Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Query / PlanCompiler / PropertyRef.cs / 1305376 / PropertyRef.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; //using System.Diagnostics; // Please use PlanCompiler.Assert instead of Debug.Assert in this class... using System.Globalization; using System.Data.Common; using md = System.Data.Metadata.Edm; // // The PropertyRef class (and its subclasses) represent references to a property // of a type. // The PropertyRefList class represents a list of expected properties // where each property from the type is described as a PropertyRef // // These classes are used by the StructuredTypeEliminator module as part of // eliminating all structured types. The basic idea of this module is that all // structured types are flattened out into a single level. To avoid a large amount // of potentially unnecessary information, we try to identify what pieces of information // are really necessary at each node of the tree. This is where PropertyRef comes in. // A PropertyRef (and more generally, a PropertyRefList) identifies a list of // properties, and can be attached to a node/var to indicate that these were the // only desired properties. // namespace System.Data.Query.PlanCompiler { ////// A PropertyRef class encapsulates a reference to one or more properties of /// a complex instance - a record type, a complex type or an entity type. /// A PropertyRef may be of the following kinds. /// - a simple property reference (just a reference to a simple property) /// - a typeid reference - applies only to entitytype and complextypes /// - an entitysetid reference - applies only to ref and entity types /// - a nested property reference (a reference to a nested property - a.b) /// - an "all" property reference (all properties) /// internal abstract class PropertyRef { ////// trivial constructor /// internal PropertyRef() { } ////// Create a nested property ref, with "p" as the prefix. /// The best way to think of this function as follows. /// Consider a type T where "this" describes a property X on T. Now /// consider a new type S, where "p" is a property of S and is of type T. /// This function creates a PropertyRef that describes the same property X /// from S.p instead /// /// the property to prefix with ///the nested property reference internal virtual PropertyRef CreateNestedPropertyRef(PropertyRef p) { return new NestedPropertyRef(p, this); } ////// Create a nested property ref for a simple property. Delegates to the function /// above /// /// the simple property ///a nestedPropertyRef internal PropertyRef CreateNestedPropertyRef(md.EdmMember p) { return CreateNestedPropertyRef(new SimplePropertyRef(p)); } ////// Creates a nested property ref for a rel-property. Delegates to the function above /// /// the rel-property ///a nested property ref internal PropertyRef CreateNestedPropertyRef(InternalTrees.RelProperty p) { return CreateNestedPropertyRef(new RelPropertyRef(p)); } ////// The tostring method for easy debuggability /// ///public override string ToString() { return ""; } } /// /// A "simple" property ref - represents a simple property of the type /// internal class SimplePropertyRef : PropertyRef { private md.EdmMember m_property; ////// Simple constructor /// /// the property metadata internal SimplePropertyRef(md.EdmMember property) { m_property = property; } ////// Gets the property metadata /// internal md.EdmMember Property { get { return m_property; } } ////// Overrides the default equality function. Two SimplePropertyRefs are /// equal, if they describe the same property /// /// ///public override bool Equals(object obj) { SimplePropertyRef other = obj as SimplePropertyRef; return (other != null && InternalTrees.Command.EqualTypes(m_property.DeclaringType, other.m_property.DeclaringType) && other.m_property.Name.Equals(this.m_property.Name)); } /// /// Overrides the default hashcode function. /// Simply returns the hashcode for the property instead /// ///public override int GetHashCode() { return m_property.Name.GetHashCode(); } /// /// /// ///public override string ToString() { return m_property.Name; } } /// /// A TypeId propertyref represents a reference to the TypeId property /// of a type (complex type, entity type etc.) /// internal class TypeIdPropertyRef : PropertyRef { private TypeIdPropertyRef() : base() { } ////// Gets the default instance of this type /// internal static TypeIdPropertyRef Instance = new TypeIdPropertyRef(); ////// Friendly string for debugging. /// public override string ToString() { return "TYPEID"; } } ////// An NullSentinel propertyref represents the NullSentinel property for /// a row type. /// As with TypeId, this class is a singleton instance /// internal class NullSentinelPropertyRef : PropertyRef { private static NullSentinelPropertyRef s_singleton = new NullSentinelPropertyRef(); private NullSentinelPropertyRef() : base() { } ////// Gets the singleton instance /// internal static NullSentinelPropertyRef Instance { get { return s_singleton; } } ////// /// ///public override string ToString() { return "NULLSENTINEL"; } } /// /// An EntitySetId propertyref represents the EntitySetId property for /// an entity type or a ref type. /// As with TypeId, this class is a singleton instance /// internal class EntitySetIdPropertyRef : PropertyRef { private EntitySetIdPropertyRef() : base() { } ////// Gets the singleton instance /// internal static EntitySetIdPropertyRef Instance = new EntitySetIdPropertyRef(); ////// /// ///public override string ToString() { return "ENTITYSETID"; } } /// /// A nested propertyref describes a nested property access - think "a.b.c" /// internal class NestedPropertyRef : PropertyRef { private readonly PropertyRef m_inner; private readonly PropertyRef m_outer; ////// Basic constructor. /// Represents the access of property "propertyRef" within property "property" /// /// the inner property /// the outer property internal NestedPropertyRef(PropertyRef innerProperty, PropertyRef outerProperty) { PlanCompiler.Assert(!(innerProperty is NestedPropertyRef), "innerProperty cannot be a NestedPropertyRef"); m_inner = innerProperty; m_outer = outerProperty; } ////// the nested property /// internal PropertyRef OuterProperty { get { return m_outer; } } ////// the parent property /// internal PropertyRef InnerProperty { get { return m_inner; } } ////// Overrides the default equality function. Two NestedPropertyRefs are /// equal if the have the same property name, and the types are the same /// /// ///public override bool Equals(object obj) { NestedPropertyRef other = obj as NestedPropertyRef; return (other != null && m_inner.Equals(other.m_inner) && m_outer.Equals(other.m_outer)); } /// /// Overrides the default hashcode function. Simply adds the hashcodes /// of the "property" and "propertyRef" fields /// ///public override int GetHashCode() { return m_inner.GetHashCode() ^ m_outer.GetHashCode(); } /// /// /// ///public override string ToString() { return m_inner + "." + m_outer; } } /// /// A reference to "all" properties of a type /// internal class AllPropertyRef : PropertyRef { private AllPropertyRef() : base() { } ////// Get the singleton instance /// internal static AllPropertyRef Instance = new AllPropertyRef(); ////// Create a nested property ref, with "p" as the prefix /// /// the property to prefix with ///the nested property reference internal override PropertyRef CreateNestedPropertyRef(PropertyRef p) { return p; } ////// /// ///public override string ToString() { return "ALL"; } } /// /// A rel-property ref - represents a rel property of the type /// internal class RelPropertyRef : PropertyRef { #region private state private InternalTrees.RelProperty m_property; #endregion #region constructor ////// Simple constructor /// /// the property metadata internal RelPropertyRef(InternalTrees.RelProperty property) { m_property = property; } #endregion #region public apis ////// Gets the property metadata /// internal InternalTrees.RelProperty Property { get { return m_property; } } ////// Overrides the default equality function. Two RelPropertyRefs are /// equal, if they describe the same property /// /// the other object to compare to ///true, if the objects are equal public override bool Equals(object obj) { RelPropertyRef other = obj as RelPropertyRef; return (other != null && m_property.Equals(other.m_property)); } ////// Overrides the default hashcode function. /// Simply returns the hashcode for the property instead /// ///hashcode for the relpropertyref public override int GetHashCode() { return m_property.GetHashCode(); } ////// debugging support /// ///public override string ToString() { return m_property.ToString(); } #endregion } /// /// Represents a collection of property references /// internal class PropertyRefList { private Dictionarym_propertyReferences; private bool m_allProperties; /// /// Get something that represents "all" property references /// internal static PropertyRefList All = new PropertyRefList(true); ////// Trivial constructor /// internal PropertyRefList() : this(false) {} private PropertyRefList(bool allProps) { this.m_propertyReferences = new Dictionary(); if (allProps) { MakeAllProperties(); } } private void MakeAllProperties() { m_allProperties = true; m_propertyReferences.Clear(); m_propertyReferences.Add(AllPropertyRef.Instance, AllPropertyRef.Instance); } /// /// Add a new property reference to this list /// /// new property reference internal void Add(PropertyRef property) { if (m_allProperties) return; else if (property is AllPropertyRef) MakeAllProperties(); else m_propertyReferences[property] = property; } ////// Append an existing list of property references to myself /// /// list of property references internal void Append(PropertyRefList propertyRefs) { if (m_allProperties) return; foreach (PropertyRef p in propertyRefs.m_propertyReferences.Keys) { this.Add(p); } } ////// Do I contain "all" properties? /// internal bool AllProperties { get { return m_allProperties; } } ////// Create a clone of myself /// ///a clone of myself internal PropertyRefList Clone() { PropertyRefList newProps = new PropertyRefList(m_allProperties); foreach (PropertyRef p in this.m_propertyReferences.Keys) newProps.Add(p); return newProps; } ////// Do I contain the specifed property? /// /// The property ///true, if I do internal bool Contains(PropertyRef p) { return m_allProperties || m_propertyReferences.ContainsKey(p); } ////// Get the list of all properties /// internal IEnumerableProperties { get { return m_propertyReferences.Keys; } } /// /// /// ///public override string ToString() { string x = "{"; foreach (PropertyRef p in m_propertyReferences.Keys) x += p.ToString() + ","; x += "}"; return x; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; //using System.Diagnostics; // Please use PlanCompiler.Assert instead of Debug.Assert in this class... using System.Globalization; using System.Data.Common; using md = System.Data.Metadata.Edm; // // The PropertyRef class (and its subclasses) represent references to a property // of a type. // The PropertyRefList class represents a list of expected properties // where each property from the type is described as a PropertyRef // // These classes are used by the StructuredTypeEliminator module as part of // eliminating all structured types. The basic idea of this module is that all // structured types are flattened out into a single level. To avoid a large amount // of potentially unnecessary information, we try to identify what pieces of information // are really necessary at each node of the tree. This is where PropertyRef comes in. // A PropertyRef (and more generally, a PropertyRefList) identifies a list of // properties, and can be attached to a node/var to indicate that these were the // only desired properties. // namespace System.Data.Query.PlanCompiler { ////// A PropertyRef class encapsulates a reference to one or more properties of /// a complex instance - a record type, a complex type or an entity type. /// A PropertyRef may be of the following kinds. /// - a simple property reference (just a reference to a simple property) /// - a typeid reference - applies only to entitytype and complextypes /// - an entitysetid reference - applies only to ref and entity types /// - a nested property reference (a reference to a nested property - a.b) /// - an "all" property reference (all properties) /// internal abstract class PropertyRef { ////// trivial constructor /// internal PropertyRef() { } ////// Create a nested property ref, with "p" as the prefix. /// The best way to think of this function as follows. /// Consider a type T where "this" describes a property X on T. Now /// consider a new type S, where "p" is a property of S and is of type T. /// This function creates a PropertyRef that describes the same property X /// from S.p instead /// /// the property to prefix with ///the nested property reference internal virtual PropertyRef CreateNestedPropertyRef(PropertyRef p) { return new NestedPropertyRef(p, this); } ////// Create a nested property ref for a simple property. Delegates to the function /// above /// /// the simple property ///a nestedPropertyRef internal PropertyRef CreateNestedPropertyRef(md.EdmMember p) { return CreateNestedPropertyRef(new SimplePropertyRef(p)); } ////// Creates a nested property ref for a rel-property. Delegates to the function above /// /// the rel-property ///a nested property ref internal PropertyRef CreateNestedPropertyRef(InternalTrees.RelProperty p) { return CreateNestedPropertyRef(new RelPropertyRef(p)); } ////// The tostring method for easy debuggability /// ///public override string ToString() { return ""; } } /// /// A "simple" property ref - represents a simple property of the type /// internal class SimplePropertyRef : PropertyRef { private md.EdmMember m_property; ////// Simple constructor /// /// the property metadata internal SimplePropertyRef(md.EdmMember property) { m_property = property; } ////// Gets the property metadata /// internal md.EdmMember Property { get { return m_property; } } ////// Overrides the default equality function. Two SimplePropertyRefs are /// equal, if they describe the same property /// /// ///public override bool Equals(object obj) { SimplePropertyRef other = obj as SimplePropertyRef; return (other != null && InternalTrees.Command.EqualTypes(m_property.DeclaringType, other.m_property.DeclaringType) && other.m_property.Name.Equals(this.m_property.Name)); } /// /// Overrides the default hashcode function. /// Simply returns the hashcode for the property instead /// ///public override int GetHashCode() { return m_property.Name.GetHashCode(); } /// /// /// ///public override string ToString() { return m_property.Name; } } /// /// A TypeId propertyref represents a reference to the TypeId property /// of a type (complex type, entity type etc.) /// internal class TypeIdPropertyRef : PropertyRef { private TypeIdPropertyRef() : base() { } ////// Gets the default instance of this type /// internal static TypeIdPropertyRef Instance = new TypeIdPropertyRef(); ////// Friendly string for debugging. /// public override string ToString() { return "TYPEID"; } } ////// An NullSentinel propertyref represents the NullSentinel property for /// a row type. /// As with TypeId, this class is a singleton instance /// internal class NullSentinelPropertyRef : PropertyRef { private static NullSentinelPropertyRef s_singleton = new NullSentinelPropertyRef(); private NullSentinelPropertyRef() : base() { } ////// Gets the singleton instance /// internal static NullSentinelPropertyRef Instance { get { return s_singleton; } } ////// /// ///public override string ToString() { return "NULLSENTINEL"; } } /// /// An EntitySetId propertyref represents the EntitySetId property for /// an entity type or a ref type. /// As with TypeId, this class is a singleton instance /// internal class EntitySetIdPropertyRef : PropertyRef { private EntitySetIdPropertyRef() : base() { } ////// Gets the singleton instance /// internal static EntitySetIdPropertyRef Instance = new EntitySetIdPropertyRef(); ////// /// ///public override string ToString() { return "ENTITYSETID"; } } /// /// A nested propertyref describes a nested property access - think "a.b.c" /// internal class NestedPropertyRef : PropertyRef { private readonly PropertyRef m_inner; private readonly PropertyRef m_outer; ////// Basic constructor. /// Represents the access of property "propertyRef" within property "property" /// /// the inner property /// the outer property internal NestedPropertyRef(PropertyRef innerProperty, PropertyRef outerProperty) { PlanCompiler.Assert(!(innerProperty is NestedPropertyRef), "innerProperty cannot be a NestedPropertyRef"); m_inner = innerProperty; m_outer = outerProperty; } ////// the nested property /// internal PropertyRef OuterProperty { get { return m_outer; } } ////// the parent property /// internal PropertyRef InnerProperty { get { return m_inner; } } ////// Overrides the default equality function. Two NestedPropertyRefs are /// equal if the have the same property name, and the types are the same /// /// ///public override bool Equals(object obj) { NestedPropertyRef other = obj as NestedPropertyRef; return (other != null && m_inner.Equals(other.m_inner) && m_outer.Equals(other.m_outer)); } /// /// Overrides the default hashcode function. Simply adds the hashcodes /// of the "property" and "propertyRef" fields /// ///public override int GetHashCode() { return m_inner.GetHashCode() ^ m_outer.GetHashCode(); } /// /// /// ///public override string ToString() { return m_inner + "." + m_outer; } } /// /// A reference to "all" properties of a type /// internal class AllPropertyRef : PropertyRef { private AllPropertyRef() : base() { } ////// Get the singleton instance /// internal static AllPropertyRef Instance = new AllPropertyRef(); ////// Create a nested property ref, with "p" as the prefix /// /// the property to prefix with ///the nested property reference internal override PropertyRef CreateNestedPropertyRef(PropertyRef p) { return p; } ////// /// ///public override string ToString() { return "ALL"; } } /// /// A rel-property ref - represents a rel property of the type /// internal class RelPropertyRef : PropertyRef { #region private state private InternalTrees.RelProperty m_property; #endregion #region constructor ////// Simple constructor /// /// the property metadata internal RelPropertyRef(InternalTrees.RelProperty property) { m_property = property; } #endregion #region public apis ////// Gets the property metadata /// internal InternalTrees.RelProperty Property { get { return m_property; } } ////// Overrides the default equality function. Two RelPropertyRefs are /// equal, if they describe the same property /// /// the other object to compare to ///true, if the objects are equal public override bool Equals(object obj) { RelPropertyRef other = obj as RelPropertyRef; return (other != null && m_property.Equals(other.m_property)); } ////// Overrides the default hashcode function. /// Simply returns the hashcode for the property instead /// ///hashcode for the relpropertyref public override int GetHashCode() { return m_property.GetHashCode(); } ////// debugging support /// ///public override string ToString() { return m_property.ToString(); } #endregion } /// /// Represents a collection of property references /// internal class PropertyRefList { private Dictionarym_propertyReferences; private bool m_allProperties; /// /// Get something that represents "all" property references /// internal static PropertyRefList All = new PropertyRefList(true); ////// Trivial constructor /// internal PropertyRefList() : this(false) {} private PropertyRefList(bool allProps) { this.m_propertyReferences = new Dictionary(); if (allProps) { MakeAllProperties(); } } private void MakeAllProperties() { m_allProperties = true; m_propertyReferences.Clear(); m_propertyReferences.Add(AllPropertyRef.Instance, AllPropertyRef.Instance); } /// /// Add a new property reference to this list /// /// new property reference internal void Add(PropertyRef property) { if (m_allProperties) return; else if (property is AllPropertyRef) MakeAllProperties(); else m_propertyReferences[property] = property; } ////// Append an existing list of property references to myself /// /// list of property references internal void Append(PropertyRefList propertyRefs) { if (m_allProperties) return; foreach (PropertyRef p in propertyRefs.m_propertyReferences.Keys) { this.Add(p); } } ////// Do I contain "all" properties? /// internal bool AllProperties { get { return m_allProperties; } } ////// Create a clone of myself /// ///a clone of myself internal PropertyRefList Clone() { PropertyRefList newProps = new PropertyRefList(m_allProperties); foreach (PropertyRef p in this.m_propertyReferences.Keys) newProps.Add(p); return newProps; } ////// Do I contain the specifed property? /// /// The property ///true, if I do internal bool Contains(PropertyRef p) { return m_allProperties || m_propertyReferences.ContainsKey(p); } ////// Get the list of all properties /// internal IEnumerableProperties { get { return m_propertyReferences.Keys; } } /// /// /// ///public override string ToString() { string x = "{"; foreach (PropertyRef p in m_propertyReferences.Keys) x += p.ToString() + ","; x += "}"; return x; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
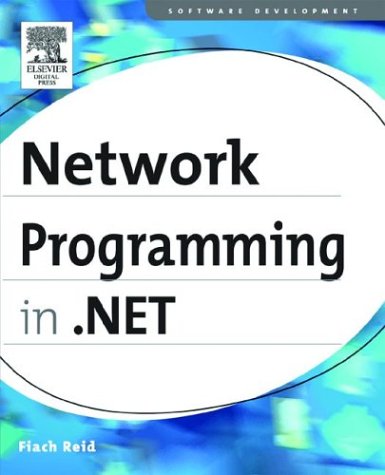
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OdbcEnvironment.cs
- TokenBasedSet.cs
- EmptyEnumerable.cs
- ThousandthOfEmRealPoints.cs
- httpstaticobjectscollection.cs
- TypeTypeConverter.cs
- Win32Native.cs
- PageCodeDomTreeGenerator.cs
- ModelUtilities.cs
- HyperLinkDesigner.cs
- KeyEvent.cs
- ProjectionAnalyzer.cs
- ListViewEditEventArgs.cs
- SymbolUsageManager.cs
- ServicePointManagerElement.cs
- MemberDescriptor.cs
- CustomAttributeBuilder.cs
- TypeGeneratedEventArgs.cs
- DateTimeOffsetConverter.cs
- DbSetClause.cs
- CollectionConverter.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- Thickness.cs
- Lease.cs
- FormatException.cs
- DeliveryStrategy.cs
- ListViewItemEventArgs.cs
- DataTemplate.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- JsonFormatWriterGenerator.cs
- BoundsDrawingContextWalker.cs
- ZipIOModeEnforcingStream.cs
- XmlSignatureManifest.cs
- BinHexEncoder.cs
- LogWriteRestartAreaState.cs
- ADMembershipUser.cs
- TextTabProperties.cs
- WpfKnownType.cs
- FtpWebRequest.cs
- DataSourceExpressionCollection.cs
- TextBreakpoint.cs
- BitSet.cs
- ReachVisualSerializer.cs
- MethodCallTranslator.cs
- BevelBitmapEffect.cs
- JsonEncodingStreamWrapper.cs
- ControlAdapter.cs
- NullToBooleanConverter.cs
- ClientRoleProvider.cs
- DetailsView.cs
- TimerEventSubscriptionCollection.cs
- WebConfigurationFileMap.cs
- CodeSubDirectoriesCollection.cs
- NullRuntimeConfig.cs
- CodeRegionDirective.cs
- ScriptingSectionGroup.cs
- AsynchronousChannelMergeEnumerator.cs
- DataIdProcessor.cs
- GlobalizationAssembly.cs
- SafeNativeMethods.cs
- ImageAnimator.cs
- StateManagedCollection.cs
- CompilerHelpers.cs
- BlurBitmapEffect.cs
- Menu.cs
- SectionInput.cs
- ExcludeFromCodeCoverageAttribute.cs
- ValidatorCompatibilityHelper.cs
- FileInfo.cs
- SystemColors.cs
- Errors.cs
- Window.cs
- FixedTextPointer.cs
- BaseProcessor.cs
- SqlDependencyUtils.cs
- ListBox.cs
- DodSequenceMerge.cs
- DataGridViewRowCollection.cs
- SqlXml.cs
- DuplicateWaitObjectException.cs
- Rotation3D.cs
- HandlerBase.cs
- ThreadExceptionDialog.cs
- ConcatQueryOperator.cs
- ControlCachePolicy.cs
- ByteStreamBufferedMessageData.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- FactoryMaker.cs
- BindingListCollectionView.cs
- InputBuffer.cs
- MouseCaptureWithinProperty.cs
- ViewCellRelation.cs
- DrawingContext.cs
- WebPartRestoreVerb.cs
- HexParser.cs
- HttpDebugHandler.cs
- PersonalizationState.cs
- DataSourceExpression.cs
- ApplicationException.cs
- ISO2022Encoding.cs