Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / ComponentModel / ToolboxItemFilterAttribute.cs / 1 / ToolboxItemFilterAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.Diagnostics; using System.Security.Permissions; ////// This attribute allows you to configure a filter that is used enable or disable toolbox items /// from being used on particular designers. For example, you may have a class of components /// that can only be used on a certain type of designer. You can configure a toolbox item /// filter to enforce that rule. For example, take a report designer that uses a component /// base class of ReportElement. You may want to make ReportElement toolbox items enabled only /// when a ReportDesigner is the active designer. To do this, you would add the following /// ToolboxItemFilter attributes to each class: /// /// [ToolboxItemFilter("MyReportFilterString", ToolboxItemFilterType.Require)] /// public class ReportElement : Component {} /// /// [ToolboxItemFilter("MyReportFilterString", ToolboxItemFilterType.Require)] /// public class ReportDesigner : Component {} /// /// These two filters specify that ReportElement toolbox items will only be /// enabled when a ReportDesigner is visible. By specifying a filter type of /// Require on the report designer class, this will disable any toolbox items /// that are not report elements. If the report designer specifed a filter type /// of "Allow" instead of "Require", other components would be enabled when the /// report designer was active. ReportElements would still be disabled when /// other designers were active, however, because ReportElement requires designers /// to have the given filter string. /// /// Toolbox item filtering is a useful way to restrict toolbox item visibility to /// cases where it is appropriate. This can help to avoid confusion for users, but /// you should use caution not to make items unusually restrictive. If you have a /// general purpose component, for example, you should allow the component to appear /// on any designer. /// /// The ASP.NET and Windows Forms designers both use filter attributes to prevent /// each other's components from being enabled. This is a useful restriction because, /// since each has several duplicate class names, it may be confusing to users and /// they may not know which controls to choose. /// /// [ AttributeUsage(AttributeTargets.Class, AllowMultiple=true, Inherited=true), Serializable ] public sealed class ToolboxItemFilterAttribute : Attribute { private ToolboxItemFilterType filterType; private string filterString; private string typeId; ////// Initializes a new ToolboxItemFilterAttribute with the provide filter string and a filter type of /// "Allow". /// public ToolboxItemFilterAttribute(string filterString) : this(filterString, ToolboxItemFilterType.Allow) { } ////// Initializes a new ToolboxItemFilterAttribute with the provide filter string and filter type. /// public ToolboxItemFilterAttribute(string filterString, ToolboxItemFilterType filterType) { if (filterString == null) filterString = String.Empty; this.filterString = filterString; this.filterType = filterType; } ////// Retrieves the filter string for this attribute. The filter string is a user-defined string that /// is used to identify matching attributes. /// public string FilterString { get { return filterString; } } ////// Retrieves the filter type for this attribute. The filter type determines how the filter string should /// be applied. /// public ToolboxItemFilterType FilterType { get { return filterType; } } ////// The unique identifier for this attribute. All ToolboxItemFilterAttributes with the same filter string /// are considered the same, so they return the same TypeId. /// public override object TypeId { get { if (typeId == null) { typeId = GetType().FullName + filterString; } return typeId; } } public override bool Equals(object obj) { if (obj == this) { return true; } ToolboxItemFilterAttribute other = obj as ToolboxItemFilterAttribute; return (other != null && other.FilterType.Equals(FilterType) && other.FilterString.Equals(FilterString)); } public override int GetHashCode() { // No need to hash on filter type as well; there shouldn't be that many duplicates. return filterString.GetHashCode(); } public override bool Match(object obj) { ToolboxItemFilterAttribute other = obj as ToolboxItemFilterAttribute; if (other == null) { return false; } // different filter string kills a match immediately. // if (!other.FilterString.Equals(FilterString)) { return false; } return true; } public override string ToString() { return filterString + "," + Enum.GetName(typeof(ToolboxItemFilterType), filterType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.Diagnostics; using System.Security.Permissions; ////// This attribute allows you to configure a filter that is used enable or disable toolbox items /// from being used on particular designers. For example, you may have a class of components /// that can only be used on a certain type of designer. You can configure a toolbox item /// filter to enforce that rule. For example, take a report designer that uses a component /// base class of ReportElement. You may want to make ReportElement toolbox items enabled only /// when a ReportDesigner is the active designer. To do this, you would add the following /// ToolboxItemFilter attributes to each class: /// /// [ToolboxItemFilter("MyReportFilterString", ToolboxItemFilterType.Require)] /// public class ReportElement : Component {} /// /// [ToolboxItemFilter("MyReportFilterString", ToolboxItemFilterType.Require)] /// public class ReportDesigner : Component {} /// /// These two filters specify that ReportElement toolbox items will only be /// enabled when a ReportDesigner is visible. By specifying a filter type of /// Require on the report designer class, this will disable any toolbox items /// that are not report elements. If the report designer specifed a filter type /// of "Allow" instead of "Require", other components would be enabled when the /// report designer was active. ReportElements would still be disabled when /// other designers were active, however, because ReportElement requires designers /// to have the given filter string. /// /// Toolbox item filtering is a useful way to restrict toolbox item visibility to /// cases where it is appropriate. This can help to avoid confusion for users, but /// you should use caution not to make items unusually restrictive. If you have a /// general purpose component, for example, you should allow the component to appear /// on any designer. /// /// The ASP.NET and Windows Forms designers both use filter attributes to prevent /// each other's components from being enabled. This is a useful restriction because, /// since each has several duplicate class names, it may be confusing to users and /// they may not know which controls to choose. /// /// [ AttributeUsage(AttributeTargets.Class, AllowMultiple=true, Inherited=true), Serializable ] public sealed class ToolboxItemFilterAttribute : Attribute { private ToolboxItemFilterType filterType; private string filterString; private string typeId; ////// Initializes a new ToolboxItemFilterAttribute with the provide filter string and a filter type of /// "Allow". /// public ToolboxItemFilterAttribute(string filterString) : this(filterString, ToolboxItemFilterType.Allow) { } ////// Initializes a new ToolboxItemFilterAttribute with the provide filter string and filter type. /// public ToolboxItemFilterAttribute(string filterString, ToolboxItemFilterType filterType) { if (filterString == null) filterString = String.Empty; this.filterString = filterString; this.filterType = filterType; } ////// Retrieves the filter string for this attribute. The filter string is a user-defined string that /// is used to identify matching attributes. /// public string FilterString { get { return filterString; } } ////// Retrieves the filter type for this attribute. The filter type determines how the filter string should /// be applied. /// public ToolboxItemFilterType FilterType { get { return filterType; } } ////// The unique identifier for this attribute. All ToolboxItemFilterAttributes with the same filter string /// are considered the same, so they return the same TypeId. /// public override object TypeId { get { if (typeId == null) { typeId = GetType().FullName + filterString; } return typeId; } } public override bool Equals(object obj) { if (obj == this) { return true; } ToolboxItemFilterAttribute other = obj as ToolboxItemFilterAttribute; return (other != null && other.FilterType.Equals(FilterType) && other.FilterString.Equals(FilterString)); } public override int GetHashCode() { // No need to hash on filter type as well; there shouldn't be that many duplicates. return filterString.GetHashCode(); } public override bool Match(object obj) { ToolboxItemFilterAttribute other = obj as ToolboxItemFilterAttribute; if (other == null) { return false; } // different filter string kills a match immediately. // if (!other.FilterString.Equals(FilterString)) { return false; } return true; } public override string ToString() { return filterString + "," + Enum.GetName(typeof(ToolboxItemFilterType), filterType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
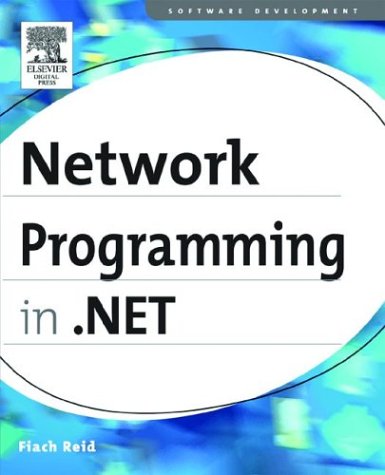
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MembershipPasswordException.cs
- RegexCharClass.cs
- Transactions.cs
- LoadGrammarCompletedEventArgs.cs
- CleanUpVirtualizedItemEventArgs.cs
- AttributeExtensions.cs
- SerializerProvider.cs
- TableHeaderCell.cs
- ShapeTypeface.cs
- DbDataRecord.cs
- CorePropertiesFilter.cs
- AssemblyNameProxy.cs
- Section.cs
- CodeCatchClauseCollection.cs
- DataGridBeginningEditEventArgs.cs
- WebBrowserNavigatingEventHandler.cs
- FontWeightConverter.cs
- EntitySet.cs
- CodeArrayIndexerExpression.cs
- InternalControlCollection.cs
- CodeNamespace.cs
- PathSegmentCollection.cs
- XmlnsPrefixAttribute.cs
- DataGridViewButtonColumn.cs
- ConstNode.cs
- XmlReturnReader.cs
- SoundPlayerAction.cs
- SqlUDTStorage.cs
- SapiRecoContext.cs
- FilterQueryOptionExpression.cs
- MailWebEventProvider.cs
- DocumentViewerAutomationPeer.cs
- ResourceDescriptionAttribute.cs
- SmiEventSink_Default.cs
- SqlGatherConsumedAliases.cs
- FrameworkReadOnlyPropertyMetadata.cs
- SafeRightsManagementEnvironmentHandle.cs
- Propagator.Evaluator.cs
- Point4DValueSerializer.cs
- PropertyInformationCollection.cs
- DesignerCategoryAttribute.cs
- DesignerValidatorAdapter.cs
- ImageCodecInfo.cs
- AsyncCompletedEventArgs.cs
- SelectionChangedEventArgs.cs
- Highlights.cs
- EmptyImpersonationContext.cs
- DbConnectionInternal.cs
- BitmapEffectCollection.cs
- IntPtr.cs
- StylusEditingBehavior.cs
- WebZone.cs
- SectionUpdates.cs
- XmlnsCompatibleWithAttribute.cs
- ConfigurationStrings.cs
- DesignerForm.cs
- NamespaceImport.cs
- CompilationRelaxations.cs
- EntityViewGenerationAttribute.cs
- RepeatButtonAutomationPeer.cs
- RegexCompiler.cs
- DeviceSpecificChoiceCollection.cs
- ColorAnimation.cs
- ResourceType.cs
- RefreshPropertiesAttribute.cs
- ProxyWebPartConnectionCollection.cs
- DeferredTextReference.cs
- CustomSignedXml.cs
- PackageFilter.cs
- EnterpriseServicesHelper.cs
- StreamInfo.cs
- SqlConnectionPoolProviderInfo.cs
- HelpInfo.cs
- SiteIdentityPermission.cs
- BamlTreeMap.cs
- DataFieldConverter.cs
- XmlDictionaryReaderQuotas.cs
- CheckBox.cs
- FixedSOMGroup.cs
- RoutingConfiguration.cs
- ProfilePropertyNameValidator.cs
- DesignerTransaction.cs
- BufferAllocator.cs
- ConnectionStringEditor.cs
- RadioButtonStandardAdapter.cs
- DelegateArgument.cs
- _LazyAsyncResult.cs
- OleTxTransaction.cs
- Nullable.cs
- HttpStreamFormatter.cs
- DataRow.cs
- XPathNodePointer.cs
- Transform.cs
- LocalizationComments.cs
- _Semaphore.cs
- DrawingImage.cs
- MissingMemberException.cs
- DynamicValueConverter.cs
- HtmlInputPassword.cs
- FixedTextContainer.cs