Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / WebControls / CalendarDay.cs / 1 / CalendarDay.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.ComponentModel; using System; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class CalendarDay { private DateTime date; private bool isSelectable; private bool isToday; private bool isWeekend; private bool isOtherMonth; private bool isSelected; private string dayNumberText; ///Represents a calendar day. ////// public CalendarDay(DateTime date, bool isWeekend, bool isToday, bool isSelected, bool isOtherMonth, string dayNumberText) { this.date = date; this.isWeekend = isWeekend; this.isToday = isToday; this.isOtherMonth = isOtherMonth; this.isSelected = isSelected; this.dayNumberText = dayNumberText; } ///[To be supplied.] ////// public DateTime Date { get { return date; } } ///Gets the date represented by an instance of this class. This /// property is read-only. ////// public string DayNumberText { get { return dayNumberText; } } ///Gets the string equivilent of the date represented by an instance of this class. This property is read-only. ////// public bool IsOtherMonth { get { return isOtherMonth; } } ///Gets a value indicating whether the date represented by an instance of /// this class is in a different month from the month currently being displayed. This /// property is read-only. ////// public bool IsSelectable { get { return isSelectable; } set { isSelectable = value; } } ///Gets or sets a value indicating whether the date represented /// by an instance of /// this class can be selected. ////// public bool IsSelected { get { return isSelected; } } ///Gets a value indicating whether date represented by an instance of this class is selected. This property is read-only. ////// public bool IsToday { get { return isToday; } } ///Gets a value indicating whether the date represented by an instance of this class is today's date. This property is read-only. ////// public bool IsWeekend { get { return isWeekend; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Gets a value indicating whether the date represented by an instance of /// this class is on a weekend day. This property is read-only. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.ComponentModel; using System; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class CalendarDay { private DateTime date; private bool isSelectable; private bool isToday; private bool isWeekend; private bool isOtherMonth; private bool isSelected; private string dayNumberText; ///Represents a calendar day. ////// public CalendarDay(DateTime date, bool isWeekend, bool isToday, bool isSelected, bool isOtherMonth, string dayNumberText) { this.date = date; this.isWeekend = isWeekend; this.isToday = isToday; this.isOtherMonth = isOtherMonth; this.isSelected = isSelected; this.dayNumberText = dayNumberText; } ///[To be supplied.] ////// public DateTime Date { get { return date; } } ///Gets the date represented by an instance of this class. This /// property is read-only. ////// public string DayNumberText { get { return dayNumberText; } } ///Gets the string equivilent of the date represented by an instance of this class. This property is read-only. ////// public bool IsOtherMonth { get { return isOtherMonth; } } ///Gets a value indicating whether the date represented by an instance of /// this class is in a different month from the month currently being displayed. This /// property is read-only. ////// public bool IsSelectable { get { return isSelectable; } set { isSelectable = value; } } ///Gets or sets a value indicating whether the date represented /// by an instance of /// this class can be selected. ////// public bool IsSelected { get { return isSelected; } } ///Gets a value indicating whether date represented by an instance of this class is selected. This property is read-only. ////// public bool IsToday { get { return isToday; } } ///Gets a value indicating whether the date represented by an instance of this class is today's date. This property is read-only. ////// public bool IsWeekend { get { return isWeekend; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Gets a value indicating whether the date represented by an instance of /// this class is on a weekend day. This property is read-only. ///
Link Menu
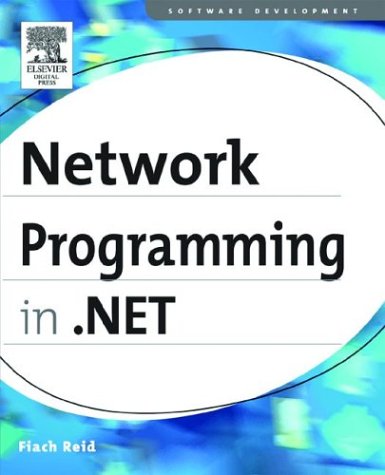
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ColorAnimation.cs
- SqlXml.cs
- ThrowHelper.cs
- TypeLibConverter.cs
- CodeVariableReferenceExpression.cs
- PropertyTabAttribute.cs
- ExpressionBindingCollection.cs
- PermissionAttributes.cs
- ReversePositionQuery.cs
- EditCommandColumn.cs
- COM2PropertyDescriptor.cs
- CompoundFileStorageReference.cs
- Point4DConverter.cs
- PersianCalendar.cs
- NotCondition.cs
- MaskedTextProvider.cs
- GeneratedCodeAttribute.cs
- WindowsListViewScroll.cs
- TokenBasedSetEnumerator.cs
- CmsInterop.cs
- DataError.cs
- InputScope.cs
- CustomCategoryAttribute.cs
- ForwardPositionQuery.cs
- UInt16Storage.cs
- WindowsFormsLinkLabel.cs
- CacheAxisQuery.cs
- Bits.cs
- HtmlInputControl.cs
- MaskDescriptor.cs
- RenderDataDrawingContext.cs
- Validator.cs
- GridPattern.cs
- ErrorWebPart.cs
- EmptyEnumerable.cs
- NativeMethods.cs
- InternalBase.cs
- DecodeHelper.cs
- MbpInfo.cs
- ToolStripRendererSwitcher.cs
- XslCompiledTransform.cs
- ToolStripContextMenu.cs
- CorruptingExceptionCommon.cs
- EventLevel.cs
- BamlLocalizer.cs
- SecurityPolicySection.cs
- PageThemeBuildProvider.cs
- XdrBuilder.cs
- HttpRequestCacheValidator.cs
- DataGridRowHeader.cs
- ContentPropertyAttribute.cs
- RegexStringValidator.cs
- ExpressionBuilder.cs
- GenericUriParser.cs
- SpecialNameAttribute.cs
- PropertyTab.cs
- ListBox.cs
- ToolStripCustomTypeDescriptor.cs
- StringFunctions.cs
- ComIntegrationManifestGenerator.cs
- StringFunctions.cs
- ScriptServiceAttribute.cs
- PositiveTimeSpanValidatorAttribute.cs
- MissingMethodException.cs
- SqlUserDefinedTypeAttribute.cs
- PagedControl.cs
- Int16AnimationBase.cs
- WmlMobileTextWriter.cs
- MessageBodyDescription.cs
- EncryptedData.cs
- OpenFileDialog.cs
- MultiSelectRootGridEntry.cs
- DataBoundControlHelper.cs
- StringArrayConverter.cs
- WebServiceTypeData.cs
- Point4DValueSerializer.cs
- HttpWebRequestElement.cs
- dataobject.cs
- SettingsContext.cs
- XmlQueryRuntime.cs
- RealProxy.cs
- LinkButton.cs
- DataObject.cs
- TPLETWProvider.cs
- OdbcConnection.cs
- ExpressionBuilderCollection.cs
- Vector3DConverter.cs
- DataGridView.cs
- OleDbError.cs
- Point4D.cs
- DoneReceivingAsyncResult.cs
- IApplicationTrustManager.cs
- HandlerMappingMemo.cs
- ExecutedRoutedEventArgs.cs
- ArcSegment.cs
- COM2ExtendedBrowsingHandler.cs
- Int32RectValueSerializer.cs
- FloaterParagraph.cs
- ColorConverter.cs
- CountAggregationOperator.cs