Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / ComponentModel / Design / DesignerVerbCollection.cs / 1 / DesignerVerbCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design { using System; using System.Collections; using System.Diagnostics; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] [System.Runtime.InteropServices.ComVisible(true)] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class DesignerVerbCollection : CollectionBase { ///[To be supplied.] ////// public DesignerVerbCollection() { } ///[To be supplied.] ////// public DesignerVerbCollection(DesignerVerb[] value) { AddRange(value); } ///[To be supplied.] ////// public DesignerVerb this[int index] { get { return (DesignerVerb)(List[index]); } set { List[index] = value; } } ///[To be supplied.] ////// public int Add(DesignerVerb value) { return List.Add(value); } ///[To be supplied.] ////// public void AddRange(DesignerVerb[] value) { if (value == null) { throw new ArgumentNullException("value"); } for (int i = 0; ((i) < (value.Length)); i = ((i) + (1))) { this.Add(value[i]); } } ///[To be supplied.] ////// public void AddRange(DesignerVerbCollection value) { if (value == null) { throw new ArgumentNullException("value"); } int currentCount = value.Count; for (int i = 0; i < currentCount; i = ((i) + (1))) { this.Add(value[i]); } } ///[To be supplied.] ////// public void Insert(int index, DesignerVerb value) { List.Insert(index, value); } ///[To be supplied.] ////// public int IndexOf(DesignerVerb value) { return List.IndexOf(value); } ///[To be supplied.] ////// public bool Contains(DesignerVerb value) { return List.Contains(value); } ///[To be supplied.] ////// public void Remove(DesignerVerb value) { List.Remove(value); } ///[To be supplied.] ////// public void CopyTo(DesignerVerb[] array, int index) { List.CopyTo(array, index); } ///[To be supplied.] ////// protected override void OnSet(int index, object oldValue, object newValue) { } ///[To be supplied.] ////// protected override void OnInsert(int index, object value) { } ///[To be supplied.] ////// protected override void OnClear() { } ///[To be supplied.] ////// protected override void OnRemove(int index, object value) { } ///[To be supplied.] ////// protected override void OnValidate(object value) { Debug.Assert(value != null, "Don't add null verbs!"); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design { using System; using System.Collections; using System.Diagnostics; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] [System.Runtime.InteropServices.ComVisible(true)] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class DesignerVerbCollection : CollectionBase { ///[To be supplied.] ////// public DesignerVerbCollection() { } ///[To be supplied.] ////// public DesignerVerbCollection(DesignerVerb[] value) { AddRange(value); } ///[To be supplied.] ////// public DesignerVerb this[int index] { get { return (DesignerVerb)(List[index]); } set { List[index] = value; } } ///[To be supplied.] ////// public int Add(DesignerVerb value) { return List.Add(value); } ///[To be supplied.] ////// public void AddRange(DesignerVerb[] value) { if (value == null) { throw new ArgumentNullException("value"); } for (int i = 0; ((i) < (value.Length)); i = ((i) + (1))) { this.Add(value[i]); } } ///[To be supplied.] ////// public void AddRange(DesignerVerbCollection value) { if (value == null) { throw new ArgumentNullException("value"); } int currentCount = value.Count; for (int i = 0; i < currentCount; i = ((i) + (1))) { this.Add(value[i]); } } ///[To be supplied.] ////// public void Insert(int index, DesignerVerb value) { List.Insert(index, value); } ///[To be supplied.] ////// public int IndexOf(DesignerVerb value) { return List.IndexOf(value); } ///[To be supplied.] ////// public bool Contains(DesignerVerb value) { return List.Contains(value); } ///[To be supplied.] ////// public void Remove(DesignerVerb value) { List.Remove(value); } ///[To be supplied.] ////// public void CopyTo(DesignerVerb[] array, int index) { List.CopyTo(array, index); } ///[To be supplied.] ////// protected override void OnSet(int index, object oldValue, object newValue) { } ///[To be supplied.] ////// protected override void OnInsert(int index, object value) { } ///[To be supplied.] ////// protected override void OnClear() { } ///[To be supplied.] ////// protected override void OnRemove(int index, object value) { } ///[To be supplied.] ////// protected override void OnValidate(object value) { Debug.Assert(value != null, "Don't add null verbs!"); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
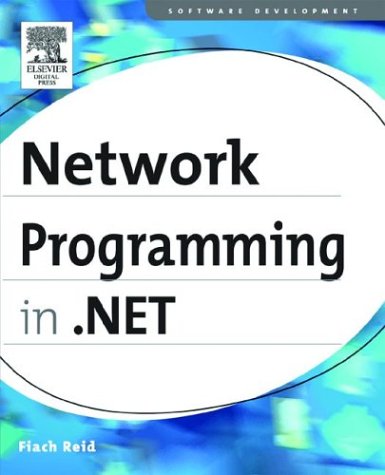
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSiteMapProvider.cs
- BaseWebProxyFinder.cs
- AdvancedBindingEditor.cs
- validationstate.cs
- FileUtil.cs
- WindowsEditBoxRange.cs
- DesignerView.Commands.cs
- AuthenticatedStream.cs
- GeneralEndpointIdentity.cs
- ModifierKeysConverter.cs
- coordinatorscratchpad.cs
- HttpListenerContext.cs
- WsdlBuildProvider.cs
- FontStyleConverter.cs
- httpstaticobjectscollection.cs
- BitmapDecoder.cs
- PropertyGrid.cs
- FastEncoderWindow.cs
- ConfigUtil.cs
- GiveFeedbackEventArgs.cs
- StorageEndPropertyMapping.cs
- RequestCachePolicy.cs
- FormsAuthenticationModule.cs
- TimeSpanMinutesConverter.cs
- DataTrigger.cs
- MouseWheelEventArgs.cs
- ReadOnlyCollection.cs
- DriveInfo.cs
- SHA384Cng.cs
- FlowDocumentPaginator.cs
- ManipulationDevice.cs
- XmlLangPropertyAttribute.cs
- EntityDataSourceContextCreatingEventArgs.cs
- HttpConfigurationContext.cs
- FontNamesConverter.cs
- _NetworkingPerfCounters.cs
- SymbolPair.cs
- IPEndPointCollection.cs
- UICuesEvent.cs
- HtmlContainerControl.cs
- CreateUserWizard.cs
- Matrix3DConverter.cs
- EncryptedPackage.cs
- Profiler.cs
- CommandPlan.cs
- SimpleLine.cs
- Point3DAnimationUsingKeyFrames.cs
- SystemFonts.cs
- QueryCorrelationInitializer.cs
- WebPartUtil.cs
- SqlGenerator.cs
- DataViewListener.cs
- CompositeDataBoundControl.cs
- TraceContext.cs
- TextRange.cs
- MethodAccessException.cs
- DecoderExceptionFallback.cs
- ForeignKeyConstraint.cs
- ColumnWidthChangedEvent.cs
- XmlCDATASection.cs
- RuleInfoComparer.cs
- BooleanFunctions.cs
- UserControlBuildProvider.cs
- ConfigurationStrings.cs
- Socket.cs
- ComponentCache.cs
- DbException.cs
- TaskResultSetter.cs
- EnterpriseServicesHelper.cs
- SpeechUI.cs
- ElapsedEventArgs.cs
- SimpleType.cs
- SafeThreadHandle.cs
- WebPartActionVerb.cs
- ObjectTypeMapping.cs
- SymLanguageVendor.cs
- HtmlElementEventArgs.cs
- ErrorHandlerFaultInfo.cs
- FrameworkElementFactory.cs
- DataGridColumnHeader.cs
- DataGridViewCheckBoxCell.cs
- VirtualDirectoryMapping.cs
- QueueTransferProtocol.cs
- LinkDescriptor.cs
- SiteMapNode.cs
- SQLSingleStorage.cs
- ScriptControl.cs
- OLEDB_Util.cs
- ComboBox.cs
- TogglePattern.cs
- DataControlLinkButton.cs
- DrawingCollection.cs
- SqlClientPermission.cs
- WebPartManager.cs
- InternalBufferOverflowException.cs
- XmlSchemaAnnotation.cs
- RepeatInfo.cs
- BooleanKeyFrameCollection.cs
- XPathDocumentIterator.cs
- AnimationClock.cs