Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Configuration / System / Configuration / StringValidatorAttribute.cs / 1305376 / StringValidatorAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration.Internal; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.IO; using System.Reflection; using System.Security.Permissions; using System.Xml; using System.Globalization; using System.ComponentModel; using System.Security; using System.Text; namespace System.Configuration { [AttributeUsage(AttributeTargets.Property)] public sealed class StringValidatorAttribute : ConfigurationValidatorAttribute { private int _minLength = 0; private int _maxLength = int.MaxValue; private string _invalidChars; public StringValidatorAttribute() { } public override ConfigurationValidatorBase ValidatorInstance { get { return new StringValidator(_minLength, _maxLength, _invalidChars); } } public int MinLength { get { return _minLength; } set { if (_maxLength < value) { throw new ArgumentOutOfRangeException("value", SR.GetString(SR.Validator_min_greater_than_max)); } _minLength = value; } } public int MaxLength { get { return _maxLength; } set { if (_minLength > value) { throw new ArgumentOutOfRangeException("value", SR.GetString(SR.Validator_min_greater_than_max)); } _maxLength = value; } } public string InvalidCharacters { get { return _invalidChars; } set { _invalidChars = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration.Internal; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.IO; using System.Reflection; using System.Security.Permissions; using System.Xml; using System.Globalization; using System.ComponentModel; using System.Security; using System.Text; namespace System.Configuration { [AttributeUsage(AttributeTargets.Property)] public sealed class StringValidatorAttribute : ConfigurationValidatorAttribute { private int _minLength = 0; private int _maxLength = int.MaxValue; private string _invalidChars; public StringValidatorAttribute() { } public override ConfigurationValidatorBase ValidatorInstance { get { return new StringValidator(_minLength, _maxLength, _invalidChars); } } public int MinLength { get { return _minLength; } set { if (_maxLength < value) { throw new ArgumentOutOfRangeException("value", SR.GetString(SR.Validator_min_greater_than_max)); } _minLength = value; } } public int MaxLength { get { return _maxLength; } set { if (_minLength > value) { throw new ArgumentOutOfRangeException("value", SR.GetString(SR.Validator_min_greater_than_max)); } _maxLength = value; } } public string InvalidCharacters { get { return _invalidChars; } set { _invalidChars = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
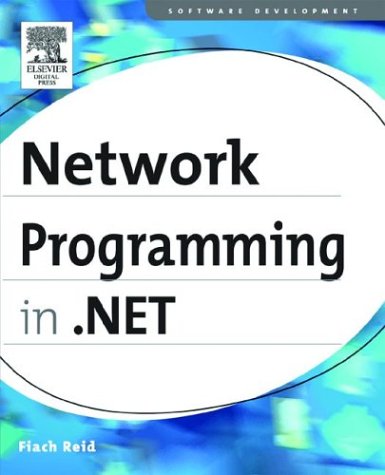
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaExporter.cs
- ComponentChangedEvent.cs
- RootBrowserWindow.cs
- ColumnHeaderCollectionEditor.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- IDReferencePropertyAttribute.cs
- ContextBase.cs
- DataGridViewComboBoxEditingControl.cs
- RemotingAttributes.cs
- HealthMonitoringSection.cs
- XmlSchemaSimpleType.cs
- BuildProvidersCompiler.cs
- CLSCompliantAttribute.cs
- ClientSettings.cs
- BasicKeyConstraint.cs
- FormsAuthenticationUser.cs
- BatchParser.cs
- Exception.cs
- DataGridViewHitTestInfo.cs
- Rights.cs
- ErrorEventArgs.cs
- ConstraintManager.cs
- ErrorHandler.cs
- DataServiceException.cs
- Pkcs9Attribute.cs
- PointAnimationUsingKeyFrames.cs
- SemanticAnalyzer.cs
- Preprocessor.cs
- Errors.cs
- LoginCancelEventArgs.cs
- WebControlParameterProxy.cs
- ServiceOperation.cs
- BasicAsyncResult.cs
- ExecutedRoutedEventArgs.cs
- BasicKeyConstraint.cs
- MediaPlayer.cs
- OracleEncoding.cs
- ViewKeyConstraint.cs
- UIElement.cs
- SqlClientWrapperSmiStream.cs
- ObjectSpanRewriter.cs
- BaseTemplateParser.cs
- XPathItem.cs
- DynamicILGenerator.cs
- UriTemplateVariableQueryValue.cs
- GrammarBuilderRuleRef.cs
- XmlSignatureProperties.cs
- Version.cs
- XmlDataLoader.cs
- BookmarkWorkItem.cs
- FileIOPermission.cs
- XPathExpr.cs
- BrowserDefinitionCollection.cs
- Int32Rect.cs
- QuerySelectOp.cs
- HttpPostProtocolReflector.cs
- ClientBuildManagerCallback.cs
- ClientTargetSection.cs
- DataGridToolTip.cs
- RootContext.cs
- BindingMAnagerBase.cs
- VarRemapper.cs
- Stack.cs
- Pkcs7Signer.cs
- ComponentResourceManager.cs
- HelpEvent.cs
- ActiveXSite.cs
- SharedStatics.cs
- StringUtil.cs
- StringCollection.cs
- MsmqIntegrationChannelListener.cs
- ToolStripArrowRenderEventArgs.cs
- Models.cs
- BodyWriter.cs
- ItemCheckEvent.cs
- DockingAttribute.cs
- codemethodreferenceexpression.cs
- diagnosticsswitches.cs
- LazyTextWriterCreator.cs
- CodeMethodReturnStatement.cs
- GeneralTransform3DTo2DTo3D.cs
- ThreadBehavior.cs
- SafeNativeMethods.cs
- DesignerVerbCollection.cs
- Quaternion.cs
- EmptyReadOnlyDictionaryInternal.cs
- Viewport3DAutomationPeer.cs
- XmlLanguageConverter.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- UserControl.cs
- ObjectTag.cs
- DataColumnPropertyDescriptor.cs
- SqlDataSourceQuery.cs
- RichTextBox.cs
- IsolatedStorage.cs
- SqlRetyper.cs
- UrlMapping.cs
- NonVisualControlAttribute.cs
- SqlCacheDependencySection.cs
- WindowsListViewScroll.cs