Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / ChannelManager.cs / 1 / ChannelManager.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The ChannelManager is a helper structure internal to MediaContext // that abstracts out channel creation, storage and destruction. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using System.Windows.Threading; using System.Windows; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using MS.Internal; using MS.Utility; using MS.Win32; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods.MilCoreApi; namespace System.Windows.Media { partial class MediaContext { ////// A helper structure that abstracts channel management. /// ////// The common agreement (avcomptm, avpttrev) is that channel creation /// should be considered unsafe and therefore subject to control via /// the SecurityCritical/SecurityTreatAsSafe mechanism. /// /// On the other hand, using a channel that has been created by the /// MediaContext is considered safe. /// /// This class expresses the above policy by hiding channel creation /// and storage within the MediaContext while allowing to access /// the channels at will. /// private struct ChannelManager { ////// Opens an asynchronous channel. /// ////// Critical - Creates a channel, calls a Channel method, calls methods performing elevations. /// TreatAsSafe - It is safe for the media context to create and use a channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void CreateChannels(bool hasLayeredWindows) { Invariant.Assert(_asyncChannel == null); Invariant.Assert(_asyncOutOfBandChannel == null); // Create a channel into the async composition device. // Pass in a reference to the global mediasystem channel so that it uses // the same partition. _asyncChannel = new DUCE.Channel( System.Windows.Media.MediaSystem.ServiceChannel, false, // not out of band false, // not a layered window specific channel System.Windows.Media.MediaSystem.Connection, IntPtr.Zero // sync transport ); _asyncOutOfBandChannel = new DUCE.Channel( System.Windows.Media.MediaSystem.ServiceChannel, true, // out of band false, // not a layered window specific channel System.Windows.Media.MediaSystem.Connection, IntPtr.Zero // sync transport ); if (hasLayeredWindows && _asyncChannel.MarshalType == ChannelMarshalType.ChannelMarshalTypeCrossMachine) { CreateLocalAsynchronousChannels(); } } ////// Closes all opened channels. /// ////// Critical - Closes channels. /// TreatAsSafe - It is safe for the media context to sync channels channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void RemoveSyncChannels() { // // Close the synchronous channels. // if (_freeSyncChannels != null) { while (_freeSyncChannels.Count > 0) { _freeSyncChannels.Dequeue().Close(); } _freeSyncChannels = null; } if (_syncServiceChannel != null) { _syncServiceChannel.Close(); _syncServiceChannel = null; } } ////// Closes all opened channels. /// ////// Critical - Closes channels. /// TreatAsSafe - It is safe for the media context to close a channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void RemoveChannels() { if (_asyncChannel != null) { _asyncChannel.Close(); _asyncChannel = null; } if (_asyncOutOfBandChannel != null) { _asyncOutOfBandChannel.Close(); _asyncOutOfBandChannel = null; } if (_localAsynchronousChannel != null) { _localAsynchronousChannel.Close(); _localAsynchronousChannel = null; } if (_localAsynchronousOutOfBandChannel != null) { _localAsynchronousOutOfBandChannel.Close(); _localAsynchronousOutOfBandChannel = null; } RemoveSyncChannels(); if (_pSyncConnection != IntPtr.Zero) { HRESULT.Check(UnsafeNativeMethods.MilTransport_DisconnectTransport( _pSyncConnection)); _pSyncConnection = IntPtr.Zero; } } ////// Create a fresh or fetch one from the pool synchronous channel. /// ////// Critical - Creates and returns a synchronous channel. /// TreatAsSafe - Using a channel created by the media context is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal DUCE.Channel AllocateSyncChannel() { DUCE.Channel syncChannel; if (_pSyncConnection == IntPtr.Zero) { _pSyncConnection = System.Windows.Media.MediaSystem.ConnectSyncTransport( out _pSyncPacketTransport ); } if (_freeSyncChannels == null) { // // Ensure the free sync channels queue... // _freeSyncChannels = new Queue(3); } if (_freeSyncChannels.Count > 0) { // // If there is a free sync channel in the queue, we're done: // return _freeSyncChannels.Dequeue(); } else { // // Otherwise, create a new channel. We will try to cache it // when the ReleaseSyncChannel call is made. Also ensure the // synchronous service channel and glyph cache. // if (_syncServiceChannel == null) { _syncServiceChannel = new DUCE.Channel( null, false, // not out of band false, // not a layered window specific channel _pSyncConnection, _pSyncPacketTransport ); } syncChannel = new DUCE.Channel( _syncServiceChannel, false, // not out of band false, // not a layered window specific channel _pSyncConnection, _pSyncPacketTransport ); return syncChannel; } } /// /// Returns a sync channel back to the pool. /// ////// Critical - Creates and returns a synchronous channel. /// TreatAsSafe - Closing a channel is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal void ReleaseSyncChannel(DUCE.Channel channel) { Invariant.Assert(_freeSyncChannels != null); // // A decision needs to be made whether or not we're interested // in re-using this channel later. For now, store up to three // synchronous channel in the queue. // if (_freeSyncChannels.Count <= 3) { // // Since we don't close the channel, verify that // the RemoveAndReleaseQueue is empty for resources. // Debug.Assert(channel.IsRemoveAndReleaseQueueEmpty()); _freeSyncChannels.Enqueue(channel); } else { channel.Close(); } } ////// Flushes the remove and release queues of all channels /// ////// Critical - Performs commands on channels. /// TreatAsSafe - Flushing commands that have already been enqueued is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal void FlushAllRemoveReleaseQueues() { FlushRemoveAndReleaseQueues(_asyncChannel); FlushRemoveAndReleaseQueues(_asyncOutOfBandChannel); FlushRemoveAndReleaseQueues(_localAsynchronousChannel); FlushRemoveAndReleaseQueues(_localAsynchronousOutOfBandChannel); } ////// Private helper function to flush the remove and release queues of a channel /// ////// Critical - Performs commands on channels. /// [SecurityCritical] private void FlushRemoveAndReleaseQueues(DUCE.Channel channel) { if (channel != null) { channel.ProcessRemoveCommand(); channel.ProcessReleaseCommand(); channel.ClearRemoveAndReleaseQueue(); } } ////// Returns the asynchronous channel. /// ////// Critical - Channels are a controlled unmanaged resource. /// TreatAsSafe - Using a channel created by the media context is safe. /// internal DUCE.Channel Channel { [SecurityCritical, SecurityTreatAsSafe] get { return _asyncChannel; } } ////// Returns the asynchronous out-of-band channel. /// ////// Critical - Channels are a controlled unmanaged resource. /// TreatAsSafe - Using a channel created by the media context is safe. /// internal DUCE.Channel OutOfBandChannel { [SecurityCritical, SecurityTreatAsSafe] get { return _asyncOutOfBandChannel; } } ////// Get the local channel that this target is on. /// ////// Critical - Channels are a controlled unmanaged resource. /// TreatAsSafe - Using a channel created by the media context is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal DUCE.Channel LocalAsynchronousChannel { [SecurityCritical, SecurityTreatAsSafe] get { return _localAsynchronousChannel; } } ////// Get the local channel out of band that this target is on. /// ////// Critical - Channels are a controlled unmanaged resource. /// TreatAsSafe - Using a channel created by the media context is safe. /// [SecurityCritical, SecurityTreatAsSafe] internal DUCE.Channel LocalAsynchronousOutOfBandChannel { [SecurityCritical, SecurityTreatAsSafe] get { return _localAsynchronousOutOfBandChannel; } } ////// Opens an asynchronous channel on a local transport. This is to allow /// layered windows to be correctly rendered over TS. /// ////// Critical - Creates a channel, calls a Channel method, calls methods performing elevations. /// TreatAsSafe - It is safe for the media context to create and use a channel. /// [SecurityCritical, SecurityTreatAsSafe] internal void CreateLocalAsynchronousChannels() { Invariant.Assert(_localAsynchronousChannel == null); Invariant.Assert(_localAsynchronousOutOfBandChannel == null); _localAsynchronousChannel = new DUCE.Channel( null, false, // not out of band true, // is a layered window specific channel MediaSystem.LocalTransport, IntPtr.Zero ); _localAsynchronousOutOfBandChannel = new DUCE.Channel( _localAsynchronousChannel, true, // out of band true, // is a layered window specific channel System.Windows.Media.MediaSystem.LocalTransport, IntPtr.Zero ); } ////// The asynchronous channel. /// ////// This field will be replaced with an asynchronous channel table as per task #26681. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _asyncChannel; ////// The asynchronous out-of-band channel. /// ////// This is needed by CompositionTarget for handling WM_PAINT, etc. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _asyncOutOfBandChannel; ////// We store the free synchronous channels for later re-use in this queue. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private Queue_freeSyncChannels; /// /// The service channel to the synchronous partition. /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _syncServiceChannel; ////// Pointer to the unmanaged transport object. /// ////// Critical - Controlled unmanaged resource. /// [SecurityCritical] private IntPtr _pSyncPacketTransport; ////// Pointer to the unmanaged connection object. /// ////// Critical - Controlled unmanaged resource. /// [SecurityCritical] private IntPtr _pSyncConnection; ////// The asynchronous local channel channel /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _localAsynchronousChannel; ////// The asynchronous local out-of-band channel /// ////// Critical - Channels are a controlled unmanaged resource. /// [SecurityCritical] private DUCE.Channel _localAsynchronousOutOfBandChannel; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
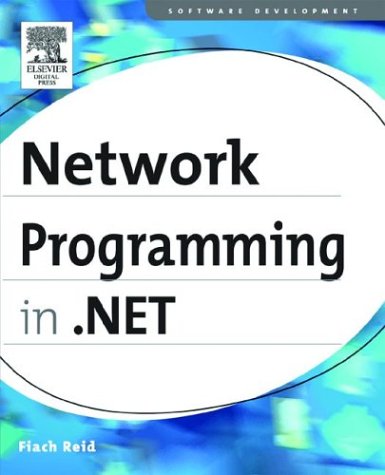
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FrameworkReadOnlyPropertyMetadata.cs
- PagedControl.cs
- DataGridPagerStyle.cs
- CfgArc.cs
- EventHandlerList.cs
- DefaultExpression.cs
- Listener.cs
- XmlSchemaImport.cs
- TextTreeInsertElementUndoUnit.cs
- Stylesheet.cs
- AssemblyCollection.cs
- Attribute.cs
- DrawingAttributesDefaultValueFactory.cs
- PersonalizableAttribute.cs
- WriteFileContext.cs
- WmpBitmapDecoder.cs
- DiffuseMaterial.cs
- GridViewRow.cs
- MorphHelper.cs
- NotImplementedException.cs
- ResourcesBuildProvider.cs
- HttpServerChannel.cs
- XmlSchemaChoice.cs
- TimeZone.cs
- UIElement3D.cs
- PrintController.cs
- LineMetrics.cs
- ContentHostHelper.cs
- ModelItem.cs
- UpdatePanelTrigger.cs
- DeleteHelper.cs
- ProcessingInstructionAction.cs
- AuthenticationService.cs
- UserControlFileEditor.cs
- ClientProxyGenerator.cs
- Compensate.cs
- RtfToXamlLexer.cs
- DisplayInformation.cs
- CqlLexerHelpers.cs
- NativeWindow.cs
- WmpBitmapDecoder.cs
- EntityDataSourceMemberPath.cs
- WebPartConnectionsCancelVerb.cs
- DataSourceCacheDurationConverter.cs
- IssuanceLicense.cs
- Serializer.cs
- SQLDecimal.cs
- GatewayIPAddressInformationCollection.cs
- TextFormatterImp.cs
- ApplicationTrust.cs
- WebPartEditorCancelVerb.cs
- _Connection.cs
- DocobjHost.cs
- SoapExtensionReflector.cs
- OdbcHandle.cs
- FlowDocumentReaderAutomationPeer.cs
- XmlAttribute.cs
- VBCodeProvider.cs
- ComplexObject.cs
- XmlAttributeHolder.cs
- ContainerUtilities.cs
- TextContainerChangeEventArgs.cs
- PropertyRef.cs
- StateInitializationDesigner.cs
- WebBrowser.cs
- SyndicationItem.cs
- sqlinternaltransaction.cs
- NameValuePermission.cs
- FeatureSupport.cs
- PageAsyncTaskManager.cs
- TiffBitmapDecoder.cs
- RSACryptoServiceProvider.cs
- BidirectionalDictionary.cs
- RotateTransform.cs
- OleDbDataAdapter.cs
- ByteAnimationUsingKeyFrames.cs
- TransformCollection.cs
- HistoryEventArgs.cs
- MatrixKeyFrameCollection.cs
- DurationConverter.cs
- Light.cs
- SmiMetaData.cs
- TextContainerChangedEventArgs.cs
- ArrangedElementCollection.cs
- ToolboxComponentsCreatedEventArgs.cs
- DocumentReference.cs
- ScopelessEnumAttribute.cs
- ShaperBuffers.cs
- BuildManager.cs
- WsatConfiguration.cs
- SecondaryViewProvider.cs
- XmlDocumentType.cs
- DefaultHttpHandler.cs
- TableCellCollection.cs
- PartialTrustVisibleAssembly.cs
- TemplateControlCodeDomTreeGenerator.cs
- PageCache.cs
- ProxyAssemblyNotLoadedException.cs
- SafeLocalMemHandle.cs
- AvTraceFormat.cs