Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Selectors / X509SecurityTokenProvider.cs / 1 / X509SecurityTokenProvider.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Selectors { using System.IdentityModel.Tokens; using System.Security.Cryptography.X509Certificates; public class X509SecurityTokenProvider : SecurityTokenProvider, IDisposable { X509Certificate2 certificate; public X509SecurityTokenProvider(X509Certificate2 certificate) { if (certificate == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("certificate"); } this.certificate = new X509Certificate2(certificate); } public X509SecurityTokenProvider(StoreLocation storeLocation, StoreName storeName, X509FindType findType, object findValue) { if (findValue == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("findValue"); } X509CertificateStore store = new X509CertificateStore(storeName, storeLocation); X509Certificate2Collection certificates = null; try { store.Open(OpenFlags.ReadOnly); certificates = store.Find(findType, findValue, false); if (certificates.Count < 1) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.CannotFindCert, storeName, storeLocation, findType, findValue))); } if (certificates.Count > 1) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.FoundMultipleCerts, storeName, storeLocation, findType, findValue))); } this.certificate = new X509Certificate2(certificates[0]); } finally { SecurityUtils.ResetAllCertificates(certificates); store.Close(); } } public X509Certificate2 Certificate { get { return this.certificate; } } protected override SecurityToken GetTokenCore(TimeSpan timeout) { return new X509SecurityToken(this.certificate); } public void Dispose() { this.certificate.Reset(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
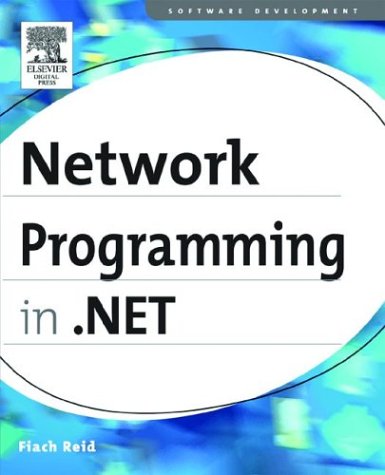
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TrackingMemoryStreamFactory.cs
- Brushes.cs
- Pool.cs
- RegexRunner.cs
- AssemblyBuilder.cs
- WinFormsComponentEditor.cs
- DictionaryEntry.cs
- TableLayoutCellPaintEventArgs.cs
- WebPartAuthorizationEventArgs.cs
- AddInToken.cs
- PtsContext.cs
- EntityModelBuildProvider.cs
- PropertyIDSet.cs
- Vector3D.cs
- FrameworkTextComposition.cs
- SignedXml.cs
- AdCreatedEventArgs.cs
- HttpListenerContext.cs
- RandomNumberGenerator.cs
- AttributeCollection.cs
- WinFormsSecurity.cs
- RecipientInfo.cs
- GroupQuery.cs
- FilterableAttribute.cs
- SafeNativeMethods.cs
- GetMemberBinder.cs
- ColumnResizeAdorner.cs
- PropertyDescriptors.cs
- DataGridViewButtonColumn.cs
- EngineSiteSapi.cs
- DiscoveryDocumentSearchPattern.cs
- EntityDescriptor.cs
- WindowsFormsDesignerOptionService.cs
- LogPolicy.cs
- Visual3D.cs
- AmbiguousMatchException.cs
- KeyPullup.cs
- NameHandler.cs
- ContextBase.cs
- FunctionCommandText.cs
- DrawingVisual.cs
- UniqueIdentifierService.cs
- XamlSerializerUtil.cs
- MissingSatelliteAssemblyException.cs
- TextParentUndoUnit.cs
- AppDomainEvidenceFactory.cs
- SerialPort.cs
- WebPartZoneCollection.cs
- QueryOperationResponseOfT.cs
- ConstructorArgumentAttribute.cs
- _ShellExpression.cs
- TransformationRules.cs
- TextEditorSelection.cs
- PartitionedStream.cs
- ReferenceEqualityComparer.cs
- AssemblyCache.cs
- IdentifierCollection.cs
- Int64Converter.cs
- PropertyPushdownHelper.cs
- TextTreeTextBlock.cs
- XPathNodePointer.cs
- ComponentResourceManager.cs
- RequestNavigateEventArgs.cs
- ValidatorCompatibilityHelper.cs
- XmlValidatingReader.cs
- ItemsPresenter.cs
- CqlLexerHelpers.cs
- MachineSettingsSection.cs
- TTSVoice.cs
- StringValidatorAttribute.cs
- SoapMessage.cs
- OleDbInfoMessageEvent.cs
- Encoding.cs
- ButtonStandardAdapter.cs
- diagnosticsswitches.cs
- EntityProviderFactory.cs
- Transform.cs
- TemplateContent.cs
- OleDbParameter.cs
- ToolStripDropDownClosingEventArgs.cs
- PointLight.cs
- PolicyLevel.cs
- Stopwatch.cs
- CodeSnippetTypeMember.cs
- ShortcutKeysEditor.cs
- ServiceModelTimeSpanValidator.cs
- cookiecollection.cs
- DoubleLink.cs
- ParsedRoute.cs
- MessagePropertyDescriptionCollection.cs
- CellIdBoolean.cs
- Currency.cs
- UserControlBuildProvider.cs
- ComplexLine.cs
- XmlWrappingReader.cs
- Rotation3DKeyFrameCollection.cs
- CellConstantDomain.cs
- embossbitmapeffect.cs
- XmlImplementation.cs
- PropertyItem.cs