Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Compilation / WsdlBuildProvider.cs / 1 / WsdlBuildProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Globalization; using System.IO; using System.Diagnostics; using System.Web.Services.Description; using System.Xml; using System.Xml.Serialization; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Configuration; using System.Web.Hosting; using System.Web.Util; using Util=System.Web.UI.Util; [BuildProviderAppliesTo(BuildProviderAppliesTo.Code)] internal class WsdlBuildProvider: BuildProvider { public override void GenerateCode(AssemblyBuilder assemblyBuilder) { // Get the namespace that we will use string ns = Util.GetNamespaceFromVirtualPath(VirtualPathObject); ServiceDescription sd; // Load the wsdl file using (Stream stream = VirtualPathObject.OpenFile()) { try { sd = ServiceDescription.Read(stream); } catch (InvalidOperationException e) { // It can throw an InvalidOperationException, with the relevant // XmlException as the inner exception. If so, throw that instead. XmlException xmlException = e.InnerException as XmlException; if (xmlException != null) throw xmlException; throw; } } ServiceDescriptionImporter importer = new ServiceDescriptionImporter(); #if !FEATURE_PAL importer.CodeGenerator = assemblyBuilder.CodeDomProvider; importer.CodeGenerationOptions = CodeGenerationOptions.GenerateProperties | CodeGenerationOptions.GenerateNewAsync | CodeGenerationOptions.GenerateOldAsync; #endif // !FEATURE_PAL importer.ServiceDescriptions.Add(sd); CodeCompileUnit codeCompileUnit = new CodeCompileUnit(); CodeNamespace codeNamespace = new CodeNamespace(ns); codeCompileUnit.Namespaces.Add(codeNamespace); // Create the code compile unit importer.Import(codeNamespace, codeCompileUnit); // Add the CodeCompileUnit to the compilation assemblyBuilder.AddCodeCompileUnit(this, codeCompileUnit); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Globalization; using System.IO; using System.Diagnostics; using System.Web.Services.Description; using System.Xml; using System.Xml.Serialization; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Configuration; using System.Web.Hosting; using System.Web.Util; using Util=System.Web.UI.Util; [BuildProviderAppliesTo(BuildProviderAppliesTo.Code)] internal class WsdlBuildProvider: BuildProvider { public override void GenerateCode(AssemblyBuilder assemblyBuilder) { // Get the namespace that we will use string ns = Util.GetNamespaceFromVirtualPath(VirtualPathObject); ServiceDescription sd; // Load the wsdl file using (Stream stream = VirtualPathObject.OpenFile()) { try { sd = ServiceDescription.Read(stream); } catch (InvalidOperationException e) { // It can throw an InvalidOperationException, with the relevant // XmlException as the inner exception. If so, throw that instead. XmlException xmlException = e.InnerException as XmlException; if (xmlException != null) throw xmlException; throw; } } ServiceDescriptionImporter importer = new ServiceDescriptionImporter(); #if !FEATURE_PAL importer.CodeGenerator = assemblyBuilder.CodeDomProvider; importer.CodeGenerationOptions = CodeGenerationOptions.GenerateProperties | CodeGenerationOptions.GenerateNewAsync | CodeGenerationOptions.GenerateOldAsync; #endif // !FEATURE_PAL importer.ServiceDescriptions.Add(sd); CodeCompileUnit codeCompileUnit = new CodeCompileUnit(); CodeNamespace codeNamespace = new CodeNamespace(ns); codeCompileUnit.Namespaces.Add(codeNamespace); // Create the code compile unit importer.Import(codeNamespace, codeCompileUnit); // Add the CodeCompileUnit to the compilation assemblyBuilder.AddCodeCompileUnit(this, codeCompileUnit); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
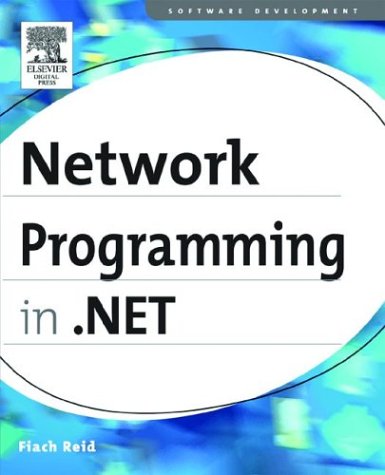
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextElementEditingBehaviorAttribute.cs
- RefreshEventArgs.cs
- ISAPIRuntime.cs
- WebConfigurationHostFileChange.cs
- TreeNodeStyleCollection.cs
- WebPartZoneBase.cs
- DataGridViewDataConnection.cs
- UdpDiscoveryEndpointProvider.cs
- ResourceReader.cs
- FocusTracker.cs
- ServiceSecurityContext.cs
- SpeechRecognitionEngine.cs
- BitFlagsGenerator.cs
- BitmapInitialize.cs
- OracleSqlParser.cs
- OleDbPropertySetGuid.cs
- Vector3DValueSerializer.cs
- ToolBarPanel.cs
- InheritanceRules.cs
- TempFiles.cs
- XmlSerializerVersionAttribute.cs
- EmbossBitmapEffect.cs
- XhtmlBasicImageAdapter.cs
- ProcessHostFactoryHelper.cs
- JapaneseLunisolarCalendar.cs
- _PooledStream.cs
- DataGridCellAutomationPeer.cs
- DocComment.cs
- TypeUtil.cs
- ListBoxAutomationPeer.cs
- ContainsRowNumberChecker.cs
- GridViewColumn.cs
- MemberExpression.cs
- UrlPath.cs
- ReturnType.cs
- Invariant.cs
- ResourceDisplayNameAttribute.cs
- WhitespaceSignificantCollectionAttribute.cs
- RuntimeIdentifierPropertyAttribute.cs
- DoubleLinkList.cs
- VideoDrawing.cs
- StatusBarPanelClickEvent.cs
- MatrixIndependentAnimationStorage.cs
- _BasicClient.cs
- PermissionListSet.cs
- BooleanAnimationBase.cs
- ParameterExpression.cs
- FrameworkContentElementAutomationPeer.cs
- ClientSideProviderDescription.cs
- LabelAutomationPeer.cs
- StandardCommands.cs
- ArraySortHelper.cs
- ToolStripScrollButton.cs
- InfocardChannelParameter.cs
- InkCanvas.cs
- ConfigXmlSignificantWhitespace.cs
- Imaging.cs
- WorkerRequest.cs
- AssociationSetMetadata.cs
- ImageSource.cs
- CompareInfo.cs
- SystemInfo.cs
- StorageMappingItemLoader.cs
- Point3DCollectionValueSerializer.cs
- HtmlTableCellCollection.cs
- ZoneLinkButton.cs
- SplineKeyFrames.cs
- LocalBuilder.cs
- MenuRendererStandards.cs
- ClusterSafeNativeMethods.cs
- DataExpression.cs
- Comparer.cs
- TransformGroup.cs
- HostSecurityManager.cs
- DecodeHelper.cs
- ConnectionStringSettings.cs
- PlainXmlWriter.cs
- PropertyExpression.cs
- ColumnBinding.cs
- UInt64.cs
- Int32CollectionConverter.cs
- CodeMethodInvokeExpression.cs
- _ListenerResponseStream.cs
- SafeNativeMethodsMilCoreApi.cs
- _UriTypeConverter.cs
- MetadataItemCollectionFactory.cs
- CrossContextChannel.cs
- CompModHelpers.cs
- PartialCachingAttribute.cs
- XmlDesignerDataSourceView.cs
- WorkflowOwnerAsyncResult.cs
- ScriptControlManager.cs
- DataGridViewSelectedRowCollection.cs
- SelectionRange.cs
- Stroke2.cs
- X500Name.cs
- SettingsAttributeDictionary.cs
- DbModificationCommandTree.cs
- ProviderCommandInfoUtils.cs
- SamlAdvice.cs