Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Policy / AppDomainEvidenceFactory.cs / 1305376 / AppDomainEvidenceFactory.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Reflection; namespace System.Security.Policy { ////// Factory class which creates evidence on demand for an AppDomain /// internal sealed class AppDomainEvidenceFactory : IRuntimeEvidenceFactory { private AppDomain m_targetDomain; private Evidence m_entryPointEvidence; internal AppDomainEvidenceFactory(AppDomain target) { Contract.Assert(target != null); Contract.Assert(target == AppDomain.CurrentDomain, "AppDomainEvidenceFactory should not be used across domains."); m_targetDomain = target; } ////// AppDomain this factory generates evidence for /// public IEvidenceFactory Target { get { return m_targetDomain; } } ////// Return any evidence supplied by the AppDomain itself /// public IEnumerableGetFactorySuppliedEvidence() { // AppDomains do not contain serialized evidence return new EvidenceBase[] { }; } /// /// Generate evidence on demand for an AppDomain /// [SecuritySafeCritical] public EvidenceBase GenerateEvidence(Type evidenceType) { // For v1.x compatibility, the default AppDomain has the same evidence as the entry point // assembly. Since other AppDomains inherit their evidence from the default AppDomain by // default, they also use the entry point assembly. BCLDebug.Assert(m_targetDomain == AppDomain.CurrentDomain, "AppDomainEvidenceFactory should not be used across domains."); if (m_targetDomain.IsDefaultAppDomain()) { // If we don't already know the evidence for the entry point assembly, get that now. If we // have a RuntimeAssembly go directly to its EvidenceNoDemand property to avoid the full // demand that it will do on access to its Evidence property. if (m_entryPointEvidence == null) { Assembly entryAssembly = Assembly.GetEntryAssembly(); RuntimeAssembly entryRuntimeAssembly = entryAssembly as RuntimeAssembly; if (entryRuntimeAssembly != null) { m_entryPointEvidence = entryRuntimeAssembly.EvidenceNoDemand.Clone(); } else if (entryAssembly != null) { m_entryPointEvidence = entryAssembly.Evidence; } } // If the entry point assembly provided evidence, then we use that for the AppDomain if (m_entryPointEvidence != null) { return m_entryPointEvidence.GetHostEvidence(evidenceType); } } else { // If we're not the default domain, then we should inherit our evidence from the default // domain -- so ask it what evidence it has of this type. AppDomain defaultDomain = AppDomain.GetDefaultDomain(); return defaultDomain.GetHostEvidence(evidenceType); } // AppDomains do not generate any evidence on demand return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Reflection; namespace System.Security.Policy { ////// Factory class which creates evidence on demand for an AppDomain /// internal sealed class AppDomainEvidenceFactory : IRuntimeEvidenceFactory { private AppDomain m_targetDomain; private Evidence m_entryPointEvidence; internal AppDomainEvidenceFactory(AppDomain target) { Contract.Assert(target != null); Contract.Assert(target == AppDomain.CurrentDomain, "AppDomainEvidenceFactory should not be used across domains."); m_targetDomain = target; } ////// AppDomain this factory generates evidence for /// public IEvidenceFactory Target { get { return m_targetDomain; } } ////// Return any evidence supplied by the AppDomain itself /// public IEnumerableGetFactorySuppliedEvidence() { // AppDomains do not contain serialized evidence return new EvidenceBase[] { }; } /// /// Generate evidence on demand for an AppDomain /// [SecuritySafeCritical] public EvidenceBase GenerateEvidence(Type evidenceType) { // For v1.x compatibility, the default AppDomain has the same evidence as the entry point // assembly. Since other AppDomains inherit their evidence from the default AppDomain by // default, they also use the entry point assembly. BCLDebug.Assert(m_targetDomain == AppDomain.CurrentDomain, "AppDomainEvidenceFactory should not be used across domains."); if (m_targetDomain.IsDefaultAppDomain()) { // If we don't already know the evidence for the entry point assembly, get that now. If we // have a RuntimeAssembly go directly to its EvidenceNoDemand property to avoid the full // demand that it will do on access to its Evidence property. if (m_entryPointEvidence == null) { Assembly entryAssembly = Assembly.GetEntryAssembly(); RuntimeAssembly entryRuntimeAssembly = entryAssembly as RuntimeAssembly; if (entryRuntimeAssembly != null) { m_entryPointEvidence = entryRuntimeAssembly.EvidenceNoDemand.Clone(); } else if (entryAssembly != null) { m_entryPointEvidence = entryAssembly.Evidence; } } // If the entry point assembly provided evidence, then we use that for the AppDomain if (m_entryPointEvidence != null) { return m_entryPointEvidence.GetHostEvidence(evidenceType); } } else { // If we're not the default domain, then we should inherit our evidence from the default // domain -- so ask it what evidence it has of this type. AppDomain defaultDomain = AppDomain.GetDefaultDomain(); return defaultDomain.GetHostEvidence(evidenceType); } // AppDomains do not generate any evidence on demand return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
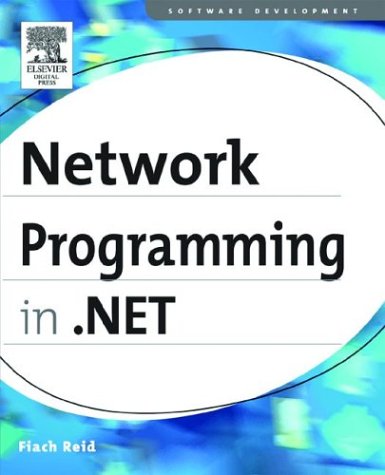
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Matrix.cs
- PersonalizationStateInfo.cs
- AsyncPostBackTrigger.cs
- GenericsInstances.cs
- SoapServerMethod.cs
- EditorZone.cs
- DataControlImageButton.cs
- HtmlInputReset.cs
- StrongName.cs
- FormViewDeleteEventArgs.cs
- StructuralComparisons.cs
- WorkflowInstanceQuery.cs
- GACIdentityPermission.cs
- Int32CollectionConverter.cs
- CollectionExtensions.cs
- DesignerVerbCollection.cs
- MemberDescriptor.cs
- StorageFunctionMapping.cs
- SqlInternalConnectionTds.cs
- DomainConstraint.cs
- SessionState.cs
- NativeMethods.cs
- DelegatingConfigHost.cs
- _WinHttpWebProxyDataBuilder.cs
- MappingMetadataHelper.cs
- DeviceSpecificChoice.cs
- DesignTimeDataBinding.cs
- ToolZone.cs
- SecurityKeyIdentifierClause.cs
- formatter.cs
- MultiTrigger.cs
- DiscoveryDocument.cs
- Base64Decoder.cs
- UIElementParagraph.cs
- _AutoWebProxyScriptHelper.cs
- Update.cs
- _PooledStream.cs
- IntegerValidatorAttribute.cs
- NodeFunctions.cs
- EmptyEnumerator.cs
- DrawingAttributesDefaultValueFactory.cs
- StickyNoteContentControl.cs
- MailDefinition.cs
- DataServiceStreamResponse.cs
- DescendantQuery.cs
- LayeredChannelListener.cs
- ExpressionBuilder.cs
- TrayIconDesigner.cs
- XmlAggregates.cs
- ReceiveActivityDesigner.cs
- GroupAggregateExpr.cs
- StreamGeometry.cs
- EmbeddedMailObject.cs
- ApplicationServiceManager.cs
- MappingMetadataHelper.cs
- CompilationPass2Task.cs
- Lookup.cs
- SchemaImporter.cs
- _AcceptOverlappedAsyncResult.cs
- NamespaceCollection.cs
- HtmlControlPersistable.cs
- XmlBufferReader.cs
- ApplicationManager.cs
- Graph.cs
- TypeDescriptionProvider.cs
- HttpGetClientProtocol.cs
- Scheduler.cs
- WindowsFormsLinkLabel.cs
- MulticastNotSupportedException.cs
- IncomingWebRequestContext.cs
- GeometryGroup.cs
- WindowsAuthenticationModule.cs
- ChildDocumentBlock.cs
- CleanUpVirtualizedItemEventArgs.cs
- LinearGradientBrush.cs
- DesignerSerializerAttribute.cs
- Parameter.cs
- RegexTree.cs
- BmpBitmapDecoder.cs
- CngAlgorithm.cs
- CriticalHandle.cs
- WebPartMenu.cs
- CaseExpr.cs
- DataGridViewCheckBoxCell.cs
- complextypematerializer.cs
- AnimationTimeline.cs
- TableMethodGenerator.cs
- TemplateControlParser.cs
- XmlSerializer.cs
- XmlnsDictionary.cs
- BitmapVisualManager.cs
- ResourceReader.cs
- DataSourceControl.cs
- OutputCacheProfile.cs
- StylusButtonCollection.cs
- TableHeaderCell.cs
- ThreadStaticAttribute.cs
- OpenTypeCommon.cs
- IdSpace.cs
- ContextProperty.cs