent(eventArgument); } ///Raises events on post back for the ////// control. /// /// protected virtual void RaisePostBackEvent(string eventArgument) { ValidateEvent(this.UniqueID, eventArgument); if (CausesValidation) { Page.Validate(ValidationGroup); } OnClick(new ImageClickEventArgs(x,y)); OnCommand(new CommandEventArgs(CommandName, CommandArgument)); } ///Raises events on post back for the ////// control. /// /// Raised when posted data for a control has changed. /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ////// /// Raised when posted data for a control has changed. /// protected virtual void RaisePostDataChangedEvent() { } ////// /// IButtonControl.Text implementation. IButtonControl is used internally for adaptive rendering, but /// property is explicitly implemented because the developer should use AlternateText, as in V1. /// string IButtonControl.Text { get { return Text; } set { Text = value; } } ////// /// IButtonControl.Text implementation. IButtonControl is used internally for adaptive rendering, but /// property is explicitly implemented because the developer should use AlternateText, as in V1. /// protected virtual string Text { get { return AlternateText; } set { AlternateText = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Drawing.Design; using System.Globalization; using System.Web; using System.Web.UI; using System.Web.Util; ////// [ DefaultEvent("Click"), Designer("System.Web.UI.Design.WebControls.PreviewControlDesigner, " + AssemblyRef.SystemDesign), SupportsEventValidation, ] public class ImageButton : Image, IPostBackDataHandler, IPostBackEventHandler, IButtonControl { private static readonly object EventClick = new object(); private static readonly object EventButtonClick = new object(); private static readonly object EventCommand = new object(); private int x = 0; private int y = 0; ///Creates a control that displays an image, responds to mouse clicks, /// and records the mouse pointer position. ////// public ImageButton() { } ///Initializes a new instance of the ///class. /// [ DefaultValue(""), WebCategory("Behavior"), WebSysDescription(SR.WebControl_CommandName), Themeable(false), ] public string CommandName { get { string s = (string)ViewState["CommandName"]; return((s == null) ? String.Empty : s); } set { ViewState["CommandName"] = value; } } ///Gets or sets the command associated with the ///that is propogated in the event along with the /// property. /// [ Bindable(true), DefaultValue(""), Themeable(false), WebCategory("Behavior"), WebSysDescription(SR.WebControl_CommandArgument), ] public string CommandArgument { get { string s = (string)ViewState["CommandArgument"]; return((s == null) ? String.Empty : s); } set { ViewState["CommandArgument"] = value; } } ///Gets or sets an optional argument that is propogated in /// the ///event with the associated /// /// property. /// [ Themeable(false), DefaultValue(true), WebCategory("Behavior"), WebSysDescription(SR.Button_CausesValidation) ] public virtual bool CausesValidation { get { object b = ViewState["CausesValidation"]; return((b == null) ? true : (bool)b); } set { ViewState["CausesValidation"] = value; } } [ Browsable(true), EditorBrowsableAttribute(EditorBrowsableState.Always), Bindable(true), WebCategory("Behavior"), DefaultValue(true), WebSysDescription(SR.WebControl_Enabled) ] public override bool Enabled { get { return base.Enabled; } set { base.Enabled = value; } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never), Themeable(false), ] public override bool GenerateEmptyAlternateText { get { return base.GenerateEmptyAlternateText; } set { throw new NotSupportedException(SR.GetString( SR.Property_Set_Not_Supported, "GenerateEmptyAlternateText", this.GetType().ToString())); } } ///Gets or sets whether pressing the button causes page validation to fire. This defaults to True so that when /// using validation controls, the validation state of all controls are updated when the button is clicked, both /// on the client and the server. Setting this to False is useful when defining a cancel or reset button on a page /// that has validators. ////// The script that is executed on a client-side click. /// [ DefaultValue(""), WebCategory("Behavior"), WebSysDescription(SR.Button_OnClientClick), Themeable(false), ] public virtual string OnClientClick { get { string s = (string)ViewState["OnClientClick"]; if (s == null) { return String.Empty; } return s; } set { ViewState["OnClientClick"] = value; } } [ DefaultValue(""), Editor("System.Web.UI.Design.UrlEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), Themeable(false), UrlProperty("*.aspx"), WebCategory("Behavior"), WebSysDescription(SR.Button_PostBackUrl), ] public virtual string PostBackUrl { get { string s = (string)ViewState["PostBackUrl"]; return s == null? String.Empty : s; } set { ViewState["PostBackUrl"] = value; } } public override bool SupportsDisabledAttribute { get { return true; } } ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] protected override HtmlTextWriterTag TagKey { get { return HtmlTextWriterTag.Input; } } [ WebCategory("Behavior"), Themeable(false), DefaultValue(""), WebSysDescription(SR.PostBackControl_ValidationGroup) ] public virtual string ValidationGroup { get { string s = (string)ViewState["ValidationGroup"]; return((s == null) ? String.Empty : s); } set { ViewState["ValidationGroup"] = value; } } ///Gets a value that represents the tag HtmlTextWriterTag.Input. This property is read-only. ////// [ WebCategory("Action"), WebSysDescription(SR.ImageButton_OnClick) ] public event ImageClickEventHandler Click { add { Events.AddHandler(EventClick, value); } remove { Events.RemoveHandler(EventClick, value); } } ///Represents the method that will handle the ///event of an . /// event EventHandler IButtonControl.Click { add { Events.AddHandler(EventButtonClick, value); } remove { Events.RemoveHandler(EventButtonClick, value); } } ///Represents the method that will handle the ///event of an . /// [ WebCategory("Action"), WebSysDescription(SR.ImageButton_OnCommand) ] public event CommandEventHandler Command { add { Events.AddHandler(EventCommand, value); } remove { Events.RemoveHandler(EventCommand, value); } } ///Represents the method that will handle the ///event of an . /// /// protected override void AddAttributesToRender(HtmlTextWriter writer) { Page page = Page; // Make sure we are in a form tag with runat=server. if (page != null) { page.VerifyRenderingInServerForm(this); } writer.AddAttribute(HtmlTextWriterAttribute.Type,"image"); string uniqueID = UniqueID; PostBackOptions options = GetPostBackOptions(); // Don't render Name on a button if __doPostBack is posting back to a different control // because Page will register this control as requiring post back notification even though // it's not the target of the postback. If the TargetControl isn't this control, this control's // RaisePostBackEvent should never get called. See VSWhidbey 477095. if (uniqueID != null && (options == null || options.TargetControl == this)) { writer.AddAttribute(HtmlTextWriterAttribute.Name, uniqueID); } // bool effectiveEnabled = IsEnabled; string onClick = String.Empty; if (effectiveEnabled) { // Need to merge the onclick attribute with the OnClientClick onClick = Util.EnsureEndWithSemiColon(OnClientClick); if (HasAttributes) { string userOnClick = Attributes["onclick"]; if (userOnClick != null) { onClick += Util.EnsureEndWithSemiColon(userOnClick); Attributes.Remove("onclick"); } } } if (Enabled && !effectiveEnabled && SupportsDisabledAttribute) { // We need to do the cascade effect on the server, because the browser // only renders as disabled, but doesn't disable the functionality. writer.AddAttribute(HtmlTextWriterAttribute.Disabled, "disabled"); } base.AddAttributesToRender(writer); if (page != null && options != null) { page.ClientScript.RegisterForEventValidation(options); if (effectiveEnabled) { string postBackEventReference = page.ClientScript.GetPostBackEventReference(options, false); if (!String.IsNullOrEmpty(postBackEventReference)) { onClick = Util.MergeScript(onClick, postBackEventReference); if (options.ClientSubmit) { // Dev10 Bugs 584471 // a derived control may return PostBackOptions with ClientSubmit=true, // such as DataControlImageButton. Without a return false, there would be // a double submit. onClick = Util.EnsureEndWithSemiColon(onClick) + "return false;"; } } } } if (onClick.Length > 0) { writer.AddAttribute(HtmlTextWriterAttribute.Onclick, onClick); if (EnableLegacyRendering) { writer.AddAttribute("language", "javascript", false); } } } // Returns the client post back options. protected virtual PostBackOptions GetPostBackOptions() { PostBackOptions options = new PostBackOptions(this, String.Empty); // The postback script ends up in onclick, not href, // so we shouldn't force the javascript protocol like we do in LinkButton. options.ClientSubmit = false; if (!String.IsNullOrEmpty(PostBackUrl)) { options.ActionUrl = HttpUtility.UrlPathEncode(ResolveClientUrl(PostBackUrl)); } if (CausesValidation && Page != null && Page.GetValidators(ValidationGroup).Count > 0) { options.PerformValidation = true; options.ValidationGroup = ValidationGroup; } return options; } ///Adds the attributes of an ///to the output /// stream for rendering on the client. /// /// bool IPostBackDataHandler.LoadPostData(string postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } ///Processes posted data for the ///control. /// /// protected virtual bool LoadPostData(string postDataKey, NameValueCollection postCollection) { string name = UniqueID; string postX = postCollection[name + ".x"]; string postY = postCollection[name + ".y"]; if (postX != null && postY != null && postX.Length > 0 && postY.Length > 0) { x = Int32.Parse(postX, CultureInfo.InvariantCulture); y = Int32.Parse(postY, CultureInfo.InvariantCulture); if (Page != null) { Page.RegisterRequiresRaiseEvent(this); } } return false; } ///Processes posted data for the ///control. /// protected virtual void OnClick(ImageClickEventArgs e) { ImageClickEventHandler onClickHandler = (ImageClickEventHandler)Events[EventClick]; if (onClickHandler != null) onClickHandler(this,e); EventHandler onButtonClickHandler = (EventHandler)Events[EventButtonClick]; if (onButtonClickHandler != null) onButtonClickHandler(this,(EventArgs)e); } ///Raises the ///event. /// protected virtual void OnCommand(CommandEventArgs e) { CommandEventHandler onCommandHandler = (CommandEventHandler)Events[EventCommand]; if (onCommandHandler != null) onCommandHandler(this,e); // Command events are bubbled up the control heirarchy RaiseBubbleEvent(this, e); } ///Raises the ///event. /// /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null) { Page.RegisterRequiresPostBack(this); if (IsEnabled && ((CausesValidation && Page.GetValidators(ValidationGroup).Count > 0) || !String.IsNullOrEmpty(PostBackUrl))) { Page.RegisterWebFormsScript(); // VSWhidbey 489577 } } } ////// Determine /// if the image has been clicked prior to rendering on the client. ////// /// void IPostBackEventHandler.RaisePostBackEvent(string eventArgument) { RaisePostBackEvent(eventArgument); } ///Raises events on post back for the ////// control. /// /// protected virtual void RaisePostBackEvent(string eventArgument) { ValidateEvent(this.UniqueID, eventArgument); if (CausesValidation) { Page.Validate(ValidationGroup); } OnClick(new ImageClickEventArgs(x,y)); OnCommand(new CommandEventArgs(CommandName, CommandArgument)); } ///Raises events on post back for the ////// control. /// /// Raised when posted data for a control has changed. /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ////// /// Raised when posted data for a control has changed. /// protected virtual void RaisePostDataChangedEvent() { } ////// /// IButtonControl.Text implementation. IButtonControl is used internally for adaptive rendering, but /// property is explicitly implemented because the developer should use AlternateText, as in V1. /// string IButtonControl.Text { get { return Text; } set { Text = value; } } ////// /// IButtonControl.Text implementation. IButtonControl is used internally for adaptive rendering, but /// property is explicitly implemented because the developer should use AlternateText, as in V1. /// protected virtual string Text { get { return AlternateText; } set { AlternateText = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
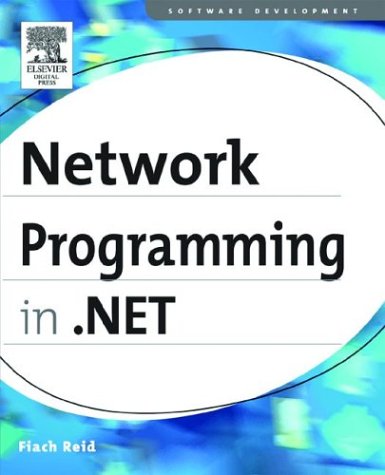
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsNonControl.cs
- adornercollection.cs
- BamlWriter.cs
- SizeConverter.cs
- ADMembershipProvider.cs
- EventBookmark.cs
- StringDictionaryWithComparer.cs
- MetadataSource.cs
- VerificationAttribute.cs
- StreamUpgradeInitiator.cs
- WebServiceEnumData.cs
- TextServicesCompartment.cs
- SystemSounds.cs
- Menu.cs
- RawStylusActions.cs
- PerformanceCounterPermissionAttribute.cs
- MergablePropertyAttribute.cs
- ProtocolsConfigurationEntry.cs
- BoolExpr.cs
- QuaternionAnimationBase.cs
- HuffModule.cs
- Rijndael.cs
- ConfigurationLockCollection.cs
- FamilyTypeface.cs
- EntityExpressionVisitor.cs
- MasterPageParser.cs
- Margins.cs
- NegatedCellConstant.cs
- HandledEventArgs.cs
- ReadOnlyHierarchicalDataSourceView.cs
- WebPartUserCapability.cs
- PrePrepareMethodAttribute.cs
- TokenCreationParameter.cs
- TextServicesManager.cs
- hwndwrapper.cs
- WebBrowserSiteBase.cs
- Camera.cs
- GZipDecoder.cs
- DLinqTableProvider.cs
- DBCommandBuilder.cs
- XmlText.cs
- KeyGesture.cs
- GlyphsSerializer.cs
- ListChangedEventArgs.cs
- SymbolPair.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- GCHandleCookieTable.cs
- ConditionalAttribute.cs
- ImageButton.cs
- ControlHelper.cs
- PropertyGridDesigner.cs
- RuntimeHelpers.cs
- PolyBezierSegmentFigureLogic.cs
- DataGridHeadersVisibilityToVisibilityConverter.cs
- DecoderBestFitFallback.cs
- InternalControlCollection.cs
- OrderPreservingSpoolingTask.cs
- EasingKeyFrames.cs
- SecurityResources.cs
- UdpAnnouncementEndpoint.cs
- path.cs
- EventItfInfo.cs
- Crypto.cs
- HwndAppCommandInputProvider.cs
- ModuleBuilder.cs
- SafeFreeMibTable.cs
- PointValueSerializer.cs
- JobPageOrder.cs
- ObjectListCommandsPage.cs
- activationcontext.cs
- ArgumentOutOfRangeException.cs
- PrimitiveType.cs
- GroupBox.cs
- ReversePositionQuery.cs
- BrushMappingModeValidation.cs
- CatalogZone.cs
- Symbol.cs
- XmlSyndicationContent.cs
- IsolatedStorage.cs
- TranslateTransform3D.cs
- WorkflowQueueInfo.cs
- SiteMapDataSourceView.cs
- StylusEventArgs.cs
- FontStyle.cs
- WsatStrings.cs
- ScriptServiceAttribute.cs
- InlineUIContainer.cs
- AssociatedControlConverter.cs
- LongValidator.cs
- ConnectionPoint.cs
- InheritanceContextChangedEventManager.cs
- HttpCachePolicyElement.cs
- TrackingAnnotationCollection.cs
- StyleModeStack.cs
- SchemaConstraints.cs
- EventLogger.cs
- VisualBrush.cs
- Figure.cs
- XsdValidatingReader.cs
- TreeViewTemplateSelector.cs