Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / ControlFilterExpression.cs / 1305376 / ControlFilterExpression.cs
using System.Collections.Generic; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Linq; using System.Linq.Expressions; using System.Web.Resources; using System.Web.UI; using System.Web.UI.WebControls; #if ORYX_VNEXT using Microsoft.Web.Data.UI.WebControls.Expressions; using Microsoft.Web.Data.UI.WebControls; #else using System.Web.UI.WebControls.Expressions; #endif namespace System.Web.DynamicData { ////// A Dynamic Data-specific implementation of DataSourceExpression that modifies an IQueryable based on a data key (selected row) /// in a data bound controls such as GridView, ListView, DetailsView, or FormView. /// If the Column property is left empty, the control treats the data key as the primary key of current table (this is useful /// in a List-Details scenario where the databound control and the datasource are displaying items of the same type). If the /// Column property is not empty, this control treats the data key as a foreign key (this is useful in a Parent-Children scenario, /// where the databound control is displaying a list of Categories, and the data source is to be filtered to only display the /// Products that belong to the selected Category). /// public class ControlFilterExpression : DataSourceExpression { private PropertyExpression _propertyExpression; ////// The ID of a data-bound control such as a GridView, ListView, DetailsView, or FormView whose data key will be used to build /// the expression that gets used in a QueryExtender. /// [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID", Justification = "The property refers to the ID property of a Control")] public string ControlID { get; set; } ////// Optional property which when set indicates that the data key should be treated as a foreign key. /// public string Column { get; set; } private PropertyExpression Expression { get { if (_propertyExpression == null) { _propertyExpression = new PropertyExpression(); } return _propertyExpression; } } public override void SetContext(Control owner, HttpContext context, IQueryableDataSource dataSource) { base.SetContext(owner, context, dataSource); Owner.Page.InitComplete += new EventHandler(Page_InitComplete); Owner.Page.LoadComplete += new EventHandler(Page_LoadComplete); } private void Page_InitComplete(object sender, EventArgs e) { if (!Owner.Page.IsPostBack) { // Do not reconfigure the Expression on postback. It's values should be preserved via ViewState. Control control = FindTargetControl(); MetaTable table = DataSource.GetMetaTable(); if (String.IsNullOrEmpty(Column)) { foreach (var param in GetPrimaryKeyControlParameters(control, table)) { Expression.Parameters.Add(param); } } else { MetaForeignKeyColumn column = (MetaForeignKeyColumn)table.GetColumn(Column); foreach (var param in GetForeignKeyControlParameters(control, column)) { Expression.Parameters.Add(param); } } } Expression.SetContext(Owner, Context, DataSource); } private Control FindTargetControl() { Control control = Misc.FindControl(Owner, ControlID); if (control == null) { throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture, DynamicDataResources.ControlFilterExpression_CouldNotFindControlID, Owner.ID, ControlID)); } return control; } private void Page_LoadComplete(object sender, EventArgs e) { Expression.Parameters.UpdateValues(Context, Owner); } protected override object SaveViewState() { Pair p = new Pair(); p.First = base.SaveViewState(); p.Second = ((IStateManager)Expression.Parameters).SaveViewState(); return p; } protected override void LoadViewState(object savedState) { Pair p = (Pair)savedState; base.LoadViewState(p.First); if (p.Second != null) { ((IStateManager)Expression.Parameters).LoadViewState(p.Second); } } protected override void TrackViewState() { base.TrackViewState(); ((IStateManager)Expression.Parameters).TrackViewState(); } private IEnumerableGetPrimaryKeyControlParameters(Control control, MetaTable table) { // For each PK column in the table, we need to create a ControlParameter var nameColumnMapping = table.PrimaryKeyColumns.ToDictionary(c => c.Name); return GetControlParameters(control, nameColumnMapping); } private IEnumerable GetForeignKeyControlParameters(Control control, MetaForeignKeyColumn column) { // For each underlying FK, we need to create a ControlParameter MetaTable otherTable = column.ParentTable; Dictionary nameColumnMapping = CreateColumnMapping(column, otherTable.PrimaryKeyColumns); return GetControlParameters(control, nameColumnMapping); } private static Dictionary CreateColumnMapping(MetaForeignKeyColumn column, IList columns) { var names = column.ForeignKeyNames; Debug.Assert(names.Count == columns.Count); Dictionary nameColumnMapping = new Dictionary (); for (int i = 0; i < names.Count; i++) { // Get the filter expression for this foreign key name string filterExpression = column.GetFilterExpression(names[i]); nameColumnMapping[filterExpression] = columns[i]; } return nameColumnMapping; } internal static IEnumerable GetControlParameters(Control control, IDictionary nameColumnMapping) { IControlParameterTarget target = null; target = DynamicDataManager.GetControlParameterTarget(control); Debug.Assert(target != null); foreach (var entry in nameColumnMapping) { string parameterName = entry.Key; MetaColumn column = entry.Value; ControlParameter controlParameter = new ControlParameter() { Name = parameterName, ControlID = control.UniqueID }; if (target != null) { // this means the relationship consists of more than one key and we need to expand the property name controlParameter.PropertyName = target.GetPropertyNameExpression(column.Name); } DataSourceUtil.SetParameterTypeCodeAndDbType(controlParameter, column); yield return controlParameter; } } public override IQueryable GetQueryable(IQueryable source) { return Expression.GetQueryable(source); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Collections.Generic; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Linq; using System.Linq.Expressions; using System.Web.Resources; using System.Web.UI; using System.Web.UI.WebControls; #if ORYX_VNEXT using Microsoft.Web.Data.UI.WebControls.Expressions; using Microsoft.Web.Data.UI.WebControls; #else using System.Web.UI.WebControls.Expressions; #endif namespace System.Web.DynamicData { /// /// A Dynamic Data-specific implementation of DataSourceExpression that modifies an IQueryable based on a data key (selected row) /// in a data bound controls such as GridView, ListView, DetailsView, or FormView. /// If the Column property is left empty, the control treats the data key as the primary key of current table (this is useful /// in a List-Details scenario where the databound control and the datasource are displaying items of the same type). If the /// Column property is not empty, this control treats the data key as a foreign key (this is useful in a Parent-Children scenario, /// where the databound control is displaying a list of Categories, and the data source is to be filtered to only display the /// Products that belong to the selected Category). /// public class ControlFilterExpression : DataSourceExpression { private PropertyExpression _propertyExpression; ////// The ID of a data-bound control such as a GridView, ListView, DetailsView, or FormView whose data key will be used to build /// the expression that gets used in a QueryExtender. /// [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID", Justification = "The property refers to the ID property of a Control")] public string ControlID { get; set; } ////// Optional property which when set indicates that the data key should be treated as a foreign key. /// public string Column { get; set; } private PropertyExpression Expression { get { if (_propertyExpression == null) { _propertyExpression = new PropertyExpression(); } return _propertyExpression; } } public override void SetContext(Control owner, HttpContext context, IQueryableDataSource dataSource) { base.SetContext(owner, context, dataSource); Owner.Page.InitComplete += new EventHandler(Page_InitComplete); Owner.Page.LoadComplete += new EventHandler(Page_LoadComplete); } private void Page_InitComplete(object sender, EventArgs e) { if (!Owner.Page.IsPostBack) { // Do not reconfigure the Expression on postback. It's values should be preserved via ViewState. Control control = FindTargetControl(); MetaTable table = DataSource.GetMetaTable(); if (String.IsNullOrEmpty(Column)) { foreach (var param in GetPrimaryKeyControlParameters(control, table)) { Expression.Parameters.Add(param); } } else { MetaForeignKeyColumn column = (MetaForeignKeyColumn)table.GetColumn(Column); foreach (var param in GetForeignKeyControlParameters(control, column)) { Expression.Parameters.Add(param); } } } Expression.SetContext(Owner, Context, DataSource); } private Control FindTargetControl() { Control control = Misc.FindControl(Owner, ControlID); if (control == null) { throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture, DynamicDataResources.ControlFilterExpression_CouldNotFindControlID, Owner.ID, ControlID)); } return control; } private void Page_LoadComplete(object sender, EventArgs e) { Expression.Parameters.UpdateValues(Context, Owner); } protected override object SaveViewState() { Pair p = new Pair(); p.First = base.SaveViewState(); p.Second = ((IStateManager)Expression.Parameters).SaveViewState(); return p; } protected override void LoadViewState(object savedState) { Pair p = (Pair)savedState; base.LoadViewState(p.First); if (p.Second != null) { ((IStateManager)Expression.Parameters).LoadViewState(p.Second); } } protected override void TrackViewState() { base.TrackViewState(); ((IStateManager)Expression.Parameters).TrackViewState(); } private IEnumerableGetPrimaryKeyControlParameters(Control control, MetaTable table) { // For each PK column in the table, we need to create a ControlParameter var nameColumnMapping = table.PrimaryKeyColumns.ToDictionary(c => c.Name); return GetControlParameters(control, nameColumnMapping); } private IEnumerable GetForeignKeyControlParameters(Control control, MetaForeignKeyColumn column) { // For each underlying FK, we need to create a ControlParameter MetaTable otherTable = column.ParentTable; Dictionary nameColumnMapping = CreateColumnMapping(column, otherTable.PrimaryKeyColumns); return GetControlParameters(control, nameColumnMapping); } private static Dictionary CreateColumnMapping(MetaForeignKeyColumn column, IList columns) { var names = column.ForeignKeyNames; Debug.Assert(names.Count == columns.Count); Dictionary nameColumnMapping = new Dictionary (); for (int i = 0; i < names.Count; i++) { // Get the filter expression for this foreign key name string filterExpression = column.GetFilterExpression(names[i]); nameColumnMapping[filterExpression] = columns[i]; } return nameColumnMapping; } internal static IEnumerable GetControlParameters(Control control, IDictionary nameColumnMapping) { IControlParameterTarget target = null; target = DynamicDataManager.GetControlParameterTarget(control); Debug.Assert(target != null); foreach (var entry in nameColumnMapping) { string parameterName = entry.Key; MetaColumn column = entry.Value; ControlParameter controlParameter = new ControlParameter() { Name = parameterName, ControlID = control.UniqueID }; if (target != null) { // this means the relationship consists of more than one key and we need to expand the property name controlParameter.PropertyName = target.GetPropertyNameExpression(column.Name); } DataSourceUtil.SetParameterTypeCodeAndDbType(controlParameter, column); yield return controlParameter; } } public override IQueryable GetQueryable(IQueryable source) { return Expression.GetQueryable(source); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
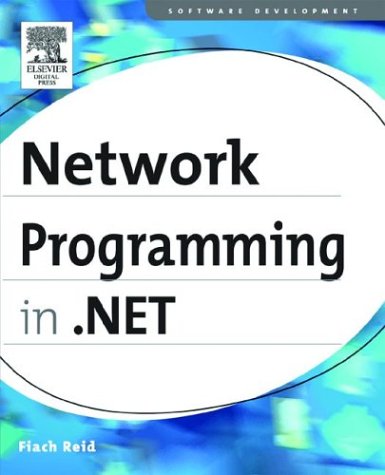
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Symbol.cs
- OverrideMode.cs
- ErrorRuntimeConfig.cs
- ResolveNameEventArgs.cs
- DispatcherObject.cs
- ClientProxyGenerator.cs
- SqlNotificationRequest.cs
- GetPageCompletedEventArgs.cs
- IisTraceListener.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- SqlBooleanMismatchVisitor.cs
- ActivitySurrogate.cs
- sqlmetadatafactory.cs
- ExpressionHelper.cs
- CompilerCollection.cs
- SingleAnimation.cs
- ApplicationProxyInternal.cs
- KeyTime.cs
- BulletedListEventArgs.cs
- XmlSerializerVersionAttribute.cs
- RegexBoyerMoore.cs
- Task.cs
- BufferBuilder.cs
- TranslateTransform3D.cs
- ToolStripGripRenderEventArgs.cs
- ConfigurationFileMap.cs
- Helpers.cs
- StoreContentChangedEventArgs.cs
- TaskExceptionHolder.cs
- WebPartCollection.cs
- ConfigPathUtility.cs
- ParamArrayAttribute.cs
- FormView.cs
- DBNull.cs
- XmlElement.cs
- WeakReferenceEnumerator.cs
- PrivateUnsafeNativeCompoundFileMethods.cs
- Stream.cs
- SrgsNameValueTag.cs
- VerticalAlignConverter.cs
- SqlDelegatedTransaction.cs
- TabControl.cs
- WebPartCatalogCloseVerb.cs
- GenericWebPart.cs
- httpapplicationstate.cs
- BuilderPropertyEntry.cs
- Win32SafeHandles.cs
- PersonalizationProvider.cs
- FileDialogCustomPlaces.cs
- ArithmeticException.cs
- ModifierKeysValueSerializer.cs
- ActivitySurrogateSelector.cs
- PropertyStore.cs
- PrintDialog.cs
- PasswordBoxAutomationPeer.cs
- ResizingMessageFilter.cs
- SqlInternalConnectionTds.cs
- PtsHost.cs
- SourceSwitch.cs
- TraceContextRecord.cs
- SystemTcpConnection.cs
- DocumentGrid.cs
- SafeRightsManagementSessionHandle.cs
- AsymmetricKeyExchangeFormatter.cs
- SortDescription.cs
- DisplayMemberTemplateSelector.cs
- OleStrCAMarshaler.cs
- DrawingCollection.cs
- DrawListViewSubItemEventArgs.cs
- BitmapFrameDecode.cs
- CodeCastExpression.cs
- NamespaceDecl.cs
- StringKeyFrameCollection.cs
- KeyValueConfigurationCollection.cs
- EventHandlingScope.cs
- HttpCapabilitiesEvaluator.cs
- AutoScrollExpandMessageFilter.cs
- RelOps.cs
- MessagingDescriptionAttribute.cs
- DataObjectCopyingEventArgs.cs
- AssemblyInfo.cs
- BoolExpression.cs
- NavigatorInput.cs
- SchemaComplexType.cs
- TagPrefixAttribute.cs
- NumberSubstitution.cs
- StandardToolWindows.cs
- ServiceContractGenerator.cs
- SubstitutionList.cs
- XpsSerializationManager.cs
- NotCondition.cs
- LocatorPartList.cs
- ServiceOperationWrapper.cs
- XmlReflectionMember.cs
- TypeConverter.cs
- TreeNodeConverter.cs
- MethodImplAttribute.cs
- DataGridViewRowsRemovedEventArgs.cs
- LoginName.cs
- RefreshPropertiesAttribute.cs