Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Stylus / StylusEventArgs.cs / 1305600 / StylusEventArgs.cs
using System; using System.Collections; using System.Windows.Media; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// The StylusEventArgs class provides access to the logical /// Stylus device for all derived event args. /// public class StylusEventArgs : InputEventArgs { ///////////////////////////////////////////////////////////////////// ////// Initializes a new instance of the StylusEventArgs class. /// /// /// The logical Stylus device associated with this event. /// /// /// The time when the input occured. /// public StylusEventArgs(StylusDevice stylus, int timestamp) : base(stylus, timestamp) { if( stylus == null ) { throw new System.ArgumentNullException("stylus"); } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to the stylus device associated with this /// event. /// public StylusDevice StylusDevice { get { return (StylusDevice)this.Device; } } ///////////////////////////////////////////////////////////////////// ////// Calculates the position of the stylus relative to a particular element. /// public Point GetPosition(IInputElement relativeTo) { return StylusDevice.GetPosition(relativeTo); } ///////////////////////////////////////////////////////////////////// ////// Indicates the stylus is not touching the surface. /// public bool InAir { get { return StylusDevice.InAir; } } ///////////////////////////////////////////////////////////////////// ////// Indicates stylusDevice is in the inverted state. /// public bool Inverted { get { return StylusDevice.Inverted; } } ///////////////////////////////////////////////////////////////////// ////// Returns a StylusPointCollection for processing the data from input. /// This method creates a new StylusPointCollection and copies the data. /// public StylusPointCollection GetStylusPoints(IInputElement relativeTo) { return StylusDevice.GetStylusPoints(relativeTo); } ///////////////////////////////////////////////////////////////////// ////// Returns a StylusPointCollection for processing the data from input. /// This method creates a new StylusPointCollection and copies the data. /// public StylusPointCollection GetStylusPoints(IInputElement relativeTo, StylusPointDescription subsetToReformatTo) { return StylusDevice.GetStylusPoints(relativeTo, subsetToReformatTo); } ///////////////////////////////////////////////////////////////////// ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { StylusEventHandler handler = (StylusEventHandler) genericHandler; handler(genericTarget, this); } ///////////////////////////////////////////////////////////////////// internal RawStylusInputReport InputReport { get { return _inputReport; } set { _inputReport = value; } } ///////////////////////////////////////////////////////////////////// RawStylusInputReport _inputReport; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
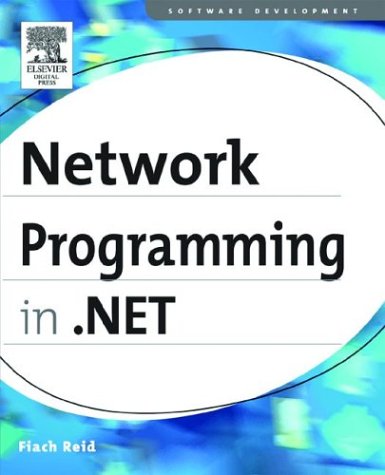
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InvalidCastException.cs
- Parameter.cs
- RelatedImageListAttribute.cs
- NonClientArea.cs
- KeyboardNavigation.cs
- ElementFactory.cs
- DataRow.cs
- SecurityPermission.cs
- XmlSchema.cs
- GrammarBuilderDictation.cs
- AnnouncementService.cs
- FontUnit.cs
- SqlDataReaderSmi.cs
- EnterpriseServicesHelper.cs
- WebPartActionVerb.cs
- HotSpot.cs
- SqlConnectionFactory.cs
- LogFlushAsyncResult.cs
- TextModifierScope.cs
- XmlNamedNodeMap.cs
- ProgressBarBrushConverter.cs
- SerializationHelper.cs
- RuntimeCompatibilityAttribute.cs
- UnicodeEncoding.cs
- SimpleMailWebEventProvider.cs
- NativeRightsManagementAPIsStructures.cs
- ListBoxChrome.cs
- Fonts.cs
- Emitter.cs
- LongPath.cs
- DeflateEmulationStream.cs
- UrlAuthFailedErrorFormatter.cs
- PaginationProgressEventArgs.cs
- LinkedResourceCollection.cs
- BookmarkTable.cs
- DataGridViewCellEventArgs.cs
- HyperLinkStyle.cs
- BuilderInfo.cs
- EntityCommandCompilationException.cs
- TagMapInfo.cs
- WindowsImpersonationContext.cs
- Brush.cs
- DetailsViewInsertedEventArgs.cs
- EmptyQuery.cs
- WebRequestModuleElementCollection.cs
- TextWriter.cs
- ProviderConnectionPointCollection.cs
- DesignerView.xaml.cs
- NativeMethods.cs
- WebPartRestoreVerb.cs
- ITextView.cs
- Registry.cs
- DataGridViewButtonCell.cs
- WebPartDisplayModeCollection.cs
- PasswordDeriveBytes.cs
- CodeRemoveEventStatement.cs
- ImageBrush.cs
- SpellerError.cs
- SrgsSemanticInterpretationTag.cs
- CultureSpecificStringDictionary.cs
- HttpSessionStateBase.cs
- SerializerWriterEventHandlers.cs
- GZipStream.cs
- DataGridViewCellStyleChangedEventArgs.cs
- XPathNode.cs
- Color.cs
- GridViewHeaderRowPresenter.cs
- SetStoryboardSpeedRatio.cs
- GeometryDrawing.cs
- DataRecordInfo.cs
- PointCollectionValueSerializer.cs
- TypeValidationEventArgs.cs
- X509Certificate2Collection.cs
- Section.cs
- DesignerPerfEventProvider.cs
- TrackingParticipant.cs
- HostingEnvironmentException.cs
- ConfigurationManager.cs
- SlipBehavior.cs
- AssemblyBuilderData.cs
- FilterEventArgs.cs
- DesignerSerializationOptionsAttribute.cs
- Int32Rect.cs
- Translator.cs
- OutputCacheModule.cs
- MailWebEventProvider.cs
- StreamSecurityUpgradeInitiatorBase.cs
- RowType.cs
- ValueSerializer.cs
- XmlSchemaIdentityConstraint.cs
- RectangleF.cs
- HttpCookieCollection.cs
- References.cs
- RequestResizeEvent.cs
- UnitControl.cs
- SqlUDTStorage.cs
- ServiceBuildProvider.cs
- CharacterHit.cs
- PackageRelationshipCollection.cs
- login.cs